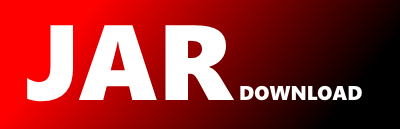
com.diffplug.common.base.Predicates Maven / Gradle / Ivy
/*
* Copyright 2015 DiffPlug
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.diffplug.common.base;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.Objects;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.regex.Pattern;
import javax.annotation.Nullable;
/**
* Helper functions for manipulating {@link Predicate}, copied from Guava.
*
* The function signatures below are identical to Google's Guava 18.0, except that guava's functional
* interfaces have been swapped with Java 8's. It is tested against the same test suite
* as Google Guava to ensure functional compatibility.
*
* Most of the implementation has been replaced with lambdas, which means that the following
* functionality has been removed: equals(), hashCode(), toString(), GWT, and serialization.
*
* Lambdas don't support these methods, and there isn't much reason why they should, so we
* removed them in Durian.
*/
public final class Predicates {
private Predicates() {}
/**
* Returns a predicate that always evaluates to {@code true}.
*/
public static Predicate alwaysTrue() {
return t -> true;
}
/**
* Returns a predicate that always evaluates to {@code false}.
*/
public static Predicate alwaysFalse() {
return t -> false;
}
/**
* Returns a predicate that evaluates to {@code true} if the object reference
* being tested is null.
*/
public static Predicate isNull() {
return Objects::isNull;
}
/**
* Returns a predicate that evaluates to {@code true} if the object reference
* being tested is not null.
*/
public static Predicate notNull() {
return Objects::nonNull;
}
/**
* Returns a predicate that evaluates to {@code true} if the given predicate
* evaluates to {@code false}.
*/
public static Predicate not(Predicate predicate) {
return predicate.negate();
}
/**
* Returns a predicate that evaluates to {@code true} if each of its
* components evaluates to {@code true}. The components are evaluated in
* order, and evaluation will be "short-circuited" as soon as a false
* predicate is found. It defensively copies the iterable passed in, so future
* changes to it won't alter the behavior of this predicate. If {@code
* components} is empty, the returned predicate will always evaluate to {@code
* true}.
*/
public static Predicate and(Iterable extends Predicate super T>> components) {
return new AndPredicate(defensiveCopy(components));
}
/**
* Returns a predicate that evaluates to {@code true} if each of its
* components evaluates to {@code true}. The components are evaluated in
* order, and evaluation will be "short-circuited" as soon as a false
* predicate is found. It defensively copies the array passed in, so future
* changes to it won't alter the behavior of this predicate. If {@code
* components} is empty, the returned predicate will always evaluate to {@code
* true}.
*/
@SafeVarargs
public static Predicate and(Predicate super T>... components) {
return new AndPredicate(defensiveCopy(components));
}
/**
* Returns a predicate that evaluates to {@code true} if both of its
* components evaluate to {@code true}. The components are evaluated in
* order, and evaluation will be "short-circuited" as soon as a false
* predicate is found.
*/
public static Predicate and(Predicate super T> first, Predicate super T> second) {
return new AndPredicate(Predicates. asList(Objects.requireNonNull(first), Objects.requireNonNull(second)));
}
/**
* Returns a predicate that evaluates to {@code true} if any one of its
* components evaluates to {@code true}. The components are evaluated in
* order, and evaluation will be "short-circuited" as soon as a
* true predicate is found. It defensively copies the iterable passed in, so
* future changes to it won't alter the behavior of this predicate. If {@code
* components} is empty, the returned predicate will always evaluate to {@code
* false}.
*/
public static Predicate or(Iterable extends Predicate super T>> components) {
return new OrPredicate(defensiveCopy(components));
}
/**
* Returns a predicate that evaluates to {@code true} if any one of its
* components evaluates to {@code true}. The components are evaluated in
* order, and evaluation will be "short-circuited" as soon as a
* true predicate is found. It defensively copies the array passed in, so
* future changes to it won't alter the behavior of this predicate. If {@code
* components} is empty, the returned predicate will always evaluate to {@code
* false}.
*/
@SafeVarargs
public static Predicate or(Predicate super T>... components) {
return new OrPredicate(defensiveCopy(components));
}
/**
* Returns a predicate that evaluates to {@code true} if either of its
* components evaluates to {@code true}. The components are evaluated in
* order, and evaluation will be "short-circuited" as soon as a
* true predicate is found.
*/
public static Predicate or(Predicate super T> first, Predicate super T> second) {
return new OrPredicate(Predicates. asList(Objects.requireNonNull(first), Objects.requireNonNull(second)));
}
/**
* Returns a predicate that evaluates to {@code true} if the object being
* tested {@code equals()} the given target or both are null.
*/
public static Predicate equalTo(@Nullable T target) {
return target == null ? isNull() : t -> Objects.equals(t, target);
}
/**
* Returns a predicate that evaluates to {@code true} if the object being
* tested is an instance of the given class. If the object being tested
* is {@code null} this predicate evaluates to {@code false}.
*/
public static Predicate