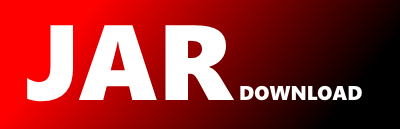
com.denimgroup.threadfix.util.IntegerUtils Maven / Gradle / Ivy
package com.denimgroup.threadfix.util;
import com.denimgroup.threadfix.logging.SanitizedLogger;
public class IntegerUtils {
private static final SanitizedLogger log = new SanitizedLogger(IntegerUtils.class);
private IntegerUtils(){}
/**
* Returns Integer.valueOf(input) with exception handling. Will return -1 if it fails to parse.
*
* @param input String representation of an integer
* @return the parsed number, or -1 on failure
*/
public static int getPrimitive(String input) {
if (input == null) {
log.warn("Null string passed to getPrimitive");
return -1;
}
if (!input.matches("^[0-9]+$")) {
log.warn("Non-numeric String encountered.");
return -1;
}
try {
return Integer.valueOf(input);
} catch (NumberFormatException e) {
log.warn("Non-numeric input encountered: " + input, e);
return -1;
}
}
/**
* Returns Integer.valueOf(input) with exception handling. Will return null if it fails to parse.
*
* @param input String representation of an integer
* @return the parsed number, or null on failure
*/
public static Integer getIntegerOrNull(String input){
String regex = "^[-+]?[0-9]+$";
if (input == null) {
log.debug("Null passed to getInteger or null.");
return null;
}
if (!input.matches(regex)) {
log.warn("Non-numeric String encountered: " + input);
return null;
}
try {
return Integer.valueOf(input);
} catch (NumberFormatException e) {
log.warn("Non-numeric input encountered: " + input, e);
return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy