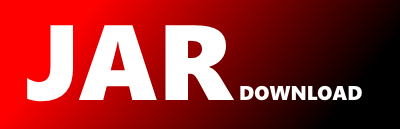
com.denimgroup.threadfix.data.entities.cicd.CICDTest Maven / Gradle / Ivy
////////////////////////////////////////////////////////////////////////
//
// Copyright (c) 2009-2016 Denim Group, Ltd.
//
// The contents of this file are subject to the Mozilla Public License
// Version 2.0 (the "License"); you may not use this file except in
// compliance with the License. You may obtain a copy of the License at
// http://www.mozilla.org/MPL/
//
// Software distributed under the License is distributed on an "AS IS"
// basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See the
// License for the specific language governing rights and limitations
// under the License.
//
// The Original Code is ThreadFix.
//
// The Initial Developer of the Original Code is Denim Group, Ltd.
// Portions created by Denim Group, Ltd. are Copyright (C)
// Denim Group, Ltd. All Rights Reserved.
//
// Contributor(s): Denim Group, Ltd.
//
////////////////////////////////////////////////////////////////////////
package com.denimgroup.threadfix.data.entities.cicd;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.Table;
import javax.persistence.Transient;
/**
* Object used to store the data contained in the test xml element.
*
*/
@Entity
@Table(name = "CICDTestConfig")
public class CICDTest extends AbstractCICDPolicySectionItem {
private final static int CONFIG_LENGTH = 1024;
/**
* String field to store the data in the type attribute.
*/
private String typeConfig;
/**
* boolean field to store the data in the preseed attribute.
*/
private boolean preseedConfig;
/**
* boolean field to store the data in the wait attribute.
*/
private boolean waitConfig;
/**
* String field to store the data in the config attribute.
*/
private String configuration;
/**
* boolean field to store the data in the incremental attribute.
*/
private boolean incrementalConfig;
/**
* Getter for the typeConfig field.
* @return The value stored in the typeConfig field.
*/
@Column(length = CONFIG_LENGTH)
public String getTypeConfig() {
return typeConfig;
}
/**
* Setter for the typeConfig field.
* @param typeConfig The String value to be stored in typeConfig.
*/
public void setTypeConfig(String typeConfig) {
this.typeConfig = typeConfig;
}
/**
* Getter for the preseedConfig field.
* @return The value store in the preseedConfig field.
*/
@Column
public boolean isPreseedConfig() {
return preseedConfig;
}
/**
* Setter for the PreseedConfig field.
* @param preseedConfig The boolean value to be stored in the preseedConfig field.
*/
public void setPreseedConfig(boolean preseedConfig) {
this.preseedConfig = preseedConfig;
}
/**
* Getter for the waitConfig field.
* @return The boolean value stored in the waitConfig field.
*/
@Column
public boolean isWaitConfig() {
return waitConfig;
}
/**
* Setter for the waitConfig field.
* @param waitConfig The boolean value to be stored in the waitConfig field.
*/
public void setWaitConfig(boolean waitConfig) {
this.waitConfig = waitConfig;
}
/**
* Getter for the configuration field.
* @return The String value stored in the configuration field.
*/
@Column(length = CONFIG_LENGTH)
public String getConfiguration() {
return configuration;
}
/**
* Setter for the configuration field
* @param configuration The String value to be stored in the configuration field.
*/
public void setConfiguration(String configuration) {
this.configuration = configuration;
}
/**
* Getter for the incrementalConfig field.
* @return The boolean value stored in the incrementalConfig field.
*/
@Column
public boolean isIncrementalConfig() {
return incrementalConfig;
}
/**
* Setter fo the incrementalConfig field.
* @param incrementalConfig The boolean value to be stored in the incrementalConfig field.
*/
public void setIncrementalConfig(boolean incrementalConfig) {
this.incrementalConfig = incrementalConfig;
}
@Transient
public String getSortValue() {
return typeConfig;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy