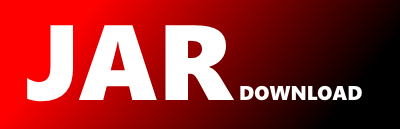
matlabcontrol.demo.ArrayPanel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of matconsolectl Show documentation
Show all versions of matconsolectl Show documentation
MatConsoleCtl - control MATLAB from Java
/*
* Code licensed under new-style BSD (see LICENSE).
* All code up to tags/original: Copyright (c) 2013, Joshua Kaplan
* All code after tags/original: Copyright (c) 2016, DiffPlug
*/
package matlabcontrol.demo;
import java.awt.Color;
import java.awt.GridLayout;
import java.util.ArrayList;
import javax.swing.JComboBox;
import javax.swing.JPanel;
import javax.swing.JTextField;
/**
* The panel that contains the options to select elements of the array.
*
* @author Joshua Kaplan
*/
@SuppressWarnings("serial")
class ArrayPanel extends JPanel {
//Input options: String or Double
private static final int DOUBLE_INDEX = 0, STRING_INDEX = 1;
private static final String[] OPTIONS = new String[2];
static {
OPTIONS[DOUBLE_INDEX] = "Double";
OPTIONS[STRING_INDEX] = "String";
}
/**
* Number of fields and drop down lists.
*/
public static final int NUM_ENTRIES = 3;
/**
* Drop down lists to choose between object types.
*/
private final JComboBox[] _optionBoxes;
/**
* Fields for inputting values.
*/
private final JTextField[] _entryFields;
public ArrayPanel() {
super(new GridLayout(NUM_ENTRIES, 2));
this.setBackground(Color.WHITE);
//Drop down lists and input fields
_optionBoxes = new JComboBox[NUM_ENTRIES];
_entryFields = new JTextField[NUM_ENTRIES];
for (int i = 0; i < NUM_ENTRIES; i++) {
_optionBoxes[i] = new JComboBox(OPTIONS);
_entryFields[i] = new JTextField(8);
this.add(_optionBoxes[i]);
this.add(_entryFields[i]);
}
}
/**
* Take the elements of the fields and put them into an array.
*
* @return
*/
public Object[] getArray() {
ArrayList
© 2015 - 2024 Weber Informatics LLC | Privacy Policy