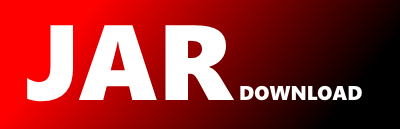
shade.com.alibaba.fastjson2.reader.ObjectReaderImplValueInt Maven / Gradle / Ivy
package com.alibaba.fastjson2.reader;
import com.alibaba.fastjson2.JSONException;
import com.alibaba.fastjson2.JSONReader;
import com.alibaba.fastjson2.schema.JSONSchema;
import java.lang.reflect.Type;
import java.util.function.IntFunction;
public class ObjectReaderImplValueInt
implements ObjectReader {
final long features;
final IntFunction function;
final JSONSchema schema;
public ObjectReaderImplValueInt(
Class objectClass,
long features,
JSONSchema schema,
IntFunction function
) {
this.features = features;
this.schema = schema;
this.function = function;
}
@Override
public T readJSONBObject(JSONReader jsonReader, Type fieldType, Object fieldName, long features) {
return readObject(jsonReader, fieldType, fieldName, features);
}
@Override
public T readObject(JSONReader jsonReader, Type fieldType, Object fieldName, long features) {
if (jsonReader.nextIfNullOrEmptyString()) {
return null;
}
int value = jsonReader.readInt32Value();
if (schema != null) {
schema.validate(value);
}
T object;
try {
object = function.apply(value);
} catch (Exception ex) {
throw new JSONException(jsonReader.info("create object error"), ex);
}
return object;
}
public static ObjectReaderImplValueInt of(
Class objectClass,
IntFunction function
) {
return new ObjectReaderImplValueInt(
objectClass,
0L,
null,
function
);
}
public static ObjectReaderImplValueInt of(
Class objectClass,
long features,
JSONSchema schema,
IntFunction function
) {
return new ObjectReaderImplValueInt(
objectClass,
features,
schema,
function
);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy