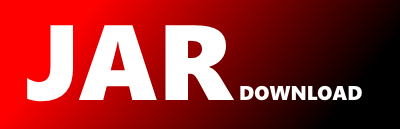
com.diffplug.common.swt.widgets.RadioGroup Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of durian-swt Show documentation
Show all versions of durian-swt Show documentation
DurianSwt - Reactive utilities and fluent builders for SWT
The newest version!
/*
* Copyright 2020 DiffPlug
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.diffplug.common.swt.widgets;
import com.diffplug.common.base.Preconditions;
import com.diffplug.common.collect.BiMap;
import com.diffplug.common.collect.HashBiMap;
import com.diffplug.common.collect.Iterables;
import com.diffplug.common.collect.Maps;
import com.diffplug.common.rx.RxBox;
import com.diffplug.common.swt.Coat;
import com.diffplug.common.swt.Layouts;
import com.diffplug.common.swt.SwtExec;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.Optional;
import java.util.function.Function;
import org.eclipse.swt.SWT;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Composite;
public class RadioGroup {
private final Optional> source;
private LinkedHashMap options = Maps.newLinkedHashMap();
/** Guava-style constructor. */
public static RadioGroup create() {
return new RadioGroup(Optional.empty());
}
/** Guava-style constructor. */
public static RadioGroup create(RxBox source) {
return new RadioGroup(Optional.of(source));
}
protected RadioGroup(Optional> source) {
this.source = source;
}
public RadioGroup addOption(T value, String name) {
options.put(name, value);
return this;
}
public RadioGroup addOptions(Iterable values, Function labelProvider) {
for (T value : values) {
addOption(value, labelProvider.apply(value));
}
return this;
}
public RxBox buildOn(Composite cmp) {
// object which will hold the current selection
RxBox selection = source.orElseGet(() -> RxBox.of(Iterables.get(options.values(), 0)));
// mapping from button to objects
BiMap
© 2015 - 2025 Weber Informatics LLC | Privacy Policy