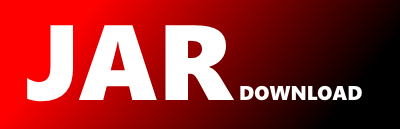
matlabcontrol.link.MatlabReturns Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of matconsolectl Show documentation
Show all versions of matconsolectl Show documentation
MatConsoleCtl - control MATLAB from Java
/*
* Code licensed under new-style BSD (see LICENSE).
* All code up to tags/original: Copyright (c) 2013, Joshua Kaplan
* All code after tags/original: Copyright (c) 2016, DiffPlug
*/
package matlabcontrol.link;
import java.util.Arrays;
/**
* MATLAB return containers.
*
* @since 4.2.0
* @author Joshua Kaplan
*/
@SuppressWarnings("unchecked")
public final class MatlabReturns {
private MatlabReturns() {}
/**
* Hidden super class of all of the {@code Return}X classes. This class is hidden because it is not a valid return
* type from a method declared in an interface provided to {@link MatlabFunctionLinker} and there is no need for a
* user to make use of this class.
*/
static class ReturnN {
private final Object[] _values;
ReturnN(Object[] values) {
_values = values;
}
Object get(int i) {
return _values[i];
}
/**
* Returns a brief description of this container. The exact details of this representation are unspecified and
* are subject to change.
*
* @return
*/
@Override
public String toString() {
return "[" + this.getClass().getCanonicalName() +
" size=" + _values.length + "," +
" values=" + Arrays.toString(_values) + "]";
}
}
/**
* Container for two MATLAB return values.
*
* @param first return type
* @param second return type
*/
public static class Return2 extends ReturnN {
Return2(Object[] values) {
super(values);
}
/**
* The first return argument.
*
* @return
*/
public A getFirst() {
return (A) get(0);
}
/**
* The second return argument.
*
* @return
*/
public B getSecond() {
return (B) get(1);
}
}
/**
* Container for three MATLAB return values.
*
* @param first return type
* @param second return type
* @param third return type
*/
public static class Return3 extends Return2 {
Return3(Object[] values) {
super(values);
}
/**
* The third return argument.
*
* @return
*/
public C getThird() {
return (C) get(2);
}
}
/**
* Container for four MATLAB return values.
*
* @param first return type
* @param second return type
* @param third return type
* @param fourth return type
*/
public static class Return4 extends Return3 {
Return4(Object[] values) {
super(values);
}
/**
* The fourth return argument.
*
* @return
*/
public D getFourth() {
return (D) get(3);
}
}
/**
* Container for five MATLAB return values.
*
* @param first return type
* @param second return type
* @param third return type
* @param fourth return type
* @param fifth return type
*/
public static class Return5 extends Return4 {
Return5(Object[] values) {
super(values);
}
/**
* The fifth return argument.
*
* @return
*/
public E getFifth() {
return (E) get(4);
}
}
/**
* Container for six MATLAB return values.
*
* @param first return type
* @param second return type
* @param third return type
* @param fourth return type
* @param fifth return type
* @param sixth return type
*/
public static class Return6 extends Return5 {
Return6(Object[] values) {
super(values);
}
/**
* The sixth return argument.
*
* @return
*/
public F getSixth() {
return (F) get(5);
}
}
/**
* Container for seven MATLAB return values.
*
* @param first return type
* @param second return type
* @param third return type
* @param fourth return type
* @param fifth return type
* @param sixth return type
* @param seventh return type
*/
public static class Return7 extends Return6 {
Return7(Object[] values) {
super(values);
}
/**
* The seventh return argument.
*
* @return
*/
public G getSeventh() {
return (G) get(6);
}
}
/**
* Container for eight MATLAB return values.
*
* @param first return type
* @param second return type
* @param third return type
* @param fourth return type
* @param fifth return type
* @param sixth return type
* @param seventh return type
* @param eight return type
*/
public static class Return8 extends Return7 {
Return8(Object[] values) {
super(values);
}
/**
* The eight return argument.
*
* @return
*/
public H getEighth() {
return (H) get(7);
}
}
/**
* Container for nine MATLAB return values.
*
* @param first return type
* @param second return type
* @param third return type
* @param fourth return type
* @param fifth return type
* @param sixth return type
* @param seventh return type
* @param eight return type
* @param ninth return type
*/
public static class Return9 extends Return8 {
Return9(Object[] values) {
super(values);
}
/**
* The ninth argument.
*
* @return
*/
public I getNinth() {
return (I) get(8);
}
}
@SuppressWarnings("rawtypes")
static ReturnN getMaxReturn(Object[] values) {
return new Return9(values);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy