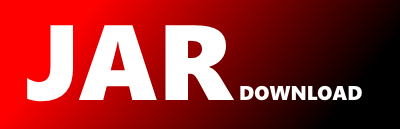
com.denimgroup.threadfix.data.entities.PendingScan Maven / Gradle / Ivy
package com.denimgroup.threadfix.data.entities;
import com.denimgroup.threadfix.data.enums.PendingScanStatus;
import com.denimgroup.threadfix.data.enums.ScanStatusType;
import com.denimgroup.threadfix.views.AllViews;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonView;
import javax.persistence.*;
import javax.validation.constraints.Size;
import java.util.Date;
import java.util.List;
import static com.denimgroup.threadfix.data.enums.PendingScanStatus.UPLOADED;
import static com.denimgroup.threadfix.data.enums.ScanStatusType.UPLOAD;
/**
* Created by jtomsett on 11/22/16.
*/
@Entity
@Table
public class PendingScan extends AuditableEntity {
private static final int ERROR_LENGTH = 1024;
private List fileDataList;
private Boolean isBulkScans;
private Integer appId;
private Integer orgId;
private Integer remoteProviderId;
private Integer channelId;
private String errorMessage;
private Boolean processed = false;
private PendingScanStatus status = UPLOADED;
private Boolean error = false;
private Integer userId;
private Integer scanId;
private String type;
public PendingScan(){}
public PendingScan(ScanStatusType scanStatusType) {
this.type = scanStatusType.toString();
}
@Transient
@JsonView({Object.class, AllViews.RestViewRemoteProviderApplicationStatus.class})
public Date getTime() {
return super.getCreatedDate();
}
@OneToMany(mappedBy = "pendingScan", cascade = CascadeType.ALL, fetch = FetchType.EAGER)
@JsonView({Object.class, AllViews.RestViewRemoteProviderApplicationStatus.class})
public List getFileDataList() {
return fileDataList;
}
public void setFileDataList(List fileDataList) {
this.fileDataList = fileDataList;
}
@Column
@JsonIgnore
public boolean isBulkScans() {
return isBulkScans == null ? false : isBulkScans;
}
public void setBulkScans(boolean bulkScans) {
isBulkScans = bulkScans;
}
@Column
@JsonView({Object.class, AllViews.RestViewRemoteProviderApplicationStatus.class})
public Integer getAppId() {
return appId;
}
public void setAppId(Integer appId) {
this.appId = appId;
}
@Column
@JsonIgnore
public Integer getOrgId() {
return orgId;
}
public void setOrgId(Integer orgId) {
this.orgId = orgId;
}
@Column
@JsonIgnore
public Integer getChannelId() {
return channelId;
}
public void setChannelId(Integer channelId) {
this.channelId = channelId;
}
@Column
@Size(max = ERROR_LENGTH, message = "{errors.maxlength} " + ERROR_LENGTH + ".")
@JsonView({Object.class, AllViews.RestViewRemoteProviderApplicationStatus.class})
public String getErrorMessage() {
return errorMessage;
}
public void setErrorMessage(String errorMessage) {
if (errorMessage != null && errorMessage.length() > ERROR_LENGTH) {
errorMessage = errorMessage.substring(0, ERROR_LENGTH);
}
this.errorMessage = errorMessage;
}
@Column
@JsonIgnore
public Boolean isProcessed() {
return processed;
}
public void setProcessed(Boolean processed) {
this.processed = processed;
}
@Column
@JsonView({Object.class, AllViews.RestViewRemoteProviderApplicationStatus.class})
public PendingScanStatus getStatus() {
return status;
}
public void setStatus(PendingScanStatus status) {
this.status = status;
}
@Column
@JsonView({Object.class, AllViews.RestViewRemoteProviderApplicationStatus.class})
public Boolean isError() {
return error == null ? false : error;
}
public void setError(Boolean error) {
this.error = error;
}
@Column
@JsonView({Object.class, AllViews.RestViewRemoteProviderApplicationStatus.class})
public String getType() {
return type != null ? type : UPLOAD.toString();
}
public void setType(String type) {
this.type = type;
}
@Column
@JsonIgnore
public Integer getRemoteProviderId() {
return remoteProviderId;
}
public void setRemoteProviderId(Integer remoteProviderId) {
this.remoteProviderId = remoteProviderId;
}
@Column
@JsonIgnore
public Integer getUserId() {
return userId;
}
public void setUserId(Integer userId) {
this.userId = userId;
}
@Column
@JsonIgnore
public Integer getScanId() {
return scanId;
}
public void setScanId(Integer scanId) {
this.scanId = scanId;
}
@Transient
@JsonView({Object.class})
public String getName() {
String name = "";
List fileData = getFileDataList();
if (fileData != null && fileData.size() > 0) {
name = fileData.get(0).getOriginalName();
}
return name;
}
@Transient
@JsonView({Object.class})
public String getScanMessage() {
return getStatus().toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy