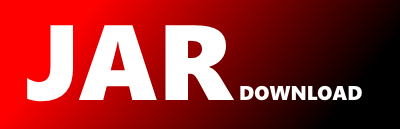
com.pushtechnology.diffusion.client.features.control.topics.views.TopicViews Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (c) 2019, 2023 DiffusionData Ltd., All Rights Reserved.
*
* Use is subject to licence terms.
*
* NOTICE: All information contained herein is, and remains the
* property of DiffusionData. The intellectual and technical
* concepts contained herein are proprietary to DiffusionData and
* may be covered by U.S. and Foreign Patents, patents in process, and
* are protected by trade secret or copyright law.
*******************************************************************************/
package com.pushtechnology.diffusion.client.features.control.topics.views;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.CompletionException;
import com.pushtechnology.diffusion.client.features.ClusterRoutingException;
import com.pushtechnology.diffusion.client.features.ScriptException;
import com.pushtechnology.diffusion.client.features.Topics;
import com.pushtechnology.diffusion.client.features.control.RemoteServers;
import com.pushtechnology.diffusion.client.session.Feature;
import com.pushtechnology.diffusion.client.session.PermissionsException;
import com.pushtechnology.diffusion.client.session.Session;
import com.pushtechnology.diffusion.client.session.SessionClosedException;
import com.pushtechnology.diffusion.client.types.GlobalPermission;
import com.pushtechnology.diffusion.client.types.PathPermission;
/**
* This feature allows a client session to manage topic views.
*
*
* A topic view maps one part of a server's topic tree to another. It
* dynamically creates a set of reference topics from a set of
* source topics, based on a declarative topic view
* specification. The capabilities of topic views range from simple
* mirroring of topics within the topic tree to advanced capabilities including
* publication of partial values, expanding a single topic value into many
* topics, changing topic values, inserting values from other topics, throttling
* the rate of publication, and applying a fixed delay to the publication.
*
*
* A topic view can also map topics from another server (in a different
* cluster). This capability is referred to as 'remote topic views'. The view
* can specify the server that the source topics are hosted on in terms
* of a remote server (see {@link RemoteServers} for details of how to
* create and maintain remote servers).
*
*
* Each reference topic has a single source topic and has the same topic type as
* its source topic. Reference topics are read-only (they cannot be updated),
* nor can they be created or removed directly. Otherwise, they behave just like
* standard topics. A client session can subscribe to a reference topic, and can
* fetch the reference topic's current value if it has one.
*
*
* The source topics of a topic view are defined by a topic selector. One or
* more reference topics are created for each source topic, according to the
* topic view. If a source topic is removed, reference topics that are derived
* from it will automatically be removed. If a topic is added that matches the
* source topic selector of a topic view, corresponding reference topics will be
* created. Removing a topic view will remove all of its reference topics.
*
*
Topic view specifications
*
* Topic views are specified using a Domain Specific Language (DSL) which
* provides many powerful features for manipulating topic data. For a full and
* detailed description of the topic views DSL see the user
* manual.
*
*
* The following is a simple topic view specification that mirrors all topics
* below the path a
to reference topics below the path
* b
.
*
*
* map ?a// to b/<path(1)>
*
*
*
* A topic view with this specification will map a source topic at the path
* a/x/y/z
to a reference topic at the path b/x/y/z
.
* The specification is simple, so the reference topic will exactly mirror the
* source topic. Other topic views features allow a single topic to be mapped to
* many reference topics and have the data transformed in the process.
*
*
Topic view persistence and replication
*
* Reference topics are neither replicated nor persisted. They are created and
* removed based on their source topics. However, topic views are replicated and
* persisted. A server that restarts will restore topic views during recovery.
* Each topic view will then create reference topics based on the source topics
* that have been recovered.
*
*
* The server records all changes to topic views in a persistent store. Topic
* views are restored if the server is started.
*
*
* If a server belongs to a cluster, topic views (and remote servers) will be
* replicated to each server in the cluster. Topic views are evaluated locally
* within a server. Replicated topic views that select non-replicated source
* topics can create different reference topics on each server in the cluster.
* When remote topic views are in use, each server in the cluster will make a
* connection to the specified remote server and will separately manage their
* remote topic views.
*
*
* A view with a delay clause uses temporary storage to record delayed events.
* If there is a high volume of updates, temporary per-server disk files will be
* used to save server memory. The storage is per-server, and does not survive
* server restart. When a server is started, no data will be published by a view
* with a delay clause until the delay time has expired.
*
*
Access control
*
* The following access control restrictions are applied:
*
*
* - To {@link #listTopicViews() list the topic views}, a session needs the
* {@link GlobalPermission#READ_TOPIC_VIEWS READ_TOPIC_VIEWS} global permission.
*
*
- To {@link #createTopicView(String, String) create, replace}, or
* {@link #removeTopicView(String) remove} a topic view, a session needs the
* {@link GlobalPermission#MODIFY_TOPIC_VIEWS MODIFY_TOPIC_VIEWS} global
* permission and {@link PathPermission#SELECT_TOPIC SELECT_TOPIC} permission
* for the path prefix of the source topic selector.
*
*
- Each topic view records the principal and security roles of the session
* that created it as the topic view security context. When a topic
* view is evaluated, this security context is used to constrain the creation of
* reference topics. A reference topic will only be created if the security
* context has {@link PathPermission#READ_TOPIC READ_TOPIC} permission for the
* source topic path, and {@link PathPermission#MODIFY_TOPIC MODIFY_TOPIC}
* permission for the reference topic path. The topic view security context is
* copied from the creating session at the time the topic view is created or
* replaced, and is persisted with the topic view. The topic view security
* context is not updated if the roles associated with the session are changed.
*
*
*
* Accessing the feature
*
* This feature may be obtained from a {@link Session session} as follows:
*
*
* TopicViews topicViews = session.feature(TopicViews.class);
*
*
*
* This feature is also extended by the {@link Topics Topics} feature. This
* means is it possible to use the methods described here through the
* {@link Topics Topics} feature.
*
* @author DiffusionData Limited
* @since 6.3
*/
public interface TopicViews extends Feature {
/**
* Create a new named topic view.
*
* If a view with the same name already exists the new view will update the
* existing view.
*
* @param name the name of the view. If the name is empty, the operation
* will throw an InvalidArgumentException.
* @param specification the
* specification of the topic view
* @return a CompletableFuture that completes when a response is received
* from the server, returning the topic view created by the
* operation.
*
*
* If the task fails, the CompletableFuture will complete
* exceptionally with a {@link CompletionException}. Common reasons
* for failure, listed by the exception reported as the
* {@link CompletionException#getCause() cause}, include:
*
*
* - {@link ScriptException} – if {@code specification} is
* invalid;
*
- {@link ClusterRoutingException} – if the operation
* failed due to a transient cluster error;
*
- {@link PermissionsException} – if the calling session
* does not have MODIFY_TOPIC_VIEW permission or appropriate path
* prefix permissions;
*
- {@link SessionClosedException} – if the session is
* closed.
*
*/
CompletableFuture createTopicView(String name,
String specification);
/**
* List all the topic views that have been created.
*
* @return a CompletableFuture that completes when a response is received
* from the server, returning a list of views sorted by their
* creation order.
*
*
* If the task fails, the CompletableFuture will complete
* exceptionally with a {@link CompletionException}. Common reasons
* for failure, listed by the exception reported as the
* {@link CompletionException#getCause() cause}, include:
*
*
* - {@link ClusterRoutingException} – if the operation
* failed due to a transient cluster error;
*
- {@link PermissionsException} – if the calling session
* does not have READ_TOPIC_VIEW permission or appropriate path
* prefix permissions;
*
- {@link SessionClosedException} – if the session is
* closed.
*
*/
CompletableFuture> listTopicViews();
/**
* Get a named Topic View.
*
* If the named view does not exist the completable future will complete
* successfully with a null result.
*
* @param name the name of the view
* @return a CompletableFuture that completes when a response is received
* from the server, returning a named view if it exists
*
*
* If the task fails, the CompletableFuture will complete
* exceptionally with a {@link CompletionException}. Common reasons
* for failure, listed by the exception reported as the
* {@link CompletionException#getCause() cause}, include:
*
*
* - {@link ClusterRoutingException} – if the operation
* failed due to a transient cluster error;
*
- {@link PermissionsException} – if the calling session
* does not have READ_TOPIC_VIEW permission or appropriate path
* prefix permissions;
*
- {@link SessionClosedException} – if the session is
* closed.
*
*/
CompletableFuture getTopicView(String name);
/**
* Remove a named topic view if it exists.
*
* If the named view does not exist the completable future will complete
* successfully.
*
* @param name the name of the view
* @return a CompletableFuture that completes when a response is received
* from the server.
*
*
* If the task fails, the CompletableFuture will complete
* exceptionally with a {@link CompletionException}. Common reasons
* for failure, listed by the exception reported as the
* {@link CompletionException#getCause() cause}, include:
*
*
* - {@link ClusterRoutingException} – if the operation
* failed due to a transient cluster error;
*
- {@link PermissionsException} – if the calling session
* does not have MODIFY_TOPIC_VIEW permission or appropriate path
* prefix permissions;
*
- {@link SessionClosedException} – if the session is
* closed.
*
*/
CompletableFuture> removeTopicView(String name);
}