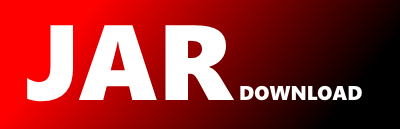
com.dimajix.shaded.everit.schema.loader.JsonArray Maven / Gradle / Ivy
package com.dimajix.shaded.everit.schema.loader;
import static java.util.Objects.requireNonNull;
import java.util.ArrayList;
import java.util.List;
import java.util.function.Function;
/**
* @author erosb
*/
final class JsonArray extends JsonValue {
private List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy