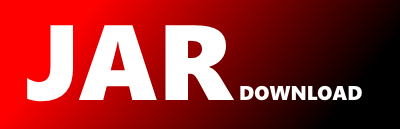
com.digitalpetri.strictmachine.dsl.TransitionBuilder Maven / Gradle / Ivy
/*
* Copyright 2018 Kevin Herron
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.digitalpetri.strictmachine.dsl;
import java.util.List;
import java.util.function.Predicate;
public class TransitionBuilder, E> {
private final S from;
private final List> transitions;
private final List> transitionActions;
TransitionBuilder(
S from,
List> transitions,
List> transitionActions) {
this.from = from;
this.transitions = transitions;
this.transitionActions = transitionActions;
}
/**
* Continue defining a {@link Transition} that is triggered by {@code event}.
*
* @param event the event that triggers this transition.
* @return a {@link TransitionTo}.
*/
public TransitionTo on(E event) {
return to -> {
PredicatedTransition transition =
Transitions.fromInstanceViaInstance(from, event, to);
transitions.add(transition);
return new GuardBuilder<>(transition, transitionActions);
};
}
/**
* Continue defining a {@link Transition} that is triggered by an event of type {@code eventClass}.
*
* @param eventClass the †ype of event that triggers this transition.
* @return a {@link TransitionTo}.
*/
public TransitionTo on(Class extends E> eventClass) {
return to -> {
PredicatedTransition transition =
Transitions.fromInstanceViaClass(from, eventClass, to);
transitions.add(transition);
return new GuardBuilder<>(transition, transitionActions);
};
}
/**
* Continue defining a {@link Transition} that is triggered by any event that passes {@code eventFilter}.
*
* @param eventFilter the filter for events that trigger this transition.
* @return a {@link TransitionTo}.
*/
public TransitionTo on(Predicate eventFilter) {
return to -> {
PredicatedTransition transition =
Transitions.fromInstanceViaDynamic(from, eventFilter, to);
transitions.add(transition);
return new GuardBuilder<>(transition, transitionActions);
};
}
/**
* Continue defining a {@link Transition} that is triggered by any event.
*
* @return a {@link TransitionTo}.
*/
public TransitionTo onAny() {
return on(e -> true);
}
public interface TransitionTo {
GuardBuilder transitionTo(S state);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy