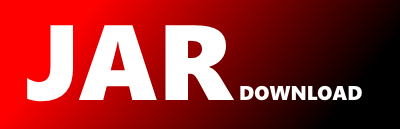
com.dimajix.flowman.kernel.proto.project.ProjectDetailsOrBuilder Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: project.proto
package com.dimajix.flowman.kernel.proto.project;
public interface ProjectDetailsOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.dimajix.flowman.kernel.project.ProjectDetails)
com.google.protobuf.MessageOrBuilder {
/**
* string name = 2;
* @return The name.
*/
java.lang.String getName();
/**
* string name = 2;
* @return The bytes for name.
*/
com.dimajix.shaded.protobuf.ByteString
getNameBytes();
/**
* optional string version = 3;
* @return Whether the version field is set.
*/
boolean hasVersion();
/**
* optional string version = 3;
* @return The version.
*/
java.lang.String getVersion();
/**
* optional string version = 3;
* @return The bytes for version.
*/
com.dimajix.shaded.protobuf.ByteString
getVersionBytes();
/**
* optional string basedir = 4;
* @return Whether the basedir field is set.
*/
boolean hasBasedir();
/**
* optional string basedir = 4;
* @return The basedir.
*/
java.lang.String getBasedir();
/**
* optional string basedir = 4;
* @return The bytes for basedir.
*/
com.dimajix.shaded.protobuf.ByteString
getBasedirBytes();
/**
* optional string filename = 5;
* @return Whether the filename field is set.
*/
boolean hasFilename();
/**
* optional string filename = 5;
* @return The filename.
*/
java.lang.String getFilename();
/**
* optional string filename = 5;
* @return The bytes for filename.
*/
com.dimajix.shaded.protobuf.ByteString
getFilenameBytes();
/**
* optional string description = 6;
* @return Whether the description field is set.
*/
boolean hasDescription();
/**
* optional string description = 6;
* @return The description.
*/
java.lang.String getDescription();
/**
* optional string description = 6;
* @return The bytes for description.
*/
com.dimajix.shaded.protobuf.ByteString
getDescriptionBytes();
/**
* repeated string targets = 7;
* @return A list containing the targets.
*/
java.util.List
getTargetsList();
/**
* repeated string targets = 7;
* @return The count of targets.
*/
int getTargetsCount();
/**
* repeated string targets = 7;
* @param index The index of the element to return.
* @return The targets at the given index.
*/
java.lang.String getTargets(int index);
/**
* repeated string targets = 7;
* @param index The index of the value to return.
* @return The bytes of the targets at the given index.
*/
com.dimajix.shaded.protobuf.ByteString
getTargetsBytes(int index);
/**
* repeated string tests = 8;
* @return A list containing the tests.
*/
java.util.List
getTestsList();
/**
* repeated string tests = 8;
* @return The count of tests.
*/
int getTestsCount();
/**
* repeated string tests = 8;
* @param index The index of the element to return.
* @return The tests at the given index.
*/
java.lang.String getTests(int index);
/**
* repeated string tests = 8;
* @param index The index of the value to return.
* @return The bytes of the tests at the given index.
*/
com.dimajix.shaded.protobuf.ByteString
getTestsBytes(int index);
/**
* repeated string jobs = 9;
* @return A list containing the jobs.
*/
java.util.List
getJobsList();
/**
* repeated string jobs = 9;
* @return The count of jobs.
*/
int getJobsCount();
/**
* repeated string jobs = 9;
* @param index The index of the element to return.
* @return The jobs at the given index.
*/
java.lang.String getJobs(int index);
/**
* repeated string jobs = 9;
* @param index The index of the value to return.
* @return The bytes of the jobs at the given index.
*/
com.dimajix.shaded.protobuf.ByteString
getJobsBytes(int index);
/**
* repeated string mappings = 10;
* @return A list containing the mappings.
*/
java.util.List
getMappingsList();
/**
* repeated string mappings = 10;
* @return The count of mappings.
*/
int getMappingsCount();
/**
* repeated string mappings = 10;
* @param index The index of the element to return.
* @return The mappings at the given index.
*/
java.lang.String getMappings(int index);
/**
* repeated string mappings = 10;
* @param index The index of the value to return.
* @return The bytes of the mappings at the given index.
*/
com.dimajix.shaded.protobuf.ByteString
getMappingsBytes(int index);
/**
* repeated string relations = 11;
* @return A list containing the relations.
*/
java.util.List
getRelationsList();
/**
* repeated string relations = 11;
* @return The count of relations.
*/
int getRelationsCount();
/**
* repeated string relations = 11;
* @param index The index of the element to return.
* @return The relations at the given index.
*/
java.lang.String getRelations(int index);
/**
* repeated string relations = 11;
* @param index The index of the value to return.
* @return The bytes of the relations at the given index.
*/
com.dimajix.shaded.protobuf.ByteString
getRelationsBytes(int index);
/**
* repeated string connections = 12;
* @return A list containing the connections.
*/
java.util.List
getConnectionsList();
/**
* repeated string connections = 12;
* @return The count of connections.
*/
int getConnectionsCount();
/**
* repeated string connections = 12;
* @param index The index of the element to return.
* @return The connections at the given index.
*/
java.lang.String getConnections(int index);
/**
* repeated string connections = 12;
* @param index The index of the value to return.
* @return The bytes of the connections at the given index.
*/
com.dimajix.shaded.protobuf.ByteString
getConnectionsBytes(int index);
/**
* map<string, string> environment = 14;
*/
int getEnvironmentCount();
/**
* map<string, string> environment = 14;
*/
boolean containsEnvironment(
java.lang.String key);
/**
* Use {@link #getEnvironmentMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getEnvironment();
/**
* map<string, string> environment = 14;
*/
java.util.Map
getEnvironmentMap();
/**
* map<string, string> environment = 14;
*/
/* nullable */
java.lang.String getEnvironmentOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue);
/**
* map<string, string> environment = 14;
*/
java.lang.String getEnvironmentOrThrow(
java.lang.String key);
/**
* map<string, string> config = 15;
*/
int getConfigCount();
/**
* map<string, string> config = 15;
*/
boolean containsConfig(
java.lang.String key);
/**
* Use {@link #getConfigMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getConfig();
/**
* map<string, string> config = 15;
*/
java.util.Map
getConfigMap();
/**
* map<string, string> config = 15;
*/
/* nullable */
java.lang.String getConfigOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue);
/**
* map<string, string> config = 15;
*/
java.lang.String getConfigOrThrow(
java.lang.String key);
/**
* repeated string profiles = 16;
* @return A list containing the profiles.
*/
java.util.List
getProfilesList();
/**
* repeated string profiles = 16;
* @return The count of profiles.
*/
int getProfilesCount();
/**
* repeated string profiles = 16;
* @param index The index of the element to return.
* @return The profiles at the given index.
*/
java.lang.String getProfiles(int index);
/**
* repeated string profiles = 16;
* @param index The index of the value to return.
* @return The bytes of the profiles at the given index.
*/
com.dimajix.shaded.protobuf.ByteString
getProfilesBytes(int index);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy