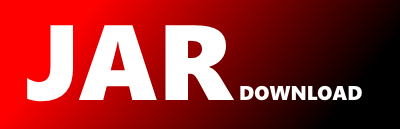
org.eclipse.osgi.internal.permadmin.EquinoxSecurityManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spotless-ext-greclipse Show documentation
Show all versions of spotless-ext-greclipse Show documentation
Groovy Eclipse's formatter bundled for Spotless
The newest version!
/*******************************************************************************
* Copyright (c) 2008, 2013 IBM Corporation and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* IBM Corporation - initial API and implementation
*******************************************************************************/
package org.eclipse.osgi.internal.permadmin;
import java.security.*;
import java.util.*;
import org.eclipse.osgi.internal.permadmin.SecurityRow.Decision;
import org.osgi.service.condpermadmin.Condition;
/**
*
* This security manager implements the ConditionalPermission processing for
* OSGi. It is to be used with ConditionalPermissionAdmin.
*
*/
public class EquinoxSecurityManager extends SecurityManager {
/*
* This is super goofy, but we need to make sure that the CheckContext and
* CheckPermissionAction classes load early. Otherwise, we run into problems later.
*/
static {
Class> c;
c = CheckPermissionAction.class;
c = CheckContext.class;
c.getName(); // to prevent compiler warnings
}
static class CheckContext {
// A non zero depth indicates that we are doing a recursive permission check.
List> depthCondSets = new ArrayList>(2);
List accs = new ArrayList(2);
List> CondClassSet;
public int getDepth() {
return depthCondSets.size() - 1;
}
}
static class CheckPermissionAction implements PrivilegedAction {
Permission perm;
Object context;
EquinoxSecurityManager fsm;
CheckPermissionAction(EquinoxSecurityManager fsm, Permission perm, Object context) {
this.fsm = fsm;
this.perm = perm;
this.context = context;
}
public Void run() {
fsm.internalCheckPermission(perm, context);
return null;
}
}
private final ThreadLocal localCheckContext = new ThreadLocal();
boolean addConditionsForDomain(Decision[] results) {
CheckContext cc = localCheckContext.get();
if (cc == null) {
// We are being invoked in a weird way. Perhaps the ProtectionDomain is
// getting invoked directly.
return false;
}
List condSets = cc.depthCondSets.get(cc.getDepth());
if (condSets == null) {
condSets = new ArrayList(1);
cc.depthCondSets.set(cc.getDepth(), condSets);
}
condSets.add(results);
return true;
}
boolean inCheckPermission() {
return localCheckContext.get() != null;
}
public void checkPermission(Permission perm, Object context) {
AccessController.doPrivileged(new CheckPermissionAction(this, perm, context));
}
/**
* Gets the AccessControlContext currently being evaluated by
* the SecurityManager.
*
* @return the AccessControlContext currently being evaluated by the SecurityManager, or
* null if no AccessControlContext is being evaluated. Note: this method will
* return null if the permission check is being done directly on the AccessControlContext
* rather than the SecurityManager.
*/
public AccessControlContext getContextToBeChecked() {
CheckContext cc = localCheckContext.get();
if (cc != null && cc.accs != null && !cc.accs.isEmpty())
return cc.accs.get(cc.accs.size() - 1);
return null;
}
void internalCheckPermission(Permission perm, Object context) {
AccessControlContext acc = (AccessControlContext) context;
CheckContext cc = localCheckContext.get();
if (cc == null) {
cc = new CheckContext();
localCheckContext.set(cc);
}
cc.depthCondSets.add(null); // initialize postponed condition set to null
cc.accs.add(acc);
try {
acc.checkPermission(perm);
// We want to pop the first set of postponed conditions and process them
List conditionSets = cc.depthCondSets.get(cc.getDepth());
if (conditionSets == null)
return;
// TODO the spec seems impossible to implement just doing the simple thing for now
Map, Dictionary
© 2015 - 2024 Weber Informatics LLC | Privacy Policy