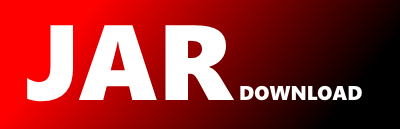
com.denimgroup.threadfix.data.entities.Version Maven / Gradle / Ivy
package com.denimgroup.threadfix.data.entities;
import com.denimgroup.threadfix.util.IntegerUtils;
/**
* Created by jtomsett on 5/20/2016.
*/
public class Version implements Comparable{
private static final String INVALID_VERSION = "INVALID_VERSION";
private String versionString;
private Integer major;
private Integer minor;
private Integer build;
private Integer revision;
public Version(String versionString){
setVersionString(versionString);
major = 0;
minor = 0;
build = 0;
revision = 0;
buildVersion();
}
public String getVersionString() {
return versionString;
}
public void setVersionString(String versionString) {
this.versionString = versionString.trim();
}
public Integer getMajor() {
return major;
}
public void setMajor(Integer major) {
this.major = major;
}
public Integer getMinor() {
return minor;
}
public void setMinor(Integer minor) {
this.minor = minor;
}
public Integer getBuild() {
return build;
}
public void setBuild(Integer build) {
this.build = build;
}
public Integer getRevision() {
return revision;
}
public void setRevision(Integer revision) {
this.revision = revision;
}
public static String getInvalidVersion() {
return INVALID_VERSION;
}
public int compareTo(Version otherVersion){
if(otherVersion.getMajor() > major){
/**
* otherVersion has a greater Major version number
*/
return -1;
}else if(otherVersion.getMajor() == major){
if(otherVersion.getMinor() > minor){
/**
* otherVersion has the same Major version number
* otherVersion has a greater Minor Version number
*/
return -1;
}else if(otherVersion.getMinor() == minor){
if(otherVersion.getBuild() > build){
/**
* otherVersion has the same Major version number
* otherVersion has the same Minor version number
* otherVersion has a greater build version number
*/
return -1;
}else if(otherVersion.getBuild() == build){
if(otherVersion.getRevision() > revision){
/**
* otherVersion has the same Major version number
* otherVersion has the same Minor version number
* otherVersion has the same build version number
* otherVersion has a greater revision version number
*/
return -1;
}else if(otherVersion.getRevision() == revision){
/**
* otherVersion equals this version
*/
return 0;
}
/**
* otherVersion has the same Major version number
* otherVersion has the same Minor version number
* otherVersion has the same build version number
* this Version has a greater revision version number
*/
return 1;
}
/**
* otherVersion has the same Major version number
* otherVersion has the same Minor version number
* this version has a greater build version number
*/
return 1;
}
/**
* otherVersion has the same Major version number
* this version has a greater Minor version number
*/
return 1;
}
/**
* this version has a greater Major version number
*/
return 1;
}
public void buildVersion(){
String[] versionParts = versionString.split("\\.");
if (versionParts.length == 0) {
versionString = INVALID_VERSION;
}else {
for (int i = 0; i < versionParts.length; i++) {
if (i > 3) {
break;
}
if (i == 0) {
major = IntegerUtils.getIntegerOrNull(versionParts[i]);
if (major == null) {
versionString = INVALID_VERSION;
break;
}
}
if (i == 1) {
minor = IntegerUtils.getIntegerOrNull(versionParts[i]);
if (minor == null) {
versionString = INVALID_VERSION;
break;
}
}
if (i == 2) {
build = IntegerUtils.getIntegerOrNull(versionParts[i]);
if (build == null) {
versionString = INVALID_VERSION;
break;
}
}
if (i == 3) {
revision = IntegerUtils.getIntegerOrNull(versionParts[i]);
if (revision == null) {
versionString = INVALID_VERSION;
break;
}
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy