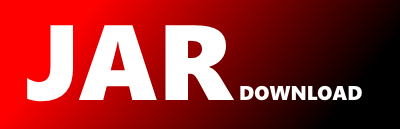
com.dimajix.flowman.kernel.proto.session.SessionDetailsOrBuilder Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: session.proto
package com.dimajix.flowman.kernel.proto.session;
public interface SessionDetailsOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.dimajix.flowman.kernel.session.SessionDetails)
com.google.protobuf.MessageOrBuilder {
/**
* string id = 1;
* @return The id.
*/
java.lang.String getId();
/**
* string id = 1;
* @return The bytes for id.
*/
com.dimajix.shaded.protobuf.ByteString
getIdBytes();
/**
* optional string name = 2;
* @return Whether the name field is set.
*/
boolean hasName();
/**
* optional string name = 2;
* @return The name.
*/
java.lang.String getName();
/**
* optional string name = 2;
* @return The bytes for name.
*/
com.dimajix.shaded.protobuf.ByteString
getNameBytes();
/**
* optional string workspace = 3;
* @return Whether the workspace field is set.
*/
boolean hasWorkspace();
/**
* optional string workspace = 3;
* @return The workspace.
*/
java.lang.String getWorkspace();
/**
* optional string workspace = 3;
* @return The bytes for workspace.
*/
com.dimajix.shaded.protobuf.ByteString
getWorkspaceBytes();
/**
* optional string namespace = 4;
* @return Whether the namespace field is set.
*/
boolean hasNamespace();
/**
* optional string namespace = 4;
* @return The namespace.
*/
java.lang.String getNamespace();
/**
* optional string namespace = 4;
* @return The bytes for namespace.
*/
com.dimajix.shaded.protobuf.ByteString
getNamespaceBytes();
/**
* optional string project = 5;
* @return Whether the project field is set.
*/
boolean hasProject();
/**
* optional string project = 5;
* @return The project.
*/
java.lang.String getProject();
/**
* optional string project = 5;
* @return The bytes for project.
*/
com.dimajix.shaded.protobuf.ByteString
getProjectBytes();
/**
* optional string projectVersion = 6;
* @return Whether the projectVersion field is set.
*/
boolean hasProjectVersion();
/**
* optional string projectVersion = 6;
* @return The projectVersion.
*/
java.lang.String getProjectVersion();
/**
* optional string projectVersion = 6;
* @return The bytes for projectVersion.
*/
com.dimajix.shaded.protobuf.ByteString
getProjectVersionBytes();
/**
* optional string projectDescription = 7;
* @return Whether the projectDescription field is set.
*/
boolean hasProjectDescription();
/**
* optional string projectDescription = 7;
* @return The projectDescription.
*/
java.lang.String getProjectDescription();
/**
* optional string projectDescription = 7;
* @return The bytes for projectDescription.
*/
com.dimajix.shaded.protobuf.ByteString
getProjectDescriptionBytes();
/**
* optional string projectBasedir = 8;
* @return Whether the projectBasedir field is set.
*/
boolean hasProjectBasedir();
/**
* optional string projectBasedir = 8;
* @return The projectBasedir.
*/
java.lang.String getProjectBasedir();
/**
* optional string projectBasedir = 8;
* @return The bytes for projectBasedir.
*/
com.dimajix.shaded.protobuf.ByteString
getProjectBasedirBytes();
/**
* optional string projectFilename = 9;
* @return Whether the projectFilename field is set.
*/
boolean hasProjectFilename();
/**
* optional string projectFilename = 9;
* @return The projectFilename.
*/
java.lang.String getProjectFilename();
/**
* optional string projectFilename = 9;
* @return The bytes for projectFilename.
*/
com.dimajix.shaded.protobuf.ByteString
getProjectFilenameBytes();
/**
* repeated string profiles = 10;
* @return A list containing the profiles.
*/
java.util.List
getProfilesList();
/**
* repeated string profiles = 10;
* @return The count of profiles.
*/
int getProfilesCount();
/**
* repeated string profiles = 10;
* @param index The index of the element to return.
* @return The profiles at the given index.
*/
java.lang.String getProfiles(int index);
/**
* repeated string profiles = 10;
* @param index The index of the value to return.
* @return The bytes of the profiles at the given index.
*/
com.dimajix.shaded.protobuf.ByteString
getProfilesBytes(int index);
/**
* map<string, string> environment = 11;
*/
int getEnvironmentCount();
/**
* map<string, string> environment = 11;
*/
boolean containsEnvironment(
java.lang.String key);
/**
* Use {@link #getEnvironmentMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getEnvironment();
/**
* map<string, string> environment = 11;
*/
java.util.Map
getEnvironmentMap();
/**
* map<string, string> environment = 11;
*/
/* nullable */
java.lang.String getEnvironmentOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue);
/**
* map<string, string> environment = 11;
*/
java.lang.String getEnvironmentOrThrow(
java.lang.String key);
/**
* map<string, string> config = 12;
*/
int getConfigCount();
/**
* map<string, string> config = 12;
*/
boolean containsConfig(
java.lang.String key);
/**
* Use {@link #getConfigMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getConfig();
/**
* map<string, string> config = 12;
*/
java.util.Map
getConfigMap();
/**
* map<string, string> config = 12;
*/
/* nullable */
java.lang.String getConfigOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue);
/**
* map<string, string> config = 12;
*/
java.lang.String getConfigOrThrow(
java.lang.String key);
/**
* map<string, string> flowmanConfig = 13;
*/
int getFlowmanConfigCount();
/**
* map<string, string> flowmanConfig = 13;
*/
boolean containsFlowmanConfig(
java.lang.String key);
/**
* Use {@link #getFlowmanConfigMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getFlowmanConfig();
/**
* map<string, string> flowmanConfig = 13;
*/
java.util.Map
getFlowmanConfigMap();
/**
* map<string, string> flowmanConfig = 13;
*/
/* nullable */
java.lang.String getFlowmanConfigOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue);
/**
* map<string, string> flowmanConfig = 13;
*/
java.lang.String getFlowmanConfigOrThrow(
java.lang.String key);
/**
* map<string, string> hadoopConfig = 14;
*/
int getHadoopConfigCount();
/**
* map<string, string> hadoopConfig = 14;
*/
boolean containsHadoopConfig(
java.lang.String key);
/**
* Use {@link #getHadoopConfigMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getHadoopConfig();
/**
* map<string, string> hadoopConfig = 14;
*/
java.util.Map
getHadoopConfigMap();
/**
* map<string, string> hadoopConfig = 14;
*/
/* nullable */
java.lang.String getHadoopConfigOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue);
/**
* map<string, string> hadoopConfig = 14;
*/
java.lang.String getHadoopConfigOrThrow(
java.lang.String key);
/**
* map<string, string> sparkConfig = 15;
*/
int getSparkConfigCount();
/**
* map<string, string> sparkConfig = 15;
*/
boolean containsSparkConfig(
java.lang.String key);
/**
* Use {@link #getSparkConfigMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getSparkConfig();
/**
* map<string, string> sparkConfig = 15;
*/
java.util.Map
getSparkConfigMap();
/**
* map<string, string> sparkConfig = 15;
*/
/* nullable */
java.lang.String getSparkConfigOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue);
/**
* map<string, string> sparkConfig = 15;
*/
java.lang.String getSparkConfigOrThrow(
java.lang.String key);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy