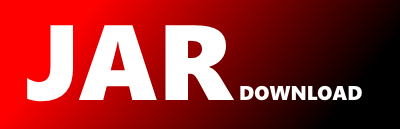
com.diozero.remote.message.protobuf.GpioServiceGrpc Maven / Gradle / Ivy
The newest version!
package com.diozero.remote.message.protobuf;
import static io.grpc.MethodDescriptor.generateFullMethodName;
/**
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.68.0)",
comments = "Source: diozero.proto")
@io.grpc.stub.annotations.GrpcGenerated
public final class GpioServiceGrpc {
private GpioServiceGrpc() {}
public static final java.lang.String SERVICE_NAME = "diozero.GpioService";
// Static method descriptors that strictly reflect the proto.
private static volatile io.grpc.MethodDescriptor getProvisionDigitalInputDeviceMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ProvisionDigitalInputDevice",
requestType = com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest.class,
responseType = com.diozero.remote.message.protobuf.Response.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getProvisionDigitalInputDeviceMethod() {
io.grpc.MethodDescriptor getProvisionDigitalInputDeviceMethod;
if ((getProvisionDigitalInputDeviceMethod = GpioServiceGrpc.getProvisionDigitalInputDeviceMethod) == null) {
synchronized (GpioServiceGrpc.class) {
if ((getProvisionDigitalInputDeviceMethod = GpioServiceGrpc.getProvisionDigitalInputDeviceMethod) == null) {
GpioServiceGrpc.getProvisionDigitalInputDeviceMethod = getProvisionDigitalInputDeviceMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ProvisionDigitalInputDevice"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Response.getDefaultInstance()))
.setSchemaDescriptor(new GpioServiceMethodDescriptorSupplier("ProvisionDigitalInputDevice"))
.build();
}
}
}
return getProvisionDigitalInputDeviceMethod;
}
private static volatile io.grpc.MethodDescriptor getProvisionDigitalOutputDeviceMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ProvisionDigitalOutputDevice",
requestType = com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest.class,
responseType = com.diozero.remote.message.protobuf.Response.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getProvisionDigitalOutputDeviceMethod() {
io.grpc.MethodDescriptor getProvisionDigitalOutputDeviceMethod;
if ((getProvisionDigitalOutputDeviceMethod = GpioServiceGrpc.getProvisionDigitalOutputDeviceMethod) == null) {
synchronized (GpioServiceGrpc.class) {
if ((getProvisionDigitalOutputDeviceMethod = GpioServiceGrpc.getProvisionDigitalOutputDeviceMethod) == null) {
GpioServiceGrpc.getProvisionDigitalOutputDeviceMethod = getProvisionDigitalOutputDeviceMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ProvisionDigitalOutputDevice"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Response.getDefaultInstance()))
.setSchemaDescriptor(new GpioServiceMethodDescriptorSupplier("ProvisionDigitalOutputDevice"))
.build();
}
}
}
return getProvisionDigitalOutputDeviceMethod;
}
private static volatile io.grpc.MethodDescriptor getProvisionDigitalInputOutputDeviceMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ProvisionDigitalInputOutputDevice",
requestType = com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest.class,
responseType = com.diozero.remote.message.protobuf.Response.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getProvisionDigitalInputOutputDeviceMethod() {
io.grpc.MethodDescriptor getProvisionDigitalInputOutputDeviceMethod;
if ((getProvisionDigitalInputOutputDeviceMethod = GpioServiceGrpc.getProvisionDigitalInputOutputDeviceMethod) == null) {
synchronized (GpioServiceGrpc.class) {
if ((getProvisionDigitalInputOutputDeviceMethod = GpioServiceGrpc.getProvisionDigitalInputOutputDeviceMethod) == null) {
GpioServiceGrpc.getProvisionDigitalInputOutputDeviceMethod = getProvisionDigitalInputOutputDeviceMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ProvisionDigitalInputOutputDevice"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Response.getDefaultInstance()))
.setSchemaDescriptor(new GpioServiceMethodDescriptorSupplier("ProvisionDigitalInputOutputDevice"))
.build();
}
}
}
return getProvisionDigitalInputOutputDeviceMethod;
}
private static volatile io.grpc.MethodDescriptor getProvisionPwmOutputDeviceMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ProvisionPwmOutputDevice",
requestType = com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest.class,
responseType = com.diozero.remote.message.protobuf.Response.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getProvisionPwmOutputDeviceMethod() {
io.grpc.MethodDescriptor getProvisionPwmOutputDeviceMethod;
if ((getProvisionPwmOutputDeviceMethod = GpioServiceGrpc.getProvisionPwmOutputDeviceMethod) == null) {
synchronized (GpioServiceGrpc.class) {
if ((getProvisionPwmOutputDeviceMethod = GpioServiceGrpc.getProvisionPwmOutputDeviceMethod) == null) {
GpioServiceGrpc.getProvisionPwmOutputDeviceMethod = getProvisionPwmOutputDeviceMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ProvisionPwmOutputDevice"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Response.getDefaultInstance()))
.setSchemaDescriptor(new GpioServiceMethodDescriptorSupplier("ProvisionPwmOutputDevice"))
.build();
}
}
}
return getProvisionPwmOutputDeviceMethod;
}
private static volatile io.grpc.MethodDescriptor getProvisionServoDeviceMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ProvisionServoDevice",
requestType = com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest.class,
responseType = com.diozero.remote.message.protobuf.Response.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getProvisionServoDeviceMethod() {
io.grpc.MethodDescriptor getProvisionServoDeviceMethod;
if ((getProvisionServoDeviceMethod = GpioServiceGrpc.getProvisionServoDeviceMethod) == null) {
synchronized (GpioServiceGrpc.class) {
if ((getProvisionServoDeviceMethod = GpioServiceGrpc.getProvisionServoDeviceMethod) == null) {
GpioServiceGrpc.getProvisionServoDeviceMethod = getProvisionServoDeviceMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ProvisionServoDevice"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Response.getDefaultInstance()))
.setSchemaDescriptor(new GpioServiceMethodDescriptorSupplier("ProvisionServoDevice"))
.build();
}
}
}
return getProvisionServoDeviceMethod;
}
private static volatile io.grpc.MethodDescriptor getProvisionAnalogInputDeviceMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ProvisionAnalogInputDevice",
requestType = com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest.class,
responseType = com.diozero.remote.message.protobuf.Response.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getProvisionAnalogInputDeviceMethod() {
io.grpc.MethodDescriptor getProvisionAnalogInputDeviceMethod;
if ((getProvisionAnalogInputDeviceMethod = GpioServiceGrpc.getProvisionAnalogInputDeviceMethod) == null) {
synchronized (GpioServiceGrpc.class) {
if ((getProvisionAnalogInputDeviceMethod = GpioServiceGrpc.getProvisionAnalogInputDeviceMethod) == null) {
GpioServiceGrpc.getProvisionAnalogInputDeviceMethod = getProvisionAnalogInputDeviceMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ProvisionAnalogInputDevice"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Response.getDefaultInstance()))
.setSchemaDescriptor(new GpioServiceMethodDescriptorSupplier("ProvisionAnalogInputDevice"))
.build();
}
}
}
return getProvisionAnalogInputDeviceMethod;
}
private static volatile io.grpc.MethodDescriptor getProvisionAnalogOutputDeviceMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ProvisionAnalogOutputDevice",
requestType = com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest.class,
responseType = com.diozero.remote.message.protobuf.Response.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getProvisionAnalogOutputDeviceMethod() {
io.grpc.MethodDescriptor getProvisionAnalogOutputDeviceMethod;
if ((getProvisionAnalogOutputDeviceMethod = GpioServiceGrpc.getProvisionAnalogOutputDeviceMethod) == null) {
synchronized (GpioServiceGrpc.class) {
if ((getProvisionAnalogOutputDeviceMethod = GpioServiceGrpc.getProvisionAnalogOutputDeviceMethod) == null) {
GpioServiceGrpc.getProvisionAnalogOutputDeviceMethod = getProvisionAnalogOutputDeviceMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ProvisionAnalogOutputDevice"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Response.getDefaultInstance()))
.setSchemaDescriptor(new GpioServiceMethodDescriptorSupplier("ProvisionAnalogOutputDevice"))
.build();
}
}
}
return getProvisionAnalogOutputDeviceMethod;
}
private static volatile io.grpc.MethodDescriptor getDigitalReadMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "DigitalRead",
requestType = com.diozero.remote.message.protobuf.Gpio.Identifier.class,
responseType = com.diozero.remote.message.protobuf.BooleanResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getDigitalReadMethod() {
io.grpc.MethodDescriptor getDigitalReadMethod;
if ((getDigitalReadMethod = GpioServiceGrpc.getDigitalReadMethod) == null) {
synchronized (GpioServiceGrpc.class) {
if ((getDigitalReadMethod = GpioServiceGrpc.getDigitalReadMethod) == null) {
GpioServiceGrpc.getDigitalReadMethod = getDigitalReadMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "DigitalRead"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Gpio.Identifier.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.BooleanResponse.getDefaultInstance()))
.setSchemaDescriptor(new GpioServiceMethodDescriptorSupplier("DigitalRead"))
.build();
}
}
}
return getDigitalReadMethod;
}
private static volatile io.grpc.MethodDescriptor getDigitalWriteMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "DigitalWrite",
requestType = com.diozero.remote.message.protobuf.Gpio.BooleanMessage.class,
responseType = com.diozero.remote.message.protobuf.Response.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getDigitalWriteMethod() {
io.grpc.MethodDescriptor getDigitalWriteMethod;
if ((getDigitalWriteMethod = GpioServiceGrpc.getDigitalWriteMethod) == null) {
synchronized (GpioServiceGrpc.class) {
if ((getDigitalWriteMethod = GpioServiceGrpc.getDigitalWriteMethod) == null) {
GpioServiceGrpc.getDigitalWriteMethod = getDigitalWriteMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "DigitalWrite"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Gpio.BooleanMessage.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Response.getDefaultInstance()))
.setSchemaDescriptor(new GpioServiceMethodDescriptorSupplier("DigitalWrite"))
.build();
}
}
}
return getDigitalWriteMethod;
}
private static volatile io.grpc.MethodDescriptor getPwmReadMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "PwmRead",
requestType = com.diozero.remote.message.protobuf.Gpio.Identifier.class,
responseType = com.diozero.remote.message.protobuf.FloatResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getPwmReadMethod() {
io.grpc.MethodDescriptor getPwmReadMethod;
if ((getPwmReadMethod = GpioServiceGrpc.getPwmReadMethod) == null) {
synchronized (GpioServiceGrpc.class) {
if ((getPwmReadMethod = GpioServiceGrpc.getPwmReadMethod) == null) {
GpioServiceGrpc.getPwmReadMethod = getPwmReadMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "PwmRead"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Gpio.Identifier.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.FloatResponse.getDefaultInstance()))
.setSchemaDescriptor(new GpioServiceMethodDescriptorSupplier("PwmRead"))
.build();
}
}
}
return getPwmReadMethod;
}
private static volatile io.grpc.MethodDescriptor getPwmWriteMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "PwmWrite",
requestType = com.diozero.remote.message.protobuf.Gpio.FloatMessage.class,
responseType = com.diozero.remote.message.protobuf.Response.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getPwmWriteMethod() {
io.grpc.MethodDescriptor getPwmWriteMethod;
if ((getPwmWriteMethod = GpioServiceGrpc.getPwmWriteMethod) == null) {
synchronized (GpioServiceGrpc.class) {
if ((getPwmWriteMethod = GpioServiceGrpc.getPwmWriteMethod) == null) {
GpioServiceGrpc.getPwmWriteMethod = getPwmWriteMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "PwmWrite"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Gpio.FloatMessage.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Response.getDefaultInstance()))
.setSchemaDescriptor(new GpioServiceMethodDescriptorSupplier("PwmWrite"))
.build();
}
}
}
return getPwmWriteMethod;
}
private static volatile io.grpc.MethodDescriptor getServoReadMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ServoRead",
requestType = com.diozero.remote.message.protobuf.Gpio.Identifier.class,
responseType = com.diozero.remote.message.protobuf.IntegerResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getServoReadMethod() {
io.grpc.MethodDescriptor getServoReadMethod;
if ((getServoReadMethod = GpioServiceGrpc.getServoReadMethod) == null) {
synchronized (GpioServiceGrpc.class) {
if ((getServoReadMethod = GpioServiceGrpc.getServoReadMethod) == null) {
GpioServiceGrpc.getServoReadMethod = getServoReadMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ServoRead"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Gpio.Identifier.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.IntegerResponse.getDefaultInstance()))
.setSchemaDescriptor(new GpioServiceMethodDescriptorSupplier("ServoRead"))
.build();
}
}
}
return getServoReadMethod;
}
private static volatile io.grpc.MethodDescriptor getServoWriteMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ServoWrite",
requestType = com.diozero.remote.message.protobuf.Gpio.IntegerMessage.class,
responseType = com.diozero.remote.message.protobuf.Response.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getServoWriteMethod() {
io.grpc.MethodDescriptor getServoWriteMethod;
if ((getServoWriteMethod = GpioServiceGrpc.getServoWriteMethod) == null) {
synchronized (GpioServiceGrpc.class) {
if ((getServoWriteMethod = GpioServiceGrpc.getServoWriteMethod) == null) {
GpioServiceGrpc.getServoWriteMethod = getServoWriteMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ServoWrite"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Gpio.IntegerMessage.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Response.getDefaultInstance()))
.setSchemaDescriptor(new GpioServiceMethodDescriptorSupplier("ServoWrite"))
.build();
}
}
}
return getServoWriteMethod;
}
private static volatile io.grpc.MethodDescriptor getGetPwmFrequencyMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetPwmFrequency",
requestType = com.diozero.remote.message.protobuf.Gpio.Identifier.class,
responseType = com.diozero.remote.message.protobuf.IntegerResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetPwmFrequencyMethod() {
io.grpc.MethodDescriptor getGetPwmFrequencyMethod;
if ((getGetPwmFrequencyMethod = GpioServiceGrpc.getGetPwmFrequencyMethod) == null) {
synchronized (GpioServiceGrpc.class) {
if ((getGetPwmFrequencyMethod = GpioServiceGrpc.getGetPwmFrequencyMethod) == null) {
GpioServiceGrpc.getGetPwmFrequencyMethod = getGetPwmFrequencyMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetPwmFrequency"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Gpio.Identifier.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.IntegerResponse.getDefaultInstance()))
.setSchemaDescriptor(new GpioServiceMethodDescriptorSupplier("GetPwmFrequency"))
.build();
}
}
}
return getGetPwmFrequencyMethod;
}
private static volatile io.grpc.MethodDescriptor getSetPwmFrequencyMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "SetPwmFrequency",
requestType = com.diozero.remote.message.protobuf.Gpio.IntegerMessage.class,
responseType = com.diozero.remote.message.protobuf.Response.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getSetPwmFrequencyMethod() {
io.grpc.MethodDescriptor getSetPwmFrequencyMethod;
if ((getSetPwmFrequencyMethod = GpioServiceGrpc.getSetPwmFrequencyMethod) == null) {
synchronized (GpioServiceGrpc.class) {
if ((getSetPwmFrequencyMethod = GpioServiceGrpc.getSetPwmFrequencyMethod) == null) {
GpioServiceGrpc.getSetPwmFrequencyMethod = getSetPwmFrequencyMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "SetPwmFrequency"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Gpio.IntegerMessage.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Response.getDefaultInstance()))
.setSchemaDescriptor(new GpioServiceMethodDescriptorSupplier("SetPwmFrequency"))
.build();
}
}
}
return getSetPwmFrequencyMethod;
}
private static volatile io.grpc.MethodDescriptor getGetServoFrequencyMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetServoFrequency",
requestType = com.diozero.remote.message.protobuf.Gpio.Identifier.class,
responseType = com.diozero.remote.message.protobuf.IntegerResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetServoFrequencyMethod() {
io.grpc.MethodDescriptor getGetServoFrequencyMethod;
if ((getGetServoFrequencyMethod = GpioServiceGrpc.getGetServoFrequencyMethod) == null) {
synchronized (GpioServiceGrpc.class) {
if ((getGetServoFrequencyMethod = GpioServiceGrpc.getGetServoFrequencyMethod) == null) {
GpioServiceGrpc.getGetServoFrequencyMethod = getGetServoFrequencyMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetServoFrequency"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Gpio.Identifier.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.IntegerResponse.getDefaultInstance()))
.setSchemaDescriptor(new GpioServiceMethodDescriptorSupplier("GetServoFrequency"))
.build();
}
}
}
return getGetServoFrequencyMethod;
}
private static volatile io.grpc.MethodDescriptor getSetServoFrequencyMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "SetServoFrequency",
requestType = com.diozero.remote.message.protobuf.Gpio.IntegerMessage.class,
responseType = com.diozero.remote.message.protobuf.Response.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getSetServoFrequencyMethod() {
io.grpc.MethodDescriptor getSetServoFrequencyMethod;
if ((getSetServoFrequencyMethod = GpioServiceGrpc.getSetServoFrequencyMethod) == null) {
synchronized (GpioServiceGrpc.class) {
if ((getSetServoFrequencyMethod = GpioServiceGrpc.getSetServoFrequencyMethod) == null) {
GpioServiceGrpc.getSetServoFrequencyMethod = getSetServoFrequencyMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "SetServoFrequency"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Gpio.IntegerMessage.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Response.getDefaultInstance()))
.setSchemaDescriptor(new GpioServiceMethodDescriptorSupplier("SetServoFrequency"))
.build();
}
}
}
return getSetServoFrequencyMethod;
}
private static volatile io.grpc.MethodDescriptor getAnalogReadMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "AnalogRead",
requestType = com.diozero.remote.message.protobuf.Gpio.Identifier.class,
responseType = com.diozero.remote.message.protobuf.FloatResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getAnalogReadMethod() {
io.grpc.MethodDescriptor getAnalogReadMethod;
if ((getAnalogReadMethod = GpioServiceGrpc.getAnalogReadMethod) == null) {
synchronized (GpioServiceGrpc.class) {
if ((getAnalogReadMethod = GpioServiceGrpc.getAnalogReadMethod) == null) {
GpioServiceGrpc.getAnalogReadMethod = getAnalogReadMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "AnalogRead"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Gpio.Identifier.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.FloatResponse.getDefaultInstance()))
.setSchemaDescriptor(new GpioServiceMethodDescriptorSupplier("AnalogRead"))
.build();
}
}
}
return getAnalogReadMethod;
}
private static volatile io.grpc.MethodDescriptor getAnalogWriteMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "AnalogWrite",
requestType = com.diozero.remote.message.protobuf.Gpio.FloatMessage.class,
responseType = com.diozero.remote.message.protobuf.Response.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getAnalogWriteMethod() {
io.grpc.MethodDescriptor getAnalogWriteMethod;
if ((getAnalogWriteMethod = GpioServiceGrpc.getAnalogWriteMethod) == null) {
synchronized (GpioServiceGrpc.class) {
if ((getAnalogWriteMethod = GpioServiceGrpc.getAnalogWriteMethod) == null) {
GpioServiceGrpc.getAnalogWriteMethod = getAnalogWriteMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "AnalogWrite"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Gpio.FloatMessage.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Response.getDefaultInstance()))
.setSchemaDescriptor(new GpioServiceMethodDescriptorSupplier("AnalogWrite"))
.build();
}
}
}
return getAnalogWriteMethod;
}
private static volatile io.grpc.MethodDescriptor getSetOutputMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "SetOutput",
requestType = com.diozero.remote.message.protobuf.Gpio.BooleanMessage.class,
responseType = com.diozero.remote.message.protobuf.Response.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getSetOutputMethod() {
io.grpc.MethodDescriptor getSetOutputMethod;
if ((getSetOutputMethod = GpioServiceGrpc.getSetOutputMethod) == null) {
synchronized (GpioServiceGrpc.class) {
if ((getSetOutputMethod = GpioServiceGrpc.getSetOutputMethod) == null) {
GpioServiceGrpc.getSetOutputMethod = getSetOutputMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "SetOutput"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Gpio.BooleanMessage.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Response.getDefaultInstance()))
.setSchemaDescriptor(new GpioServiceMethodDescriptorSupplier("SetOutput"))
.build();
}
}
}
return getSetOutputMethod;
}
private static volatile io.grpc.MethodDescriptor getSubscribeMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Subscribe",
requestType = com.diozero.remote.message.protobuf.Gpio.Identifier.class,
responseType = com.diozero.remote.message.protobuf.Gpio.Event.class,
methodType = io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING)
public static io.grpc.MethodDescriptor getSubscribeMethod() {
io.grpc.MethodDescriptor getSubscribeMethod;
if ((getSubscribeMethod = GpioServiceGrpc.getSubscribeMethod) == null) {
synchronized (GpioServiceGrpc.class) {
if ((getSubscribeMethod = GpioServiceGrpc.getSubscribeMethod) == null) {
GpioServiceGrpc.getSubscribeMethod = getSubscribeMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "Subscribe"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Gpio.Identifier.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Gpio.Event.getDefaultInstance()))
.setSchemaDescriptor(new GpioServiceMethodDescriptorSupplier("Subscribe"))
.build();
}
}
}
return getSubscribeMethod;
}
private static volatile io.grpc.MethodDescriptor getUnsubscribeMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Unsubscribe",
requestType = com.diozero.remote.message.protobuf.Gpio.Identifier.class,
responseType = com.diozero.remote.message.protobuf.Response.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getUnsubscribeMethod() {
io.grpc.MethodDescriptor getUnsubscribeMethod;
if ((getUnsubscribeMethod = GpioServiceGrpc.getUnsubscribeMethod) == null) {
synchronized (GpioServiceGrpc.class) {
if ((getUnsubscribeMethod = GpioServiceGrpc.getUnsubscribeMethod) == null) {
GpioServiceGrpc.getUnsubscribeMethod = getUnsubscribeMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "Unsubscribe"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Gpio.Identifier.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Response.getDefaultInstance()))
.setSchemaDescriptor(new GpioServiceMethodDescriptorSupplier("Unsubscribe"))
.build();
}
}
}
return getUnsubscribeMethod;
}
private static volatile io.grpc.MethodDescriptor getCloseMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Close",
requestType = com.diozero.remote.message.protobuf.Gpio.Identifier.class,
responseType = com.diozero.remote.message.protobuf.Response.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getCloseMethod() {
io.grpc.MethodDescriptor getCloseMethod;
if ((getCloseMethod = GpioServiceGrpc.getCloseMethod) == null) {
synchronized (GpioServiceGrpc.class) {
if ((getCloseMethod = GpioServiceGrpc.getCloseMethod) == null) {
GpioServiceGrpc.getCloseMethod = getCloseMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "Close"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Gpio.Identifier.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Response.getDefaultInstance()))
.setSchemaDescriptor(new GpioServiceMethodDescriptorSupplier("Close"))
.build();
}
}
}
return getCloseMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static GpioServiceStub newStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public GpioServiceStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new GpioServiceStub(channel, callOptions);
}
};
return GpioServiceStub.newStub(factory, channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static GpioServiceBlockingStub newBlockingStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public GpioServiceBlockingStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new GpioServiceBlockingStub(channel, callOptions);
}
};
return GpioServiceBlockingStub.newStub(factory, channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static GpioServiceFutureStub newFutureStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public GpioServiceFutureStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new GpioServiceFutureStub(channel, callOptions);
}
};
return GpioServiceFutureStub.newStub(factory, channel);
}
/**
*/
public interface AsyncService {
/**
*/
default void provisionDigitalInputDevice(com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getProvisionDigitalInputDeviceMethod(), responseObserver);
}
/**
*/
default void provisionDigitalOutputDevice(com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getProvisionDigitalOutputDeviceMethod(), responseObserver);
}
/**
*/
default void provisionDigitalInputOutputDevice(com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getProvisionDigitalInputOutputDeviceMethod(), responseObserver);
}
/**
*/
default void provisionPwmOutputDevice(com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getProvisionPwmOutputDeviceMethod(), responseObserver);
}
/**
*/
default void provisionServoDevice(com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getProvisionServoDeviceMethod(), responseObserver);
}
/**
*/
default void provisionAnalogInputDevice(com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getProvisionAnalogInputDeviceMethod(), responseObserver);
}
/**
*/
default void provisionAnalogOutputDevice(com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getProvisionAnalogOutputDeviceMethod(), responseObserver);
}
/**
*/
default void digitalRead(com.diozero.remote.message.protobuf.Gpio.Identifier request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getDigitalReadMethod(), responseObserver);
}
/**
*/
default void digitalWrite(com.diozero.remote.message.protobuf.Gpio.BooleanMessage request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getDigitalWriteMethod(), responseObserver);
}
/**
*/
default void pwmRead(com.diozero.remote.message.protobuf.Gpio.Identifier request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getPwmReadMethod(), responseObserver);
}
/**
*/
default void pwmWrite(com.diozero.remote.message.protobuf.Gpio.FloatMessage request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getPwmWriteMethod(), responseObserver);
}
/**
*/
default void servoRead(com.diozero.remote.message.protobuf.Gpio.Identifier request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getServoReadMethod(), responseObserver);
}
/**
*/
default void servoWrite(com.diozero.remote.message.protobuf.Gpio.IntegerMessage request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getServoWriteMethod(), responseObserver);
}
/**
*/
default void getPwmFrequency(com.diozero.remote.message.protobuf.Gpio.Identifier request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetPwmFrequencyMethod(), responseObserver);
}
/**
*/
default void setPwmFrequency(com.diozero.remote.message.protobuf.Gpio.IntegerMessage request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getSetPwmFrequencyMethod(), responseObserver);
}
/**
*/
default void getServoFrequency(com.diozero.remote.message.protobuf.Gpio.Identifier request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetServoFrequencyMethod(), responseObserver);
}
/**
*/
default void setServoFrequency(com.diozero.remote.message.protobuf.Gpio.IntegerMessage request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getSetServoFrequencyMethod(), responseObserver);
}
/**
*/
default void analogRead(com.diozero.remote.message.protobuf.Gpio.Identifier request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getAnalogReadMethod(), responseObserver);
}
/**
*/
default void analogWrite(com.diozero.remote.message.protobuf.Gpio.FloatMessage request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getAnalogWriteMethod(), responseObserver);
}
/**
*/
default void setOutput(com.diozero.remote.message.protobuf.Gpio.BooleanMessage request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getSetOutputMethod(), responseObserver);
}
/**
*/
default void subscribe(com.diozero.remote.message.protobuf.Gpio.Identifier request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getSubscribeMethod(), responseObserver);
}
/**
*/
default void unsubscribe(com.diozero.remote.message.protobuf.Gpio.Identifier request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getUnsubscribeMethod(), responseObserver);
}
/**
*/
default void close(com.diozero.remote.message.protobuf.Gpio.Identifier request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getCloseMethod(), responseObserver);
}
}
/**
* Base class for the server implementation of the service GpioService.
*/
public static abstract class GpioServiceImplBase
implements io.grpc.BindableService, AsyncService {
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return GpioServiceGrpc.bindService(this);
}
}
/**
* A stub to allow clients to do asynchronous rpc calls to service GpioService.
*/
public static final class GpioServiceStub
extends io.grpc.stub.AbstractAsyncStub {
private GpioServiceStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected GpioServiceStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new GpioServiceStub(channel, callOptions);
}
/**
*/
public void provisionDigitalInputDevice(com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getProvisionDigitalInputDeviceMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void provisionDigitalOutputDevice(com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getProvisionDigitalOutputDeviceMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void provisionDigitalInputOutputDevice(com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getProvisionDigitalInputOutputDeviceMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void provisionPwmOutputDevice(com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getProvisionPwmOutputDeviceMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void provisionServoDevice(com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getProvisionServoDeviceMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void provisionAnalogInputDevice(com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getProvisionAnalogInputDeviceMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void provisionAnalogOutputDevice(com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getProvisionAnalogOutputDeviceMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void digitalRead(com.diozero.remote.message.protobuf.Gpio.Identifier request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getDigitalReadMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void digitalWrite(com.diozero.remote.message.protobuf.Gpio.BooleanMessage request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getDigitalWriteMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void pwmRead(com.diozero.remote.message.protobuf.Gpio.Identifier request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getPwmReadMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void pwmWrite(com.diozero.remote.message.protobuf.Gpio.FloatMessage request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getPwmWriteMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void servoRead(com.diozero.remote.message.protobuf.Gpio.Identifier request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getServoReadMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void servoWrite(com.diozero.remote.message.protobuf.Gpio.IntegerMessage request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getServoWriteMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void getPwmFrequency(com.diozero.remote.message.protobuf.Gpio.Identifier request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGetPwmFrequencyMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void setPwmFrequency(com.diozero.remote.message.protobuf.Gpio.IntegerMessage request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getSetPwmFrequencyMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void getServoFrequency(com.diozero.remote.message.protobuf.Gpio.Identifier request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGetServoFrequencyMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void setServoFrequency(com.diozero.remote.message.protobuf.Gpio.IntegerMessage request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getSetServoFrequencyMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void analogRead(com.diozero.remote.message.protobuf.Gpio.Identifier request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getAnalogReadMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void analogWrite(com.diozero.remote.message.protobuf.Gpio.FloatMessage request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getAnalogWriteMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void setOutput(com.diozero.remote.message.protobuf.Gpio.BooleanMessage request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getSetOutputMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void subscribe(com.diozero.remote.message.protobuf.Gpio.Identifier request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncServerStreamingCall(
getChannel().newCall(getSubscribeMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void unsubscribe(com.diozero.remote.message.protobuf.Gpio.Identifier request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getUnsubscribeMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void close(com.diozero.remote.message.protobuf.Gpio.Identifier request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getCloseMethod(), getCallOptions()), request, responseObserver);
}
}
/**
* A stub to allow clients to do synchronous rpc calls to service GpioService.
*/
public static final class GpioServiceBlockingStub
extends io.grpc.stub.AbstractBlockingStub {
private GpioServiceBlockingStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected GpioServiceBlockingStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new GpioServiceBlockingStub(channel, callOptions);
}
/**
*/
public com.diozero.remote.message.protobuf.Response provisionDigitalInputDevice(com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getProvisionDigitalInputDeviceMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.Response provisionDigitalOutputDevice(com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getProvisionDigitalOutputDeviceMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.Response provisionDigitalInputOutputDevice(com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getProvisionDigitalInputOutputDeviceMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.Response provisionPwmOutputDevice(com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getProvisionPwmOutputDeviceMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.Response provisionServoDevice(com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getProvisionServoDeviceMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.Response provisionAnalogInputDevice(com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getProvisionAnalogInputDeviceMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.Response provisionAnalogOutputDevice(com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getProvisionAnalogOutputDeviceMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.BooleanResponse digitalRead(com.diozero.remote.message.protobuf.Gpio.Identifier request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getDigitalReadMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.Response digitalWrite(com.diozero.remote.message.protobuf.Gpio.BooleanMessage request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getDigitalWriteMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.FloatResponse pwmRead(com.diozero.remote.message.protobuf.Gpio.Identifier request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getPwmReadMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.Response pwmWrite(com.diozero.remote.message.protobuf.Gpio.FloatMessage request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getPwmWriteMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.IntegerResponse servoRead(com.diozero.remote.message.protobuf.Gpio.Identifier request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getServoReadMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.Response servoWrite(com.diozero.remote.message.protobuf.Gpio.IntegerMessage request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getServoWriteMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.IntegerResponse getPwmFrequency(com.diozero.remote.message.protobuf.Gpio.Identifier request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGetPwmFrequencyMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.Response setPwmFrequency(com.diozero.remote.message.protobuf.Gpio.IntegerMessage request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getSetPwmFrequencyMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.IntegerResponse getServoFrequency(com.diozero.remote.message.protobuf.Gpio.Identifier request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGetServoFrequencyMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.Response setServoFrequency(com.diozero.remote.message.protobuf.Gpio.IntegerMessage request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getSetServoFrequencyMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.FloatResponse analogRead(com.diozero.remote.message.protobuf.Gpio.Identifier request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getAnalogReadMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.Response analogWrite(com.diozero.remote.message.protobuf.Gpio.FloatMessage request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getAnalogWriteMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.Response setOutput(com.diozero.remote.message.protobuf.Gpio.BooleanMessage request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getSetOutputMethod(), getCallOptions(), request);
}
/**
*/
public java.util.Iterator subscribe(
com.diozero.remote.message.protobuf.Gpio.Identifier request) {
return io.grpc.stub.ClientCalls.blockingServerStreamingCall(
getChannel(), getSubscribeMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.Response unsubscribe(com.diozero.remote.message.protobuf.Gpio.Identifier request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getUnsubscribeMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.Response close(com.diozero.remote.message.protobuf.Gpio.Identifier request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getCloseMethod(), getCallOptions(), request);
}
}
/**
* A stub to allow clients to do ListenableFuture-style rpc calls to service GpioService.
*/
public static final class GpioServiceFutureStub
extends io.grpc.stub.AbstractFutureStub {
private GpioServiceFutureStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected GpioServiceFutureStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new GpioServiceFutureStub(channel, callOptions);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture provisionDigitalInputDevice(
com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getProvisionDigitalInputDeviceMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture provisionDigitalOutputDevice(
com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getProvisionDigitalOutputDeviceMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture provisionDigitalInputOutputDevice(
com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getProvisionDigitalInputOutputDeviceMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture provisionPwmOutputDevice(
com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getProvisionPwmOutputDeviceMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture provisionServoDevice(
com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getProvisionServoDeviceMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture provisionAnalogInputDevice(
com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getProvisionAnalogInputDeviceMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture provisionAnalogOutputDevice(
com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getProvisionAnalogOutputDeviceMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture digitalRead(
com.diozero.remote.message.protobuf.Gpio.Identifier request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getDigitalReadMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture digitalWrite(
com.diozero.remote.message.protobuf.Gpio.BooleanMessage request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getDigitalWriteMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture pwmRead(
com.diozero.remote.message.protobuf.Gpio.Identifier request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getPwmReadMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture pwmWrite(
com.diozero.remote.message.protobuf.Gpio.FloatMessage request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getPwmWriteMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture servoRead(
com.diozero.remote.message.protobuf.Gpio.Identifier request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getServoReadMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture servoWrite(
com.diozero.remote.message.protobuf.Gpio.IntegerMessage request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getServoWriteMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture getPwmFrequency(
com.diozero.remote.message.protobuf.Gpio.Identifier request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getGetPwmFrequencyMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture setPwmFrequency(
com.diozero.remote.message.protobuf.Gpio.IntegerMessage request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getSetPwmFrequencyMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture getServoFrequency(
com.diozero.remote.message.protobuf.Gpio.Identifier request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getGetServoFrequencyMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture setServoFrequency(
com.diozero.remote.message.protobuf.Gpio.IntegerMessage request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getSetServoFrequencyMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture analogRead(
com.diozero.remote.message.protobuf.Gpio.Identifier request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getAnalogReadMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture analogWrite(
com.diozero.remote.message.protobuf.Gpio.FloatMessage request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getAnalogWriteMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture setOutput(
com.diozero.remote.message.protobuf.Gpio.BooleanMessage request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getSetOutputMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture unsubscribe(
com.diozero.remote.message.protobuf.Gpio.Identifier request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getUnsubscribeMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture close(
com.diozero.remote.message.protobuf.Gpio.Identifier request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getCloseMethod(), getCallOptions()), request);
}
}
private static final int METHODID_PROVISION_DIGITAL_INPUT_DEVICE = 0;
private static final int METHODID_PROVISION_DIGITAL_OUTPUT_DEVICE = 1;
private static final int METHODID_PROVISION_DIGITAL_INPUT_OUTPUT_DEVICE = 2;
private static final int METHODID_PROVISION_PWM_OUTPUT_DEVICE = 3;
private static final int METHODID_PROVISION_SERVO_DEVICE = 4;
private static final int METHODID_PROVISION_ANALOG_INPUT_DEVICE = 5;
private static final int METHODID_PROVISION_ANALOG_OUTPUT_DEVICE = 6;
private static final int METHODID_DIGITAL_READ = 7;
private static final int METHODID_DIGITAL_WRITE = 8;
private static final int METHODID_PWM_READ = 9;
private static final int METHODID_PWM_WRITE = 10;
private static final int METHODID_SERVO_READ = 11;
private static final int METHODID_SERVO_WRITE = 12;
private static final int METHODID_GET_PWM_FREQUENCY = 13;
private static final int METHODID_SET_PWM_FREQUENCY = 14;
private static final int METHODID_GET_SERVO_FREQUENCY = 15;
private static final int METHODID_SET_SERVO_FREQUENCY = 16;
private static final int METHODID_ANALOG_READ = 17;
private static final int METHODID_ANALOG_WRITE = 18;
private static final int METHODID_SET_OUTPUT = 19;
private static final int METHODID_SUBSCRIBE = 20;
private static final int METHODID_UNSUBSCRIBE = 21;
private static final int METHODID_CLOSE = 22;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final AsyncService serviceImpl;
private final int methodId;
MethodHandlers(AsyncService serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_PROVISION_DIGITAL_INPUT_DEVICE:
serviceImpl.provisionDigitalInputDevice((com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_PROVISION_DIGITAL_OUTPUT_DEVICE:
serviceImpl.provisionDigitalOutputDevice((com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_PROVISION_DIGITAL_INPUT_OUTPUT_DEVICE:
serviceImpl.provisionDigitalInputOutputDevice((com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_PROVISION_PWM_OUTPUT_DEVICE:
serviceImpl.provisionPwmOutputDevice((com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_PROVISION_SERVO_DEVICE:
serviceImpl.provisionServoDevice((com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_PROVISION_ANALOG_INPUT_DEVICE:
serviceImpl.provisionAnalogInputDevice((com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_PROVISION_ANALOG_OUTPUT_DEVICE:
serviceImpl.provisionAnalogOutputDevice((com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_DIGITAL_READ:
serviceImpl.digitalRead((com.diozero.remote.message.protobuf.Gpio.Identifier) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_DIGITAL_WRITE:
serviceImpl.digitalWrite((com.diozero.remote.message.protobuf.Gpio.BooleanMessage) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_PWM_READ:
serviceImpl.pwmRead((com.diozero.remote.message.protobuf.Gpio.Identifier) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_PWM_WRITE:
serviceImpl.pwmWrite((com.diozero.remote.message.protobuf.Gpio.FloatMessage) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_SERVO_READ:
serviceImpl.servoRead((com.diozero.remote.message.protobuf.Gpio.Identifier) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_SERVO_WRITE:
serviceImpl.servoWrite((com.diozero.remote.message.protobuf.Gpio.IntegerMessage) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_PWM_FREQUENCY:
serviceImpl.getPwmFrequency((com.diozero.remote.message.protobuf.Gpio.Identifier) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_SET_PWM_FREQUENCY:
serviceImpl.setPwmFrequency((com.diozero.remote.message.protobuf.Gpio.IntegerMessage) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_SERVO_FREQUENCY:
serviceImpl.getServoFrequency((com.diozero.remote.message.protobuf.Gpio.Identifier) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_SET_SERVO_FREQUENCY:
serviceImpl.setServoFrequency((com.diozero.remote.message.protobuf.Gpio.IntegerMessage) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_ANALOG_READ:
serviceImpl.analogRead((com.diozero.remote.message.protobuf.Gpio.Identifier) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_ANALOG_WRITE:
serviceImpl.analogWrite((com.diozero.remote.message.protobuf.Gpio.FloatMessage) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_SET_OUTPUT:
serviceImpl.setOutput((com.diozero.remote.message.protobuf.Gpio.BooleanMessage) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_SUBSCRIBE:
serviceImpl.subscribe((com.diozero.remote.message.protobuf.Gpio.Identifier) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_UNSUBSCRIBE:
serviceImpl.unsubscribe((com.diozero.remote.message.protobuf.Gpio.Identifier) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_CLOSE:
serviceImpl.close((com.diozero.remote.message.protobuf.Gpio.Identifier) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new AssertionError();
}
}
}
public static final io.grpc.ServerServiceDefinition bindService(AsyncService service) {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
getProvisionDigitalInputDeviceMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputDeviceRequest,
com.diozero.remote.message.protobuf.Response>(
service, METHODID_PROVISION_DIGITAL_INPUT_DEVICE)))
.addMethod(
getProvisionDigitalOutputDeviceMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalOutputDeviceRequest,
com.diozero.remote.message.protobuf.Response>(
service, METHODID_PROVISION_DIGITAL_OUTPUT_DEVICE)))
.addMethod(
getProvisionDigitalInputOutputDeviceMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.Gpio.ProvisionDigitalInputOutputDeviceRequest,
com.diozero.remote.message.protobuf.Response>(
service, METHODID_PROVISION_DIGITAL_INPUT_OUTPUT_DEVICE)))
.addMethod(
getProvisionPwmOutputDeviceMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.Gpio.ProvisionPwmOutputDeviceRequest,
com.diozero.remote.message.protobuf.Response>(
service, METHODID_PROVISION_PWM_OUTPUT_DEVICE)))
.addMethod(
getProvisionServoDeviceMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.Gpio.ProvisionServoDeviceRequest,
com.diozero.remote.message.protobuf.Response>(
service, METHODID_PROVISION_SERVO_DEVICE)))
.addMethod(
getProvisionAnalogInputDeviceMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogInputDeviceRequest,
com.diozero.remote.message.protobuf.Response>(
service, METHODID_PROVISION_ANALOG_INPUT_DEVICE)))
.addMethod(
getProvisionAnalogOutputDeviceMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.Gpio.ProvisionAnalogOutputDeviceRequest,
com.diozero.remote.message.protobuf.Response>(
service, METHODID_PROVISION_ANALOG_OUTPUT_DEVICE)))
.addMethod(
getDigitalReadMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.Gpio.Identifier,
com.diozero.remote.message.protobuf.BooleanResponse>(
service, METHODID_DIGITAL_READ)))
.addMethod(
getDigitalWriteMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.Gpio.BooleanMessage,
com.diozero.remote.message.protobuf.Response>(
service, METHODID_DIGITAL_WRITE)))
.addMethod(
getPwmReadMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.Gpio.Identifier,
com.diozero.remote.message.protobuf.FloatResponse>(
service, METHODID_PWM_READ)))
.addMethod(
getPwmWriteMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.Gpio.FloatMessage,
com.diozero.remote.message.protobuf.Response>(
service, METHODID_PWM_WRITE)))
.addMethod(
getServoReadMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.Gpio.Identifier,
com.diozero.remote.message.protobuf.IntegerResponse>(
service, METHODID_SERVO_READ)))
.addMethod(
getServoWriteMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.Gpio.IntegerMessage,
com.diozero.remote.message.protobuf.Response>(
service, METHODID_SERVO_WRITE)))
.addMethod(
getGetPwmFrequencyMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.Gpio.Identifier,
com.diozero.remote.message.protobuf.IntegerResponse>(
service, METHODID_GET_PWM_FREQUENCY)))
.addMethod(
getSetPwmFrequencyMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.Gpio.IntegerMessage,
com.diozero.remote.message.protobuf.Response>(
service, METHODID_SET_PWM_FREQUENCY)))
.addMethod(
getGetServoFrequencyMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.Gpio.Identifier,
com.diozero.remote.message.protobuf.IntegerResponse>(
service, METHODID_GET_SERVO_FREQUENCY)))
.addMethod(
getSetServoFrequencyMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.Gpio.IntegerMessage,
com.diozero.remote.message.protobuf.Response>(
service, METHODID_SET_SERVO_FREQUENCY)))
.addMethod(
getAnalogReadMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.Gpio.Identifier,
com.diozero.remote.message.protobuf.FloatResponse>(
service, METHODID_ANALOG_READ)))
.addMethod(
getAnalogWriteMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.Gpio.FloatMessage,
com.diozero.remote.message.protobuf.Response>(
service, METHODID_ANALOG_WRITE)))
.addMethod(
getSetOutputMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.Gpio.BooleanMessage,
com.diozero.remote.message.protobuf.Response>(
service, METHODID_SET_OUTPUT)))
.addMethod(
getSubscribeMethod(),
io.grpc.stub.ServerCalls.asyncServerStreamingCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.Gpio.Identifier,
com.diozero.remote.message.protobuf.Gpio.Event>(
service, METHODID_SUBSCRIBE)))
.addMethod(
getUnsubscribeMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.Gpio.Identifier,
com.diozero.remote.message.protobuf.Response>(
service, METHODID_UNSUBSCRIBE)))
.addMethod(
getCloseMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.Gpio.Identifier,
com.diozero.remote.message.protobuf.Response>(
service, METHODID_CLOSE)))
.build();
}
private static abstract class GpioServiceBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoFileDescriptorSupplier, io.grpc.protobuf.ProtoServiceDescriptorSupplier {
GpioServiceBaseDescriptorSupplier() {}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("GpioService");
}
}
private static final class GpioServiceFileDescriptorSupplier
extends GpioServiceBaseDescriptorSupplier {
GpioServiceFileDescriptorSupplier() {}
}
private static final class GpioServiceMethodDescriptorSupplier
extends GpioServiceBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final java.lang.String methodName;
GpioServiceMethodDescriptorSupplier(java.lang.String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (GpioServiceGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new GpioServiceFileDescriptorSupplier())
.addMethod(getProvisionDigitalInputDeviceMethod())
.addMethod(getProvisionDigitalOutputDeviceMethod())
.addMethod(getProvisionDigitalInputOutputDeviceMethod())
.addMethod(getProvisionPwmOutputDeviceMethod())
.addMethod(getProvisionServoDeviceMethod())
.addMethod(getProvisionAnalogInputDeviceMethod())
.addMethod(getProvisionAnalogOutputDeviceMethod())
.addMethod(getDigitalReadMethod())
.addMethod(getDigitalWriteMethod())
.addMethod(getPwmReadMethod())
.addMethod(getPwmWriteMethod())
.addMethod(getServoReadMethod())
.addMethod(getServoWriteMethod())
.addMethod(getGetPwmFrequencyMethod())
.addMethod(getSetPwmFrequencyMethod())
.addMethod(getGetServoFrequencyMethod())
.addMethod(getSetServoFrequencyMethod())
.addMethod(getAnalogReadMethod())
.addMethod(getAnalogWriteMethod())
.addMethod(getSetOutputMethod())
.addMethod(getSubscribeMethod())
.addMethod(getUnsubscribeMethod())
.addMethod(getCloseMethod())
.build();
}
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy