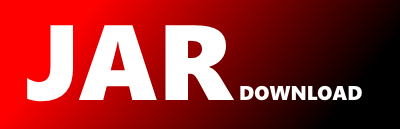
com.denimgroup.threadfix.data.entities.VulnerabilitySearchParameters Maven / Gradle / Ivy
////////////////////////////////////////////////////////////////////////
//
// Copyright (c) 2009-2016 Denim Group, Ltd.
//
// The contents of this file are subject to the Mozilla Public License
// Version 2.0 (the "License"); you may not use this file except in
// compliance with the License. You may obtain a copy of the License at
// http://www.mozilla.org/MPL/
//
// Software distributed under the License is distributed on an "AS IS"
// basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See the
// License for the specific language governing rights and limitations
// under the License.
//
// The Original Code is ThreadFix.
//
// The Initial Developer of the Original Code is Denim Group, Ltd.
// Portions created by Denim Group, Ltd. are Copyright (C)
// Denim Group, Ltd. All Rights Reserved.
//
// Contributor(s): Denim Group, Ltd.
//
////////////////////////////////////////////////////////////////////////
package com.denimgroup.threadfix.data.entities;
import com.denimgroup.threadfix.views.AllViews;
import com.fasterxml.jackson.annotation.JsonView;
import javax.persistence.Column;
import java.util.Date;
import java.util.List;
import static com.denimgroup.threadfix.CollectionUtils.list;
/**
* Created by mac on 5/7/14.
*/
public class VulnerabilitySearchParameters {
List genericVulnerabilities = list();
List teams = list();
List applications = list();
List genericSeverities = list();
List channelTypes = list();
List ascList = list();
List descList = list();
List tags = list();
List vulnTags = list();
List commentTags = list();
List permissionsList = list();
Integer numberVulnerabilities = 10;
Integer page = 1;
String daysOldModifier = null, parameter = null, path = null, defectId = null, nativeId = null;
Integer daysOld = null, numberMerged = null;
Boolean showOpen = null, showClosed = null, showFalsePositive = null, showHidden = null,
showDefectPresent = null, showDefectNotPresent = null, showDefectOpen = null, showDefectClosed = null,
showInconsistentClosedDefectNeedsScan = null, showInconsistentClosedDefectOpenInScan = null, showInconsistentOpenDefect = null,
usingComponentsWithKnownVulnerabilities = null, showUnknown = null, showAuthenticated = null, showUnauthenticated = null,
showCommentPresent = null, showSharedVulnFound = false, showSharedVulnNotFound = false;
Date startDate = null, endDate = null;
Date startCloseDate = null, endCloseDate = null;
String searchAppText = null;
String primaryPivotName = null;
String secondaryPivotName = null;
String assignToUser = null;
VulnerabilitySearchPivotType primaryPivot = VulnerabilitySearchPivotType.SEVERITY;
VulnerabilitySearchPivotType secondaryPivot = VulnerabilitySearchPivotType.CWE;
@JsonView({ AllViews.VulnSearchApplications.class })
public Integer getPage() {
return page;
}
public void setPage(Integer page) {
this.page = page;
}
@Column
@JsonView({ AllViews.VulnSearchApplications.class })
public Integer getNumberVulnerabilities() {
return numberVulnerabilities;
}
public void setNumberVulnerabilities(Integer numberVulnerabilities) {
this.numberVulnerabilities = numberVulnerabilities;
}
@JsonView({ AllViews.VulnSearchApplications.class })
public List getGenericSeverities() {
return genericSeverities;
}
public void setGenericSeverities(List genericSeverities) {
this.genericSeverities = genericSeverities;
}
@JsonView({ AllViews.VulnSearchApplications.class })
public List getApplications() {
return applications;
}
public void setApplications(List applications) {
this.applications = applications;
}
@JsonView({ AllViews.VulnSearchApplications.class })
public List getTeams() {
return teams;
}
public void setTeams(List teams) {
this.teams = teams;
}
public List getChannelTypes() {
return channelTypes;
}
public void setChannelTypes(List channelTypes) {
this.channelTypes = channelTypes;
}
@JsonView({ AllViews.VulnSearchApplications.class })
public List getGenericVulnerabilities() {
return genericVulnerabilities;
}
public void setGenericVulnerabilities(List genericVulnerabilities) {
this.genericVulnerabilities = genericVulnerabilities;
}
public String getDaysOldModifier() {
return daysOldModifier;
}
public void setDaysOldModifier(String daysOldModifier) {
this.daysOldModifier = daysOldModifier;
}
public Integer getDaysOld() {
return daysOld;
}
public void setDaysOld(Integer daysOld) {
this.daysOld = daysOld;
}
public String getParameter() {
return parameter;
}
public void setParameter(String parameter) {
this.parameter = parameter;
}
public String getNativeId() {
return nativeId;
}
public void setNativeId(String nativeId) {
this.nativeId = nativeId;
}
public String getPath() {
return path;
}
public void setPath(String path) {
this.path = path;
}
public String getDefectId() {
return defectId;
}
public void setDefectId(String defectId) {
this.defectId = defectId;
}
public Integer getNumberMerged() {
return numberMerged;
}
public void setNumberMerged(Integer numberMerged) {
this.numberMerged = numberMerged;
}
@JsonView({ AllViews.VulnSearchApplications.class })
public Boolean getShowOpen() {
return showOpen;
}
public void setShowOpen(Boolean showOpen) {
this.showOpen = showOpen;
}
@JsonView({ AllViews.VulnSearchApplications.class })
public Boolean getShowClosed() {
return showClosed;
}
public void setShowClosed(Boolean showClosed) {
this.showClosed = showClosed;
}
@JsonView({ AllViews.VulnSearchApplications.class })
public Boolean getShowFalsePositive() {
return showFalsePositive;
}
public void setShowFalsePositive(Boolean showFalsePositive) {
this.showFalsePositive = showFalsePositive;
}
@JsonView({ AllViews.VulnSearchApplications.class })
public Boolean getShowHidden() {
return showHidden;
}
public void setShowHidden(Boolean showHidden) {
this.showHidden = showHidden;
}
public Boolean getShowDefectPresent() {
return showDefectPresent;
}
public void setShowDefectPresent(Boolean showDefectPresent) {
this.showDefectPresent = showDefectPresent;
}
public Boolean getShowDefectNotPresent() {
return showDefectNotPresent;
}
public void setShowDefectNotPresent(Boolean showDefectNotPresent) {
this.showDefectNotPresent = showDefectNotPresent;
}
public Boolean getShowDefectOpen() {
return showDefectOpen;
}
public void setShowDefectOpen(Boolean showDefectOpen) {
this.showDefectOpen = showDefectOpen;
}
public Boolean getShowDefectClosed() {
return showDefectClosed;
}
public void setShowDefectClosed(Boolean showDefectClosed) {
this.showDefectClosed = showDefectClosed;
}
public Boolean getShowInconsistentClosedDefectNeedsScan() {
return showInconsistentClosedDefectNeedsScan;
}
public void setShowInconsistentClosedDefectNeedsScan(Boolean showInconsistentClosedDefectNeedsScan) {
this.showInconsistentClosedDefectNeedsScan = showInconsistentClosedDefectNeedsScan;
}
public Boolean getShowInconsistentClosedDefectOpenInScan() {
return showInconsistentClosedDefectOpenInScan;
}
public void setShowInconsistentClosedDefectOpenInScan(Boolean showInconsistentClosedDefectOpenInScan) {
this.showInconsistentClosedDefectOpenInScan = showInconsistentClosedDefectOpenInScan;
}
public Boolean getShowInconsistentOpenDefect() {
return showInconsistentOpenDefect;
}
public void setShowInconsistentOpenDefect(Boolean showInconsistentOpenDefect) {
this.showInconsistentOpenDefect = showInconsistentOpenDefect;
}
@JsonView({ AllViews.VulnSearchApplications.class })
public Date getStartDate() {
return startDate;
}
public void setStartDate(Date startDate) {
this.startDate = startDate;
}
@JsonView({ AllViews.VulnSearchApplications.class })
public Date getEndDate() {
return endDate;
}
public void setEndDate(Date endDate) {
this.endDate = endDate;
}
@JsonView({ AllViews.VulnSearchApplications.class })
public Date getStartCloseDate() {
return startCloseDate;
}
public void setStartCloseDate(Date startCloseDate) {
this.startCloseDate = startCloseDate;
}
@JsonView({ AllViews.VulnSearchApplications.class })
public Date getEndCloseDate() {
return endCloseDate;
}
public void setEndCloseDate(Date endCloseDate) {
this.endCloseDate = endCloseDate;
}
public List getAscList() {
return ascList;
}
public void setAscList(List ascList) {
this.ascList = ascList;
}
public List getDescList() {
return descList;
}
public void setDescList(List descList) {
this.descList = descList;
}
@JsonView({ AllViews.VulnSearchApplications.class })
public List getTags() {
return tags;
}
public void setTags(List tags) {
this.tags = tags;
}
@JsonView({ AllViews.VulnSearchApplications.class })
public List getVulnTags() {
return vulnTags;
}
public void setVulnTags(List vulnTags) {
this.vulnTags = vulnTags;
}
@JsonView({ AllViews.VulnSearchApplications.class })
public List getCommentTags() {
return commentTags;
}
public void setCommentTags(List commentTags) {
this.commentTags = commentTags;
}
@JsonView({ AllViews.VulnSearchApplications.class })
public Boolean getUsingComponentsWithKnownVulnerabilities() {
return usingComponentsWithKnownVulnerabilities;
}
public void setUsingComponentsWithKnownVulnerabilities(Boolean usingComponentsWithKnownVulnerabilities) {
this.usingComponentsWithKnownVulnerabilities = usingComponentsWithKnownVulnerabilities;
}
@JsonView({ AllViews.VulnSearchApplications.class })
public List getPermissionsList() {
return permissionsList;
}
public void setPermissionsList(List permissionsList) {
this.permissionsList = permissionsList;
}
public Boolean getShowUnknown() {
return showUnknown != null && showUnknown;
}
public void setShowUnknown(Boolean showUnknown) {
this.showUnknown = showUnknown;
}
public Boolean getShowAuthenticated() {
return showAuthenticated != null && showAuthenticated;
}
public void setShowAuthenticated(Boolean showAuthenticated) {
this.showAuthenticated = showAuthenticated;
}
public Boolean getShowUnauthenticated() {
return showUnauthenticated != null && showUnauthenticated;
}
public void setShowUnauthenticated(Boolean showUnauthenticated) {
this.showUnauthenticated = showUnauthenticated;
}
public Boolean getShowCommentPresent() {
return showCommentPresent;
}
public void setShowCommentPresent(Boolean showCommentPresent) {
this.showCommentPresent = showCommentPresent;
}
public Boolean getShowSharedVulnFound() {
return showSharedVulnFound;
}
public void setShowSharedVulnFound(Boolean showSharedVulnFound) {
this.showSharedVulnFound = showSharedVulnFound;
}
public Boolean getShowSharedVulnNotFound() {
return showSharedVulnNotFound;
}
public void setShowSharedVulnNotFound(Boolean showSharedVulnNotFound) {
this.showSharedVulnNotFound = showSharedVulnNotFound;
}
public String getSearchAppText() {
return searchAppText;
}
public void setSearchAppText(String searchAppText) {
this.searchAppText = searchAppText;
}
@JsonView({ AllViews.VulnSearchApplications.class })
public VulnerabilitySearchPivotType getPrimaryPivot() {
return primaryPivot;
}
public void setPrimaryPivot(VulnerabilitySearchPivotType primaryPivot) {
this.primaryPivot = primaryPivot;
}
@JsonView({ AllViews.VulnSearchApplications.class })
public VulnerabilitySearchPivotType getSecondaryPivot() {
return secondaryPivot;
}
public void setSecondaryPivot(VulnerabilitySearchPivotType secondaryPivot) {
this.secondaryPivot = secondaryPivot;
}
@JsonView({ AllViews.VulnSearchApplications.class })
public String getPrimaryPivotName() {
return primaryPivotName;
}
public void setPrimaryPivotName(String primaryPivotName) {
this.primaryPivotName = primaryPivotName;
}
@JsonView({ AllViews.VulnSearchApplications.class })
public String getSecondaryPivotName() {
return secondaryPivotName;
}
public void setSecondaryPivotName(String secondaryPivotName) {
this.secondaryPivotName = secondaryPivotName;
}
@JsonView({ AllViews.VulnSearchApplications.class })
public String getAssignToUser() {
return assignToUser;
}
public void setAssignToUser(String assignToUser) {
this.assignToUser = assignToUser;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy