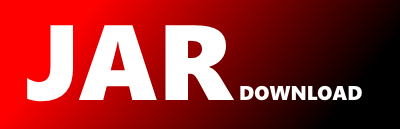
com.dimajix.shaded.everit.schema.loader.JsonObject Maven / Gradle / Ivy
package com.dimajix.shaded.everit.schema.loader;
import static java.lang.String.format;
import static java.util.Collections.unmodifiableMap;
import static java.util.Collections.unmodifiableSet;
import java.util.HashMap;
import java.util.Map;
import java.util.Optional;
import java.util.Set;
import java.util.function.Consumer;
import java.util.function.Function;
import com.dimajix.shaded.everit.schema.SchemaException;
/**
* @author erosb
*/
class JsonObject extends JsonValue {
final Map storage;
JsonObject(Map storage) {
super(storage);
this.storage = storage;
}
JsonValue childFor(String key) {
return ls.childFor(key);
}
boolean containsKey(String key) {
return storage.containsKey(key);
}
void require(String key, Consumer consumer) {
if (storage.containsKey(key)) {
consumer.accept(childFor(key));
} else {
throw failureOfMissingKey(key);
}
}
JsonValue require(String key) {
return requireMapping(key, e -> e);
}
R requireMapping(String key, Function fn) {
if (storage.containsKey(key)) {
return fn.apply(childFor(key));
} else {
throw failureOfMissingKey(key);
}
}
private SchemaException failureOfMissingKey(String key) {
return ls.createSchemaException(format("required key [%s] not found", key));
}
void maybe(String key, Consumer consumer) {
if (storage.containsKey(key)) {
consumer.accept(childFor(key));
}
}
Optional maybe(String key) {
return maybeMapping(key, identity());
}
Optional maybeMapping(String key, Function fn) {
if (storage.containsKey(key)) {
return Optional.of(fn.apply(childFor(key)));
} else {
return Optional.empty();
}
}
void forEach(JsonObjectIterator iterator) {
storage.entrySet().forEach(entry -> iterateOnEntry(entry, iterator));
}
private void iterateOnEntry(Map.Entry entry, JsonObjectIterator iterator) {
String key = entry.getKey();
iterator.apply(key, childFor(key));
}
@Override public R requireObject(Function mapper) {
return mapper.apply(this);
}
@Override protected Class> typeOfValue() {
return JsonObject.class;
}
@Override protected Object value() {
return this;
}
@Override protected Object unwrap() {
return new HashMap<>(storage);
}
Map toMap() {
if (storage == null) {
return null;
}
return unmodifiableMap(storage);
}
boolean isEmpty() {
return storage.isEmpty();
}
public Set keySet() {
return unmodifiableSet(storage.keySet());
}
public Object get(String name) {
return storage.get(name);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy