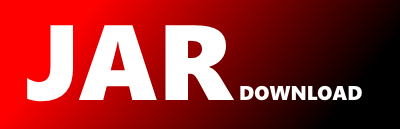
com.diboot.core.util.ContextHelper Maven / Gradle / Ivy
/*
* Copyright (c) 2015-2020, www.dibo.ltd ([email protected]).
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.diboot.core.util;
import com.baomidou.mybatisplus.annotation.DbType;
import com.baomidou.mybatisplus.core.mapper.BaseMapper;
import com.baomidou.mybatisplus.extension.service.IService;
import com.baomidou.mybatisplus.extension.toolkit.JdbcUtils;
import com.diboot.core.binding.cache.BindingCacheManager;
import com.diboot.core.binding.parser.EntityInfoCache;
import com.diboot.core.binding.parser.ParserCache;
import com.diboot.core.binding.parser.PropInfo;
import com.diboot.core.exception.InvalidUsageException;
import com.diboot.core.service.BaseService;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.BeansException;
import org.springframework.boot.context.event.ApplicationReadyEvent;
import org.springframework.context.ApplicationContext;
import org.springframework.context.ApplicationContextAware;
import org.springframework.context.ApplicationListener;
import org.springframework.context.annotation.Lazy;
import org.springframework.core.env.Environment;
import org.springframework.stereotype.Component;
import org.springframework.web.context.ContextLoader;
import javax.sql.DataSource;
import java.lang.annotation.Annotation;
import java.sql.Connection;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
/**
* Spring上下文帮助类
* @author [email protected]
* @version 2.0
* @date 2019/01/01
*/
@Component
@Lazy(false)
public class ContextHelper implements ApplicationContextAware, ApplicationListener {
private static final Logger log = LoggerFactory.getLogger(ContextHelper.class);
/***
* ApplicationContext上下文
*/
private static ApplicationContext APPLICATION_CONTEXT = null;
/**
* 数据库类型
*/
private static String DATABASE_TYPE = null;
@Override
public void setApplicationContext(ApplicationContext applicationContext) throws BeansException {
APPLICATION_CONTEXT = applicationContext;
log.debug("ApplicationContext已赋值: {}", APPLICATION_CONTEXT.getDisplayName());
}
@Override
public void onApplicationEvent(ApplicationReadyEvent event) {
APPLICATION_CONTEXT = event.getApplicationContext();
log.debug("ApplicationContext已注入: {}", APPLICATION_CONTEXT.getDisplayName());
}
/***
* 获取ApplicationContext上下文
*/
public static ApplicationContext getApplicationContext() {
if (APPLICATION_CONTEXT == null){
log.debug("ApplicationContext未初始化,通过ContextLoader获取!");
APPLICATION_CONTEXT = ContextLoader.getCurrentWebApplicationContext();
}
if(APPLICATION_CONTEXT == null){
log.warn("无法获取ApplicationContext,请确保ComponentScan扫描路径包含com.diboot包路径,并在Spring初始化之后调用接口!");
new InvalidUsageException("检查调用时机").printStackTrace();
}
return APPLICATION_CONTEXT;
}
/***
* 根据beanId获取Bean实例
* @param beanId
* @return
*/
public static Object getBean(String beanId){
return getApplicationContext().getBean(beanId);
}
/***
* 获取指定类型的单个Bean实例
* @param clazz
* @return
*/
public static T getBean(Class clazz){
try{
return getApplicationContext().getBean(clazz);
}
catch (Exception e){
log.debug("instance not found: {}", clazz.getSimpleName());
return null;
}
}
/***
* 获取指定类型的全部实现类
* @param type
* @param
* @return
*/
public static List getBeans(Class type){
Map map = getApplicationContext().getBeansOfType(type);
if(V.isEmpty(map)){
return null;
}
List beanList = new ArrayList<>(map.size());
beanList.addAll(map.values());
return beanList;
}
/***
* 根据注解获取beans
* @param annotationType
* @return
*/
public static List