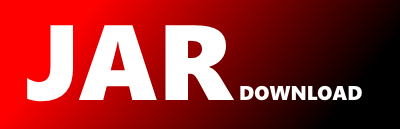
com.diboot.core.util.ContextHelper Maven / Gradle / Ivy
package com.diboot.core.util;
import com.baomidou.mybatisplus.extension.service.IService;
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl;
import com.diboot.core.service.BaseService;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.BeansException;
import org.springframework.context.ApplicationContext;
import org.springframework.context.ApplicationContextAware;
import org.springframework.context.annotation.Lazy;
import org.springframework.core.ResolvableType;
import org.springframework.stereotype.Component;
import java.lang.annotation.Annotation;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
/**
* Spring上下文帮助类
* @author Mazhicheng
* @version 2.0
* @date 2019/01/01
*/
@Component
@Lazy(false)
public class ContextHelper implements ApplicationContextAware {
private static final Logger log = LoggerFactory.getLogger(ContextHelper.class);
/***
* ApplicationContext上下文
*/
private static ApplicationContext APPLICATION_CONTEXT = null;
/**
* Entity-对应的Service缓存
*/
private static Map ENTITY_SERVICE_CACHE = null;
@Override
public void setApplicationContext(ApplicationContext applicationContext) throws BeansException {
if(APPLICATION_CONTEXT == null){
APPLICATION_CONTEXT = applicationContext;
}
}
/***
* 获取ApplicationContext上下文
*/
public static ApplicationContext getApplicationContext() {
return APPLICATION_CONTEXT;
}
/***
* 根据beanId获取Bean实例
* @param beanId
* @return
*/
public static Object getBean(String beanId){
return getApplicationContext().getBean(beanId);
}
/***
* 获取指定类型的单个Bean实例
* @param type
* @return
*/
public static Object getBean(Class type){
return getApplicationContext().getBean(type);
}
/***
* 获取指定类型的全部实例
* @param type
* @param
* @return
*/
public static List getBeans(Class type){
// 获取所有的定时任务实现类
Map map = getApplicationContext().getBeansOfType(type);
if(V.isEmpty(map)){
return null;
}
List beanList = new ArrayList<>();
beanList.addAll(map.values());
return beanList;
}
/***
* 根据注解获取beans
* @param annotationType
* @return
*/
public static List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy