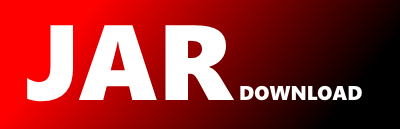
com.diffplug.common.swt.jface.LabelProviders Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of durian-swt Show documentation
Show all versions of durian-swt Show documentation
DurianSwt - Reactive utilities and fluent builders for SWT
The newest version!
/*
* Copyright 2020 DiffPlug
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.diffplug.common.swt.jface;
import com.diffplug.common.base.Functions;
import java.util.function.Function;
import org.eclipse.jface.viewers.CellLabelProvider;
import org.eclipse.jface.viewers.ColumnLabelProvider;
import org.eclipse.jface.viewers.ILabelProvider;
import org.eclipse.swt.graphics.Color;
import org.eclipse.swt.graphics.Font;
import org.eclipse.swt.graphics.Image;
/** Utilities for creating JFace {@link CellLabelProvider} (which is also appropriate for plain-jane {@link ILabelProvider}). */
public class LabelProviders {
/** Returns a fluent builder for creating a {@link ColumnLabelProvider}. */
public static Builder builder() {
return new Builder();
}
/** Creates a {@link ColumnLabelProvider} for text. */
public static ColumnLabelProvider createWithText(Function super T, ? extends String> text) {
Builder builder = builder();
builder.setText(text);
return builder.build();
}
/** Creates a {@link ColumnLabelProvider} for images. */
public static ColumnLabelProvider createWithImage(Function super T, ? extends Image> image) {
Builder builder = builder();
builder.setImage(image);
return builder.build();
}
/** Creates a {@link ColumnLabelProvider} for text and images. */
public static ColumnLabelProvider createWithTextAndImage(Function super T, ? extends String> text, Function super T, ? extends Image> image) {
Builder builder = builder();
builder.setText(text);
builder.setImage(image);
return builder.build();
}
/** An override of {@link ColumnLabelProvider}. */
@SuppressWarnings("unchecked")
private static class Imp extends ColumnLabelProvider {
private final Function super T, ? extends String> text;
private final Function super T, ? extends Image> image;
private final Function super T, ? extends Color> background;
private final Function super T, ? extends Color> foreground;
private final Function super T, ? extends Font> font;
private Imp(Function super T, ? extends String> text, Function super T, ? extends Image> image, Function super T, ? extends Color> background, Function super T, ? extends Color> foreground, Function super T, ? extends Font> font) {
this.text = text;
this.image = image;
this.background = background;
this.foreground = foreground;
this.font = font;
}
@Override
public String getText(Object element) {
return text.apply((T) element);
}
@Override
public Image getImage(Object element) {
return image.apply((T) element);
}
@Override
public Color getBackground(Object element) {
return background.apply((T) element);
}
@Override
public Color getForeground(Object element) {
return foreground.apply((T) element);
}
@Override
public Font getFont(Object element) {
return font.apply((T) element);
}
}
/** A fluent API for creating a {@link ColumnLabelProvider}. */
public static class Builder extends ColumnLabelProvider {
private Function super T, ? extends String> text = Functions.constant(null);
private Function super T, ? extends Image> image = Functions.constant(null);
private Function super T, ? extends Color> background = Functions.constant(null);
private Function super T, ? extends Color> foreground = Functions.constant(null);
private Function super T, ? extends Font> font = Functions.constant(null);
private Builder() {}
/** Sets the function used to determine the text. */
public Builder setText(Function super T, ? extends String> text) {
this.text = text;
return this;
}
/** Sets the function used to determine the image. */
public Builder setImage(Function super T, ? extends Image> image) {
this.image = image;
return this;
}
/** Sets the function used to determine the background color. */
public Builder setBackground(Function super T, ? extends Color> background) {
this.background = background;
return this;
}
/** Sets the function used to determine the foreground color. */
public Builder setForeground(Function super T, ? extends Color> foreground) {
this.foreground = foreground;
return this;
}
/** Sets the function used to determine the font. */
public Builder setFont(Function super T, ? extends Font> font) {
this.font = font;
return this;
}
/** Returns a new ColumnLabelProvider. */
public ColumnLabelProvider build() {
return new Imp(text, image, background, foreground, font);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy