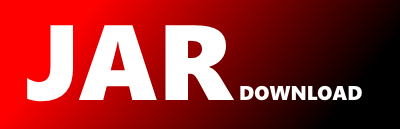
com.diozero.remote.message.protobuf.Gpio Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// NO CHECKED-IN PROTOBUF GENCODE
// source: diozero.proto
// Protobuf Java Version: 4.28.2
package com.diozero.remote.message.protobuf;
/**
* Protobuf type {@code diozero.Gpio}
*/
public final class Gpio extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:diozero.Gpio)
GpioOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
Gpio.class.getName());
}
// Use Gpio.newBuilder() to construct.
private Gpio(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Gpio() {
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.Gpio.class, com.diozero.remote.message.protobuf.Gpio.Builder.class);
}
/**
* Protobuf enum {@code diozero.Gpio.PullUpDown}
*/
public enum PullUpDown
implements com.google.protobuf.ProtocolMessageEnum {
/**
* PUD_NONE = 0;
*/
PUD_NONE(0),
/**
* PUD_PULL_UP = 1;
*/
PUD_PULL_UP(1),
/**
* PUD_PULL_DOWN = 2;
*/
PUD_PULL_DOWN(2),
UNRECOGNIZED(-1),
;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
PullUpDown.class.getName());
}
/**
* PUD_NONE = 0;
*/
public static final int PUD_NONE_VALUE = 0;
/**
* PUD_PULL_UP = 1;
*/
public static final int PUD_PULL_UP_VALUE = 1;
/**
* PUD_PULL_DOWN = 2;
*/
public static final int PUD_PULL_DOWN_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static PullUpDown valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static PullUpDown forNumber(int value) {
switch (value) {
case 0: return PUD_NONE;
case 1: return PUD_PULL_UP;
case 2: return PUD_PULL_DOWN;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
PullUpDown> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public PullUpDown findValueByNumber(int number) {
return PullUpDown.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Gpio.getDescriptor().getEnumTypes().get(0);
}
private static final PullUpDown[] VALUES = values();
public static PullUpDown valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private PullUpDown(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:diozero.Gpio.PullUpDown)
}
/**
* Protobuf enum {@code diozero.Gpio.Trigger}
*/
public enum Trigger
implements com.google.protobuf.ProtocolMessageEnum {
/**
* TRIGGER_NONE = 0;
*/
TRIGGER_NONE(0),
/**
* TRIGGER_RISING = 1;
*/
TRIGGER_RISING(1),
/**
* TRIGGER_FALLING = 2;
*/
TRIGGER_FALLING(2),
/**
* TRIGGER_BOTH = 3;
*/
TRIGGER_BOTH(3),
UNRECOGNIZED(-1),
;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
Trigger.class.getName());
}
/**
* TRIGGER_NONE = 0;
*/
public static final int TRIGGER_NONE_VALUE = 0;
/**
* TRIGGER_RISING = 1;
*/
public static final int TRIGGER_RISING_VALUE = 1;
/**
* TRIGGER_FALLING = 2;
*/
public static final int TRIGGER_FALLING_VALUE = 2;
/**
* TRIGGER_BOTH = 3;
*/
public static final int TRIGGER_BOTH_VALUE = 3;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Trigger valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Trigger forNumber(int value) {
switch (value) {
case 0: return TRIGGER_NONE;
case 1: return TRIGGER_RISING;
case 2: return TRIGGER_FALLING;
case 3: return TRIGGER_BOTH;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Trigger> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Trigger findValueByNumber(int number) {
return Trigger.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Gpio.getDescriptor().getEnumTypes().get(1);
}
private static final Trigger[] VALUES = values();
public static Trigger valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Trigger(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:diozero.Gpio.Trigger)
}
public interface IdentifierOrBuilder extends
// @@protoc_insertion_point(interface_extends:diozero.Gpio.Identifier)
com.google.protobuf.MessageOrBuilder {
/**
* int32 gpio = 1;
* @return The gpio.
*/
int getGpio();
}
/**
* Protobuf type {@code diozero.Gpio.Identifier}
*/
public static final class Identifier extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:diozero.Gpio.Identifier)
IdentifierOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
Identifier.class.getName());
}
// Use Identifier.newBuilder() to construct.
private Identifier(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Identifier() {
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_Identifier_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_Identifier_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.Gpio.Identifier.class, com.diozero.remote.message.protobuf.Gpio.Identifier.Builder.class);
}
public static final int GPIO_FIELD_NUMBER = 1;
private int gpio_ = 0;
/**
* int32 gpio = 1;
* @return The gpio.
*/
@java.lang.Override
public int getGpio() {
return gpio_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (gpio_ != 0) {
output.writeInt32(1, gpio_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (gpio_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, gpio_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.diozero.remote.message.protobuf.Gpio.Identifier)) {
return super.equals(obj);
}
com.diozero.remote.message.protobuf.Gpio.Identifier other = (com.diozero.remote.message.protobuf.Gpio.Identifier) obj;
if (getGpio()
!= other.getGpio()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + GPIO_FIELD_NUMBER;
hash = (53 * hash) + getGpio();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.diozero.remote.message.protobuf.Gpio.Identifier parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.Identifier parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.Identifier parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.Identifier parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.Identifier parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.Identifier parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.Identifier parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.Identifier parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.Identifier parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.Identifier parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.Identifier parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.Identifier parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.diozero.remote.message.protobuf.Gpio.Identifier prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code diozero.Gpio.Identifier}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:diozero.Gpio.Identifier)
com.diozero.remote.message.protobuf.Gpio.IdentifierOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_Identifier_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_Identifier_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.Gpio.Identifier.class, com.diozero.remote.message.protobuf.Gpio.Identifier.Builder.class);
}
// Construct using com.diozero.remote.message.protobuf.Gpio.Identifier.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
gpio_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_Identifier_descriptor;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.Identifier getDefaultInstanceForType() {
return com.diozero.remote.message.protobuf.Gpio.Identifier.getDefaultInstance();
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.Identifier build() {
com.diozero.remote.message.protobuf.Gpio.Identifier result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.Identifier buildPartial() {
com.diozero.remote.message.protobuf.Gpio.Identifier result = new com.diozero.remote.message.protobuf.Gpio.Identifier(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.diozero.remote.message.protobuf.Gpio.Identifier result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.gpio_ = gpio_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.diozero.remote.message.protobuf.Gpio.Identifier) {
return mergeFrom((com.diozero.remote.message.protobuf.Gpio.Identifier)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.diozero.remote.message.protobuf.Gpio.Identifier other) {
if (other == com.diozero.remote.message.protobuf.Gpio.Identifier.getDefaultInstance()) return this;
if (other.getGpio() != 0) {
setGpio(other.getGpio());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
gpio_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int gpio_ ;
/**
* int32 gpio = 1;
* @return The gpio.
*/
@java.lang.Override
public int getGpio() {
return gpio_;
}
/**
* int32 gpio = 1;
* @param value The gpio to set.
* @return This builder for chaining.
*/
public Builder setGpio(int value) {
gpio_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* int32 gpio = 1;
* @return This builder for chaining.
*/
public Builder clearGpio() {
bitField0_ = (bitField0_ & ~0x00000001);
gpio_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:diozero.Gpio.Identifier)
}
// @@protoc_insertion_point(class_scope:diozero.Gpio.Identifier)
private static final com.diozero.remote.message.protobuf.Gpio.Identifier DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.diozero.remote.message.protobuf.Gpio.Identifier();
}
public static com.diozero.remote.message.protobuf.Gpio.Identifier getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Identifier parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.Identifier getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ProvisionDigitalInputDeviceRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:diozero.Gpio.ProvisionDigitalInputDeviceRequest)
com.google.protobuf.MessageOrBuilder {
/**
* int32 gpio = 1;
* @return The gpio.
*/
int getGpio();
/**
* .diozero.Gpio.PullUpDown pud = 2;
* @return The enum numeric value on the wire for pud.
*/
int getPudValue();
/**
* .diozero.Gpio.PullUpDown pud = 2;
* @return The pud.
*/
com.diozero.remote.message.protobuf.Gpio.PullUpDown getPud();
/**
* .diozero.Gpio.Trigger trigger = 3;
* @return The enum numeric value on the wire for trigger.
*/
int getTriggerValue();
/**
* .diozero.Gpio.Trigger trigger = 3;
* @return The trigger.
*/
com.diozero.remote.message.protobuf.Gpio.Trigger getTrigger();
}
/**
* Protobuf type {@code diozero.Gpio.ProvisionDigitalInputDeviceRequest}
*/
public static final class ProvisionDigitalInputDeviceRequest extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:diozero.Gpio.ProvisionDigitalInputDeviceRequest)
ProvisionDigitalInputDeviceRequestOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
ProvisionDigitalInputDeviceRequest.class.getName());
}
// Use ProvisionDigitalInputDeviceRequest.newBuilder() to construct.
private ProvisionDigitalInputDeviceRequest(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private ProvisionDigitalInputDeviceRequest() {
pud_ = 0;
trigger_ = 0;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_ProvisionDigitalInputDeviceRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_ProvisionDigitalInputDeviceRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest.class, com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest.Builder.class);
}
public static final int GPIO_FIELD_NUMBER = 1;
private int gpio_ = 0;
/**
* int32 gpio = 1;
* @return The gpio.
*/
@java.lang.Override
public int getGpio() {
return gpio_;
}
public static final int PUD_FIELD_NUMBER = 2;
private int pud_ = 0;
/**
* .diozero.Gpio.PullUpDown pud = 2;
* @return The enum numeric value on the wire for pud.
*/
@java.lang.Override public int getPudValue() {
return pud_;
}
/**
* .diozero.Gpio.PullUpDown pud = 2;
* @return The pud.
*/
@java.lang.Override public com.diozero.remote.message.protobuf.Gpio.PullUpDown getPud() {
com.diozero.remote.message.protobuf.Gpio.PullUpDown result = com.diozero.remote.message.protobuf.Gpio.PullUpDown.forNumber(pud_);
return result == null ? com.diozero.remote.message.protobuf.Gpio.PullUpDown.UNRECOGNIZED : result;
}
public static final int TRIGGER_FIELD_NUMBER = 3;
private int trigger_ = 0;
/**
* .diozero.Gpio.Trigger trigger = 3;
* @return The enum numeric value on the wire for trigger.
*/
@java.lang.Override public int getTriggerValue() {
return trigger_;
}
/**
* .diozero.Gpio.Trigger trigger = 3;
* @return The trigger.
*/
@java.lang.Override public com.diozero.remote.message.protobuf.Gpio.Trigger getTrigger() {
com.diozero.remote.message.protobuf.Gpio.Trigger result = com.diozero.remote.message.protobuf.Gpio.Trigger.forNumber(trigger_);
return result == null ? com.diozero.remote.message.protobuf.Gpio.Trigger.UNRECOGNIZED : result;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (gpio_ != 0) {
output.writeInt32(1, gpio_);
}
if (pud_ != com.diozero.remote.message.protobuf.Gpio.PullUpDown.PUD_NONE.getNumber()) {
output.writeEnum(2, pud_);
}
if (trigger_ != com.diozero.remote.message.protobuf.Gpio.Trigger.TRIGGER_NONE.getNumber()) {
output.writeEnum(3, trigger_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (gpio_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, gpio_);
}
if (pud_ != com.diozero.remote.message.protobuf.Gpio.PullUpDown.PUD_NONE.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, pud_);
}
if (trigger_ != com.diozero.remote.message.protobuf.Gpio.Trigger.TRIGGER_NONE.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, trigger_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest)) {
return super.equals(obj);
}
com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest other = (com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest) obj;
if (getGpio()
!= other.getGpio()) return false;
if (pud_ != other.pud_) return false;
if (trigger_ != other.trigger_) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + GPIO_FIELD_NUMBER;
hash = (53 * hash) + getGpio();
hash = (37 * hash) + PUD_FIELD_NUMBER;
hash = (53 * hash) + pud_;
hash = (37 * hash) + TRIGGER_FIELD_NUMBER;
hash = (53 * hash) + trigger_;
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code diozero.Gpio.ProvisionDigitalInputDeviceRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:diozero.Gpio.ProvisionDigitalInputDeviceRequest)
com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_ProvisionDigitalInputDeviceRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_ProvisionDigitalInputDeviceRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest.class, com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest.Builder.class);
}
// Construct using com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
gpio_ = 0;
pud_ = 0;
trigger_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_ProvisionDigitalInputDeviceRequest_descriptor;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest getDefaultInstanceForType() {
return com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest.getDefaultInstance();
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest build() {
com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest buildPartial() {
com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest result = new com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.gpio_ = gpio_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.pud_ = pud_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.trigger_ = trigger_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest) {
return mergeFrom((com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest other) {
if (other == com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest.getDefaultInstance()) return this;
if (other.getGpio() != 0) {
setGpio(other.getGpio());
}
if (other.pud_ != 0) {
setPudValue(other.getPudValue());
}
if (other.trigger_ != 0) {
setTriggerValue(other.getTriggerValue());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
gpio_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
pud_ = input.readEnum();
bitField0_ |= 0x00000002;
break;
} // case 16
case 24: {
trigger_ = input.readEnum();
bitField0_ |= 0x00000004;
break;
} // case 24
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int gpio_ ;
/**
* int32 gpio = 1;
* @return The gpio.
*/
@java.lang.Override
public int getGpio() {
return gpio_;
}
/**
* int32 gpio = 1;
* @param value The gpio to set.
* @return This builder for chaining.
*/
public Builder setGpio(int value) {
gpio_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* int32 gpio = 1;
* @return This builder for chaining.
*/
public Builder clearGpio() {
bitField0_ = (bitField0_ & ~0x00000001);
gpio_ = 0;
onChanged();
return this;
}
private int pud_ = 0;
/**
* .diozero.Gpio.PullUpDown pud = 2;
* @return The enum numeric value on the wire for pud.
*/
@java.lang.Override public int getPudValue() {
return pud_;
}
/**
* .diozero.Gpio.PullUpDown pud = 2;
* @param value The enum numeric value on the wire for pud to set.
* @return This builder for chaining.
*/
public Builder setPudValue(int value) {
pud_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* .diozero.Gpio.PullUpDown pud = 2;
* @return The pud.
*/
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.PullUpDown getPud() {
com.diozero.remote.message.protobuf.Gpio.PullUpDown result = com.diozero.remote.message.protobuf.Gpio.PullUpDown.forNumber(pud_);
return result == null ? com.diozero.remote.message.protobuf.Gpio.PullUpDown.UNRECOGNIZED : result;
}
/**
* .diozero.Gpio.PullUpDown pud = 2;
* @param value The pud to set.
* @return This builder for chaining.
*/
public Builder setPud(com.diozero.remote.message.protobuf.Gpio.PullUpDown value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
pud_ = value.getNumber();
onChanged();
return this;
}
/**
* .diozero.Gpio.PullUpDown pud = 2;
* @return This builder for chaining.
*/
public Builder clearPud() {
bitField0_ = (bitField0_ & ~0x00000002);
pud_ = 0;
onChanged();
return this;
}
private int trigger_ = 0;
/**
* .diozero.Gpio.Trigger trigger = 3;
* @return The enum numeric value on the wire for trigger.
*/
@java.lang.Override public int getTriggerValue() {
return trigger_;
}
/**
* .diozero.Gpio.Trigger trigger = 3;
* @param value The enum numeric value on the wire for trigger to set.
* @return This builder for chaining.
*/
public Builder setTriggerValue(int value) {
trigger_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* .diozero.Gpio.Trigger trigger = 3;
* @return The trigger.
*/
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.Trigger getTrigger() {
com.diozero.remote.message.protobuf.Gpio.Trigger result = com.diozero.remote.message.protobuf.Gpio.Trigger.forNumber(trigger_);
return result == null ? com.diozero.remote.message.protobuf.Gpio.Trigger.UNRECOGNIZED : result;
}
/**
* .diozero.Gpio.Trigger trigger = 3;
* @param value The trigger to set.
* @return This builder for chaining.
*/
public Builder setTrigger(com.diozero.remote.message.protobuf.Gpio.Trigger value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
trigger_ = value.getNumber();
onChanged();
return this;
}
/**
* .diozero.Gpio.Trigger trigger = 3;
* @return This builder for chaining.
*/
public Builder clearTrigger() {
bitField0_ = (bitField0_ & ~0x00000004);
trigger_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:diozero.Gpio.ProvisionDigitalInputDeviceRequest)
}
// @@protoc_insertion_point(class_scope:diozero.Gpio.ProvisionDigitalInputDeviceRequest)
private static final com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest();
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ProvisionDigitalInputDeviceRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ProvisionDigitalOutputDeviceRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:diozero.Gpio.ProvisionDigitalOutputDeviceRequest)
com.google.protobuf.MessageOrBuilder {
/**
* int32 gpio = 1;
* @return The gpio.
*/
int getGpio();
/**
* bool initialValue = 2;
* @return The initialValue.
*/
boolean getInitialValue();
}
/**
* Protobuf type {@code diozero.Gpio.ProvisionDigitalOutputDeviceRequest}
*/
public static final class ProvisionDigitalOutputDeviceRequest extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:diozero.Gpio.ProvisionDigitalOutputDeviceRequest)
ProvisionDigitalOutputDeviceRequestOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
ProvisionDigitalOutputDeviceRequest.class.getName());
}
// Use ProvisionDigitalOutputDeviceRequest.newBuilder() to construct.
private ProvisionDigitalOutputDeviceRequest(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private ProvisionDigitalOutputDeviceRequest() {
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_ProvisionDigitalOutputDeviceRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_ProvisionDigitalOutputDeviceRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest.class, com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest.Builder.class);
}
public static final int GPIO_FIELD_NUMBER = 1;
private int gpio_ = 0;
/**
* int32 gpio = 1;
* @return The gpio.
*/
@java.lang.Override
public int getGpio() {
return gpio_;
}
public static final int INITIALVALUE_FIELD_NUMBER = 2;
private boolean initialValue_ = false;
/**
* bool initialValue = 2;
* @return The initialValue.
*/
@java.lang.Override
public boolean getInitialValue() {
return initialValue_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (gpio_ != 0) {
output.writeInt32(1, gpio_);
}
if (initialValue_ != false) {
output.writeBool(2, initialValue_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (gpio_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, gpio_);
}
if (initialValue_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, initialValue_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest)) {
return super.equals(obj);
}
com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest other = (com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest) obj;
if (getGpio()
!= other.getGpio()) return false;
if (getInitialValue()
!= other.getInitialValue()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + GPIO_FIELD_NUMBER;
hash = (53 * hash) + getGpio();
hash = (37 * hash) + INITIALVALUE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getInitialValue());
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code diozero.Gpio.ProvisionDigitalOutputDeviceRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:diozero.Gpio.ProvisionDigitalOutputDeviceRequest)
com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_ProvisionDigitalOutputDeviceRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_ProvisionDigitalOutputDeviceRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest.class, com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest.Builder.class);
}
// Construct using com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
gpio_ = 0;
initialValue_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_ProvisionDigitalOutputDeviceRequest_descriptor;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest getDefaultInstanceForType() {
return com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest.getDefaultInstance();
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest build() {
com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest buildPartial() {
com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest result = new com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.gpio_ = gpio_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.initialValue_ = initialValue_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest) {
return mergeFrom((com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest other) {
if (other == com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest.getDefaultInstance()) return this;
if (other.getGpio() != 0) {
setGpio(other.getGpio());
}
if (other.getInitialValue() != false) {
setInitialValue(other.getInitialValue());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
gpio_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
initialValue_ = input.readBool();
bitField0_ |= 0x00000002;
break;
} // case 16
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int gpio_ ;
/**
* int32 gpio = 1;
* @return The gpio.
*/
@java.lang.Override
public int getGpio() {
return gpio_;
}
/**
* int32 gpio = 1;
* @param value The gpio to set.
* @return This builder for chaining.
*/
public Builder setGpio(int value) {
gpio_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* int32 gpio = 1;
* @return This builder for chaining.
*/
public Builder clearGpio() {
bitField0_ = (bitField0_ & ~0x00000001);
gpio_ = 0;
onChanged();
return this;
}
private boolean initialValue_ ;
/**
* bool initialValue = 2;
* @return The initialValue.
*/
@java.lang.Override
public boolean getInitialValue() {
return initialValue_;
}
/**
* bool initialValue = 2;
* @param value The initialValue to set.
* @return This builder for chaining.
*/
public Builder setInitialValue(boolean value) {
initialValue_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* bool initialValue = 2;
* @return This builder for chaining.
*/
public Builder clearInitialValue() {
bitField0_ = (bitField0_ & ~0x00000002);
initialValue_ = false;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:diozero.Gpio.ProvisionDigitalOutputDeviceRequest)
}
// @@protoc_insertion_point(class_scope:diozero.Gpio.ProvisionDigitalOutputDeviceRequest)
private static final com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest();
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ProvisionDigitalOutputDeviceRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ProvisionDigitalInputOutputDeviceRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:diozero.Gpio.ProvisionDigitalInputOutputDeviceRequest)
com.google.protobuf.MessageOrBuilder {
/**
* int32 gpio = 1;
* @return The gpio.
*/
int getGpio();
/**
* bool output = 2;
* @return The output.
*/
boolean getOutput();
}
/**
* Protobuf type {@code diozero.Gpio.ProvisionDigitalInputOutputDeviceRequest}
*/
public static final class ProvisionDigitalInputOutputDeviceRequest extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:diozero.Gpio.ProvisionDigitalInputOutputDeviceRequest)
ProvisionDigitalInputOutputDeviceRequestOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
ProvisionDigitalInputOutputDeviceRequest.class.getName());
}
// Use ProvisionDigitalInputOutputDeviceRequest.newBuilder() to construct.
private ProvisionDigitalInputOutputDeviceRequest(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private ProvisionDigitalInputOutputDeviceRequest() {
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_ProvisionDigitalInputOutputDeviceRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_ProvisionDigitalInputOutputDeviceRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest.class, com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest.Builder.class);
}
public static final int GPIO_FIELD_NUMBER = 1;
private int gpio_ = 0;
/**
* int32 gpio = 1;
* @return The gpio.
*/
@java.lang.Override
public int getGpio() {
return gpio_;
}
public static final int OUTPUT_FIELD_NUMBER = 2;
private boolean output_ = false;
/**
* bool output = 2;
* @return The output.
*/
@java.lang.Override
public boolean getOutput() {
return output_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (gpio_ != 0) {
output.writeInt32(1, gpio_);
}
if (output_ != false) {
output.writeBool(2, output_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (gpio_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, gpio_);
}
if (output_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, output_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest)) {
return super.equals(obj);
}
com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest other = (com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest) obj;
if (getGpio()
!= other.getGpio()) return false;
if (getOutput()
!= other.getOutput()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + GPIO_FIELD_NUMBER;
hash = (53 * hash) + getGpio();
hash = (37 * hash) + OUTPUT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getOutput());
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code diozero.Gpio.ProvisionDigitalInputOutputDeviceRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:diozero.Gpio.ProvisionDigitalInputOutputDeviceRequest)
com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_ProvisionDigitalInputOutputDeviceRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_ProvisionDigitalInputOutputDeviceRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest.class, com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest.Builder.class);
}
// Construct using com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
gpio_ = 0;
output_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_ProvisionDigitalInputOutputDeviceRequest_descriptor;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest getDefaultInstanceForType() {
return com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest.getDefaultInstance();
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest build() {
com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest buildPartial() {
com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest result = new com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.gpio_ = gpio_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.output_ = output_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest) {
return mergeFrom((com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest other) {
if (other == com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest.getDefaultInstance()) return this;
if (other.getGpio() != 0) {
setGpio(other.getGpio());
}
if (other.getOutput() != false) {
setOutput(other.getOutput());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
gpio_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
output_ = input.readBool();
bitField0_ |= 0x00000002;
break;
} // case 16
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int gpio_ ;
/**
* int32 gpio = 1;
* @return The gpio.
*/
@java.lang.Override
public int getGpio() {
return gpio_;
}
/**
* int32 gpio = 1;
* @param value The gpio to set.
* @return This builder for chaining.
*/
public Builder setGpio(int value) {
gpio_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* int32 gpio = 1;
* @return This builder for chaining.
*/
public Builder clearGpio() {
bitField0_ = (bitField0_ & ~0x00000001);
gpio_ = 0;
onChanged();
return this;
}
private boolean output_ ;
/**
* bool output = 2;
* @return The output.
*/
@java.lang.Override
public boolean getOutput() {
return output_;
}
/**
* bool output = 2;
* @param value The output to set.
* @return This builder for chaining.
*/
public Builder setOutput(boolean value) {
output_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* bool output = 2;
* @return This builder for chaining.
*/
public Builder clearOutput() {
bitField0_ = (bitField0_ & ~0x00000002);
output_ = false;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:diozero.Gpio.ProvisionDigitalInputOutputDeviceRequest)
}
// @@protoc_insertion_point(class_scope:diozero.Gpio.ProvisionDigitalInputOutputDeviceRequest)
private static final com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest();
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ProvisionDigitalInputOutputDeviceRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ProvisionPwmOutputDeviceRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:diozero.Gpio.ProvisionPwmOutputDeviceRequest)
com.google.protobuf.MessageOrBuilder {
/**
* int32 gpio = 1;
* @return The gpio.
*/
int getGpio();
/**
* int32 frequency = 2;
* @return The frequency.
*/
int getFrequency();
/**
* float initialValue = 3;
* @return The initialValue.
*/
float getInitialValue();
}
/**
* Protobuf type {@code diozero.Gpio.ProvisionPwmOutputDeviceRequest}
*/
public static final class ProvisionPwmOutputDeviceRequest extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:diozero.Gpio.ProvisionPwmOutputDeviceRequest)
ProvisionPwmOutputDeviceRequestOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
ProvisionPwmOutputDeviceRequest.class.getName());
}
// Use ProvisionPwmOutputDeviceRequest.newBuilder() to construct.
private ProvisionPwmOutputDeviceRequest(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private ProvisionPwmOutputDeviceRequest() {
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_ProvisionPwmOutputDeviceRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_ProvisionPwmOutputDeviceRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest.class, com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest.Builder.class);
}
public static final int GPIO_FIELD_NUMBER = 1;
private int gpio_ = 0;
/**
* int32 gpio = 1;
* @return The gpio.
*/
@java.lang.Override
public int getGpio() {
return gpio_;
}
public static final int FREQUENCY_FIELD_NUMBER = 2;
private int frequency_ = 0;
/**
* int32 frequency = 2;
* @return The frequency.
*/
@java.lang.Override
public int getFrequency() {
return frequency_;
}
public static final int INITIALVALUE_FIELD_NUMBER = 3;
private float initialValue_ = 0F;
/**
* float initialValue = 3;
* @return The initialValue.
*/
@java.lang.Override
public float getInitialValue() {
return initialValue_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (gpio_ != 0) {
output.writeInt32(1, gpio_);
}
if (frequency_ != 0) {
output.writeInt32(2, frequency_);
}
if (java.lang.Float.floatToRawIntBits(initialValue_) != 0) {
output.writeFloat(3, initialValue_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (gpio_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, gpio_);
}
if (frequency_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, frequency_);
}
if (java.lang.Float.floatToRawIntBits(initialValue_) != 0) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(3, initialValue_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest)) {
return super.equals(obj);
}
com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest other = (com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest) obj;
if (getGpio()
!= other.getGpio()) return false;
if (getFrequency()
!= other.getFrequency()) return false;
if (java.lang.Float.floatToIntBits(getInitialValue())
!= java.lang.Float.floatToIntBits(
other.getInitialValue())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + GPIO_FIELD_NUMBER;
hash = (53 * hash) + getGpio();
hash = (37 * hash) + FREQUENCY_FIELD_NUMBER;
hash = (53 * hash) + getFrequency();
hash = (37 * hash) + INITIALVALUE_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getInitialValue());
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code diozero.Gpio.ProvisionPwmOutputDeviceRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:diozero.Gpio.ProvisionPwmOutputDeviceRequest)
com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_ProvisionPwmOutputDeviceRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_ProvisionPwmOutputDeviceRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest.class, com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest.Builder.class);
}
// Construct using com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
gpio_ = 0;
frequency_ = 0;
initialValue_ = 0F;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_ProvisionPwmOutputDeviceRequest_descriptor;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest getDefaultInstanceForType() {
return com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest.getDefaultInstance();
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest build() {
com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest buildPartial() {
com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest result = new com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.gpio_ = gpio_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.frequency_ = frequency_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.initialValue_ = initialValue_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest) {
return mergeFrom((com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest other) {
if (other == com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest.getDefaultInstance()) return this;
if (other.getGpio() != 0) {
setGpio(other.getGpio());
}
if (other.getFrequency() != 0) {
setFrequency(other.getFrequency());
}
if (other.getInitialValue() != 0F) {
setInitialValue(other.getInitialValue());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
gpio_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
frequency_ = input.readInt32();
bitField0_ |= 0x00000002;
break;
} // case 16
case 29: {
initialValue_ = input.readFloat();
bitField0_ |= 0x00000004;
break;
} // case 29
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int gpio_ ;
/**
* int32 gpio = 1;
* @return The gpio.
*/
@java.lang.Override
public int getGpio() {
return gpio_;
}
/**
* int32 gpio = 1;
* @param value The gpio to set.
* @return This builder for chaining.
*/
public Builder setGpio(int value) {
gpio_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* int32 gpio = 1;
* @return This builder for chaining.
*/
public Builder clearGpio() {
bitField0_ = (bitField0_ & ~0x00000001);
gpio_ = 0;
onChanged();
return this;
}
private int frequency_ ;
/**
* int32 frequency = 2;
* @return The frequency.
*/
@java.lang.Override
public int getFrequency() {
return frequency_;
}
/**
* int32 frequency = 2;
* @param value The frequency to set.
* @return This builder for chaining.
*/
public Builder setFrequency(int value) {
frequency_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* int32 frequency = 2;
* @return This builder for chaining.
*/
public Builder clearFrequency() {
bitField0_ = (bitField0_ & ~0x00000002);
frequency_ = 0;
onChanged();
return this;
}
private float initialValue_ ;
/**
* float initialValue = 3;
* @return The initialValue.
*/
@java.lang.Override
public float getInitialValue() {
return initialValue_;
}
/**
* float initialValue = 3;
* @param value The initialValue to set.
* @return This builder for chaining.
*/
public Builder setInitialValue(float value) {
initialValue_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* float initialValue = 3;
* @return This builder for chaining.
*/
public Builder clearInitialValue() {
bitField0_ = (bitField0_ & ~0x00000004);
initialValue_ = 0F;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:diozero.Gpio.ProvisionPwmOutputDeviceRequest)
}
// @@protoc_insertion_point(class_scope:diozero.Gpio.ProvisionPwmOutputDeviceRequest)
private static final com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest();
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ProvisionPwmOutputDeviceRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ProvisionServoDeviceRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:diozero.Gpio.ProvisionServoDeviceRequest)
com.google.protobuf.MessageOrBuilder {
/**
* int32 gpio = 1;
* @return The gpio.
*/
int getGpio();
/**
* int32 frequency = 2;
* @return The frequency.
*/
int getFrequency();
/**
* int32 minPulseWidthUs = 3;
* @return The minPulseWidthUs.
*/
int getMinPulseWidthUs();
/**
* int32 maxPulseWidthUs = 4;
* @return The maxPulseWidthUs.
*/
int getMaxPulseWidthUs();
/**
* int32 initialPulseWidthUs = 5;
* @return The initialPulseWidthUs.
*/
int getInitialPulseWidthUs();
}
/**
* Protobuf type {@code diozero.Gpio.ProvisionServoDeviceRequest}
*/
public static final class ProvisionServoDeviceRequest extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:diozero.Gpio.ProvisionServoDeviceRequest)
ProvisionServoDeviceRequestOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
ProvisionServoDeviceRequest.class.getName());
}
// Use ProvisionServoDeviceRequest.newBuilder() to construct.
private ProvisionServoDeviceRequest(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private ProvisionServoDeviceRequest() {
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_ProvisionServoDeviceRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_ProvisionServoDeviceRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest.class, com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest.Builder.class);
}
public static final int GPIO_FIELD_NUMBER = 1;
private int gpio_ = 0;
/**
* int32 gpio = 1;
* @return The gpio.
*/
@java.lang.Override
public int getGpio() {
return gpio_;
}
public static final int FREQUENCY_FIELD_NUMBER = 2;
private int frequency_ = 0;
/**
* int32 frequency = 2;
* @return The frequency.
*/
@java.lang.Override
public int getFrequency() {
return frequency_;
}
public static final int MINPULSEWIDTHUS_FIELD_NUMBER = 3;
private int minPulseWidthUs_ = 0;
/**
* int32 minPulseWidthUs = 3;
* @return The minPulseWidthUs.
*/
@java.lang.Override
public int getMinPulseWidthUs() {
return minPulseWidthUs_;
}
public static final int MAXPULSEWIDTHUS_FIELD_NUMBER = 4;
private int maxPulseWidthUs_ = 0;
/**
* int32 maxPulseWidthUs = 4;
* @return The maxPulseWidthUs.
*/
@java.lang.Override
public int getMaxPulseWidthUs() {
return maxPulseWidthUs_;
}
public static final int INITIALPULSEWIDTHUS_FIELD_NUMBER = 5;
private int initialPulseWidthUs_ = 0;
/**
* int32 initialPulseWidthUs = 5;
* @return The initialPulseWidthUs.
*/
@java.lang.Override
public int getInitialPulseWidthUs() {
return initialPulseWidthUs_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (gpio_ != 0) {
output.writeInt32(1, gpio_);
}
if (frequency_ != 0) {
output.writeInt32(2, frequency_);
}
if (minPulseWidthUs_ != 0) {
output.writeInt32(3, minPulseWidthUs_);
}
if (maxPulseWidthUs_ != 0) {
output.writeInt32(4, maxPulseWidthUs_);
}
if (initialPulseWidthUs_ != 0) {
output.writeInt32(5, initialPulseWidthUs_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (gpio_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, gpio_);
}
if (frequency_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, frequency_);
}
if (minPulseWidthUs_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, minPulseWidthUs_);
}
if (maxPulseWidthUs_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(4, maxPulseWidthUs_);
}
if (initialPulseWidthUs_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(5, initialPulseWidthUs_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest)) {
return super.equals(obj);
}
com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest other = (com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest) obj;
if (getGpio()
!= other.getGpio()) return false;
if (getFrequency()
!= other.getFrequency()) return false;
if (getMinPulseWidthUs()
!= other.getMinPulseWidthUs()) return false;
if (getMaxPulseWidthUs()
!= other.getMaxPulseWidthUs()) return false;
if (getInitialPulseWidthUs()
!= other.getInitialPulseWidthUs()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + GPIO_FIELD_NUMBER;
hash = (53 * hash) + getGpio();
hash = (37 * hash) + FREQUENCY_FIELD_NUMBER;
hash = (53 * hash) + getFrequency();
hash = (37 * hash) + MINPULSEWIDTHUS_FIELD_NUMBER;
hash = (53 * hash) + getMinPulseWidthUs();
hash = (37 * hash) + MAXPULSEWIDTHUS_FIELD_NUMBER;
hash = (53 * hash) + getMaxPulseWidthUs();
hash = (37 * hash) + INITIALPULSEWIDTHUS_FIELD_NUMBER;
hash = (53 * hash) + getInitialPulseWidthUs();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code diozero.Gpio.ProvisionServoDeviceRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:diozero.Gpio.ProvisionServoDeviceRequest)
com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_ProvisionServoDeviceRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_ProvisionServoDeviceRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest.class, com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest.Builder.class);
}
// Construct using com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
gpio_ = 0;
frequency_ = 0;
minPulseWidthUs_ = 0;
maxPulseWidthUs_ = 0;
initialPulseWidthUs_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_ProvisionServoDeviceRequest_descriptor;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest getDefaultInstanceForType() {
return com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest.getDefaultInstance();
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest build() {
com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest buildPartial() {
com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest result = new com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.gpio_ = gpio_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.frequency_ = frequency_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.minPulseWidthUs_ = minPulseWidthUs_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.maxPulseWidthUs_ = maxPulseWidthUs_;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.initialPulseWidthUs_ = initialPulseWidthUs_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest) {
return mergeFrom((com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest other) {
if (other == com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest.getDefaultInstance()) return this;
if (other.getGpio() != 0) {
setGpio(other.getGpio());
}
if (other.getFrequency() != 0) {
setFrequency(other.getFrequency());
}
if (other.getMinPulseWidthUs() != 0) {
setMinPulseWidthUs(other.getMinPulseWidthUs());
}
if (other.getMaxPulseWidthUs() != 0) {
setMaxPulseWidthUs(other.getMaxPulseWidthUs());
}
if (other.getInitialPulseWidthUs() != 0) {
setInitialPulseWidthUs(other.getInitialPulseWidthUs());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
gpio_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
frequency_ = input.readInt32();
bitField0_ |= 0x00000002;
break;
} // case 16
case 24: {
minPulseWidthUs_ = input.readInt32();
bitField0_ |= 0x00000004;
break;
} // case 24
case 32: {
maxPulseWidthUs_ = input.readInt32();
bitField0_ |= 0x00000008;
break;
} // case 32
case 40: {
initialPulseWidthUs_ = input.readInt32();
bitField0_ |= 0x00000010;
break;
} // case 40
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int gpio_ ;
/**
* int32 gpio = 1;
* @return The gpio.
*/
@java.lang.Override
public int getGpio() {
return gpio_;
}
/**
* int32 gpio = 1;
* @param value The gpio to set.
* @return This builder for chaining.
*/
public Builder setGpio(int value) {
gpio_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* int32 gpio = 1;
* @return This builder for chaining.
*/
public Builder clearGpio() {
bitField0_ = (bitField0_ & ~0x00000001);
gpio_ = 0;
onChanged();
return this;
}
private int frequency_ ;
/**
* int32 frequency = 2;
* @return The frequency.
*/
@java.lang.Override
public int getFrequency() {
return frequency_;
}
/**
* int32 frequency = 2;
* @param value The frequency to set.
* @return This builder for chaining.
*/
public Builder setFrequency(int value) {
frequency_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* int32 frequency = 2;
* @return This builder for chaining.
*/
public Builder clearFrequency() {
bitField0_ = (bitField0_ & ~0x00000002);
frequency_ = 0;
onChanged();
return this;
}
private int minPulseWidthUs_ ;
/**
* int32 minPulseWidthUs = 3;
* @return The minPulseWidthUs.
*/
@java.lang.Override
public int getMinPulseWidthUs() {
return minPulseWidthUs_;
}
/**
* int32 minPulseWidthUs = 3;
* @param value The minPulseWidthUs to set.
* @return This builder for chaining.
*/
public Builder setMinPulseWidthUs(int value) {
minPulseWidthUs_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* int32 minPulseWidthUs = 3;
* @return This builder for chaining.
*/
public Builder clearMinPulseWidthUs() {
bitField0_ = (bitField0_ & ~0x00000004);
minPulseWidthUs_ = 0;
onChanged();
return this;
}
private int maxPulseWidthUs_ ;
/**
* int32 maxPulseWidthUs = 4;
* @return The maxPulseWidthUs.
*/
@java.lang.Override
public int getMaxPulseWidthUs() {
return maxPulseWidthUs_;
}
/**
* int32 maxPulseWidthUs = 4;
* @param value The maxPulseWidthUs to set.
* @return This builder for chaining.
*/
public Builder setMaxPulseWidthUs(int value) {
maxPulseWidthUs_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* int32 maxPulseWidthUs = 4;
* @return This builder for chaining.
*/
public Builder clearMaxPulseWidthUs() {
bitField0_ = (bitField0_ & ~0x00000008);
maxPulseWidthUs_ = 0;
onChanged();
return this;
}
private int initialPulseWidthUs_ ;
/**
* int32 initialPulseWidthUs = 5;
* @return The initialPulseWidthUs.
*/
@java.lang.Override
public int getInitialPulseWidthUs() {
return initialPulseWidthUs_;
}
/**
* int32 initialPulseWidthUs = 5;
* @param value The initialPulseWidthUs to set.
* @return This builder for chaining.
*/
public Builder setInitialPulseWidthUs(int value) {
initialPulseWidthUs_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
* int32 initialPulseWidthUs = 5;
* @return This builder for chaining.
*/
public Builder clearInitialPulseWidthUs() {
bitField0_ = (bitField0_ & ~0x00000010);
initialPulseWidthUs_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:diozero.Gpio.ProvisionServoDeviceRequest)
}
// @@protoc_insertion_point(class_scope:diozero.Gpio.ProvisionServoDeviceRequest)
private static final com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest();
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ProvisionServoDeviceRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ProvisionAnalogInputDeviceRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:diozero.Gpio.ProvisionAnalogInputDeviceRequest)
com.google.protobuf.MessageOrBuilder {
/**
* int32 gpio = 1;
* @return The gpio.
*/
int getGpio();
}
/**
* Protobuf type {@code diozero.Gpio.ProvisionAnalogInputDeviceRequest}
*/
public static final class ProvisionAnalogInputDeviceRequest extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:diozero.Gpio.ProvisionAnalogInputDeviceRequest)
ProvisionAnalogInputDeviceRequestOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
ProvisionAnalogInputDeviceRequest.class.getName());
}
// Use ProvisionAnalogInputDeviceRequest.newBuilder() to construct.
private ProvisionAnalogInputDeviceRequest(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private ProvisionAnalogInputDeviceRequest() {
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_ProvisionAnalogInputDeviceRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_ProvisionAnalogInputDeviceRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest.class, com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest.Builder.class);
}
public static final int GPIO_FIELD_NUMBER = 1;
private int gpio_ = 0;
/**
* int32 gpio = 1;
* @return The gpio.
*/
@java.lang.Override
public int getGpio() {
return gpio_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (gpio_ != 0) {
output.writeInt32(1, gpio_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (gpio_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, gpio_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest)) {
return super.equals(obj);
}
com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest other = (com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest) obj;
if (getGpio()
!= other.getGpio()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + GPIO_FIELD_NUMBER;
hash = (53 * hash) + getGpio();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code diozero.Gpio.ProvisionAnalogInputDeviceRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:diozero.Gpio.ProvisionAnalogInputDeviceRequest)
com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_ProvisionAnalogInputDeviceRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_ProvisionAnalogInputDeviceRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest.class, com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest.Builder.class);
}
// Construct using com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
gpio_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_ProvisionAnalogInputDeviceRequest_descriptor;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest getDefaultInstanceForType() {
return com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest.getDefaultInstance();
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest build() {
com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest buildPartial() {
com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest result = new com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.gpio_ = gpio_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest) {
return mergeFrom((com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest other) {
if (other == com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest.getDefaultInstance()) return this;
if (other.getGpio() != 0) {
setGpio(other.getGpio());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
gpio_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int gpio_ ;
/**
* int32 gpio = 1;
* @return The gpio.
*/
@java.lang.Override
public int getGpio() {
return gpio_;
}
/**
* int32 gpio = 1;
* @param value The gpio to set.
* @return This builder for chaining.
*/
public Builder setGpio(int value) {
gpio_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* int32 gpio = 1;
* @return This builder for chaining.
*/
public Builder clearGpio() {
bitField0_ = (bitField0_ & ~0x00000001);
gpio_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:diozero.Gpio.ProvisionAnalogInputDeviceRequest)
}
// @@protoc_insertion_point(class_scope:diozero.Gpio.ProvisionAnalogInputDeviceRequest)
private static final com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest();
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ProvisionAnalogInputDeviceRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ProvisionAnalogOutputDeviceRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:diozero.Gpio.ProvisionAnalogOutputDeviceRequest)
com.google.protobuf.MessageOrBuilder {
/**
* int32 gpio = 1;
* @return The gpio.
*/
int getGpio();
/**
* float initialValue = 2;
* @return The initialValue.
*/
float getInitialValue();
}
/**
* Protobuf type {@code diozero.Gpio.ProvisionAnalogOutputDeviceRequest}
*/
public static final class ProvisionAnalogOutputDeviceRequest extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:diozero.Gpio.ProvisionAnalogOutputDeviceRequest)
ProvisionAnalogOutputDeviceRequestOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
ProvisionAnalogOutputDeviceRequest.class.getName());
}
// Use ProvisionAnalogOutputDeviceRequest.newBuilder() to construct.
private ProvisionAnalogOutputDeviceRequest(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private ProvisionAnalogOutputDeviceRequest() {
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_ProvisionAnalogOutputDeviceRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_ProvisionAnalogOutputDeviceRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest.class, com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest.Builder.class);
}
public static final int GPIO_FIELD_NUMBER = 1;
private int gpio_ = 0;
/**
* int32 gpio = 1;
* @return The gpio.
*/
@java.lang.Override
public int getGpio() {
return gpio_;
}
public static final int INITIALVALUE_FIELD_NUMBER = 2;
private float initialValue_ = 0F;
/**
* float initialValue = 2;
* @return The initialValue.
*/
@java.lang.Override
public float getInitialValue() {
return initialValue_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (gpio_ != 0) {
output.writeInt32(1, gpio_);
}
if (java.lang.Float.floatToRawIntBits(initialValue_) != 0) {
output.writeFloat(2, initialValue_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (gpio_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, gpio_);
}
if (java.lang.Float.floatToRawIntBits(initialValue_) != 0) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(2, initialValue_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest)) {
return super.equals(obj);
}
com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest other = (com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest) obj;
if (getGpio()
!= other.getGpio()) return false;
if (java.lang.Float.floatToIntBits(getInitialValue())
!= java.lang.Float.floatToIntBits(
other.getInitialValue())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + GPIO_FIELD_NUMBER;
hash = (53 * hash) + getGpio();
hash = (37 * hash) + INITIALVALUE_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getInitialValue());
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code diozero.Gpio.ProvisionAnalogOutputDeviceRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:diozero.Gpio.ProvisionAnalogOutputDeviceRequest)
com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_ProvisionAnalogOutputDeviceRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_ProvisionAnalogOutputDeviceRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest.class, com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest.Builder.class);
}
// Construct using com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
gpio_ = 0;
initialValue_ = 0F;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_ProvisionAnalogOutputDeviceRequest_descriptor;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest getDefaultInstanceForType() {
return com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest.getDefaultInstance();
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest build() {
com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest buildPartial() {
com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest result = new com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.gpio_ = gpio_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.initialValue_ = initialValue_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest) {
return mergeFrom((com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest other) {
if (other == com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest.getDefaultInstance()) return this;
if (other.getGpio() != 0) {
setGpio(other.getGpio());
}
if (other.getInitialValue() != 0F) {
setInitialValue(other.getInitialValue());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
gpio_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 21: {
initialValue_ = input.readFloat();
bitField0_ |= 0x00000002;
break;
} // case 21
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int gpio_ ;
/**
* int32 gpio = 1;
* @return The gpio.
*/
@java.lang.Override
public int getGpio() {
return gpio_;
}
/**
* int32 gpio = 1;
* @param value The gpio to set.
* @return This builder for chaining.
*/
public Builder setGpio(int value) {
gpio_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* int32 gpio = 1;
* @return This builder for chaining.
*/
public Builder clearGpio() {
bitField0_ = (bitField0_ & ~0x00000001);
gpio_ = 0;
onChanged();
return this;
}
private float initialValue_ ;
/**
* float initialValue = 2;
* @return The initialValue.
*/
@java.lang.Override
public float getInitialValue() {
return initialValue_;
}
/**
* float initialValue = 2;
* @param value The initialValue to set.
* @return This builder for chaining.
*/
public Builder setInitialValue(float value) {
initialValue_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* float initialValue = 2;
* @return This builder for chaining.
*/
public Builder clearInitialValue() {
bitField0_ = (bitField0_ & ~0x00000002);
initialValue_ = 0F;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:diozero.Gpio.ProvisionAnalogOutputDeviceRequest)
}
// @@protoc_insertion_point(class_scope:diozero.Gpio.ProvisionAnalogOutputDeviceRequest)
private static final com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest();
}
public static com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ProvisionAnalogOutputDeviceRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface BooleanMessageOrBuilder extends
// @@protoc_insertion_point(interface_extends:diozero.Gpio.BooleanMessage)
com.google.protobuf.MessageOrBuilder {
/**
* int32 gpio = 1;
* @return The gpio.
*/
int getGpio();
/**
* bool value = 2;
* @return The value.
*/
boolean getValue();
}
/**
* Protobuf type {@code diozero.Gpio.BooleanMessage}
*/
public static final class BooleanMessage extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:diozero.Gpio.BooleanMessage)
BooleanMessageOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
BooleanMessage.class.getName());
}
// Use BooleanMessage.newBuilder() to construct.
private BooleanMessage(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private BooleanMessage() {
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_BooleanMessage_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_BooleanMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.Gpio.BooleanMessage.class, com.diozero.remote.message.protobuf.Gpio.BooleanMessage.Builder.class);
}
public static final int GPIO_FIELD_NUMBER = 1;
private int gpio_ = 0;
/**
* int32 gpio = 1;
* @return The gpio.
*/
@java.lang.Override
public int getGpio() {
return gpio_;
}
public static final int VALUE_FIELD_NUMBER = 2;
private boolean value_ = false;
/**
* bool value = 2;
* @return The value.
*/
@java.lang.Override
public boolean getValue() {
return value_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (gpio_ != 0) {
output.writeInt32(1, gpio_);
}
if (value_ != false) {
output.writeBool(2, value_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (gpio_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, gpio_);
}
if (value_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, value_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.diozero.remote.message.protobuf.Gpio.BooleanMessage)) {
return super.equals(obj);
}
com.diozero.remote.message.protobuf.Gpio.BooleanMessage other = (com.diozero.remote.message.protobuf.Gpio.BooleanMessage) obj;
if (getGpio()
!= other.getGpio()) return false;
if (getValue()
!= other.getValue()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + GPIO_FIELD_NUMBER;
hash = (53 * hash) + getGpio();
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getValue());
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.diozero.remote.message.protobuf.Gpio.BooleanMessage parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.BooleanMessage parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.BooleanMessage parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.BooleanMessage parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.BooleanMessage parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.BooleanMessage parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.BooleanMessage parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.BooleanMessage parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.BooleanMessage parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.BooleanMessage parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.BooleanMessage parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.BooleanMessage parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.diozero.remote.message.protobuf.Gpio.BooleanMessage prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code diozero.Gpio.BooleanMessage}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:diozero.Gpio.BooleanMessage)
com.diozero.remote.message.protobuf.Gpio.BooleanMessageOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_BooleanMessage_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_BooleanMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.Gpio.BooleanMessage.class, com.diozero.remote.message.protobuf.Gpio.BooleanMessage.Builder.class);
}
// Construct using com.diozero.remote.message.protobuf.Gpio.BooleanMessage.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
gpio_ = 0;
value_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_BooleanMessage_descriptor;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.BooleanMessage getDefaultInstanceForType() {
return com.diozero.remote.message.protobuf.Gpio.BooleanMessage.getDefaultInstance();
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.BooleanMessage build() {
com.diozero.remote.message.protobuf.Gpio.BooleanMessage result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.BooleanMessage buildPartial() {
com.diozero.remote.message.protobuf.Gpio.BooleanMessage result = new com.diozero.remote.message.protobuf.Gpio.BooleanMessage(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.diozero.remote.message.protobuf.Gpio.BooleanMessage result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.gpio_ = gpio_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.value_ = value_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.diozero.remote.message.protobuf.Gpio.BooleanMessage) {
return mergeFrom((com.diozero.remote.message.protobuf.Gpio.BooleanMessage)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.diozero.remote.message.protobuf.Gpio.BooleanMessage other) {
if (other == com.diozero.remote.message.protobuf.Gpio.BooleanMessage.getDefaultInstance()) return this;
if (other.getGpio() != 0) {
setGpio(other.getGpio());
}
if (other.getValue() != false) {
setValue(other.getValue());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
gpio_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
value_ = input.readBool();
bitField0_ |= 0x00000002;
break;
} // case 16
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int gpio_ ;
/**
* int32 gpio = 1;
* @return The gpio.
*/
@java.lang.Override
public int getGpio() {
return gpio_;
}
/**
* int32 gpio = 1;
* @param value The gpio to set.
* @return This builder for chaining.
*/
public Builder setGpio(int value) {
gpio_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* int32 gpio = 1;
* @return This builder for chaining.
*/
public Builder clearGpio() {
bitField0_ = (bitField0_ & ~0x00000001);
gpio_ = 0;
onChanged();
return this;
}
private boolean value_ ;
/**
* bool value = 2;
* @return The value.
*/
@java.lang.Override
public boolean getValue() {
return value_;
}
/**
* bool value = 2;
* @param value The value to set.
* @return This builder for chaining.
*/
public Builder setValue(boolean value) {
value_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* bool value = 2;
* @return This builder for chaining.
*/
public Builder clearValue() {
bitField0_ = (bitField0_ & ~0x00000002);
value_ = false;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:diozero.Gpio.BooleanMessage)
}
// @@protoc_insertion_point(class_scope:diozero.Gpio.BooleanMessage)
private static final com.diozero.remote.message.protobuf.Gpio.BooleanMessage DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.diozero.remote.message.protobuf.Gpio.BooleanMessage();
}
public static com.diozero.remote.message.protobuf.Gpio.BooleanMessage getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public BooleanMessage parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.BooleanMessage getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface FloatMessageOrBuilder extends
// @@protoc_insertion_point(interface_extends:diozero.Gpio.FloatMessage)
com.google.protobuf.MessageOrBuilder {
/**
* int32 gpio = 1;
* @return The gpio.
*/
int getGpio();
/**
* float value = 2;
* @return The value.
*/
float getValue();
}
/**
* Protobuf type {@code diozero.Gpio.FloatMessage}
*/
public static final class FloatMessage extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:diozero.Gpio.FloatMessage)
FloatMessageOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
FloatMessage.class.getName());
}
// Use FloatMessage.newBuilder() to construct.
private FloatMessage(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private FloatMessage() {
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_FloatMessage_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_FloatMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.Gpio.FloatMessage.class, com.diozero.remote.message.protobuf.Gpio.FloatMessage.Builder.class);
}
public static final int GPIO_FIELD_NUMBER = 1;
private int gpio_ = 0;
/**
* int32 gpio = 1;
* @return The gpio.
*/
@java.lang.Override
public int getGpio() {
return gpio_;
}
public static final int VALUE_FIELD_NUMBER = 2;
private float value_ = 0F;
/**
* float value = 2;
* @return The value.
*/
@java.lang.Override
public float getValue() {
return value_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (gpio_ != 0) {
output.writeInt32(1, gpio_);
}
if (java.lang.Float.floatToRawIntBits(value_) != 0) {
output.writeFloat(2, value_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (gpio_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, gpio_);
}
if (java.lang.Float.floatToRawIntBits(value_) != 0) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(2, value_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.diozero.remote.message.protobuf.Gpio.FloatMessage)) {
return super.equals(obj);
}
com.diozero.remote.message.protobuf.Gpio.FloatMessage other = (com.diozero.remote.message.protobuf.Gpio.FloatMessage) obj;
if (getGpio()
!= other.getGpio()) return false;
if (java.lang.Float.floatToIntBits(getValue())
!= java.lang.Float.floatToIntBits(
other.getValue())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + GPIO_FIELD_NUMBER;
hash = (53 * hash) + getGpio();
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getValue());
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.diozero.remote.message.protobuf.Gpio.FloatMessage parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.FloatMessage parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.FloatMessage parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.FloatMessage parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.FloatMessage parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.FloatMessage parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.FloatMessage parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.FloatMessage parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.FloatMessage parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.FloatMessage parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.FloatMessage parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.FloatMessage parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.diozero.remote.message.protobuf.Gpio.FloatMessage prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code diozero.Gpio.FloatMessage}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:diozero.Gpio.FloatMessage)
com.diozero.remote.message.protobuf.Gpio.FloatMessageOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_FloatMessage_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_FloatMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.Gpio.FloatMessage.class, com.diozero.remote.message.protobuf.Gpio.FloatMessage.Builder.class);
}
// Construct using com.diozero.remote.message.protobuf.Gpio.FloatMessage.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
gpio_ = 0;
value_ = 0F;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_FloatMessage_descriptor;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.FloatMessage getDefaultInstanceForType() {
return com.diozero.remote.message.protobuf.Gpio.FloatMessage.getDefaultInstance();
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.FloatMessage build() {
com.diozero.remote.message.protobuf.Gpio.FloatMessage result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.FloatMessage buildPartial() {
com.diozero.remote.message.protobuf.Gpio.FloatMessage result = new com.diozero.remote.message.protobuf.Gpio.FloatMessage(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.diozero.remote.message.protobuf.Gpio.FloatMessage result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.gpio_ = gpio_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.value_ = value_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.diozero.remote.message.protobuf.Gpio.FloatMessage) {
return mergeFrom((com.diozero.remote.message.protobuf.Gpio.FloatMessage)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.diozero.remote.message.protobuf.Gpio.FloatMessage other) {
if (other == com.diozero.remote.message.protobuf.Gpio.FloatMessage.getDefaultInstance()) return this;
if (other.getGpio() != 0) {
setGpio(other.getGpio());
}
if (other.getValue() != 0F) {
setValue(other.getValue());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
gpio_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 21: {
value_ = input.readFloat();
bitField0_ |= 0x00000002;
break;
} // case 21
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int gpio_ ;
/**
* int32 gpio = 1;
* @return The gpio.
*/
@java.lang.Override
public int getGpio() {
return gpio_;
}
/**
* int32 gpio = 1;
* @param value The gpio to set.
* @return This builder for chaining.
*/
public Builder setGpio(int value) {
gpio_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* int32 gpio = 1;
* @return This builder for chaining.
*/
public Builder clearGpio() {
bitField0_ = (bitField0_ & ~0x00000001);
gpio_ = 0;
onChanged();
return this;
}
private float value_ ;
/**
* float value = 2;
* @return The value.
*/
@java.lang.Override
public float getValue() {
return value_;
}
/**
* float value = 2;
* @param value The value to set.
* @return This builder for chaining.
*/
public Builder setValue(float value) {
value_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* float value = 2;
* @return This builder for chaining.
*/
public Builder clearValue() {
bitField0_ = (bitField0_ & ~0x00000002);
value_ = 0F;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:diozero.Gpio.FloatMessage)
}
// @@protoc_insertion_point(class_scope:diozero.Gpio.FloatMessage)
private static final com.diozero.remote.message.protobuf.Gpio.FloatMessage DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.diozero.remote.message.protobuf.Gpio.FloatMessage();
}
public static com.diozero.remote.message.protobuf.Gpio.FloatMessage getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public FloatMessage parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.FloatMessage getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface IntegerMessageOrBuilder extends
// @@protoc_insertion_point(interface_extends:diozero.Gpio.IntegerMessage)
com.google.protobuf.MessageOrBuilder {
/**
* int32 gpio = 1;
* @return The gpio.
*/
int getGpio();
/**
* int32 value = 2;
* @return The value.
*/
int getValue();
}
/**
* Protobuf type {@code diozero.Gpio.IntegerMessage}
*/
public static final class IntegerMessage extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:diozero.Gpio.IntegerMessage)
IntegerMessageOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
IntegerMessage.class.getName());
}
// Use IntegerMessage.newBuilder() to construct.
private IntegerMessage(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private IntegerMessage() {
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_IntegerMessage_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_IntegerMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.Gpio.IntegerMessage.class, com.diozero.remote.message.protobuf.Gpio.IntegerMessage.Builder.class);
}
public static final int GPIO_FIELD_NUMBER = 1;
private int gpio_ = 0;
/**
* int32 gpio = 1;
* @return The gpio.
*/
@java.lang.Override
public int getGpio() {
return gpio_;
}
public static final int VALUE_FIELD_NUMBER = 2;
private int value_ = 0;
/**
* int32 value = 2;
* @return The value.
*/
@java.lang.Override
public int getValue() {
return value_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (gpio_ != 0) {
output.writeInt32(1, gpio_);
}
if (value_ != 0) {
output.writeInt32(2, value_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (gpio_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, gpio_);
}
if (value_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, value_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.diozero.remote.message.protobuf.Gpio.IntegerMessage)) {
return super.equals(obj);
}
com.diozero.remote.message.protobuf.Gpio.IntegerMessage other = (com.diozero.remote.message.protobuf.Gpio.IntegerMessage) obj;
if (getGpio()
!= other.getGpio()) return false;
if (getValue()
!= other.getValue()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + GPIO_FIELD_NUMBER;
hash = (53 * hash) + getGpio();
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.diozero.remote.message.protobuf.Gpio.IntegerMessage parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.IntegerMessage parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.IntegerMessage parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.IntegerMessage parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.IntegerMessage parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.IntegerMessage parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.IntegerMessage parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.IntegerMessage parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.IntegerMessage parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.IntegerMessage parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.IntegerMessage parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.IntegerMessage parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.diozero.remote.message.protobuf.Gpio.IntegerMessage prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code diozero.Gpio.IntegerMessage}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:diozero.Gpio.IntegerMessage)
com.diozero.remote.message.protobuf.Gpio.IntegerMessageOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_IntegerMessage_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_IntegerMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.Gpio.IntegerMessage.class, com.diozero.remote.message.protobuf.Gpio.IntegerMessage.Builder.class);
}
// Construct using com.diozero.remote.message.protobuf.Gpio.IntegerMessage.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
gpio_ = 0;
value_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_IntegerMessage_descriptor;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.IntegerMessage getDefaultInstanceForType() {
return com.diozero.remote.message.protobuf.Gpio.IntegerMessage.getDefaultInstance();
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.IntegerMessage build() {
com.diozero.remote.message.protobuf.Gpio.IntegerMessage result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.IntegerMessage buildPartial() {
com.diozero.remote.message.protobuf.Gpio.IntegerMessage result = new com.diozero.remote.message.protobuf.Gpio.IntegerMessage(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.diozero.remote.message.protobuf.Gpio.IntegerMessage result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.gpio_ = gpio_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.value_ = value_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.diozero.remote.message.protobuf.Gpio.IntegerMessage) {
return mergeFrom((com.diozero.remote.message.protobuf.Gpio.IntegerMessage)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.diozero.remote.message.protobuf.Gpio.IntegerMessage other) {
if (other == com.diozero.remote.message.protobuf.Gpio.IntegerMessage.getDefaultInstance()) return this;
if (other.getGpio() != 0) {
setGpio(other.getGpio());
}
if (other.getValue() != 0) {
setValue(other.getValue());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
gpio_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
value_ = input.readInt32();
bitField0_ |= 0x00000002;
break;
} // case 16
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int gpio_ ;
/**
* int32 gpio = 1;
* @return The gpio.
*/
@java.lang.Override
public int getGpio() {
return gpio_;
}
/**
* int32 gpio = 1;
* @param value The gpio to set.
* @return This builder for chaining.
*/
public Builder setGpio(int value) {
gpio_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* int32 gpio = 1;
* @return This builder for chaining.
*/
public Builder clearGpio() {
bitField0_ = (bitField0_ & ~0x00000001);
gpio_ = 0;
onChanged();
return this;
}
private int value_ ;
/**
* int32 value = 2;
* @return The value.
*/
@java.lang.Override
public int getValue() {
return value_;
}
/**
* int32 value = 2;
* @param value The value to set.
* @return This builder for chaining.
*/
public Builder setValue(int value) {
value_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* int32 value = 2;
* @return This builder for chaining.
*/
public Builder clearValue() {
bitField0_ = (bitField0_ & ~0x00000002);
value_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:diozero.Gpio.IntegerMessage)
}
// @@protoc_insertion_point(class_scope:diozero.Gpio.IntegerMessage)
private static final com.diozero.remote.message.protobuf.Gpio.IntegerMessage DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.diozero.remote.message.protobuf.Gpio.IntegerMessage();
}
public static com.diozero.remote.message.protobuf.Gpio.IntegerMessage getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public IntegerMessage parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.IntegerMessage getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface EventOrBuilder extends
// @@protoc_insertion_point(interface_extends:diozero.Gpio.Event)
com.google.protobuf.MessageOrBuilder {
/**
* int32 gpio = 1;
* @return The gpio.
*/
int getGpio();
/**
* optional int64 epochTime = 2;
* @return Whether the epochTime field is set.
*/
boolean hasEpochTime();
/**
* optional int64 epochTime = 2;
* @return The epochTime.
*/
long getEpochTime();
/**
* optional int64 nanoTime = 3;
* @return Whether the nanoTime field is set.
*/
boolean hasNanoTime();
/**
* optional int64 nanoTime = 3;
* @return The nanoTime.
*/
long getNanoTime();
/**
* optional bool value = 4;
* @return Whether the value field is set.
*/
boolean hasValue();
/**
* optional bool value = 4;
* @return The value.
*/
boolean getValue();
/**
* optional .diozero.Status status = 5;
* @return Whether the status field is set.
*/
boolean hasStatus();
/**
* optional .diozero.Status status = 5;
* @return The enum numeric value on the wire for status.
*/
int getStatusValue();
/**
* optional .diozero.Status status = 5;
* @return The status.
*/
com.diozero.remote.message.protobuf.Status getStatus();
/**
* optional string detail = 6;
* @return Whether the detail field is set.
*/
boolean hasDetail();
/**
* optional string detail = 6;
* @return The detail.
*/
java.lang.String getDetail();
/**
* optional string detail = 6;
* @return The bytes for detail.
*/
com.google.protobuf.ByteString
getDetailBytes();
}
/**
* Protobuf type {@code diozero.Gpio.Event}
*/
public static final class Event extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:diozero.Gpio.Event)
EventOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
Event.class.getName());
}
// Use Event.newBuilder() to construct.
private Event(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Event() {
status_ = 0;
detail_ = "";
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_Event_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_Event_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.Gpio.Event.class, com.diozero.remote.message.protobuf.Gpio.Event.Builder.class);
}
private int bitField0_;
public static final int GPIO_FIELD_NUMBER = 1;
private int gpio_ = 0;
/**
* int32 gpio = 1;
* @return The gpio.
*/
@java.lang.Override
public int getGpio() {
return gpio_;
}
public static final int EPOCHTIME_FIELD_NUMBER = 2;
private long epochTime_ = 0L;
/**
* optional int64 epochTime = 2;
* @return Whether the epochTime field is set.
*/
@java.lang.Override
public boolean hasEpochTime() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional int64 epochTime = 2;
* @return The epochTime.
*/
@java.lang.Override
public long getEpochTime() {
return epochTime_;
}
public static final int NANOTIME_FIELD_NUMBER = 3;
private long nanoTime_ = 0L;
/**
* optional int64 nanoTime = 3;
* @return Whether the nanoTime field is set.
*/
@java.lang.Override
public boolean hasNanoTime() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional int64 nanoTime = 3;
* @return The nanoTime.
*/
@java.lang.Override
public long getNanoTime() {
return nanoTime_;
}
public static final int VALUE_FIELD_NUMBER = 4;
private boolean value_ = false;
/**
* optional bool value = 4;
* @return Whether the value field is set.
*/
@java.lang.Override
public boolean hasValue() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional bool value = 4;
* @return The value.
*/
@java.lang.Override
public boolean getValue() {
return value_;
}
public static final int STATUS_FIELD_NUMBER = 5;
private int status_ = 0;
/**
* optional .diozero.Status status = 5;
* @return Whether the status field is set.
*/
@java.lang.Override public boolean hasStatus() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional .diozero.Status status = 5;
* @return The enum numeric value on the wire for status.
*/
@java.lang.Override public int getStatusValue() {
return status_;
}
/**
* optional .diozero.Status status = 5;
* @return The status.
*/
@java.lang.Override public com.diozero.remote.message.protobuf.Status getStatus() {
com.diozero.remote.message.protobuf.Status result = com.diozero.remote.message.protobuf.Status.forNumber(status_);
return result == null ? com.diozero.remote.message.protobuf.Status.UNRECOGNIZED : result;
}
public static final int DETAIL_FIELD_NUMBER = 6;
@SuppressWarnings("serial")
private volatile java.lang.Object detail_ = "";
/**
* optional string detail = 6;
* @return Whether the detail field is set.
*/
@java.lang.Override
public boolean hasDetail() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional string detail = 6;
* @return The detail.
*/
@java.lang.Override
public java.lang.String getDetail() {
java.lang.Object ref = detail_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
detail_ = s;
return s;
}
}
/**
* optional string detail = 6;
* @return The bytes for detail.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDetailBytes() {
java.lang.Object ref = detail_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
detail_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (gpio_ != 0) {
output.writeInt32(1, gpio_);
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt64(2, epochTime_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeInt64(3, nanoTime_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeBool(4, value_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeEnum(5, status_);
}
if (((bitField0_ & 0x00000010) != 0)) {
com.google.protobuf.GeneratedMessage.writeString(output, 6, detail_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (gpio_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, gpio_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, epochTime_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, nanoTime_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, value_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(5, status_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(6, detail_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.diozero.remote.message.protobuf.Gpio.Event)) {
return super.equals(obj);
}
com.diozero.remote.message.protobuf.Gpio.Event other = (com.diozero.remote.message.protobuf.Gpio.Event) obj;
if (getGpio()
!= other.getGpio()) return false;
if (hasEpochTime() != other.hasEpochTime()) return false;
if (hasEpochTime()) {
if (getEpochTime()
!= other.getEpochTime()) return false;
}
if (hasNanoTime() != other.hasNanoTime()) return false;
if (hasNanoTime()) {
if (getNanoTime()
!= other.getNanoTime()) return false;
}
if (hasValue() != other.hasValue()) return false;
if (hasValue()) {
if (getValue()
!= other.getValue()) return false;
}
if (hasStatus() != other.hasStatus()) return false;
if (hasStatus()) {
if (status_ != other.status_) return false;
}
if (hasDetail() != other.hasDetail()) return false;
if (hasDetail()) {
if (!getDetail()
.equals(other.getDetail())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + GPIO_FIELD_NUMBER;
hash = (53 * hash) + getGpio();
if (hasEpochTime()) {
hash = (37 * hash) + EPOCHTIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getEpochTime());
}
if (hasNanoTime()) {
hash = (37 * hash) + NANOTIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getNanoTime());
}
if (hasValue()) {
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getValue());
}
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + status_;
}
if (hasDetail()) {
hash = (37 * hash) + DETAIL_FIELD_NUMBER;
hash = (53 * hash) + getDetail().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.diozero.remote.message.protobuf.Gpio.Event parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.Event parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.Event parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.Event parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.Event parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio.Event parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.Event parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.Event parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.Event parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.Event parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio.Event parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio.Event parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.diozero.remote.message.protobuf.Gpio.Event prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code diozero.Gpio.Event}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:diozero.Gpio.Event)
com.diozero.remote.message.protobuf.Gpio.EventOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_Event_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_Event_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.Gpio.Event.class, com.diozero.remote.message.protobuf.Gpio.Event.Builder.class);
}
// Construct using com.diozero.remote.message.protobuf.Gpio.Event.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
gpio_ = 0;
epochTime_ = 0L;
nanoTime_ = 0L;
value_ = false;
status_ = 0;
detail_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_Event_descriptor;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.Event getDefaultInstanceForType() {
return com.diozero.remote.message.protobuf.Gpio.Event.getDefaultInstance();
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.Event build() {
com.diozero.remote.message.protobuf.Gpio.Event result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.Event buildPartial() {
com.diozero.remote.message.protobuf.Gpio.Event result = new com.diozero.remote.message.protobuf.Gpio.Event(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.diozero.remote.message.protobuf.Gpio.Event result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.gpio_ = gpio_;
}
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000002) != 0)) {
result.epochTime_ = epochTime_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.nanoTime_ = nanoTime_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.value_ = value_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.status_ = status_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.detail_ = detail_;
to_bitField0_ |= 0x00000010;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.diozero.remote.message.protobuf.Gpio.Event) {
return mergeFrom((com.diozero.remote.message.protobuf.Gpio.Event)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.diozero.remote.message.protobuf.Gpio.Event other) {
if (other == com.diozero.remote.message.protobuf.Gpio.Event.getDefaultInstance()) return this;
if (other.getGpio() != 0) {
setGpio(other.getGpio());
}
if (other.hasEpochTime()) {
setEpochTime(other.getEpochTime());
}
if (other.hasNanoTime()) {
setNanoTime(other.getNanoTime());
}
if (other.hasValue()) {
setValue(other.getValue());
}
if (other.hasStatus()) {
setStatus(other.getStatus());
}
if (other.hasDetail()) {
detail_ = other.detail_;
bitField0_ |= 0x00000020;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
gpio_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
epochTime_ = input.readInt64();
bitField0_ |= 0x00000002;
break;
} // case 16
case 24: {
nanoTime_ = input.readInt64();
bitField0_ |= 0x00000004;
break;
} // case 24
case 32: {
value_ = input.readBool();
bitField0_ |= 0x00000008;
break;
} // case 32
case 40: {
status_ = input.readEnum();
bitField0_ |= 0x00000010;
break;
} // case 40
case 50: {
detail_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000020;
break;
} // case 50
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int gpio_ ;
/**
* int32 gpio = 1;
* @return The gpio.
*/
@java.lang.Override
public int getGpio() {
return gpio_;
}
/**
* int32 gpio = 1;
* @param value The gpio to set.
* @return This builder for chaining.
*/
public Builder setGpio(int value) {
gpio_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* int32 gpio = 1;
* @return This builder for chaining.
*/
public Builder clearGpio() {
bitField0_ = (bitField0_ & ~0x00000001);
gpio_ = 0;
onChanged();
return this;
}
private long epochTime_ ;
/**
* optional int64 epochTime = 2;
* @return Whether the epochTime field is set.
*/
@java.lang.Override
public boolean hasEpochTime() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional int64 epochTime = 2;
* @return The epochTime.
*/
@java.lang.Override
public long getEpochTime() {
return epochTime_;
}
/**
* optional int64 epochTime = 2;
* @param value The epochTime to set.
* @return This builder for chaining.
*/
public Builder setEpochTime(long value) {
epochTime_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* optional int64 epochTime = 2;
* @return This builder for chaining.
*/
public Builder clearEpochTime() {
bitField0_ = (bitField0_ & ~0x00000002);
epochTime_ = 0L;
onChanged();
return this;
}
private long nanoTime_ ;
/**
* optional int64 nanoTime = 3;
* @return Whether the nanoTime field is set.
*/
@java.lang.Override
public boolean hasNanoTime() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional int64 nanoTime = 3;
* @return The nanoTime.
*/
@java.lang.Override
public long getNanoTime() {
return nanoTime_;
}
/**
* optional int64 nanoTime = 3;
* @param value The nanoTime to set.
* @return This builder for chaining.
*/
public Builder setNanoTime(long value) {
nanoTime_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* optional int64 nanoTime = 3;
* @return This builder for chaining.
*/
public Builder clearNanoTime() {
bitField0_ = (bitField0_ & ~0x00000004);
nanoTime_ = 0L;
onChanged();
return this;
}
private boolean value_ ;
/**
* optional bool value = 4;
* @return Whether the value field is set.
*/
@java.lang.Override
public boolean hasValue() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional bool value = 4;
* @return The value.
*/
@java.lang.Override
public boolean getValue() {
return value_;
}
/**
* optional bool value = 4;
* @param value The value to set.
* @return This builder for chaining.
*/
public Builder setValue(boolean value) {
value_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* optional bool value = 4;
* @return This builder for chaining.
*/
public Builder clearValue() {
bitField0_ = (bitField0_ & ~0x00000008);
value_ = false;
onChanged();
return this;
}
private int status_ = 0;
/**
* optional .diozero.Status status = 5;
* @return Whether the status field is set.
*/
@java.lang.Override public boolean hasStatus() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional .diozero.Status status = 5;
* @return The enum numeric value on the wire for status.
*/
@java.lang.Override public int getStatusValue() {
return status_;
}
/**
* optional .diozero.Status status = 5;
* @param value The enum numeric value on the wire for status to set.
* @return This builder for chaining.
*/
public Builder setStatusValue(int value) {
status_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
* optional .diozero.Status status = 5;
* @return The status.
*/
@java.lang.Override
public com.diozero.remote.message.protobuf.Status getStatus() {
com.diozero.remote.message.protobuf.Status result = com.diozero.remote.message.protobuf.Status.forNumber(status_);
return result == null ? com.diozero.remote.message.protobuf.Status.UNRECOGNIZED : result;
}
/**
* optional .diozero.Status status = 5;
* @param value The status to set.
* @return This builder for chaining.
*/
public Builder setStatus(com.diozero.remote.message.protobuf.Status value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
status_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .diozero.Status status = 5;
* @return This builder for chaining.
*/
public Builder clearStatus() {
bitField0_ = (bitField0_ & ~0x00000010);
status_ = 0;
onChanged();
return this;
}
private java.lang.Object detail_ = "";
/**
* optional string detail = 6;
* @return Whether the detail field is set.
*/
public boolean hasDetail() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
* optional string detail = 6;
* @return The detail.
*/
public java.lang.String getDetail() {
java.lang.Object ref = detail_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
detail_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string detail = 6;
* @return The bytes for detail.
*/
public com.google.protobuf.ByteString
getDetailBytes() {
java.lang.Object ref = detail_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
detail_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string detail = 6;
* @param value The detail to set.
* @return This builder for chaining.
*/
public Builder setDetail(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
detail_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
* optional string detail = 6;
* @return This builder for chaining.
*/
public Builder clearDetail() {
detail_ = getDefaultInstance().getDetail();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
return this;
}
/**
* optional string detail = 6;
* @param value The bytes for detail to set.
* @return This builder for chaining.
*/
public Builder setDetailBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
detail_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:diozero.Gpio.Event)
}
// @@protoc_insertion_point(class_scope:diozero.Gpio.Event)
private static final com.diozero.remote.message.protobuf.Gpio.Event DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.diozero.remote.message.protobuf.Gpio.Event();
}
public static com.diozero.remote.message.protobuf.Gpio.Event getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Event parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio.Event getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.diozero.remote.message.protobuf.Gpio)) {
return super.equals(obj);
}
com.diozero.remote.message.protobuf.Gpio other = (com.diozero.remote.message.protobuf.Gpio) obj;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.diozero.remote.message.protobuf.Gpio parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.Gpio parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.Gpio parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.Gpio parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.diozero.remote.message.protobuf.Gpio prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code diozero.Gpio}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:diozero.Gpio)
com.diozero.remote.message.protobuf.GpioOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.Gpio.class, com.diozero.remote.message.protobuf.Gpio.Builder.class);
}
// Construct using com.diozero.remote.message.protobuf.Gpio.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_Gpio_descriptor;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio getDefaultInstanceForType() {
return com.diozero.remote.message.protobuf.Gpio.getDefaultInstance();
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio build() {
com.diozero.remote.message.protobuf.Gpio result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio buildPartial() {
com.diozero.remote.message.protobuf.Gpio result = new com.diozero.remote.message.protobuf.Gpio(this);
onBuilt();
return result;
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.diozero.remote.message.protobuf.Gpio) {
return mergeFrom((com.diozero.remote.message.protobuf.Gpio)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.diozero.remote.message.protobuf.Gpio other) {
if (other == com.diozero.remote.message.protobuf.Gpio.getDefaultInstance()) return this;
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
// @@protoc_insertion_point(builder_scope:diozero.Gpio)
}
// @@protoc_insertion_point(class_scope:diozero.Gpio)
private static final com.diozero.remote.message.protobuf.Gpio DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.diozero.remote.message.protobuf.Gpio();
}
public static com.diozero.remote.message.protobuf.Gpio getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Gpio parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.Gpio getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy