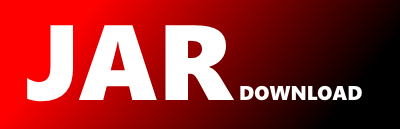
matlabcontrol.extensions.MatlabTypeConverter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of matconsolectl Show documentation
Show all versions of matconsolectl Show documentation
MatConsoleCtl - control MATLAB from Java
/*
* Code licensed under new-style BSD (see LICENSE).
* All code up to tags/original: Copyright (c) 2013, Joshua Kaplan
* All code after tags/original: Copyright (c) 2016, DiffPlug
*/
package matlabcontrol.extensions;
import java.io.Serializable;
import matlabcontrol.MatlabInvocationException;
import matlabcontrol.MatlabProxy;
import matlabcontrol.MatlabProxy.MatlabThreadCallable;
import matlabcontrol.MatlabProxy.MatlabThreadProxy;
/**
* Converts between MATLAB and Java types. Currently only supports numeric arrays.
*
* This class is unconditionally thread-safe.
*
* @since 4.0.0
*
* @author Joshua Kaplan
*/
public class MatlabTypeConverter {
private final MatlabProxy _proxy;
/**
* Constructs the converter.
*
* @param proxy
*/
public MatlabTypeConverter(MatlabProxy proxy) {
_proxy = proxy;
}
/**
* Retrieves the MATLAB numeric array with the variable name {@code arrayName}.
*
* @param arrayName
* @return the retrieved numeric array
* @throws matlabcontrol.MatlabInvocationException if thrown by the proxy
*/
public MatlabNumericArray getNumericArray(String arrayName) throws MatlabInvocationException {
ArrayInfo info = _proxy.invokeAndWait(new GetArrayCallable(arrayName));
return new MatlabNumericArray(info.real, info.imaginary, info.lengths);
}
private static class GetArrayCallable implements MatlabThreadCallable, Serializable {
private static final long serialVersionUID = -1269094288040717603L;
private final String _arrayName;
public GetArrayCallable(String arrayName) {
_arrayName = arrayName;
}
@Override
public ArrayInfo call(MatlabThreadProxy proxy) throws MatlabInvocationException {
//Retrieve real values
Object realObject = proxy.returningEval("real(" + _arrayName + ");", 1)[0];
double[] realValues = (double[]) realObject;
//Retrieve imaginary values if present
boolean isReal = ((boolean[]) proxy.returningEval("isreal(" + _arrayName + ");", 1)[0])[0];
double[] imaginaryValues = null;
if (!isReal) {
Object imaginaryObject = proxy.returningEval("imag(" + _arrayName + ");", 1)[0];
imaginaryValues = (double[]) imaginaryObject;
}
//Retrieve lengths of array
double[] size = (double[]) proxy.returningEval("size(" + _arrayName + ");", 1)[0];
int[] lengths = new int[size.length];
for (int i = 0; i < size.length; i++) {
lengths[i] = (int) size[i];
}
return new ArrayInfo(realValues, imaginaryValues, lengths);
}
}
private static class ArrayInfo implements Serializable {
private static final long serialVersionUID = 4464014916120235711L;
private final double[] real, imaginary;
private final int[] lengths;
public ArrayInfo(double[] real, double[] imaginary, int[] lengths) {
this.real = real;
this.imaginary = imaginary;
this.lengths = lengths;
}
}
/**
* Stores the {@code array} in MATLAB with the variable name {@code arrayName}.
*
* @param arrayName the variable name
* @param array
* @throws matlabcontrol.MatlabInvocationException if thrown by the proxy
*/
public void setNumericArray(String arrayName, MatlabNumericArray array) throws MatlabInvocationException {
_proxy.invokeAndWait(new SetArrayCallable(arrayName, array));
}
private static class SetArrayCallable implements MatlabThreadCallable
© 2015 - 2024 Weber Informatics LLC | Privacy Policy