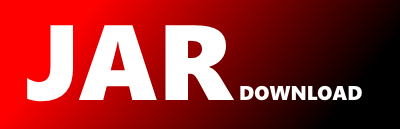
com.dimajix.flowman.kernel.proto.history.TargetHistoryQueryOrBuilder Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: history.proto
package com.dimajix.flowman.kernel.proto.history;
public interface TargetHistoryQueryOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.dimajix.flowman.kernel.history.TargetHistoryQuery)
com.google.protobuf.MessageOrBuilder {
/**
* repeated string id = 1;
* @return A list containing the id.
*/
java.util.List
getIdList();
/**
* repeated string id = 1;
* @return The count of id.
*/
int getIdCount();
/**
* repeated string id = 1;
* @param index The index of the element to return.
* @return The id at the given index.
*/
java.lang.String getId(int index);
/**
* repeated string id = 1;
* @param index The index of the value to return.
* @return The bytes of the id at the given index.
*/
com.dimajix.shaded.protobuf.ByteString
getIdBytes(int index);
/**
* repeated string namespace = 2;
* @return A list containing the namespace.
*/
java.util.List
getNamespaceList();
/**
* repeated string namespace = 2;
* @return The count of namespace.
*/
int getNamespaceCount();
/**
* repeated string namespace = 2;
* @param index The index of the element to return.
* @return The namespace at the given index.
*/
java.lang.String getNamespace(int index);
/**
* repeated string namespace = 2;
* @param index The index of the value to return.
* @return The bytes of the namespace at the given index.
*/
com.dimajix.shaded.protobuf.ByteString
getNamespaceBytes(int index);
/**
* repeated string project = 3;
* @return A list containing the project.
*/
java.util.List
getProjectList();
/**
* repeated string project = 3;
* @return The count of project.
*/
int getProjectCount();
/**
* repeated string project = 3;
* @param index The index of the element to return.
* @return The project at the given index.
*/
java.lang.String getProject(int index);
/**
* repeated string project = 3;
* @param index The index of the value to return.
* @return The bytes of the project at the given index.
*/
com.dimajix.shaded.protobuf.ByteString
getProjectBytes(int index);
/**
* repeated string job = 4;
* @return A list containing the job.
*/
java.util.List
getJobList();
/**
* repeated string job = 4;
* @return The count of job.
*/
int getJobCount();
/**
* repeated string job = 4;
* @param index The index of the element to return.
* @return The job at the given index.
*/
java.lang.String getJob(int index);
/**
* repeated string job = 4;
* @param index The index of the value to return.
* @return The bytes of the job at the given index.
*/
com.dimajix.shaded.protobuf.ByteString
getJobBytes(int index);
/**
* repeated string jobId = 5;
* @return A list containing the jobId.
*/
java.util.List
getJobIdList();
/**
* repeated string jobId = 5;
* @return The count of jobId.
*/
int getJobIdCount();
/**
* repeated string jobId = 5;
* @param index The index of the element to return.
* @return The jobId at the given index.
*/
java.lang.String getJobId(int index);
/**
* repeated string jobId = 5;
* @param index The index of the value to return.
* @return The bytes of the jobId at the given index.
*/
com.dimajix.shaded.protobuf.ByteString
getJobIdBytes(int index);
/**
* repeated string target = 6;
* @return A list containing the target.
*/
java.util.List
getTargetList();
/**
* repeated string target = 6;
* @return The count of target.
*/
int getTargetCount();
/**
* repeated string target = 6;
* @param index The index of the element to return.
* @return The target at the given index.
*/
java.lang.String getTarget(int index);
/**
* repeated string target = 6;
* @param index The index of the value to return.
* @return The bytes of the target at the given index.
*/
com.dimajix.shaded.protobuf.ByteString
getTargetBytes(int index);
/**
* repeated .com.dimajix.flowman.kernel.ExecutionStatus status = 7;
* @return A list containing the status.
*/
java.util.List getStatusList();
/**
* repeated .com.dimajix.flowman.kernel.ExecutionStatus status = 7;
* @return The count of status.
*/
int getStatusCount();
/**
* repeated .com.dimajix.flowman.kernel.ExecutionStatus status = 7;
* @param index The index of the element to return.
* @return The status at the given index.
*/
com.dimajix.flowman.kernel.proto.ExecutionStatus getStatus(int index);
/**
* repeated .com.dimajix.flowman.kernel.ExecutionStatus status = 7;
* @return A list containing the enum numeric values on the wire for status.
*/
java.util.List
getStatusValueList();
/**
* repeated .com.dimajix.flowman.kernel.ExecutionStatus status = 7;
* @param index The index of the value to return.
* @return The enum numeric value on the wire of status at the given index.
*/
int getStatusValue(int index);
/**
* repeated .com.dimajix.flowman.kernel.ExecutionPhase phase = 8;
* @return A list containing the phase.
*/
java.util.List getPhaseList();
/**
* repeated .com.dimajix.flowman.kernel.ExecutionPhase phase = 8;
* @return The count of phase.
*/
int getPhaseCount();
/**
* repeated .com.dimajix.flowman.kernel.ExecutionPhase phase = 8;
* @param index The index of the element to return.
* @return The phase at the given index.
*/
com.dimajix.flowman.kernel.proto.ExecutionPhase getPhase(int index);
/**
* repeated .com.dimajix.flowman.kernel.ExecutionPhase phase = 8;
* @return A list containing the enum numeric values on the wire for phase.
*/
java.util.List
getPhaseValueList();
/**
* repeated .com.dimajix.flowman.kernel.ExecutionPhase phase = 8;
* @param index The index of the value to return.
* @return The enum numeric value on the wire of phase at the given index.
*/
int getPhaseValue(int index);
/**
* map<string, string> partitions = 9;
*/
int getPartitionsCount();
/**
* map<string, string> partitions = 9;
*/
boolean containsPartitions(
java.lang.String key);
/**
* Use {@link #getPartitionsMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getPartitions();
/**
* map<string, string> partitions = 9;
*/
java.util.Map
getPartitionsMap();
/**
* map<string, string> partitions = 9;
*/
/* nullable */
java.lang.String getPartitionsOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue);
/**
* map<string, string> partitions = 9;
*/
java.lang.String getPartitionsOrThrow(
java.lang.String key);
/**
* optional .com.dimajix.flowman.kernel.Timestamp from = 10;
* @return Whether the from field is set.
*/
boolean hasFrom();
/**
* optional .com.dimajix.flowman.kernel.Timestamp from = 10;
* @return The from.
*/
com.dimajix.flowman.kernel.proto.Timestamp getFrom();
/**
* optional .com.dimajix.flowman.kernel.Timestamp from = 10;
*/
com.dimajix.flowman.kernel.proto.TimestampOrBuilder getFromOrBuilder();
/**
* optional .com.dimajix.flowman.kernel.Timestamp until = 11;
* @return Whether the until field is set.
*/
boolean hasUntil();
/**
* optional .com.dimajix.flowman.kernel.Timestamp until = 11;
* @return The until.
*/
com.dimajix.flowman.kernel.proto.Timestamp getUntil();
/**
* optional .com.dimajix.flowman.kernel.Timestamp until = 11;
*/
com.dimajix.flowman.kernel.proto.TimestampOrBuilder getUntilOrBuilder();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy