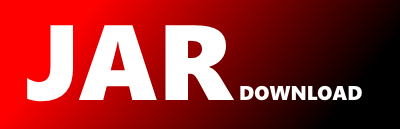
com.denimgroup.threadfix.data.enums.ApiVersions Maven / Gradle / Ivy
package com.denimgroup.threadfix.data.enums;
import com.denimgroup.threadfix.data.entities.Version;
import com.fasterxml.jackson.annotation.JsonView;
import java.util.*;
import static com.denimgroup.threadfix.CollectionUtils.list;
import static com.denimgroup.threadfix.CollectionUtils.map;
/**
* @author jtomsett
* Enum of the REST api Versions
*/
public enum ApiVersions {
LEGACY("Legacy",Integer.MIN_VALUE),
LATEST("Latest",Integer.MAX_VALUE),
V2_4("2.4",2400),
V2_4_2_1("2.4.2.1",2421),
V2_4_5("2.4.5",2450),
V2_5("2.5",2500);
/**
*
* @param displayName the display friendly REST api version name
* @param version the Integer value for the REST api version
*/
ApiVersions(String displayName, Integer version) {
this.version = version;
this.displayName = displayName;
}
/**
* the display friendly REST api version name
*/
private String displayName;
/**
* the Integer value for the REST api version
*/
private Integer version;
/**
*
* @return the display friendly REST api version name
*/
@JsonView(Object.class)
public String getDisplayName() { return displayName; }
/**
*
* @return the Integer value for the REST api version
*/
public Integer getVersion(){return version;}
/**
*
* @param input display friendly REST api version name
* @return ApiVersions enum value that corresponds to the input
*/
public static ApiVersions getApiVersion(String input) {
if(input.equalsIgnoreCase(ApiVersions.LATEST.getDisplayName())){
return ApiVersions.LATEST;
}
if(input.equalsIgnoreCase(ApiVersions.LEGACY.getDisplayName())){
return ApiVersions.LEGACY;
}
return getApiVersion(new Version(input));
}
public static ApiVersions getApiVersion(Version version){
List versionList = list();
Map apiVersionsMap = map();
if(version != null && !version.getVersionString().equals(version.getInvalidVersion())){
for(ApiVersions apiVersion : values()){
if(apiVersion.getDisplayName().equalsIgnoreCase("latest") || apiVersion.getDisplayName().equalsIgnoreCase("legacy")){
continue;
}
Version restVersion = new Version(apiVersion.getDisplayName());
if(restVersion.getVersionString().equalsIgnoreCase(restVersion.getInvalidVersion())){
continue;
}
int compareToValue = version.compareTo(restVersion);
if(compareToValue == 0){
return apiVersion;
}
if(compareToValue > 0){
versionList.add(restVersion);
apiVersionsMap.put(restVersion,apiVersion);
}
}
if(!versionList.isEmpty()) {
Collections.sort(versionList);
return apiVersionsMap.get(versionList.get(versionList.size()-1));
}else{
return ApiVersions.LEGACY;
}
}
return null;
}
/**
* Compares 2 ApiVersions enums based on their version attribute
*/
public static Comparator apiVersionsComparator = new Comparator() {
@Override
public int compare(ApiVersions o1, ApiVersions o2) {
return o1.version.compareTo(o2.getVersion());
}
};
/**
*
* @param from starting ApiVersions enum value
* @param to ending Apiversions enum value
* @return a list of ApiVersions that fall between the from and to params. This is based on the version value and is inclusive.
*/
public static List getApiVersionsList(ApiVersions from, ApiVersions to){
List apiVersionRangeList = new ArrayList(Arrays.asList(ApiVersions.values()));
Collections.sort(apiVersionRangeList,apiVersionsComparator);
int fromIndex = apiVersionRangeList.indexOf(from);
int toIndex = apiVersionRangeList.indexOf(to)+1;
return apiVersionRangeList.subList(fromIndex,toIndex);
}
public static List getApiVersionsList(ApiVersions from) {
return getApiVersionsList(from, LATEST);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy