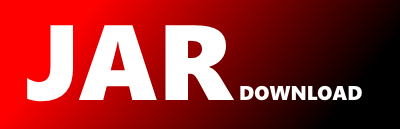
com.diffplug.common.base.Errors Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of durian Show documentation
Show all versions of durian Show documentation
Durian - Guava's spikier (unofficial) cousin
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy