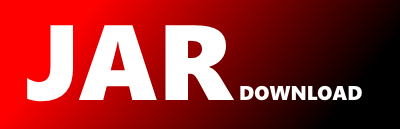
shade.com.alibaba.fastjson2.reader.ObjectReaderImplObject Maven / Gradle / Ivy
package com.alibaba.fastjson2.reader;
import com.alibaba.fastjson2.*;
import com.alibaba.fastjson2.util.Fnv;
import java.lang.reflect.Type;
import java.util.Collection;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.function.Supplier;
import static com.alibaba.fastjson2.JSONB.Constants.*;
public final class ObjectReaderImplObject
extends ObjectReaderPrimitive {
public static final ObjectReaderImplObject INSTANCE = new ObjectReaderImplObject();
public ObjectReaderImplObject() {
super(Object.class);
}
@Override
public Object createInstance(long features) {
return new JSONObject();
}
public Object createInstance(Collection collection) {
return collection;
}
@Override
public Object createInstance(Map map, long features) {
ObjectReaderProvider provider = JSONFactory.getDefaultObjectReaderProvider();
Object typeKey = map.get(getTypeKey());
if (typeKey instanceof String) {
String typeName = (String) typeKey;
long typeHash = Fnv.hashCode64(typeName);
ObjectReader reader = null;
if ((features & JSONReader.Feature.SupportAutoType.mask) != 0) {
reader = autoType(provider, typeHash);
}
if (reader == null) {
reader = provider.getObjectReader(
typeName, getObjectClass(), features | getFeatures()
);
if (reader == null) {
throw new JSONException("No suitable ObjectReader found for" + typeName);
}
}
if (reader != this) {
return reader.createInstance(map, features);
}
}
return map;
}
@Override
public Object readObject(JSONReader jsonReader, Type fieldType, Object fieldName, long features) {
if (jsonReader.jsonb) {
return jsonReader.readAny();
}
JSONReader.Context context = jsonReader.getContext();
long contextFeatures = features | context.getFeatures();
String typeName = null;
if (jsonReader.isObject()) {
jsonReader.nextIfObjectStart();
long hash = 0;
if (jsonReader.isString()) {
hash = jsonReader.readFieldNameHashCode();
if (hash == HASH_TYPE) {
boolean supportAutoType = context.isEnabled(JSONReader.Feature.SupportAutoType);
ObjectReader autoTypeObjectReader;
if (supportAutoType) {
long typeHash = jsonReader.readTypeHashCode();
autoTypeObjectReader = context.getObjectReaderAutoType(typeHash);
if (autoTypeObjectReader != null) {
Class objectClass = autoTypeObjectReader.getObjectClass();
if (objectClass != null) {
ClassLoader objectClassLoader = objectClass.getClassLoader();
ClassLoader classLoader = Thread.currentThread().getContextClassLoader();
if (objectClassLoader != classLoader) {
Class contextClass = null;
typeName = jsonReader.getString();
try {
if (classLoader == null) {
classLoader = getClass().getClassLoader();
}
contextClass = classLoader.loadClass(typeName);
} catch (ClassNotFoundException ignored) {
}
if (!objectClass.equals(contextClass)) {
autoTypeObjectReader = context.getObjectReader(contextClass);
}
}
}
}
if (autoTypeObjectReader == null) {
typeName = jsonReader.getString();
autoTypeObjectReader = context.getObjectReaderAutoType(typeName, null);
}
} else {
typeName = jsonReader.readString();
autoTypeObjectReader = context.getObjectReaderAutoType(typeName, null);
if (autoTypeObjectReader == null && jsonReader.getContext().isEnabled(JSONReader.Feature.ErrorOnNotSupportAutoType)) {
throw new JSONException(jsonReader.info("autoType not support : " + typeName));
}
}
if (autoTypeObjectReader != null) {
jsonReader.setTypeRedirect(true);
return autoTypeObjectReader.readObject(jsonReader, fieldType, fieldName, features);
}
}
}
Map object;
Supplier
© 2015 - 2025 Weber Informatics LLC | Privacy Policy