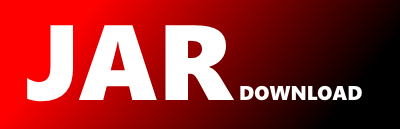
com.deque.networking.interfaces.AuthUtils.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of axe-devtools-android-core Show documentation
Show all versions of axe-devtools-android-core Show documentation
The Axe Devtools Android Core Library
package com.deque.networking.interfaces
import androidx.annotation.Keep
import com.deque.networking.AxeLogger
import com.deque.networking.analytics.AmplitudeEventConstant
import com.deque.networking.analytics.AmplitudeEventProps
import com.deque.networking.analytics.AmplitudeEventType
import com.deque.networking.analytics.AnalyticsService
import com.deque.networking.models.auth.AccessToken
import com.deque.networking.models.auth.ApiKey
import com.deque.networking.models.auth.AuthSource
import kotlinx.coroutines.runBlocking
internal object ServerConnection {
// TODO: replace this variable with function that sync w/ server at anytime when we are using new backend server
// https://app.zenhub.com/workspaces/mobile---android-618c52b298700300142de56d/issues/dequelabs/axe-devtools-android/268
var userAuthenticated = false
var userId: String? = null
var email: String? = null
fun connect(
username: String,
password: String,
serverConfig: ConnectionConfig = ConnectionConfig.DEFAULT_CONNECTION_CONFIG
): AxeDevToolsClient {
return initializeClient(
AccessToken(username, password, serverConfig.authUrl, serverConfig.realm),
serverConfig.dbUrl
)
}
fun connect(
apiKey: String,
serverConfig: ConnectionConfig = ConnectionConfig.DEFAULT_CONNECTION_CONFIG
): AxeDevToolsClient {
return initializeClient(ApiKey(apiKey), serverConfig.dbUrl)
}
fun connectLocally(port: String): AxeDevToolsClient {
return initializeClient(null, port)
}
private fun initializeClient(authSource: AuthSource?, dbUrl: String): AxeDevToolsClient {
val axeDevToolsClient: AxeDevToolsClient = when (authSource) {
is ApiKey -> ApiKeyClient(authSource, dbUrl)
is AccessToken -> runBlocking {
AccessTokenClient(authSource.fetch(), dbUrl)
}
else -> AccessTokenClient(null, "http://localhost:$dbUrl")
}
val errorEventType = if (authSource != null && authSource is ApiKey)
AmplitudeEventType.LOGIN_API_KEY_ERROR else AmplitudeEventType.LOGIN_USERNAME_ERROR
if (!isServerUp(axeDevToolsClient)) {
if (authSource == null) AxeLogger.desktopDown() else AxeLogger.serverDown()
AnalyticsService.sendEvent(
errorEventType,
AmplitudeEventProps(
reason = AmplitudeEventConstant.BACKEND_IS_DOWN
)
)
} else {
userAuthenticated = runBlocking { isUserAuthenticated(axeDevToolsClient) }
if (!userAuthenticated) {
AnalyticsService.sendEvent(
errorEventType,
AmplitudeEventProps(
reason = AmplitudeEventConstant.USER_NOT_AUTH
)
)
}
}
return axeDevToolsClient
}
private fun isServerUp(client: AxeDevToolsClient): Boolean {
val healthStatus = runBlocking { client.getHealthCheck() }.onFailure {
AxeLogger.logNetworkError(it)
}.getOrNull()?.status?.code ?: ""
return healthStatus == AxeDevToolsClient.UP
}
private suspend fun isUserAuthenticated(axeDevToolsClient: AxeDevToolsClient): Boolean {
val userInfo = axeDevToolsClient.getUserInfo().onFailure {
AxeLogger.notAuthenticated(it)
}.getOrNull()
userId = userInfo?.userId
email = userInfo?.email
return userInfo?.userId?.isNotBlank() ?: false
}
}
data class ConnectionConfig(val realm: String, val authUrl: String, val dbUrl: String) {
@Keep
companion object {
val DEFAULT_CONNECTION_CONFIG = ConnectionConfig(
AxeDevToolsClient.DEFAULT_REALM,
AccessToken.DEFAULT_URL,
AxeDevToolsClient.DB_DEFAULT_URL
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy