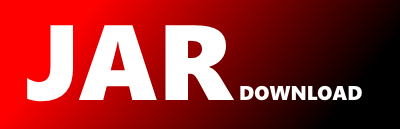
com.diffplug.common.swt.dnd.StructuredDrag Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of durian-swt Show documentation
Show all versions of durian-swt Show documentation
DurianSwt - Reactive utilities and fluent builders for SWT
The newest version!
/*
* Copyright 2020 DiffPlug
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.diffplug.common.swt.dnd;
import com.diffplug.common.base.Preconditions;
import com.diffplug.common.base.Unhandled;
import com.diffplug.common.collect.ImmutableList;
import com.diffplug.common.collect.ImmutableMap;
import com.diffplug.common.rx.RxBox;
import com.diffplug.common.rx.RxGetter;
import com.diffplug.common.swt.SwtMisc;
import java.io.File;
import java.util.Arrays;
import java.util.HashMap;
import java.util.IdentityHashMap;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Objects;
import java.util.function.Function;
import java.util.function.Predicate;
import javax.annotation.Nullable;
import org.eclipse.swt.dnd.Clipboard;
import org.eclipse.swt.dnd.DND;
import org.eclipse.swt.dnd.DragSource;
import org.eclipse.swt.dnd.DragSourceEvent;
import org.eclipse.swt.dnd.DragSourceListener;
import org.eclipse.swt.dnd.FileTransfer;
import org.eclipse.swt.dnd.TextTransfer;
import org.eclipse.swt.dnd.Transfer;
import org.eclipse.swt.widgets.Control;
/**
* Typed mechanism for implementing drag listeners.
*
* https://eclipse.org/articles/Article-SWT-DND/DND-in-SWT.html
*/
public class StructuredDrag {
public enum DropResult {
NO_DROP, COPIED, MOVED;
}
@FunctionalInterface
public interface TypedDragHandler {
/** DragSourceEvent will be null if it's starting from a 'Ctrl+C' copy. */
T dragStartData(@Nullable DragSourceEvent e);
default void dropped(DragSourceEvent e, T value, DropResult result) {}
default TypedDragHandler map(Function mapper) {
return new MappedTypedDragHandler(this, mapper);
}
}
private static class MappedTypedDragHandler implements TypedDragHandler {
TypedDragHandler delegate;
Function mapper;
MappedTypedDragHandler(TypedDragHandler delegate, Function mapper) {
this.delegate = Objects.requireNonNull(delegate);
this.mapper = Objects.requireNonNull(mapper);
}
T lastOriginal;
R lastMapped;
int count = 0;
@Override
public R dragStartData(DragSourceEvent e) {
T original = delegate.dragStartData(e);
if (original == null) {
lastOriginal = null;
lastMapped = null;
count = 0;
e.doit = false;
} else if (original.equals(lastOriginal)) {
++count;
} else {
lastOriginal = original;
lastMapped = mapper.apply(lastOriginal);
count = 1;
}
return lastMapped;
}
@Override
public void dropped(DragSourceEvent e, R value, DropResult moved) {
Preconditions.checkArgument(value.equals(lastMapped), "dropped=%s lastMapped=%s", value, lastMapped);
delegate.dropped(e, lastOriginal, moved);
if (--count == 0) {
lastOriginal = null;
lastMapped = null;
}
}
}
@FunctionalInterface
public interface TriConsumer {
void accept(A a, B b, C c);
}
private static class Handler {
Predicate
© 2015 - 2025 Weber Informatics LLC | Privacy Policy