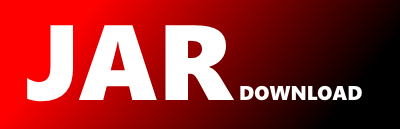
com.diboot.framework.utils.BeanUtils Maven / Gradle / Ivy
The newest version!
package com.diboot.framework.utils;
import com.diboot.framework.config.BaseCons;
import com.diboot.framework.model.BaseModel;
import com.diboot.framework.model.BaseTreeModel;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.BeanWrapper;
import org.springframework.beans.PropertyAccessorFactory;
import org.springframework.cglib.beans.BeanCopier;
import javax.servlet.http.HttpServletRequest;
import java.beans.BeanInfo;
import java.beans.Introspector;
import java.beans.PropertyDescriptor;
import java.util.*;
import java.util.concurrent.ConcurrentHashMap;
/**
* Model相关处理Helper类
* @author [email protected]
* @version 2017/8/12
*
*/
public class BeanUtils{
private static final Logger logger = LoggerFactory.getLogger(BeanUtils.class);
/**
* 连接符号
*/
private static final String CHANGE_FLAG = "->";
/**
* 关联连接符号
*/
private static final String REF_FLAG = "=";
/**
* 忽略对比的字段
*/
private static final Set IGNORE_FIELDS = new HashSet(){{
add(BaseModel.F.createTime);
add("new");
add("modelName");
}};
/***
* 缓存BeanCopier
*/
private static Map BEAN_COPIER_INST_MAP = new ConcurrentHashMap<>();
/***
* 获取实例
* @param source
* @param target
* @return
*/
private static BeanCopier getBeanCopierInstance(Object source, Object target){
//build key
String beanCopierKey = source.getClass().toString() +"_"+ target.getClass().toString();
BeanCopier copierInst = BEAN_COPIER_INST_MAP.get(beanCopierKey);
if(copierInst == null){
copierInst = BeanCopier.create(source.getClass(), target.getClass(), false);
BEAN_COPIER_INST_MAP.put(beanCopierKey, copierInst);
}
return copierInst;
}
/**
* Copy属性到另一个对象
* @param source
* @param target
*/
public static Object copyProperties(Object source, Object target){
BeanCopier copierInst = getBeanCopierInstance(source, target);
copierInst.copy(source, target, null);
return target;
}
/**
* 将字符串转换为指定类型的对象
* @param type
* @param value
* @return
* @throws Exception
*/
public static Object convertValue2Type(String value, String type) throws Exception{
if (V.isEmpty(type)){
throw new Exception("参数传递错误:类型未定义!");
}
// 如果值为空 返回包装类型null
if (value == null){
return value;
}
type = S.trim(type);
// 处理boolean值
if (Boolean.class.getSimpleName().equalsIgnoreCase(type)){
return V.isTrue(value);
}
// 其他情况交由BeanUtils转换
// 其他情况直接返回string
return value;
}
/***
* 附加Map中的属性值到Model
* @param model
* @param propMap
*/
public static void bindProperties(BaseModel model, Map propMap){
try{// 获取类属性
BeanInfo beanInfo = Introspector.getBeanInfo(model.getClass());
// 给 JavaBean 对象的属性赋值
PropertyDescriptor[] propertyDescriptors = beanInfo.getPropertyDescriptors();
for (PropertyDescriptor descriptor : propertyDescriptors) {
String propertyName = descriptor.getName();
if (propMap.containsKey(propertyName)){
Object value = propMap.get(propertyName);
Class type = descriptor.getWriteMethod().getParameterTypes()[0];
Object[] args = new Object[1];
String fieldType = type.getSimpleName();
// 类型不一致,需转型
if(!value.getClass().getTypeName().equals(fieldType)){
if(value instanceof String){
// String to Date
if(fieldType.equalsIgnoreCase(Date.class.getSimpleName())){
args[0] = D.fuzzyConvert((String)value);
}
// Map中的String型转换为其他型
else if(fieldType.equalsIgnoreCase(Boolean.class.getSimpleName())){
args[0] = V.isTrue((String)value);
}
else if (fieldType.equalsIgnoreCase(Integer.class.getSimpleName()) || "int".equals(fieldType)) {
args[0] = Integer.parseInt((String)value);
}
else if (fieldType.equalsIgnoreCase(Long.class.getSimpleName())) {
args[0] = Long.parseLong((String)value);
}
else if (fieldType.equalsIgnoreCase(Double.class.getSimpleName())) {
args[0] = Double.parseDouble((String)value);
}
else if (fieldType.equalsIgnoreCase(Float.class.getSimpleName())) {
args[0] = Float.parseFloat((String)value);
}
else{
args[0] = value;
logger.warn("类型不一致,暂无法自动绑定,请手动转型一致后调用!字段类型="+fieldType);
}
}
else{
args[0] = value;
logger.warn("类型不一致,且Map中的value非String类型,暂无法自动绑定,请手动转型一致后调用!value="+value);
}
}
else{
args[0] = value;
}
descriptor.getWriteMethod().invoke(model, args);
}
}
}
catch (Exception e){
logger.warn("复制Map属性到Model异常: " + e.getMessage(), e);
}
}
/***
* 附加属性值到Model (已废弃,请调用bindProperties)
* @param model
* @param propMap
*/
@Deprecated
public static void populate(BaseModel model, Map propMap){
bindProperties(model, propMap);
}
/***
* 将请求参数值绑定成Model
* @param request
*/
public static void buildModel(BaseModel model, HttpServletRequest request){
Map propMap = BaseHelper.convertParams2Map(request);
bindProperties(model, propMap);
}
/***
* 获取对象的属性值
* @param obj
* @param field
* @return
*/
public static Object getProperty(Object obj, String field){
BeanWrapper wrapper = PropertyAccessorFactory.forBeanPropertyAccess(obj);
return wrapper.getPropertyValue(field);
}
/***
* 设置属性值
* @param model
* @param field
* @param value
*/
public static void setProperty(Object model, String field, Object value) {
BeanWrapper wrapper = PropertyAccessorFactory.forBeanPropertyAccess(model);
wrapper.setPropertyValue(field, value);
}
/***
* Key-Model对象Map
* @param allLists
* @return
*/
public static Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy