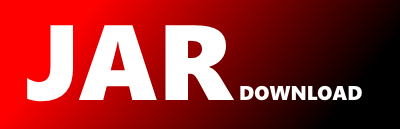
com.dimajix.flowman.kernel.proto.session.SessionServiceGrpc Maven / Gradle / Ivy
The newest version!
package com.dimajix.flowman.kernel.proto.session;
import static com.dimajix.shaded.grpc.MethodDescriptor.generateFullMethodName;
/**
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.56.0)",
comments = "Source: session.proto")
@com.dimajix.shaded.grpc.stub.annotations.GrpcGenerated
public final class SessionServiceGrpc {
private SessionServiceGrpc() {}
public static final String SERVICE_NAME = "com.dimajix.flowman.kernel.session.SessionService";
// Static method descriptors that strictly reflect the proto.
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getCreateSessionMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "CreateSession",
requestType = com.dimajix.flowman.kernel.proto.session.CreateSessionRequest.class,
responseType = com.dimajix.flowman.kernel.proto.session.CreateSessionResponse.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
public static com.dimajix.shaded.grpc.MethodDescriptor getCreateSessionMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getCreateSessionMethod;
if ((getCreateSessionMethod = SessionServiceGrpc.getCreateSessionMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getCreateSessionMethod = SessionServiceGrpc.getCreateSessionMethod) == null) {
SessionServiceGrpc.getCreateSessionMethod = getCreateSessionMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "CreateSession"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.session.CreateSessionRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.session.CreateSessionResponse.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("CreateSession"))
.build();
}
}
}
return getCreateSessionMethod;
}
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getListSessionsMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ListSessions",
requestType = com.dimajix.flowman.kernel.proto.session.ListSessionsRequest.class,
responseType = com.dimajix.flowman.kernel.proto.session.ListSessionsResponse.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
public static com.dimajix.shaded.grpc.MethodDescriptor getListSessionsMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getListSessionsMethod;
if ((getListSessionsMethod = SessionServiceGrpc.getListSessionsMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getListSessionsMethod = SessionServiceGrpc.getListSessionsMethod) == null) {
SessionServiceGrpc.getListSessionsMethod = getListSessionsMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ListSessions"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.session.ListSessionsRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.session.ListSessionsResponse.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("ListSessions"))
.build();
}
}
}
return getListSessionsMethod;
}
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getGetSessionMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetSession",
requestType = com.dimajix.flowman.kernel.proto.session.GetSessionRequest.class,
responseType = com.dimajix.flowman.kernel.proto.session.GetSessionResponse.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
public static com.dimajix.shaded.grpc.MethodDescriptor getGetSessionMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getGetSessionMethod;
if ((getGetSessionMethod = SessionServiceGrpc.getGetSessionMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getGetSessionMethod = SessionServiceGrpc.getGetSessionMethod) == null) {
SessionServiceGrpc.getGetSessionMethod = getGetSessionMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetSession"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.session.GetSessionRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.session.GetSessionResponse.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("GetSession"))
.build();
}
}
}
return getGetSessionMethod;
}
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getDeleteSessionMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "DeleteSession",
requestType = com.dimajix.flowman.kernel.proto.session.DeleteSessionRequest.class,
responseType = com.dimajix.flowman.kernel.proto.session.DeleteSessionResponse.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
public static com.dimajix.shaded.grpc.MethodDescriptor getDeleteSessionMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getDeleteSessionMethod;
if ((getDeleteSessionMethod = SessionServiceGrpc.getDeleteSessionMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getDeleteSessionMethod = SessionServiceGrpc.getDeleteSessionMethod) == null) {
SessionServiceGrpc.getDeleteSessionMethod = getDeleteSessionMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "DeleteSession"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.session.DeleteSessionRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.session.DeleteSessionResponse.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("DeleteSession"))
.build();
}
}
}
return getDeleteSessionMethod;
}
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getEnterContextMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "EnterContext",
requestType = com.dimajix.flowman.kernel.proto.session.EnterContextRequest.class,
responseType = com.dimajix.flowman.kernel.proto.session.EnterContextResponse.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
public static com.dimajix.shaded.grpc.MethodDescriptor getEnterContextMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getEnterContextMethod;
if ((getEnterContextMethod = SessionServiceGrpc.getEnterContextMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getEnterContextMethod = SessionServiceGrpc.getEnterContextMethod) == null) {
SessionServiceGrpc.getEnterContextMethod = getEnterContextMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "EnterContext"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.session.EnterContextRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.session.EnterContextResponse.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("EnterContext"))
.build();
}
}
}
return getEnterContextMethod;
}
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getLeaveContextMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "LeaveContext",
requestType = com.dimajix.flowman.kernel.proto.session.LeaveContextRequest.class,
responseType = com.dimajix.flowman.kernel.proto.session.LeaveContextResponse.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
public static com.dimajix.shaded.grpc.MethodDescriptor getLeaveContextMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getLeaveContextMethod;
if ((getLeaveContextMethod = SessionServiceGrpc.getLeaveContextMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getLeaveContextMethod = SessionServiceGrpc.getLeaveContextMethod) == null) {
SessionServiceGrpc.getLeaveContextMethod = getLeaveContextMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "LeaveContext"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.session.LeaveContextRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.session.LeaveContextResponse.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("LeaveContext"))
.build();
}
}
}
return getLeaveContextMethod;
}
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getGetContextMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetContext",
requestType = com.dimajix.flowman.kernel.proto.session.GetContextRequest.class,
responseType = com.dimajix.flowman.kernel.proto.session.GetContextResponse.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
public static com.dimajix.shaded.grpc.MethodDescriptor getGetContextMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getGetContextMethod;
if ((getGetContextMethod = SessionServiceGrpc.getGetContextMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getGetContextMethod = SessionServiceGrpc.getGetContextMethod) == null) {
SessionServiceGrpc.getGetContextMethod = getGetContextMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetContext"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.session.GetContextRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.session.GetContextResponse.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("GetContext"))
.build();
}
}
}
return getGetContextMethod;
}
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getListMappingsMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ListMappings",
requestType = com.dimajix.flowman.kernel.proto.mapping.ListMappingsRequest.class,
responseType = com.dimajix.flowman.kernel.proto.mapping.ListMappingsResponse.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
public static com.dimajix.shaded.grpc.MethodDescriptor getListMappingsMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getListMappingsMethod;
if ((getListMappingsMethod = SessionServiceGrpc.getListMappingsMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getListMappingsMethod = SessionServiceGrpc.getListMappingsMethod) == null) {
SessionServiceGrpc.getListMappingsMethod = getListMappingsMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ListMappings"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.mapping.ListMappingsRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.mapping.ListMappingsResponse.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("ListMappings"))
.build();
}
}
}
return getListMappingsMethod;
}
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getGetMappingMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetMapping",
requestType = com.dimajix.flowman.kernel.proto.mapping.GetMappingRequest.class,
responseType = com.dimajix.flowman.kernel.proto.mapping.GetMappingResponse.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
public static com.dimajix.shaded.grpc.MethodDescriptor getGetMappingMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getGetMappingMethod;
if ((getGetMappingMethod = SessionServiceGrpc.getGetMappingMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getGetMappingMethod = SessionServiceGrpc.getGetMappingMethod) == null) {
SessionServiceGrpc.getGetMappingMethod = getGetMappingMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetMapping"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.mapping.GetMappingRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.mapping.GetMappingResponse.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("GetMapping"))
.build();
}
}
}
return getGetMappingMethod;
}
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getReadMappingMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ReadMapping",
requestType = com.dimajix.flowman.kernel.proto.mapping.ReadMappingRequest.class,
responseType = com.dimajix.flowman.kernel.proto.mapping.ReadMappingResponse.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
public static com.dimajix.shaded.grpc.MethodDescriptor getReadMappingMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getReadMappingMethod;
if ((getReadMappingMethod = SessionServiceGrpc.getReadMappingMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getReadMappingMethod = SessionServiceGrpc.getReadMappingMethod) == null) {
SessionServiceGrpc.getReadMappingMethod = getReadMappingMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ReadMapping"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.mapping.ReadMappingRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.mapping.ReadMappingResponse.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("ReadMapping"))
.build();
}
}
}
return getReadMappingMethod;
}
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getDescribeMappingMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "DescribeMapping",
requestType = com.dimajix.flowman.kernel.proto.mapping.DescribeMappingRequest.class,
responseType = com.dimajix.flowman.kernel.proto.mapping.DescribeMappingResponse.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
public static com.dimajix.shaded.grpc.MethodDescriptor getDescribeMappingMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getDescribeMappingMethod;
if ((getDescribeMappingMethod = SessionServiceGrpc.getDescribeMappingMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getDescribeMappingMethod = SessionServiceGrpc.getDescribeMappingMethod) == null) {
SessionServiceGrpc.getDescribeMappingMethod = getDescribeMappingMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "DescribeMapping"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.mapping.DescribeMappingRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.mapping.DescribeMappingResponse.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("DescribeMapping"))
.build();
}
}
}
return getDescribeMappingMethod;
}
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getValidateMappingMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ValidateMapping",
requestType = com.dimajix.flowman.kernel.proto.mapping.ValidateMappingRequest.class,
responseType = com.dimajix.flowman.kernel.proto.mapping.ValidateMappingResponse.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
public static com.dimajix.shaded.grpc.MethodDescriptor getValidateMappingMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getValidateMappingMethod;
if ((getValidateMappingMethod = SessionServiceGrpc.getValidateMappingMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getValidateMappingMethod = SessionServiceGrpc.getValidateMappingMethod) == null) {
SessionServiceGrpc.getValidateMappingMethod = getValidateMappingMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ValidateMapping"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.mapping.ValidateMappingRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.mapping.ValidateMappingResponse.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("ValidateMapping"))
.build();
}
}
}
return getValidateMappingMethod;
}
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getSaveMappingOutputMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "SaveMappingOutput",
requestType = com.dimajix.flowman.kernel.proto.mapping.SaveMappingOutputRequest.class,
responseType = com.dimajix.flowman.kernel.proto.mapping.SaveMappingOutputResponse.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
public static com.dimajix.shaded.grpc.MethodDescriptor getSaveMappingOutputMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getSaveMappingOutputMethod;
if ((getSaveMappingOutputMethod = SessionServiceGrpc.getSaveMappingOutputMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getSaveMappingOutputMethod = SessionServiceGrpc.getSaveMappingOutputMethod) == null) {
SessionServiceGrpc.getSaveMappingOutputMethod = getSaveMappingOutputMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "SaveMappingOutput"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.mapping.SaveMappingOutputRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.mapping.SaveMappingOutputResponse.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("SaveMappingOutput"))
.build();
}
}
}
return getSaveMappingOutputMethod;
}
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getCountMappingMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "CountMapping",
requestType = com.dimajix.flowman.kernel.proto.mapping.CountMappingRequest.class,
responseType = com.dimajix.flowman.kernel.proto.mapping.CountMappingResponse.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
public static com.dimajix.shaded.grpc.MethodDescriptor getCountMappingMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getCountMappingMethod;
if ((getCountMappingMethod = SessionServiceGrpc.getCountMappingMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getCountMappingMethod = SessionServiceGrpc.getCountMappingMethod) == null) {
SessionServiceGrpc.getCountMappingMethod = getCountMappingMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "CountMapping"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.mapping.CountMappingRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.mapping.CountMappingResponse.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("CountMapping"))
.build();
}
}
}
return getCountMappingMethod;
}
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getCacheMappingMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "CacheMapping",
requestType = com.dimajix.flowman.kernel.proto.mapping.CacheMappingRequest.class,
responseType = com.dimajix.flowman.kernel.proto.mapping.CacheMappingResponse.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
public static com.dimajix.shaded.grpc.MethodDescriptor getCacheMappingMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getCacheMappingMethod;
if ((getCacheMappingMethod = SessionServiceGrpc.getCacheMappingMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getCacheMappingMethod = SessionServiceGrpc.getCacheMappingMethod) == null) {
SessionServiceGrpc.getCacheMappingMethod = getCacheMappingMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "CacheMapping"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.mapping.CacheMappingRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.mapping.CacheMappingResponse.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("CacheMapping"))
.build();
}
}
}
return getCacheMappingMethod;
}
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getExplainMappingMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ExplainMapping",
requestType = com.dimajix.flowman.kernel.proto.mapping.ExplainMappingRequest.class,
responseType = com.dimajix.flowman.kernel.proto.mapping.ExplainMappingResponse.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
public static com.dimajix.shaded.grpc.MethodDescriptor getExplainMappingMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getExplainMappingMethod;
if ((getExplainMappingMethod = SessionServiceGrpc.getExplainMappingMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getExplainMappingMethod = SessionServiceGrpc.getExplainMappingMethod) == null) {
SessionServiceGrpc.getExplainMappingMethod = getExplainMappingMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ExplainMapping"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.mapping.ExplainMappingRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.mapping.ExplainMappingResponse.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("ExplainMapping"))
.build();
}
}
}
return getExplainMappingMethod;
}
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getListRelationsMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ListRelations",
requestType = com.dimajix.flowman.kernel.proto.relation.ListRelationsRequest.class,
responseType = com.dimajix.flowman.kernel.proto.relation.ListRelationsResponse.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
public static com.dimajix.shaded.grpc.MethodDescriptor getListRelationsMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getListRelationsMethod;
if ((getListRelationsMethod = SessionServiceGrpc.getListRelationsMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getListRelationsMethod = SessionServiceGrpc.getListRelationsMethod) == null) {
SessionServiceGrpc.getListRelationsMethod = getListRelationsMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ListRelations"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.relation.ListRelationsRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.relation.ListRelationsResponse.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("ListRelations"))
.build();
}
}
}
return getListRelationsMethod;
}
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getGetRelationMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetRelation",
requestType = com.dimajix.flowman.kernel.proto.relation.GetRelationRequest.class,
responseType = com.dimajix.flowman.kernel.proto.relation.GetRelationResponse.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
public static com.dimajix.shaded.grpc.MethodDescriptor getGetRelationMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getGetRelationMethod;
if ((getGetRelationMethod = SessionServiceGrpc.getGetRelationMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getGetRelationMethod = SessionServiceGrpc.getGetRelationMethod) == null) {
SessionServiceGrpc.getGetRelationMethod = getGetRelationMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetRelation"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.relation.GetRelationRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.relation.GetRelationResponse.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("GetRelation"))
.build();
}
}
}
return getGetRelationMethod;
}
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getReadRelationMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ReadRelation",
requestType = com.dimajix.flowman.kernel.proto.relation.ReadRelationRequest.class,
responseType = com.dimajix.flowman.kernel.proto.relation.ReadRelationResponse.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
public static com.dimajix.shaded.grpc.MethodDescriptor getReadRelationMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getReadRelationMethod;
if ((getReadRelationMethod = SessionServiceGrpc.getReadRelationMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getReadRelationMethod = SessionServiceGrpc.getReadRelationMethod) == null) {
SessionServiceGrpc.getReadRelationMethod = getReadRelationMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ReadRelation"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.relation.ReadRelationRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.relation.ReadRelationResponse.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("ReadRelation"))
.build();
}
}
}
return getReadRelationMethod;
}
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getExecuteRelationMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ExecuteRelation",
requestType = com.dimajix.flowman.kernel.proto.relation.ExecuteRelationRequest.class,
responseType = com.dimajix.flowman.kernel.proto.relation.ExecuteRelationResponse.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
public static com.dimajix.shaded.grpc.MethodDescriptor getExecuteRelationMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getExecuteRelationMethod;
if ((getExecuteRelationMethod = SessionServiceGrpc.getExecuteRelationMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getExecuteRelationMethod = SessionServiceGrpc.getExecuteRelationMethod) == null) {
SessionServiceGrpc.getExecuteRelationMethod = getExecuteRelationMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ExecuteRelation"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.relation.ExecuteRelationRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.relation.ExecuteRelationResponse.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("ExecuteRelation"))
.build();
}
}
}
return getExecuteRelationMethod;
}
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getDescribeRelationMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "DescribeRelation",
requestType = com.dimajix.flowman.kernel.proto.relation.DescribeRelationRequest.class,
responseType = com.dimajix.flowman.kernel.proto.relation.DescribeRelationResponse.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
public static com.dimajix.shaded.grpc.MethodDescriptor getDescribeRelationMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getDescribeRelationMethod;
if ((getDescribeRelationMethod = SessionServiceGrpc.getDescribeRelationMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getDescribeRelationMethod = SessionServiceGrpc.getDescribeRelationMethod) == null) {
SessionServiceGrpc.getDescribeRelationMethod = getDescribeRelationMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "DescribeRelation"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.relation.DescribeRelationRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.relation.DescribeRelationResponse.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("DescribeRelation"))
.build();
}
}
}
return getDescribeRelationMethod;
}
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getListTargetsMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ListTargets",
requestType = com.dimajix.flowman.kernel.proto.target.ListTargetsRequest.class,
responseType = com.dimajix.flowman.kernel.proto.target.ListTargetsResponse.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
public static com.dimajix.shaded.grpc.MethodDescriptor getListTargetsMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getListTargetsMethod;
if ((getListTargetsMethod = SessionServiceGrpc.getListTargetsMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getListTargetsMethod = SessionServiceGrpc.getListTargetsMethod) == null) {
SessionServiceGrpc.getListTargetsMethod = getListTargetsMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ListTargets"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.target.ListTargetsRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.target.ListTargetsResponse.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("ListTargets"))
.build();
}
}
}
return getListTargetsMethod;
}
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getGetTargetMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetTarget",
requestType = com.dimajix.flowman.kernel.proto.target.GetTargetRequest.class,
responseType = com.dimajix.flowman.kernel.proto.target.GetTargetResponse.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
public static com.dimajix.shaded.grpc.MethodDescriptor getGetTargetMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getGetTargetMethod;
if ((getGetTargetMethod = SessionServiceGrpc.getGetTargetMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getGetTargetMethod = SessionServiceGrpc.getGetTargetMethod) == null) {
SessionServiceGrpc.getGetTargetMethod = getGetTargetMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetTarget"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.target.GetTargetRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.target.GetTargetResponse.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("GetTarget"))
.build();
}
}
}
return getGetTargetMethod;
}
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getExecuteTargetMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ExecuteTarget",
requestType = com.dimajix.flowman.kernel.proto.target.ExecuteTargetRequest.class,
responseType = com.dimajix.flowman.kernel.proto.target.ExecuteTargetResponse.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
public static com.dimajix.shaded.grpc.MethodDescriptor getExecuteTargetMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getExecuteTargetMethod;
if ((getExecuteTargetMethod = SessionServiceGrpc.getExecuteTargetMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getExecuteTargetMethod = SessionServiceGrpc.getExecuteTargetMethod) == null) {
SessionServiceGrpc.getExecuteTargetMethod = getExecuteTargetMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ExecuteTarget"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.target.ExecuteTargetRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.target.ExecuteTargetResponse.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("ExecuteTarget"))
.build();
}
}
}
return getExecuteTargetMethod;
}
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getListTestsMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ListTests",
requestType = com.dimajix.flowman.kernel.proto.test.ListTestsRequest.class,
responseType = com.dimajix.flowman.kernel.proto.test.ListTestsResponse.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
public static com.dimajix.shaded.grpc.MethodDescriptor getListTestsMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getListTestsMethod;
if ((getListTestsMethod = SessionServiceGrpc.getListTestsMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getListTestsMethod = SessionServiceGrpc.getListTestsMethod) == null) {
SessionServiceGrpc.getListTestsMethod = getListTestsMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ListTests"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.test.ListTestsRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.test.ListTestsResponse.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("ListTests"))
.build();
}
}
}
return getListTestsMethod;
}
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getGetTestMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetTest",
requestType = com.dimajix.flowman.kernel.proto.test.GetTestRequest.class,
responseType = com.dimajix.flowman.kernel.proto.test.GetTestResponse.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
public static com.dimajix.shaded.grpc.MethodDescriptor getGetTestMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getGetTestMethod;
if ((getGetTestMethod = SessionServiceGrpc.getGetTestMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getGetTestMethod = SessionServiceGrpc.getGetTestMethod) == null) {
SessionServiceGrpc.getGetTestMethod = getGetTestMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetTest"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.test.GetTestRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.test.GetTestResponse.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("GetTest"))
.build();
}
}
}
return getGetTestMethod;
}
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getExecuteTestMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ExecuteTest",
requestType = com.dimajix.flowman.kernel.proto.test.ExecuteTestRequest.class,
responseType = com.dimajix.flowman.kernel.proto.test.ExecuteTestResponse.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
public static com.dimajix.shaded.grpc.MethodDescriptor getExecuteTestMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getExecuteTestMethod;
if ((getExecuteTestMethod = SessionServiceGrpc.getExecuteTestMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getExecuteTestMethod = SessionServiceGrpc.getExecuteTestMethod) == null) {
SessionServiceGrpc.getExecuteTestMethod = getExecuteTestMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ExecuteTest"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.test.ExecuteTestRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.test.ExecuteTestResponse.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("ExecuteTest"))
.build();
}
}
}
return getExecuteTestMethod;
}
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getListJobsMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ListJobs",
requestType = com.dimajix.flowman.kernel.proto.job.ListJobsRequest.class,
responseType = com.dimajix.flowman.kernel.proto.job.ListJobsResponse.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
public static com.dimajix.shaded.grpc.MethodDescriptor getListJobsMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getListJobsMethod;
if ((getListJobsMethod = SessionServiceGrpc.getListJobsMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getListJobsMethod = SessionServiceGrpc.getListJobsMethod) == null) {
SessionServiceGrpc.getListJobsMethod = getListJobsMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ListJobs"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.job.ListJobsRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.job.ListJobsResponse.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("ListJobs"))
.build();
}
}
}
return getListJobsMethod;
}
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getGetJobMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetJob",
requestType = com.dimajix.flowman.kernel.proto.job.GetJobRequest.class,
responseType = com.dimajix.flowman.kernel.proto.job.GetJobResponse.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
public static com.dimajix.shaded.grpc.MethodDescriptor getGetJobMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getGetJobMethod;
if ((getGetJobMethod = SessionServiceGrpc.getGetJobMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getGetJobMethod = SessionServiceGrpc.getGetJobMethod) == null) {
SessionServiceGrpc.getGetJobMethod = getGetJobMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetJob"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.job.GetJobRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.job.GetJobResponse.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("GetJob"))
.build();
}
}
}
return getGetJobMethod;
}
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getExecuteJobMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ExecuteJob",
requestType = com.dimajix.flowman.kernel.proto.job.ExecuteJobRequest.class,
responseType = com.dimajix.flowman.kernel.proto.job.ExecuteJobResponse.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
public static com.dimajix.shaded.grpc.MethodDescriptor getExecuteJobMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getExecuteJobMethod;
if ((getExecuteJobMethod = SessionServiceGrpc.getExecuteJobMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getExecuteJobMethod = SessionServiceGrpc.getExecuteJobMethod) == null) {
SessionServiceGrpc.getExecuteJobMethod = getExecuteJobMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ExecuteJob"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.job.ExecuteJobRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.job.ExecuteJobResponse.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("ExecuteJob"))
.build();
}
}
}
return getExecuteJobMethod;
}
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getGetProjectMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetProject",
requestType = com.dimajix.flowman.kernel.proto.project.GetProjectRequest.class,
responseType = com.dimajix.flowman.kernel.proto.project.GetProjectResponse.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
public static com.dimajix.shaded.grpc.MethodDescriptor getGetProjectMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getGetProjectMethod;
if ((getGetProjectMethod = SessionServiceGrpc.getGetProjectMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getGetProjectMethod = SessionServiceGrpc.getGetProjectMethod) == null) {
SessionServiceGrpc.getGetProjectMethod = getGetProjectMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetProject"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.project.GetProjectRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.project.GetProjectResponse.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("GetProject"))
.build();
}
}
}
return getGetProjectMethod;
}
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getExecuteProjectMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ExecuteProject",
requestType = com.dimajix.flowman.kernel.proto.project.ExecuteProjectRequest.class,
responseType = com.dimajix.flowman.kernel.proto.project.ExecuteProjectResponse.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
public static com.dimajix.shaded.grpc.MethodDescriptor getExecuteProjectMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getExecuteProjectMethod;
if ((getExecuteProjectMethod = SessionServiceGrpc.getExecuteProjectMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getExecuteProjectMethod = SessionServiceGrpc.getExecuteProjectMethod) == null) {
SessionServiceGrpc.getExecuteProjectMethod = getExecuteProjectMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ExecuteProject"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.project.ExecuteProjectRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.project.ExecuteProjectResponse.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("ExecuteProject"))
.build();
}
}
}
return getExecuteProjectMethod;
}
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getGenerateDocumentationMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "generateDocumentation",
requestType = com.dimajix.flowman.kernel.proto.documentation.GenerateDocumentationRequest.class,
responseType = com.dimajix.flowman.kernel.proto.documentation.GenerateDocumentationResponse.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
public static com.dimajix.shaded.grpc.MethodDescriptor getGenerateDocumentationMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getGenerateDocumentationMethod;
if ((getGenerateDocumentationMethod = SessionServiceGrpc.getGenerateDocumentationMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getGenerateDocumentationMethod = SessionServiceGrpc.getGenerateDocumentationMethod) == null) {
SessionServiceGrpc.getGenerateDocumentationMethod = getGenerateDocumentationMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "generateDocumentation"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.documentation.GenerateDocumentationRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.documentation.GenerateDocumentationResponse.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("generateDocumentation"))
.build();
}
}
}
return getGenerateDocumentationMethod;
}
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getExecuteSqlMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ExecuteSql",
requestType = com.dimajix.flowman.kernel.proto.session.ExecuteSqlRequest.class,
responseType = com.dimajix.flowman.kernel.proto.session.ExecuteSqlResponse.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
public static com.dimajix.shaded.grpc.MethodDescriptor getExecuteSqlMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getExecuteSqlMethod;
if ((getExecuteSqlMethod = SessionServiceGrpc.getExecuteSqlMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getExecuteSqlMethod = SessionServiceGrpc.getExecuteSqlMethod) == null) {
SessionServiceGrpc.getExecuteSqlMethod = getExecuteSqlMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ExecuteSql"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.session.ExecuteSqlRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.session.ExecuteSqlResponse.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("ExecuteSql"))
.build();
}
}
}
return getExecuteSqlMethod;
}
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getEvaluateExpressionMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "EvaluateExpression",
requestType = com.dimajix.flowman.kernel.proto.session.EvaluateExpressionRequest.class,
responseType = com.dimajix.flowman.kernel.proto.session.EvaluateExpressionResponse.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
public static com.dimajix.shaded.grpc.MethodDescriptor getEvaluateExpressionMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getEvaluateExpressionMethod;
if ((getEvaluateExpressionMethod = SessionServiceGrpc.getEvaluateExpressionMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getEvaluateExpressionMethod = SessionServiceGrpc.getEvaluateExpressionMethod) == null) {
SessionServiceGrpc.getEvaluateExpressionMethod = getEvaluateExpressionMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "EvaluateExpression"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.session.EvaluateExpressionRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.session.EvaluateExpressionResponse.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("EvaluateExpression"))
.build();
}
}
}
return getEvaluateExpressionMethod;
}
private static volatile com.dimajix.shaded.grpc.MethodDescriptor getSubscribeLogMethod;
@com.dimajix.shaded.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "SubscribeLog",
requestType = com.dimajix.flowman.kernel.proto.session.SubscribeLogRequest.class,
responseType = com.dimajix.flowman.kernel.proto.LogEvent.class,
methodType = com.dimajix.shaded.grpc.MethodDescriptor.MethodType.SERVER_STREAMING)
public static com.dimajix.shaded.grpc.MethodDescriptor getSubscribeLogMethod() {
com.dimajix.shaded.grpc.MethodDescriptor getSubscribeLogMethod;
if ((getSubscribeLogMethod = SessionServiceGrpc.getSubscribeLogMethod) == null) {
synchronized (SessionServiceGrpc.class) {
if ((getSubscribeLogMethod = SessionServiceGrpc.getSubscribeLogMethod) == null) {
SessionServiceGrpc.getSubscribeLogMethod = getSubscribeLogMethod =
com.dimajix.shaded.grpc.MethodDescriptor.newBuilder()
.setType(com.dimajix.shaded.grpc.MethodDescriptor.MethodType.SERVER_STREAMING)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "SubscribeLog"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.session.SubscribeLogRequest.getDefaultInstance()))
.setResponseMarshaller(com.dimajix.shaded.grpc.protobuf.ProtoUtils.marshaller(
com.dimajix.flowman.kernel.proto.LogEvent.getDefaultInstance()))
.setSchemaDescriptor(new SessionServiceMethodDescriptorSupplier("SubscribeLog"))
.build();
}
}
}
return getSubscribeLogMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static SessionServiceStub newStub(com.dimajix.shaded.grpc.Channel channel) {
com.dimajix.shaded.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public SessionServiceStub newStub(com.dimajix.shaded.grpc.Channel channel, com.dimajix.shaded.grpc.CallOptions callOptions) {
return new SessionServiceStub(channel, callOptions);
}
};
return SessionServiceStub.newStub(factory, channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static SessionServiceBlockingStub newBlockingStub(
com.dimajix.shaded.grpc.Channel channel) {
com.dimajix.shaded.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public SessionServiceBlockingStub newStub(com.dimajix.shaded.grpc.Channel channel, com.dimajix.shaded.grpc.CallOptions callOptions) {
return new SessionServiceBlockingStub(channel, callOptions);
}
};
return SessionServiceBlockingStub.newStub(factory, channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static SessionServiceFutureStub newFutureStub(
com.dimajix.shaded.grpc.Channel channel) {
com.dimajix.shaded.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public SessionServiceFutureStub newStub(com.dimajix.shaded.grpc.Channel channel, com.dimajix.shaded.grpc.CallOptions callOptions) {
return new SessionServiceFutureStub(channel, callOptions);
}
};
return SessionServiceFutureStub.newStub(factory, channel);
}
/**
*/
public interface AsyncService {
/**
*/
default void createSession(com.dimajix.flowman.kernel.proto.session.CreateSessionRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getCreateSessionMethod(), responseObserver);
}
/**
*/
default void listSessions(com.dimajix.flowman.kernel.proto.session.ListSessionsRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getListSessionsMethod(), responseObserver);
}
/**
*/
default void getSession(com.dimajix.flowman.kernel.proto.session.GetSessionRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetSessionMethod(), responseObserver);
}
/**
*/
default void deleteSession(com.dimajix.flowman.kernel.proto.session.DeleteSessionRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getDeleteSessionMethod(), responseObserver);
}
/**
*/
default void enterContext(com.dimajix.flowman.kernel.proto.session.EnterContextRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getEnterContextMethod(), responseObserver);
}
/**
*/
default void leaveContext(com.dimajix.flowman.kernel.proto.session.LeaveContextRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getLeaveContextMethod(), responseObserver);
}
/**
*/
default void getContext(com.dimajix.flowman.kernel.proto.session.GetContextRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetContextMethod(), responseObserver);
}
/**
*
* Mappings stuff
*
*/
default void listMappings(com.dimajix.flowman.kernel.proto.mapping.ListMappingsRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getListMappingsMethod(), responseObserver);
}
/**
*/
default void getMapping(com.dimajix.flowman.kernel.proto.mapping.GetMappingRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetMappingMethod(), responseObserver);
}
/**
*/
default void readMapping(com.dimajix.flowman.kernel.proto.mapping.ReadMappingRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getReadMappingMethod(), responseObserver);
}
/**
*/
default void describeMapping(com.dimajix.flowman.kernel.proto.mapping.DescribeMappingRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getDescribeMappingMethod(), responseObserver);
}
/**
*/
default void validateMapping(com.dimajix.flowman.kernel.proto.mapping.ValidateMappingRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getValidateMappingMethod(), responseObserver);
}
/**
*/
default void saveMappingOutput(com.dimajix.flowman.kernel.proto.mapping.SaveMappingOutputRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getSaveMappingOutputMethod(), responseObserver);
}
/**
*/
default void countMapping(com.dimajix.flowman.kernel.proto.mapping.CountMappingRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getCountMappingMethod(), responseObserver);
}
/**
*/
default void cacheMapping(com.dimajix.flowman.kernel.proto.mapping.CacheMappingRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getCacheMappingMethod(), responseObserver);
}
/**
*/
default void explainMapping(com.dimajix.flowman.kernel.proto.mapping.ExplainMappingRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getExplainMappingMethod(), responseObserver);
}
/**
*
* Relations stuff
*
*/
default void listRelations(com.dimajix.flowman.kernel.proto.relation.ListRelationsRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getListRelationsMethod(), responseObserver);
}
/**
*/
default void getRelation(com.dimajix.flowman.kernel.proto.relation.GetRelationRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetRelationMethod(), responseObserver);
}
/**
*/
default void readRelation(com.dimajix.flowman.kernel.proto.relation.ReadRelationRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getReadRelationMethod(), responseObserver);
}
/**
*/
default void executeRelation(com.dimajix.flowman.kernel.proto.relation.ExecuteRelationRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getExecuteRelationMethod(), responseObserver);
}
/**
*/
default void describeRelation(com.dimajix.flowman.kernel.proto.relation.DescribeRelationRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getDescribeRelationMethod(), responseObserver);
}
/**
*
* Targets stuff
*
*/
default void listTargets(com.dimajix.flowman.kernel.proto.target.ListTargetsRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getListTargetsMethod(), responseObserver);
}
/**
*/
default void getTarget(com.dimajix.flowman.kernel.proto.target.GetTargetRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetTargetMethod(), responseObserver);
}
/**
*/
default void executeTarget(com.dimajix.flowman.kernel.proto.target.ExecuteTargetRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getExecuteTargetMethod(), responseObserver);
}
/**
*
* Tests stuff
*
*/
default void listTests(com.dimajix.flowman.kernel.proto.test.ListTestsRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getListTestsMethod(), responseObserver);
}
/**
*/
default void getTest(com.dimajix.flowman.kernel.proto.test.GetTestRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetTestMethod(), responseObserver);
}
/**
*/
default void executeTest(com.dimajix.flowman.kernel.proto.test.ExecuteTestRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getExecuteTestMethod(), responseObserver);
}
/**
*
* Job stuff
*
*/
default void listJobs(com.dimajix.flowman.kernel.proto.job.ListJobsRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getListJobsMethod(), responseObserver);
}
/**
*/
default void getJob(com.dimajix.flowman.kernel.proto.job.GetJobRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetJobMethod(), responseObserver);
}
/**
*/
default void executeJob(com.dimajix.flowman.kernel.proto.job.ExecuteJobRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getExecuteJobMethod(), responseObserver);
}
/**
*
* Project stuff
*
*/
default void getProject(com.dimajix.flowman.kernel.proto.project.GetProjectRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetProjectMethod(), responseObserver);
}
/**
*/
default void executeProject(com.dimajix.flowman.kernel.proto.project.ExecuteProjectRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getExecuteProjectMethod(), responseObserver);
}
/**
*
* Documentation
*
*/
default void generateDocumentation(com.dimajix.flowman.kernel.proto.documentation.GenerateDocumentationRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGenerateDocumentationMethod(), responseObserver);
}
/**
*
* Generic stuff
*
*/
default void executeSql(com.dimajix.flowman.kernel.proto.session.ExecuteSqlRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getExecuteSqlMethod(), responseObserver);
}
/**
*/
default void evaluateExpression(com.dimajix.flowman.kernel.proto.session.EvaluateExpressionRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getEvaluateExpressionMethod(), responseObserver);
}
/**
*/
default void subscribeLog(com.dimajix.flowman.kernel.proto.session.SubscribeLogRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getSubscribeLogMethod(), responseObserver);
}
}
/**
* Base class for the server implementation of the service SessionService.
*/
public static abstract class SessionServiceImplBase
implements com.dimajix.shaded.grpc.BindableService, AsyncService {
@java.lang.Override public final com.dimajix.shaded.grpc.ServerServiceDefinition bindService() {
return SessionServiceGrpc.bindService(this);
}
}
/**
* A stub to allow clients to do asynchronous rpc calls to service SessionService.
*/
public static final class SessionServiceStub
extends com.dimajix.shaded.grpc.stub.AbstractAsyncStub {
private SessionServiceStub(
com.dimajix.shaded.grpc.Channel channel, com.dimajix.shaded.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected SessionServiceStub build(
com.dimajix.shaded.grpc.Channel channel, com.dimajix.shaded.grpc.CallOptions callOptions) {
return new SessionServiceStub(channel, callOptions);
}
/**
*/
public void createSession(com.dimajix.flowman.kernel.proto.session.CreateSessionRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getCreateSessionMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void listSessions(com.dimajix.flowman.kernel.proto.session.ListSessionsRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getListSessionsMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void getSession(com.dimajix.flowman.kernel.proto.session.GetSessionRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGetSessionMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void deleteSession(com.dimajix.flowman.kernel.proto.session.DeleteSessionRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getDeleteSessionMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void enterContext(com.dimajix.flowman.kernel.proto.session.EnterContextRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getEnterContextMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void leaveContext(com.dimajix.flowman.kernel.proto.session.LeaveContextRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getLeaveContextMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void getContext(com.dimajix.flowman.kernel.proto.session.GetContextRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGetContextMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Mappings stuff
*
*/
public void listMappings(com.dimajix.flowman.kernel.proto.mapping.ListMappingsRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getListMappingsMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void getMapping(com.dimajix.flowman.kernel.proto.mapping.GetMappingRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGetMappingMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void readMapping(com.dimajix.flowman.kernel.proto.mapping.ReadMappingRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getReadMappingMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void describeMapping(com.dimajix.flowman.kernel.proto.mapping.DescribeMappingRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getDescribeMappingMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void validateMapping(com.dimajix.flowman.kernel.proto.mapping.ValidateMappingRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getValidateMappingMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void saveMappingOutput(com.dimajix.flowman.kernel.proto.mapping.SaveMappingOutputRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getSaveMappingOutputMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void countMapping(com.dimajix.flowman.kernel.proto.mapping.CountMappingRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getCountMappingMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void cacheMapping(com.dimajix.flowman.kernel.proto.mapping.CacheMappingRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getCacheMappingMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void explainMapping(com.dimajix.flowman.kernel.proto.mapping.ExplainMappingRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getExplainMappingMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Relations stuff
*
*/
public void listRelations(com.dimajix.flowman.kernel.proto.relation.ListRelationsRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getListRelationsMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void getRelation(com.dimajix.flowman.kernel.proto.relation.GetRelationRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGetRelationMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void readRelation(com.dimajix.flowman.kernel.proto.relation.ReadRelationRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getReadRelationMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void executeRelation(com.dimajix.flowman.kernel.proto.relation.ExecuteRelationRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getExecuteRelationMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void describeRelation(com.dimajix.flowman.kernel.proto.relation.DescribeRelationRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getDescribeRelationMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Targets stuff
*
*/
public void listTargets(com.dimajix.flowman.kernel.proto.target.ListTargetsRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getListTargetsMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void getTarget(com.dimajix.flowman.kernel.proto.target.GetTargetRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGetTargetMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void executeTarget(com.dimajix.flowman.kernel.proto.target.ExecuteTargetRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getExecuteTargetMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Tests stuff
*
*/
public void listTests(com.dimajix.flowman.kernel.proto.test.ListTestsRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getListTestsMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void getTest(com.dimajix.flowman.kernel.proto.test.GetTestRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGetTestMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void executeTest(com.dimajix.flowman.kernel.proto.test.ExecuteTestRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getExecuteTestMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Job stuff
*
*/
public void listJobs(com.dimajix.flowman.kernel.proto.job.ListJobsRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getListJobsMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void getJob(com.dimajix.flowman.kernel.proto.job.GetJobRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGetJobMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void executeJob(com.dimajix.flowman.kernel.proto.job.ExecuteJobRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getExecuteJobMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Project stuff
*
*/
public void getProject(com.dimajix.flowman.kernel.proto.project.GetProjectRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGetProjectMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void executeProject(com.dimajix.flowman.kernel.proto.project.ExecuteProjectRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getExecuteProjectMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Documentation
*
*/
public void generateDocumentation(com.dimajix.flowman.kernel.proto.documentation.GenerateDocumentationRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGenerateDocumentationMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Generic stuff
*
*/
public void executeSql(com.dimajix.flowman.kernel.proto.session.ExecuteSqlRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getExecuteSqlMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void evaluateExpression(com.dimajix.flowman.kernel.proto.session.EvaluateExpressionRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getEvaluateExpressionMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void subscribeLog(com.dimajix.flowman.kernel.proto.session.SubscribeLogRequest request,
com.dimajix.shaded.grpc.stub.StreamObserver responseObserver) {
com.dimajix.shaded.grpc.stub.ClientCalls.asyncServerStreamingCall(
getChannel().newCall(getSubscribeLogMethod(), getCallOptions()), request, responseObserver);
}
}
/**
* A stub to allow clients to do synchronous rpc calls to service SessionService.
*/
public static final class SessionServiceBlockingStub
extends com.dimajix.shaded.grpc.stub.AbstractBlockingStub {
private SessionServiceBlockingStub(
com.dimajix.shaded.grpc.Channel channel, com.dimajix.shaded.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected SessionServiceBlockingStub build(
com.dimajix.shaded.grpc.Channel channel, com.dimajix.shaded.grpc.CallOptions callOptions) {
return new SessionServiceBlockingStub(channel, callOptions);
}
/**
*/
public com.dimajix.flowman.kernel.proto.session.CreateSessionResponse createSession(com.dimajix.flowman.kernel.proto.session.CreateSessionRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getCreateSessionMethod(), getCallOptions(), request);
}
/**
*/
public com.dimajix.flowman.kernel.proto.session.ListSessionsResponse listSessions(com.dimajix.flowman.kernel.proto.session.ListSessionsRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getListSessionsMethod(), getCallOptions(), request);
}
/**
*/
public com.dimajix.flowman.kernel.proto.session.GetSessionResponse getSession(com.dimajix.flowman.kernel.proto.session.GetSessionRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGetSessionMethod(), getCallOptions(), request);
}
/**
*/
public com.dimajix.flowman.kernel.proto.session.DeleteSessionResponse deleteSession(com.dimajix.flowman.kernel.proto.session.DeleteSessionRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getDeleteSessionMethod(), getCallOptions(), request);
}
/**
*/
public com.dimajix.flowman.kernel.proto.session.EnterContextResponse enterContext(com.dimajix.flowman.kernel.proto.session.EnterContextRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getEnterContextMethod(), getCallOptions(), request);
}
/**
*/
public com.dimajix.flowman.kernel.proto.session.LeaveContextResponse leaveContext(com.dimajix.flowman.kernel.proto.session.LeaveContextRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getLeaveContextMethod(), getCallOptions(), request);
}
/**
*/
public com.dimajix.flowman.kernel.proto.session.GetContextResponse getContext(com.dimajix.flowman.kernel.proto.session.GetContextRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGetContextMethod(), getCallOptions(), request);
}
/**
*
* Mappings stuff
*
*/
public com.dimajix.flowman.kernel.proto.mapping.ListMappingsResponse listMappings(com.dimajix.flowman.kernel.proto.mapping.ListMappingsRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getListMappingsMethod(), getCallOptions(), request);
}
/**
*/
public com.dimajix.flowman.kernel.proto.mapping.GetMappingResponse getMapping(com.dimajix.flowman.kernel.proto.mapping.GetMappingRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGetMappingMethod(), getCallOptions(), request);
}
/**
*/
public com.dimajix.flowman.kernel.proto.mapping.ReadMappingResponse readMapping(com.dimajix.flowman.kernel.proto.mapping.ReadMappingRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getReadMappingMethod(), getCallOptions(), request);
}
/**
*/
public com.dimajix.flowman.kernel.proto.mapping.DescribeMappingResponse describeMapping(com.dimajix.flowman.kernel.proto.mapping.DescribeMappingRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getDescribeMappingMethod(), getCallOptions(), request);
}
/**
*/
public com.dimajix.flowman.kernel.proto.mapping.ValidateMappingResponse validateMapping(com.dimajix.flowman.kernel.proto.mapping.ValidateMappingRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getValidateMappingMethod(), getCallOptions(), request);
}
/**
*/
public com.dimajix.flowman.kernel.proto.mapping.SaveMappingOutputResponse saveMappingOutput(com.dimajix.flowman.kernel.proto.mapping.SaveMappingOutputRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getSaveMappingOutputMethod(), getCallOptions(), request);
}
/**
*/
public com.dimajix.flowman.kernel.proto.mapping.CountMappingResponse countMapping(com.dimajix.flowman.kernel.proto.mapping.CountMappingRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getCountMappingMethod(), getCallOptions(), request);
}
/**
*/
public com.dimajix.flowman.kernel.proto.mapping.CacheMappingResponse cacheMapping(com.dimajix.flowman.kernel.proto.mapping.CacheMappingRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getCacheMappingMethod(), getCallOptions(), request);
}
/**
*/
public com.dimajix.flowman.kernel.proto.mapping.ExplainMappingResponse explainMapping(com.dimajix.flowman.kernel.proto.mapping.ExplainMappingRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getExplainMappingMethod(), getCallOptions(), request);
}
/**
*
* Relations stuff
*
*/
public com.dimajix.flowman.kernel.proto.relation.ListRelationsResponse listRelations(com.dimajix.flowman.kernel.proto.relation.ListRelationsRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getListRelationsMethod(), getCallOptions(), request);
}
/**
*/
public com.dimajix.flowman.kernel.proto.relation.GetRelationResponse getRelation(com.dimajix.flowman.kernel.proto.relation.GetRelationRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGetRelationMethod(), getCallOptions(), request);
}
/**
*/
public com.dimajix.flowman.kernel.proto.relation.ReadRelationResponse readRelation(com.dimajix.flowman.kernel.proto.relation.ReadRelationRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getReadRelationMethod(), getCallOptions(), request);
}
/**
*/
public com.dimajix.flowman.kernel.proto.relation.ExecuteRelationResponse executeRelation(com.dimajix.flowman.kernel.proto.relation.ExecuteRelationRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getExecuteRelationMethod(), getCallOptions(), request);
}
/**
*/
public com.dimajix.flowman.kernel.proto.relation.DescribeRelationResponse describeRelation(com.dimajix.flowman.kernel.proto.relation.DescribeRelationRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getDescribeRelationMethod(), getCallOptions(), request);
}
/**
*
* Targets stuff
*
*/
public com.dimajix.flowman.kernel.proto.target.ListTargetsResponse listTargets(com.dimajix.flowman.kernel.proto.target.ListTargetsRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getListTargetsMethod(), getCallOptions(), request);
}
/**
*/
public com.dimajix.flowman.kernel.proto.target.GetTargetResponse getTarget(com.dimajix.flowman.kernel.proto.target.GetTargetRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGetTargetMethod(), getCallOptions(), request);
}
/**
*/
public com.dimajix.flowman.kernel.proto.target.ExecuteTargetResponse executeTarget(com.dimajix.flowman.kernel.proto.target.ExecuteTargetRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getExecuteTargetMethod(), getCallOptions(), request);
}
/**
*
* Tests stuff
*
*/
public com.dimajix.flowman.kernel.proto.test.ListTestsResponse listTests(com.dimajix.flowman.kernel.proto.test.ListTestsRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getListTestsMethod(), getCallOptions(), request);
}
/**
*/
public com.dimajix.flowman.kernel.proto.test.GetTestResponse getTest(com.dimajix.flowman.kernel.proto.test.GetTestRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGetTestMethod(), getCallOptions(), request);
}
/**
*/
public com.dimajix.flowman.kernel.proto.test.ExecuteTestResponse executeTest(com.dimajix.flowman.kernel.proto.test.ExecuteTestRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getExecuteTestMethod(), getCallOptions(), request);
}
/**
*
* Job stuff
*
*/
public com.dimajix.flowman.kernel.proto.job.ListJobsResponse listJobs(com.dimajix.flowman.kernel.proto.job.ListJobsRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getListJobsMethod(), getCallOptions(), request);
}
/**
*/
public com.dimajix.flowman.kernel.proto.job.GetJobResponse getJob(com.dimajix.flowman.kernel.proto.job.GetJobRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGetJobMethod(), getCallOptions(), request);
}
/**
*/
public com.dimajix.flowman.kernel.proto.job.ExecuteJobResponse executeJob(com.dimajix.flowman.kernel.proto.job.ExecuteJobRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getExecuteJobMethod(), getCallOptions(), request);
}
/**
*
* Project stuff
*
*/
public com.dimajix.flowman.kernel.proto.project.GetProjectResponse getProject(com.dimajix.flowman.kernel.proto.project.GetProjectRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGetProjectMethod(), getCallOptions(), request);
}
/**
*/
public com.dimajix.flowman.kernel.proto.project.ExecuteProjectResponse executeProject(com.dimajix.flowman.kernel.proto.project.ExecuteProjectRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getExecuteProjectMethod(), getCallOptions(), request);
}
/**
*
* Documentation
*
*/
public com.dimajix.flowman.kernel.proto.documentation.GenerateDocumentationResponse generateDocumentation(com.dimajix.flowman.kernel.proto.documentation.GenerateDocumentationRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGenerateDocumentationMethod(), getCallOptions(), request);
}
/**
*
* Generic stuff
*
*/
public com.dimajix.flowman.kernel.proto.session.ExecuteSqlResponse executeSql(com.dimajix.flowman.kernel.proto.session.ExecuteSqlRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getExecuteSqlMethod(), getCallOptions(), request);
}
/**
*/
public com.dimajix.flowman.kernel.proto.session.EvaluateExpressionResponse evaluateExpression(com.dimajix.flowman.kernel.proto.session.EvaluateExpressionRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getEvaluateExpressionMethod(), getCallOptions(), request);
}
/**
*/
public java.util.Iterator subscribeLog(
com.dimajix.flowman.kernel.proto.session.SubscribeLogRequest request) {
return io.grpc.stub.ClientCalls.blockingServerStreamingCall(
getChannel(), getSubscribeLogMethod(), getCallOptions(), request);
}
}
/**
* A stub to allow clients to do ListenableFuture-style rpc calls to service SessionService.
*/
public static final class SessionServiceFutureStub
extends com.dimajix.shaded.grpc.stub.AbstractFutureStub {
private SessionServiceFutureStub(
com.dimajix.shaded.grpc.Channel channel, com.dimajix.shaded.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected SessionServiceFutureStub build(
com.dimajix.shaded.grpc.Channel channel, com.dimajix.shaded.grpc.CallOptions callOptions) {
return new SessionServiceFutureStub(channel, callOptions);
}
/**
*/
public com.dimajix.shaded.guava.util.concurrent.ListenableFuture createSession(
com.dimajix.flowman.kernel.proto.session.CreateSessionRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getCreateSessionMethod(), getCallOptions()), request);
}
/**
*/
public com.dimajix.shaded.guava.util.concurrent.ListenableFuture listSessions(
com.dimajix.flowman.kernel.proto.session.ListSessionsRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getListSessionsMethod(), getCallOptions()), request);
}
/**
*/
public com.dimajix.shaded.guava.util.concurrent.ListenableFuture getSession(
com.dimajix.flowman.kernel.proto.session.GetSessionRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getGetSessionMethod(), getCallOptions()), request);
}
/**
*/
public com.dimajix.shaded.guava.util.concurrent.ListenableFuture deleteSession(
com.dimajix.flowman.kernel.proto.session.DeleteSessionRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getDeleteSessionMethod(), getCallOptions()), request);
}
/**
*/
public com.dimajix.shaded.guava.util.concurrent.ListenableFuture enterContext(
com.dimajix.flowman.kernel.proto.session.EnterContextRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getEnterContextMethod(), getCallOptions()), request);
}
/**
*/
public com.dimajix.shaded.guava.util.concurrent.ListenableFuture leaveContext(
com.dimajix.flowman.kernel.proto.session.LeaveContextRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getLeaveContextMethod(), getCallOptions()), request);
}
/**
*/
public com.dimajix.shaded.guava.util.concurrent.ListenableFuture getContext(
com.dimajix.flowman.kernel.proto.session.GetContextRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getGetContextMethod(), getCallOptions()), request);
}
/**
*
* Mappings stuff
*
*/
public com.dimajix.shaded.guava.util.concurrent.ListenableFuture listMappings(
com.dimajix.flowman.kernel.proto.mapping.ListMappingsRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getListMappingsMethod(), getCallOptions()), request);
}
/**
*/
public com.dimajix.shaded.guava.util.concurrent.ListenableFuture getMapping(
com.dimajix.flowman.kernel.proto.mapping.GetMappingRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getGetMappingMethod(), getCallOptions()), request);
}
/**
*/
public com.dimajix.shaded.guava.util.concurrent.ListenableFuture readMapping(
com.dimajix.flowman.kernel.proto.mapping.ReadMappingRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getReadMappingMethod(), getCallOptions()), request);
}
/**
*/
public com.dimajix.shaded.guava.util.concurrent.ListenableFuture describeMapping(
com.dimajix.flowman.kernel.proto.mapping.DescribeMappingRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getDescribeMappingMethod(), getCallOptions()), request);
}
/**
*/
public com.dimajix.shaded.guava.util.concurrent.ListenableFuture validateMapping(
com.dimajix.flowman.kernel.proto.mapping.ValidateMappingRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getValidateMappingMethod(), getCallOptions()), request);
}
/**
*/
public com.dimajix.shaded.guava.util.concurrent.ListenableFuture saveMappingOutput(
com.dimajix.flowman.kernel.proto.mapping.SaveMappingOutputRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getSaveMappingOutputMethod(), getCallOptions()), request);
}
/**
*/
public com.dimajix.shaded.guava.util.concurrent.ListenableFuture countMapping(
com.dimajix.flowman.kernel.proto.mapping.CountMappingRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getCountMappingMethod(), getCallOptions()), request);
}
/**
*/
public com.dimajix.shaded.guava.util.concurrent.ListenableFuture cacheMapping(
com.dimajix.flowman.kernel.proto.mapping.CacheMappingRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getCacheMappingMethod(), getCallOptions()), request);
}
/**
*/
public com.dimajix.shaded.guava.util.concurrent.ListenableFuture explainMapping(
com.dimajix.flowman.kernel.proto.mapping.ExplainMappingRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getExplainMappingMethod(), getCallOptions()), request);
}
/**
*
* Relations stuff
*
*/
public com.dimajix.shaded.guava.util.concurrent.ListenableFuture listRelations(
com.dimajix.flowman.kernel.proto.relation.ListRelationsRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getListRelationsMethod(), getCallOptions()), request);
}
/**
*/
public com.dimajix.shaded.guava.util.concurrent.ListenableFuture getRelation(
com.dimajix.flowman.kernel.proto.relation.GetRelationRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getGetRelationMethod(), getCallOptions()), request);
}
/**
*/
public com.dimajix.shaded.guava.util.concurrent.ListenableFuture readRelation(
com.dimajix.flowman.kernel.proto.relation.ReadRelationRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getReadRelationMethod(), getCallOptions()), request);
}
/**
*/
public com.dimajix.shaded.guava.util.concurrent.ListenableFuture executeRelation(
com.dimajix.flowman.kernel.proto.relation.ExecuteRelationRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getExecuteRelationMethod(), getCallOptions()), request);
}
/**
*/
public com.dimajix.shaded.guava.util.concurrent.ListenableFuture describeRelation(
com.dimajix.flowman.kernel.proto.relation.DescribeRelationRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getDescribeRelationMethod(), getCallOptions()), request);
}
/**
*
* Targets stuff
*
*/
public com.dimajix.shaded.guava.util.concurrent.ListenableFuture listTargets(
com.dimajix.flowman.kernel.proto.target.ListTargetsRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getListTargetsMethod(), getCallOptions()), request);
}
/**
*/
public com.dimajix.shaded.guava.util.concurrent.ListenableFuture getTarget(
com.dimajix.flowman.kernel.proto.target.GetTargetRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getGetTargetMethod(), getCallOptions()), request);
}
/**
*/
public com.dimajix.shaded.guava.util.concurrent.ListenableFuture executeTarget(
com.dimajix.flowman.kernel.proto.target.ExecuteTargetRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getExecuteTargetMethod(), getCallOptions()), request);
}
/**
*