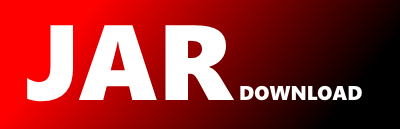
com.diboot.core.binding.JoinsBinder Maven / Gradle / Ivy
/*
* Copyright (c) 2015-2020, www.dibo.ltd ([email protected]).
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.diboot.core.binding;
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import com.baomidou.mybatisplus.core.metadata.IPage;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import com.baomidou.mybatisplus.extension.service.IService;
import com.diboot.core.binding.helper.ServiceAdaptor;
import com.diboot.core.binding.parser.ParserCache;
import com.diboot.core.binding.query.dynamic.AnnoJoiner;
import com.diboot.core.binding.query.dynamic.DynamicJoinQueryWrapper;
import com.diboot.core.binding.query.dynamic.DynamicSqlProvider;
import com.diboot.core.config.BaseConfig;
import com.diboot.core.config.Cons;
import com.diboot.core.data.ProtectFieldHandler;
import com.diboot.core.exception.InvalidUsageException;
import com.diboot.core.mapper.DynamicQueryMapper;
import com.diboot.core.util.BeanUtils;
import com.diboot.core.util.ContextHelper;
import com.diboot.core.util.S;
import com.diboot.core.util.V;
import com.diboot.core.vo.Pagination;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.BeanWrapper;
import java.lang.reflect.Field;
import java.util.*;
/**
* join连接查询绑定器
* @author [email protected]
* @version v2.1
* @date 2020/04/15
*/
@Slf4j
public class JoinsBinder {
/**
* 关联查询一条数据
* @param queryWrapper
* @param entityClazz 返回结果entity/vo类
* @return
* @throws Exception
*/
public static T queryOne(QueryWrapper queryWrapper, Class entityClazz){
List list = executeJoinQuery(queryWrapper, entityClazz, null, true);
if(V.notEmpty(list)){
return list.get(0);
}
return null;
}
/**
* 关联查询符合条件的全部数据集合(不分页)
* @param queryWrapper 调用QueryBuilder.to*QueryWrapper得到的实例
* @param entityClazz 返回结果entity/vo类
* @return
* @throws Exception
*/
public static List queryList(QueryWrapper queryWrapper, Class entityClazz){
return queryList(queryWrapper, entityClazz, null);
}
/**
* 关联查询符合条件的指定页数据(分页)
* @param queryWrapper 调用QueryBuilder.to*QueryWrapper得到的实例
* @param entityClazz 返回结果entity/vo类
* @param pagination 分页
* @return
* @throws Exception
*/
public static List queryList(QueryWrapper queryWrapper, Class entityClazz, Pagination pagination){
return executeJoinQuery(queryWrapper, entityClazz, pagination, false);
}
/**
* 关联查询(分页)
* @param queryWrapper 调用QueryBuilder.to*QueryWrapper得到的实例
* @param entityClazz 返回结果entity/vo类
* @param pagination 分页
* @return
* @throws Exception
*/
private static List executeJoinQuery(QueryWrapper queryWrapper, Class entityClazz, Pagination pagination, boolean limit1){
// 非动态查询,走BaseService
if(queryWrapper instanceof DynamicJoinQueryWrapper == false){
IService iService = ContextHelper.getIServiceByEntity(entityClazz);
if(iService != null){
return ServiceAdaptor.queryList(iService, (QueryWrapper)queryWrapper, pagination, entityClazz);
}
else{
throw new InvalidUsageException("单表查询对象无BaseService/IService实现: "+entityClazz.getSimpleName());
}
}
long begin = System.currentTimeMillis();
// 转换为queryWrapper
DynamicJoinQueryWrapper dynamicJoinWrapper = (DynamicJoinQueryWrapper)queryWrapper;
dynamicJoinWrapper.setMainEntityClass(entityClazz);
List