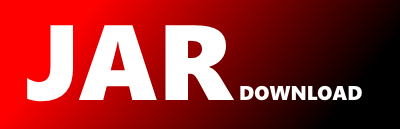
com.devebot.opflow.OpflowUtil Maven / Gradle / Ivy
package com.devebot.opflow;
import com.google.gson.Gson;
import com.rabbitmq.client.AMQP;
import java.io.UnsupportedEncodingException;
import java.util.HashMap;
import java.util.Map;
import com.devebot.opflow.exception.OpflowOperationException;
/**
*
* @author drupalex
*/
public class OpflowUtil {
private static final Gson gson = new Gson();
public static byte[] getBytes(String data) {
if (data == null) return null;
try {
return data.getBytes("UTF-8");
} catch (UnsupportedEncodingException exception) {
throw new OpflowOperationException(exception);
}
}
public static String getString(byte[] data) {
if (data == null) return null;
try {
return new String(data, "UTF-8");
} catch (UnsupportedEncodingException exception) {
throw new OpflowOperationException(exception);
}
}
public static Map cloneParameters(Map params) {
Map clonedParams = new HashMap();
for (String i : params.keySet()) {
Object value = params.get(i);
clonedParams.put(i, value);
}
return clonedParams;
}
public static boolean arrayContains(final T[] array, final T v) {
if (v == null) {
for (final T e : array) if (e == null) return true;
} else {
for (final T e : array) if (e == v || v.equals(e)) return true;
}
return false;
}
public interface MapListener {
public void transform(Map opts);
}
public static String buildJson(MapListener listener) {
Map jsonMap = new HashMap();
if (listener != null) {
listener.transform(jsonMap);
}
return gson.toJson(jsonMap);
}
public static Map buildOptions(MapListener listener) {
return buildOptions(listener, null);
}
public static Map buildOptions(MapListener listener, Map defaultOpts) {
Map jsonMap = new HashMap();
if (listener != null) {
listener.transform(jsonMap);
}
return jsonMap;
}
public static Map ensureNotNull(Map opts) {
return (opts == null) ? new HashMap() : opts;
}
public static Map getHeaders(AMQP.BasicProperties properties) {
if (properties != null && properties.getHeaders() != null) {
return properties.getHeaders();
} else {
return new HashMap();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy