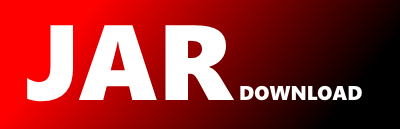
com.deque.axe.android.AxeRuleViewHierarchy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of axe-devtools-android-core Show documentation
Show all versions of axe-devtools-android-core Show documentation
The Axe Devtools Android Core Library
package com.deque.axe.android;
import androidx.annotation.CallSuper;
import com.deque.axe.android.conf.RuleConf;
import com.deque.axe.android.constants.AxeStatus;
import com.deque.axe.android.wrappers.AxeProps;
import java.util.Objects;
import java.util.Set;
public abstract class AxeRuleViewHierarchy extends AxeRule {
private static final int VIEW_INVISIBILITY_CONSTANT = 4;
private static final int VIEW_GONE_CONSTANT = 8;
protected AxeRuleViewHierarchy(String standard, int impact, String summary, boolean experimental) {
super(new RuleConf(impact, standard, summary, experimental));
}
public void setup(final AxeContext axeContext, final AxeProps axeProps) { }
/**
* Collects Props for each view.
* @param axeView to collect props from.
* @param axeProps to which props will be stored in.
*/
@CallSuper
public void collectProps(final AxeView axeView, final AxeProps axeProps) {
axeProps.put(
AxeProps.Name.OVERRIDES_ACCESSIBILITY_DELEGATE,
axeView.overridesAccessibilityDelegate
);
axeProps.put(
AxeProps.Name.IS_COMPOSE_VIEW,
axeView.isComposeView
);
axeProps.put(
AxeProps.Name.VIEW_ID,
Objects.requireNonNullElse(
axeView.viewIdResourceName,
"No ID Available"
)
);
axeProps.put(
AxeProps.Name.VISIBILITY,
axeView.visibility
);
axeProps.put(AxeProps.Name.IGNORED_RULES_LIST, axeView.ignoreRules);
if (axeView.getCalculatedProps() != null
&& axeView.getCalculatedProps().get(
AxePropCalculator.Props.IS_VISIBLE_TO_USER.getProp()) != null) {
axeProps.put(
AxeProps.Name.IS_VISIBLE_TO_USER,
axeView.getCalculatedProps().get(
AxePropCalculator.Props.IS_VISIBLE_TO_USER.getProp()
)
);
}
}
@CallSuper
public boolean isApplicable(final AxeProps axeProps) {
return !axeProps.get(AxeProps.Name.OVERRIDES_ACCESSIBILITY_DELEGATE, Boolean.class)
&& !axeProps.get(AxeProps.Name.IS_COMPOSE_VIEW, Boolean.class)
&& axeProps.get(AxeProps.Name.VISIBILITY, Integer.class) != VIEW_INVISIBILITY_CONSTANT
&& axeProps.get(AxeProps.Name.VISIBILITY, Integer.class) != VIEW_GONE_CONSTANT;
}
/**
* returns AxeStatus.IGNORED if the view is ignored.
* @param axeProps the props.
* @return IGNORED or empty string.
*/
@CallSuper
public @AxeStatus String runRule(final AxeProps axeProps) {
if (axeProps.get(AxeProps.Name.IGNORED_RULES_LIST, Set.class)
.contains(this.getClass().getSimpleName())) {
return AxeStatus.IGNORED;
}
return "";
}
public void tearDown() { }
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy