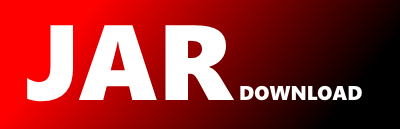
com.denimgroup.threadfix.data.entities.cicd.CICDDecision Maven / Gradle / Ivy
////////////////////////////////////////////////////////////////////////
//
// Copyright (c) 2009-2016 Denim Group, Ltd.
//
// The contents of this file are subject to the Mozilla Public License
// Version 2.0 (the "License"); you may not use this file except in
// compliance with the License. You may obtain a copy of the License at
// http://www.mozilla.org/MPL/
//
// Software distributed under the License is distributed on an "AS IS"
// basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See the
// License for the specific language governing rights and limitations
// under the License.
//
// The Original Code is ThreadFix.
//
// The Initial Developer of the Original Code is Denim Group, Ltd.
// Portions created by Denim Group, Ltd. are Copyright (C)
// Denim Group, Ltd. All Rights Reserved.
//
// Contributor(s): Denim Group, Ltd.
//
////////////////////////////////////////////////////////////////////////
package com.denimgroup.threadfix.data.entities.cicd;
import com.denimgroup.threadfix.data.entities.Application;
import com.denimgroup.threadfix.data.entities.GenericSeverity;
import com.denimgroup.threadfix.views.AllViews;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonView;
import javax.persistence.*;
import java.util.List;
import static com.denimgroup.threadfix.CollectionUtils.list;
/**
* Object used to hold the info present in the disallow element
*
*/
//TODO update entity name to Pass Criteria
@Entity
@Table(name = "PassCriteria")
public class CICDDecision extends AbstractCICDPolicySectionItem {
private List applications = list();
private GenericSeverity genericSeverity;
/**
* Field used to hold the String value in the maxAllowed attribute.
*/
private Integer maxAllowed = null;
/**
* Filed used to hold the String value in the maxIntroduced attribute.
*/
private Integer maxIntroduced = null;
@OneToOne
@JoinColumn(name = "genericSeverityId", foreignKey = @ForeignKey(name="fk_CICDPolicyFilter_GenericSeverity"))
@JsonView({ AllViews.RestViewTag.class, AllViews.TableRow.class, AllViews.RestViewApplication2_4.class })
public GenericSeverity getGenericSeverity() {
return genericSeverity;
}
public void setGenericSeverity(GenericSeverity genericSeverity) {
this.genericSeverity = genericSeverity;
}
/**
* Getter for the maxAllowed field.
* @return The Integer value of the maxAllowed field.
*/
@JsonView({Object.class,AllViews.RestView.class})
@Column
public Integer getMaxAllowed() {
return maxAllowed;
}
/**
* Setter for the maxAllowed field
* @param maxAllowed The value to set the maxAllowed field to.
*/
public void setMaxAllowed(Integer maxAllowed) {
this.maxAllowed = maxAllowed;
}
/**
* Getter for the maxIntroduced field
* @return The value stored in the maxIntroduced field.
*/
@JsonView({Object.class,AllViews.RestView.class})
@Column
public Integer getMaxIntroduced() {
return maxIntroduced;
}
/**
* Setter for the maxIntroduced field.
* @param maxIntroduced The value to set the maxIntroduced field to.
*/
public void setMaxIntroduced(Integer maxIntroduced) {
this.maxIntroduced = maxIntroduced;
}
@Transient
@JsonIgnore
public String getSortValue() {
return genericSeverity != null ? genericSeverity.getDisplayName() : "";
}
@Transient
@JsonView({Object.class,AllViews.RestView.class})
public String getSeverity(){
if(genericSeverity == null){
return "NONE";
}
return genericSeverity.getDisplayName();
}
@ManyToMany
@JoinTable(name = "PolicyFilterApplicationMap",
joinColumns = {@JoinColumn(name = "policyFilterId", foreignKey = @ForeignKey(name = "fk_policy_filter_cicd_decision_policy_filter_id"))},
inverseJoinColumns = { @JoinColumn(name = "applicationId", foreignKey = @ForeignKey(name = "fk_application_cicd_decision_application_id"))})
@JsonView({ AllViews.RestViewTag.class, AllViews.TableRow.class, AllViews.RestViewApplication2_4.class,AllViews.RestView.class })
public List getApplications() {
if (this.applications == null) {
return list();
}
return this.applications;
}
public void setApplications(List applications) {
this.applications = applications;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy