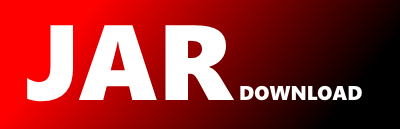
com.deque.networking.interfaces.AxeDevToolsClient.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of axe-devtools-android-core Show documentation
Show all versions of axe-devtools-android-core Show documentation
The Axe Devtools Android Core Library
package com.deque.networking.interfaces
import com.deque.axe.android.AxeResult
import com.deque.networking.models.auth.AuthSource
import com.deque.networking.models.devtools.TagsSet
import com.deque.networking.models.devtools.serializable.AxeDevToolsResult
import com.deque.networking.models.devtools.serializable.AxeDevToolsResultKey
import com.deque.networking.models.devtools.serializable.AxeDevToolsResultSummaryResponse
import com.deque.networking.models.devtools.serializable.HealthCheck
import com.deque.networking.models.devtools.serializable.UserInfo
import kotlinx.coroutines.runBlocking
sealed class AxeDevToolsClient(protected val authSource: AuthSource?) {
/**
* Download A11y Result asynchronously from the Dashboard.
*
* @param axeDevToolsResultKey scan's result key
* @return kotlin.Result object with scan's result
*/
abstract suspend fun getResult(
axeDevToolsResultKey: AxeDevToolsResultKey,
): Result
/**
* Upload result asynchronously to the Dashboard
*
* @param axeResult scan's AxeResult object
* @return kotlin.Result with a result key from the dashboard
*/
abstract suspend fun postResult(axeResult: AxeResult): Result
/**
* Delete result asynchronously from the Dashboard
*
* @param axeDevToolsResultKey scan's result key
* @return kotlin.Result object with Unit object
*/
abstract suspend fun deleteResult(axeDevToolsResultKey: AxeDevToolsResultKey): Result
/**
* Tag result asynchronously on the Dashboard
*
* @param axeDevToolsResultKey scan's result key
* @param tags a set of tags to add to the scan
* @return kotlin.Result object with tagged scan's result key
*/
abstract suspend fun tag(
axeDevToolsResultKey: AxeDevToolsResultKey,
tags: TagsSet
): Result
/**
* Set a scan name to result asynchronously on the Dashboard
*
* @param axeDevToolsResultKey scan's result key
* @param scanName a name for the scan's result
* @return kotlin.Result object with scan's result summary
*/
abstract suspend fun setScanName(
axeDevToolsResultKey: AxeDevToolsResultKey,
scanName: String
): Result
/**
* Get connected user's information asynchronously from the Dashboard
*
* @return kotlin.Result object with user's information
*/
abstract suspend fun getUserInfo(): Result
/**
* Check Dashboard's health status
*
* @return kotlin.Result object with server's status
*/
abstract suspend fun getHealthCheck(): Result
/**
* Download result synchronously from the Dashboard
*
* @param axeDevToolsResultKey scan result's key
* @return scan's result
*/
fun getResultSync(axeDevToolsResultKey: AxeDevToolsResultKey): AxeDevToolsResult? =
runBlocking {
getResult(axeDevToolsResultKey).getOrNull()
}
/**
* Upload result synchronously to the Dashboard
*
* @param axeResult scan's AxeResult object
* @return scan's result key
*/
fun postResultSync(axeResult: AxeResult): AxeDevToolsResultKey? = runBlocking {
postResult(axeResult).getOrNull()
}
/**
* Delete result synchronously from the Dashboard
*
* @param axeDevToolsResultKey scan's result key
* @return unit object
*/
fun deleteResultSync(axeDevToolsResultKey: AxeDevToolsResultKey): Unit = runBlocking {
deleteResult(axeDevToolsResultKey).getOrNull()
}
/**
* Tag result synchronously on the Dashboard
*
* @param axeDevToolsResultKey scan's result key
* @param tags a set of tags to add to the scan
* @return tagged scan's result key
*/
fun tagSync(
axeDevToolsResultKey: AxeDevToolsResultKey,
tags: TagsSet,
): AxeDevToolsResultKey? = runBlocking {
tag(axeDevToolsResultKey, tags).getOrNull()
}
/**
* Set a scan name to result synchronously on the Dashboard
*
* @param axeDevToolsResultKey scan's result key
* @param scanName a name for the scan's result
* @return scan's result summary
*/
fun setScanNameSync(
axeDevToolsResultKey: AxeDevToolsResultKey,
scanName: String
): AxeDevToolsResultSummaryResponse? = runBlocking {
setScanName(axeDevToolsResultKey, scanName).getOrNull()
}
companion object {
const val DB_DEFAULT_URL = "https://axe-mobile-backend.deque.com"
const val DB_QA_URL = "https://mobile-qa.dequelabs.com"
const val DB_DEV_URL = "https://mobile-dev.dequelabs.com"
const val DEFAULT_REALM = "axe"
const val QA_REALM = "axe-qa"
const val DEV_REALM = "axe"
const val UP = "UP"
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy