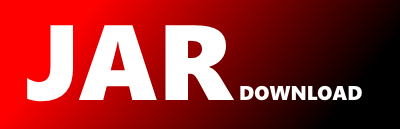
com.deque.axe.android.AxePropCalculator.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of axe-devtools-android-core Show documentation
Show all versions of axe-devtools-android-core Show documentation
The Axe Devtools Android Core Library
package com.deque.axe.android
import com.deque.axe.android.constants.AndroidClassNames
import com.deque.axe.android.utils.AxeTextUtils
import java.util.Objects
import kotlin.collections.HashMap
class AxePropCalculator(
private val text: String?,
private val contentDescription: String?,
private val labelText: String?,
private val value: String?,
private val isEnabled: Boolean,
private val className: String?,
private val hintText: String?,
private val isVisibleToUser: Boolean
) {
private val propMap: MutableMap = HashMap()
private var calculatedProp: String? = null
init {
val axePropInterface: AxePropInterface = object : AxePropInterface {}
propMap[Props.NAME.prop] = axePropInterface.getNameProp(
text,
contentDescription,
labelText,
hintText
)
propMap[Props.ROLE.prop] = axePropInterface.getRoleProp(className)
propMap[Props.VALUE.prop] = axePropInterface.getValueProp(value)
propMap[Props.STATE.prop] = null
propMap[Props.IS_VISIBLE_TO_USER.prop] =
axePropInterface.getIsVisibleToUserProp(isVisibleToUser).toString()
}
val calculatedProps: CalculatedProps
get() {
when (className) {
AndroidClassNames.SWITCH, AndroidClassNames.CHECKBOX -> {
calculatedProp = object : AxePropInterface {
override fun getNameProp(
text: String?,
contentDescription: String?,
labelText: String?,
hint: String?
): String {
return (getPropAfterNullCheck(text) + SPACE
+ getPropAfterNullCheck(contentDescription) + SPACE
+ getPropAfterNullCheck(labelText))
}
}.getNameProp(text, contentDescription, labelText, hintText)
setCalculatedProp(Props.NAME.prop, calculatedProp)
calculatedProp = object : AxePropInterface {
override fun getStateProp(state: String): String {
return if (isEnabled) "enabled" else "disabled"
}
}.getStateProp("")
setCalculatedProp(Props.STATE.prop, calculatedProp)
}
AndroidClassNames.EDIT_TEXT -> {
calculatedProp = object : AxePropInterface {
override fun getNameProp(
text: String?,
contentDescription: String?,
labelText: String?,
hint: String?
): String {
return if (!AxeTextUtils.isNullOrEmpty(contentDescription)
&& !AxeTextUtils.isNullOrEmpty(hint)
) {
(getPropAfterNullCheck(hint) + SPACE
+ getPropAfterNullCheck(labelText))
} else {
(getPropAfterNullCheck(contentDescription) + SPACE
+ getPropAfterNullCheck(hint) + SPACE
+ getPropAfterNullCheck(labelText))
}
}
}.getNameProp(text, contentDescription, labelText, hintText)
setCalculatedProp(Props.NAME.prop, calculatedProp)
calculatedProp = object : AxePropInterface {
override fun getValueProp(value: String?): String? {
return text
}
}.getValueProp(text)
setCalculatedProp(Props.VALUE.prop, calculatedProp)
}
AndroidClassNames.TEXT_VIEW -> {
calculatedProp = object : AxePropInterface {
override fun getValueProp(value: String?): String? {
return text
}
}.getValueProp(text)
setCalculatedProp(Props.VALUE.prop, calculatedProp)
}
AndroidClassNames.IMAGE_VIEW -> {
calculatedProp = object : AxePropInterface {
override fun getNameProp(
text: String?,
contentDescription: String?,
labelText: String?,
hint: String?
): String {
return (getPropAfterNullCheck(text) + SPACE
+ getPropAfterNullCheck(contentDescription) + SPACE
+ getPropAfterNullCheck(labelText))
}
}.getNameProp(text, contentDescription, labelText, hintText)
setCalculatedProp(Props.NAME.prop, calculatedProp)
}
else -> {
}
}
return propMap
}
private fun setCalculatedProp(key: String, value: String?) {
propMap[key] = value
}
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
if (other !is AxePropCalculator) {
return false
}
return (isEnabled == other.isEnabled && text == other.text
&& contentDescription == other.contentDescription
&& labelText == other.labelText
&& value == other.value
&& className == other.className
&& hintText == other.hintText
&& isVisibleToUser == other.isVisibleToUser
&& calculatedProp == other.calculatedProp)
}
override fun hashCode(): Int {
return Objects.hash(
text, contentDescription, labelText, value,
isEnabled, className, hintText, isVisibleToUser, calculatedProp
)
}
enum class Props(val prop: String) {
NAME("name"), ROLE("role"), VALUE("value"), STATE("state"), IS_VISIBLE_TO_USER("isVisibleToUser");
}
interface AxePropInterface {
fun getNameProp(
text: String?,
contentDescription: String?,
labelText: String?,
hint: String?
): String {
return getPropAfterNullCheck(text) + SPACE + getPropAfterNullCheck(contentDescription)
}
fun getRoleProp(className: String?): String? {
return className
}
fun getValueProp(value: String?): String? {
return value
}
fun getStateProp(state: String): String? {
return state
}
fun getIsVisibleToUserProp(isVisibleToUser: Boolean): Boolean? {
return isVisibleToUser
}
}
companion object {
private const val SPACE = " "
private fun getPropAfterNullCheck(propText: String?): String {
return propText ?: ""
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy