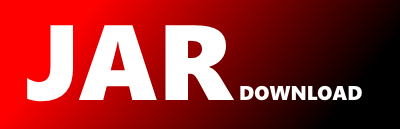
com.diboot.framework.controller.BaseController Maven / Gradle / Ivy
The newest version!
package com.diboot.framework.controller;
import com.diboot.framework.async.AsyncLogger;
import com.diboot.framework.config.BaseConfig;
import com.diboot.framework.model.BaseUser;
import com.diboot.framework.service.BaseFileService;
import com.diboot.framework.service.MetadataService;
import com.diboot.framework.service.impl.BaseServiceImpl;
import com.diboot.framework.utils.*;
import org.apache.commons.lang3.StringUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.ModelMap;
import org.springframework.validation.BindingResult;
import org.springframework.validation.ObjectError;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpSession;
import java.util.*;
/***
* Controller的父类
* @author [email protected]
* @version 20161107
*
*/
@Controller
public class BaseController {
private static final Logger logger = LoggerFactory.getLogger(BaseController.class);
/***
* URL跳转的前缀
*/
protected static final String REDIRECT_TO = "redirect:";
/***
* 分页参数名
*/
protected static final String PARAM_PAGE = "page";
/**
* 分页参数名
*/
protected static final String PARAM_PAGESIZE = "pageSize";
/***
* 排序字段参数名
*/
protected static final String PARAM_ORDERBY = "_orderBy";
/***
* model对象变量名常量
*/
protected static final String MODEL = "model";
/**
* modelList对象变量名常量
*/
protected static final String MODEL_LIST = "modelList";
/**
* 是否启用追踪日志
*/
protected boolean isEnabledOperationLog = BaseConfig.isTrue("diboot.log.operation-enabled");
/***
* 是否启用访问日志
*/
protected boolean isEnabledAccessLog = BaseConfig.isTrue("diboot.log.access-enabled");
/**
* 错误关键字
*/
protected static final String ERROR = "error";
/***
* 提示消息的key
*/
protected static final String KEY_PROMPT_MSG = "promptMsg";
/***
* 操作结果提示信息的key
*/
private static final String KEY_RESULT = "result";
/**
* 日志记录Logger
*/
@Autowired
protected AsyncLogger asyncLogger;
/***
* 元数据Service
*/
@Autowired
protected MetadataService metadataService;
/***
* 文件上传Service
*/
@Autowired
protected BaseFileService baseFileService;
/***
* 获取view视图页面的path前缀(文件夹路径)
* @return
*/
protected String getViewPrefix(){
return null;
}
/***
* 获取URL的前缀 /xx,默认=/+getViewPrefix
* @return
*/
protected String getUrlPrefix(){
String viewPrefix = getViewPrefix();
if(V.notEmpty(viewPrefix)){
return viewPrefix.startsWith("/")? viewPrefix : "/"+viewPrefix;
}
return null;
}
/***
* 跳转到URL
* @param url
* @return
*/
protected String redirectTo(Object url){
return REDIRECT_TO + url;
}
/***
* view路径+文件名
* @param fileName
* @return
*/
public String view(HttpServletRequest request, ModelMap model, String fileName){
// 绑定操作结果
bindResultMsg(request, model);
// 更新缓存
HttpSession session = request.getSession(false);
if(session != null){
BaseUser user = BaseHelper.getCurrentUser();
if(user != null){
if(session.getAttribute("user") == null){
session.setAttribute("user", user);
}
}
}
return getViewPrefix()+"/"+fileName;
}
/***
* 获取操作结果信息
* @param request
* @param model
*/
protected void bindResultMsg(HttpServletRequest request, ModelMap model){
// 将闪现信息从Session中读取
HttpSession session = request.getSession(false);
if(session != null){
String[] msg = (String[]) session.getAttribute(KEY_RESULT);
if(msg != null){
model.put(KEY_RESULT, msg);
session.removeAttribute(KEY_RESULT);
}
}
}
/***
* 添加值到缓存
* @param request
* @param attrName
* @param value
*/
protected void addIntoSession(HttpServletRequest request, String attrName, Object value){
HttpSession session = request.getSession(false);
if(session != null){
session.setAttribute(attrName, value);
}
}
/***
* 从缓存获取对象
* @param request
* @param attrName
* @return
*/
protected Object getFromSession(HttpServletRequest request, String attrName) {
HttpSession session = request.getSession(false);
if (session != null) {
return session.getAttribute(attrName);
}
return null;
}
/***
* 添加操作结果信息
* @param request
* @param success
* @param msg
*/
protected void addResultMsg(HttpServletRequest request, boolean success, String msg){
// 将闪现信息缓存到Session
addIntoSession(request, KEY_RESULT, new String[]{String.valueOf(success), msg});
}
/***
* 添加操作成功的结果信息,用于UI端提示用户
* @param request
* @param msg
*/
protected void addSuccessResultMsg(HttpServletRequest request, String msg){
// 将闪现信息缓存到Session
addIntoSession(request, KEY_RESULT, new String[]{"true", msg});
}
/***
* 添加操作失败的结果信息,用于UI端提示用户
* @param request
* @param msg
*/
protected void addFailResultMsg(HttpServletRequest request, String msg){
// 将闪现信息缓存到Session
addIntoSession(request, KEY_RESULT, new String[]{"false", msg});
}
/**
* 绑定指定的一个错误信息
* @return
*/
protected void bindError(ModelMap model, String errorMsg){
model.put("errors", errorMsg);
}
/**
* 添加附加的提示信息,加在操作成功/失败的提示后面
* @return
*/
protected void addAdditionalPromptMsg(ModelMap model, String promptMsg){
model.put(KEY_PROMPT_MSG, promptMsg);
}
/**
* 解析所有的验证错误信息
* @param result
* @return
*/
protected void bindErrors(ModelMap model, BindingResult result){
if(result == null || !result.hasErrors()){
return;
}
List errors = result.getAllErrors();
StringBuilder sb = new StringBuilder("");
for(ObjectError error : errors){
sb.append("- ").append(error.getDefaultMessage().replaceAll("\"", "'")).append("
");
}
sb.append("
");
model.put("errors", sb.toString());
}
/**
* 解析所有的验证错误信息,转换为JSON
* @param result
* @return
*/
protected String getBindingError(BindingResult result){
if(result == null || !result.hasErrors()){
return null;
}
List errors = result.getAllErrors();
List allErrors = new ArrayList(errors.size());
for(ObjectError error : errors){
allErrors.add(error.getDefaultMessage().replaceAll("\"", "'"));
}
return S.join(allErrors);
}
/**
* 封装错误信息
* @param errorMsg
* @return
*/
protected Map buildJsonError(String errorMsg){
Map map = new HashMap<>(4);
List allErrors = new ArrayList<>();
allErrors.add(errorMsg);
map.put("errors", allErrors);
return map;
}
/***
* new查询条件
* @param key
* @param value
* @return
*/
protected Map newCriteria(String key, Object value) {
Map criteria = new HashMap<>(8);
criteria.put(key, value);
return criteria;
}
/***
* 添加查询条件到ModelMap
* @param modelMap
* @param criteria
* @return
*/
protected void bindCriteria(ModelMap modelMap, Map criteria) {
modelMap.addAttribute("criteria", criteria);
}
/***
* 构建分页信息
* @param baseUrl
* @param criteria
* @param totalCount
* @param currentPage
* @param pageSize
* @return
*/
protected Map buildPagination(String baseUrl, Map criteria, int totalCount, int currentPage, int... pageSize) {
// 获取记录总数,用于前端显示分页
Map result = new HashMap(16);
result.put("baseUrl", baseUrl);
result.put("current", currentPage);
result.put("totalCount", totalCount);
result.put("params", criteria2paramString(criteria));
int pageNumber = BaseConfig.getPageSize();
if(pageSize != null && pageSize.length > 0){
pageNumber = pageSize[0];
}
result.put(PARAM_PAGESIZE, pageNumber);
int totalPages = (int)Math.ceil((float)totalCount/pageNumber);
result.put("totalPages", totalPages);
// 计算需要显示的页码
int showPageCount = BaseConfig.getShowPagesNum();
// 总页数不满 全部显示
int pageStart = 1, pageEnd = totalPages;
if(totalPages > showPageCount){
pageStart = currentPage - showPageCount/2 - 1;
if(pageStart < 1){
pageStart = 1;
}
pageEnd = currentPage + showPageCount/2;
if((pageEnd - pageStart) < showPageCount){
pageEnd = pageEnd + (showPageCount - (pageEnd - pageStart)) - 1;
}
if(pageEnd > totalPages){
pageStart = pageStart - (pageEnd -totalPages);
pageEnd = totalPages;
}
}
List diaplayPages = new ArrayList();
for (int i = pageStart; i <= pageEnd; i++) {
diaplayPages.add(i);
}
result.put("pages", diaplayPages);
return result;
}
/***
* 查询条件转换为url参数字符串
* @param criteria
* @return
*/
protected String criteria2paramString(Map criteria){
String result = "";
if(criteria != null && !criteria.isEmpty()){
for(Map.Entry entry : criteria.entrySet()){
if(BaseServiceImpl.OFFSET.equals(entry.getKey()) || BaseServiceImpl.COUNT.equals(entry.getKey()) || entry.getKey().startsWith(Query.C.ORDERBY_.name())){
continue;
}
if(entry.getValue() instanceof Set || entry.getValue() instanceof List || entry.getValue().getClass().isArray()){
logger.info("查询条件转url参数时忽略集合类型参数: "+entry.getKey());
continue;
}
String value = String.valueOf(entry.getValue());
if(StringUtils.isNotBlank(value)){
if(result == ""){
result = "?" + entry.getKey()+"="+value;
}
else{
result += "&" + entry.getKey()+"="+value;
}
}
}
}
return result;
}
/***
* 是否继续创建
* @param request
* @return
*/
public boolean isContinue(HttpServletRequest request){
return "true".equals(request.getParameter("continue"));
}
/***
* 构建查询条件Model
* @param request
* @return
*/
public Map buildQueryCriteria(HttpServletRequest request, int pageIndex) throws Exception{
Map result = getParamsMap(request);
return result;
}
/***
* 附加更多条件到modelMap中的criteria
* @param criteria
* @param modelMap
*/
protected void appendAdditionalCriteria(Map criteria, ModelMap modelMap){
// 是否已经存在指定条件,如存在则附加
if(modelMap.get("criteria") != null){
Map additionalCriteria = (Map) modelMap.get("criteria");
if(additionalCriteria != null && !additionalCriteria.isEmpty()){
for(Map.Entry entry : additionalCriteria.entrySet()){
criteria.put(entry.getKey(), entry.getValue());
}
}
}
}
/***
* 获取请求参数Map
* @param request
* @return
*/
public Map getParamsMap(HttpServletRequest request) throws Exception{
Map result = new HashMap(16);
Enumeration paramNames = request.getParameterNames();
while (paramNames.hasMoreElements()){
String paramName = (String) paramNames.nextElement();
// 拦截掉伪造的排序条件
if(paramName.startsWith(Query.C.ORDERBY_.name())){
continue;
}
String[] values = request.getParameterValues(paramName);
if(V.notEmpty(values)){
if(values.length == 1){
if(V.notEmpty(values[0])){
String paramValue = java.net.URLDecoder.decode(values[0], "utf-8");
result.put(paramName, paramValue);
// 针对OrderBy排序字段特殊处理
if(PARAM_ORDERBY.equals(paramName) && V.notEmpty(paramValue)){
String fieldName = paramValue, type = "ASC";
if(paramValue.contains(":")){
String[] orderCondition = paramValue.split(":");
if("ASC".equalsIgnoreCase(orderCondition[1]) || "DESC".equalsIgnoreCase(orderCondition[1])){
type = orderCondition[1].toUpperCase();
}
fieldName = orderCondition[0];
}
// 查询条件
result.put(Query.C.ORDERBY_+fieldName, type);
}
}
}
else{
String[] valueArray = new String[values.length];
for(int i=0; i getStringList(HttpServletRequest request, String param){
String[] strArray = getStringArray(request, param);
if(V.isEmpty(strArray)){
return null;
}
return new ArrayList<>(Arrays.asList(strArray));
}
/***
* 从request里获取Long列表
* @param request
* @param param
* @return
*/
public List getLongList(HttpServletRequest request, String param){
String[] strArray = getStringArray(request, param);
if(V.isEmpty(strArray)){
return null;
}
List longList = new ArrayList<>();
for(String str : strArray){
if(V.notEmpty(str)){
longList.add(Long.parseLong(str));
}
}
return longList;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy