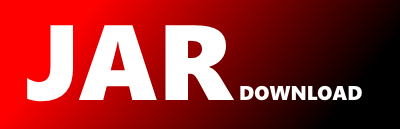
com.diboot.core.service.BaseService Maven / Gradle / Ivy
package com.diboot.core.service;
import com.baomidou.mybatisplus.core.conditions.Wrapper;
import com.diboot.core.binding.binder.EntityBinder;
import com.diboot.core.binding.binder.EntityListBinder;
import com.diboot.core.binding.binder.FieldBinder;
import com.diboot.core.vo.KeyValue;
import com.diboot.core.vo.Pagination;
import java.io.Serializable;
import java.util.Collection;
import java.util.List;
import java.util.Map;
/**
* 基础服务Service
* @author Mazhicheng
* @version 2.0
* @date 2019/01/01
*/
public interface BaseService {
/**
* 获取Entity实体
* @param id 主键
* @return entity
*/
T getEntity(Serializable id);
/**
* 创建Entity实体
* @param entity
* @return true:成功, false:失败
*/
boolean createEntity(T entity);
/***
* 批量创建Entity
* @param entityList 实体对象列表
* @return true:成功, false: 失败
*/
boolean createEntities(Collection entityList);
/**
* 更新Entity实体
* @param entity
* @return
*/
boolean updateEntity(T entity);
/**
* 更新Entity实体(更新符合条件的所有非空字段)
* @param entity
* @param updateCriteria
* @return
*/
boolean updateEntity(T entity, Wrapper updateCriteria);
/**
* 更新Entity实体(仅更新updateWrapper.set指定的字段)
* @param updateWrapper
* @return
*/
boolean updateEntity(Wrapper updateWrapper);
/***
* 创建或更新entity(entity.id存在则新建,否则更新)
* @param entity
* @return
*/
boolean createOrUpdateEntity(T entity);
/**
* 批量创建或更新entity(entity.id存在则新建,否则更新)
* @param entityList
* @return
*/
boolean createOrUpdateEntities(Collection entityList);
/**
* 根据主键删除实体
* @param id 主键
* @return true:成功, false:失败
*/
boolean deleteEntity(Serializable id);
/**
* 按条件删除实体
* @param queryWrapper
* @return
* @throws Exception
*/
boolean deleteEntities(Wrapper queryWrapper) throws Exception;
/**
* 获取符合条件的entity记录总数
* @return
*/
int getEntityListCount(Wrapper queryWrapper);
/**
* 获取指定条件的Entity集合
* @param queryWrapper
* @return
* @throws Exception
*/
List getEntityList(Wrapper queryWrapper);
/**
* 获取指定条件的Entity集合
* @param queryWrapper
* @param pagination
* @return
* @throws Exception
*/
List getEntityList(Wrapper queryWrapper, Pagination pagination);
/**
* 获取指定条件的Entity集合
* @param ids
* @return
*/
List getEntityListByIds(List ids);
/**
* 获取指定数量的entity记录
* @param queryWrapper
* @param limitCount
* @return
* @throws Exception
*/
List getEntityListLimit(Wrapper queryWrapper, int limitCount);
/**
* 获取指定属性的Map列表
* @param queryWrapper
* @return
*/
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy