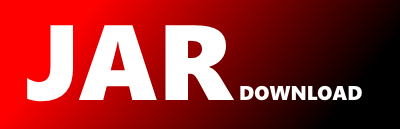
com.diozero.remote.message.protobuf.I2C Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// NO CHECKED-IN PROTOBUF GENCODE
// source: diozero.proto
// Protobuf Java Version: 4.28.2
package com.diozero.remote.message.protobuf;
/**
* Protobuf type {@code diozero.I2C}
*/
public final class I2C extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:diozero.I2C)
I2COrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
I2C.class.getName());
}
// Use I2C.newBuilder() to construct.
private I2C(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private I2C() {
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.I2C.class, com.diozero.remote.message.protobuf.I2C.Builder.class);
}
/**
* Protobuf enum {@code diozero.I2C.ProbeMode}
*/
public enum ProbeMode
implements com.google.protobuf.ProtocolMessageEnum {
/**
* QUICK = 0;
*/
QUICK(0),
/**
* READ = 1;
*/
READ(1),
/**
* AUTO = 2;
*/
AUTO(2),
UNRECOGNIZED(-1),
;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
ProbeMode.class.getName());
}
/**
* QUICK = 0;
*/
public static final int QUICK_VALUE = 0;
/**
* READ = 1;
*/
public static final int READ_VALUE = 1;
/**
* AUTO = 2;
*/
public static final int AUTO_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ProbeMode valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static ProbeMode forNumber(int value) {
switch (value) {
case 0: return QUICK;
case 1: return READ;
case 2: return AUTO;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
ProbeMode> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ProbeMode findValueByNumber(int number) {
return ProbeMode.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.I2C.getDescriptor().getEnumTypes().get(0);
}
private static final ProbeMode[] VALUES = values();
public static ProbeMode valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private ProbeMode(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:diozero.I2C.ProbeMode)
}
public interface IdentifierOrBuilder extends
// @@protoc_insertion_point(interface_extends:diozero.I2C.Identifier)
com.google.protobuf.MessageOrBuilder {
/**
* int32 controller = 1;
* @return The controller.
*/
int getController();
/**
* int32 address = 2;
* @return The address.
*/
int getAddress();
}
/**
* Protobuf type {@code diozero.I2C.Identifier}
*/
public static final class Identifier extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:diozero.I2C.Identifier)
IdentifierOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
Identifier.class.getName());
}
// Use Identifier.newBuilder() to construct.
private Identifier(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Identifier() {
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_Identifier_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_Identifier_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.I2C.Identifier.class, com.diozero.remote.message.protobuf.I2C.Identifier.Builder.class);
}
public static final int CONTROLLER_FIELD_NUMBER = 1;
private int controller_ = 0;
/**
* int32 controller = 1;
* @return The controller.
*/
@java.lang.Override
public int getController() {
return controller_;
}
public static final int ADDRESS_FIELD_NUMBER = 2;
private int address_ = 0;
/**
* int32 address = 2;
* @return The address.
*/
@java.lang.Override
public int getAddress() {
return address_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (controller_ != 0) {
output.writeInt32(1, controller_);
}
if (address_ != 0) {
output.writeInt32(2, address_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (controller_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, controller_);
}
if (address_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, address_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.diozero.remote.message.protobuf.I2C.Identifier)) {
return super.equals(obj);
}
com.diozero.remote.message.protobuf.I2C.Identifier other = (com.diozero.remote.message.protobuf.I2C.Identifier) obj;
if (getController()
!= other.getController()) return false;
if (getAddress()
!= other.getAddress()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CONTROLLER_FIELD_NUMBER;
hash = (53 * hash) + getController();
hash = (37 * hash) + ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getAddress();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.diozero.remote.message.protobuf.I2C.Identifier parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.Identifier parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.Identifier parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.Identifier parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.Identifier parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.Identifier parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.Identifier parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.Identifier parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.Identifier parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.Identifier parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.Identifier parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.Identifier parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.diozero.remote.message.protobuf.I2C.Identifier prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code diozero.I2C.Identifier}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:diozero.I2C.Identifier)
com.diozero.remote.message.protobuf.I2C.IdentifierOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_Identifier_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_Identifier_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.I2C.Identifier.class, com.diozero.remote.message.protobuf.I2C.Identifier.Builder.class);
}
// Construct using com.diozero.remote.message.protobuf.I2C.Identifier.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
controller_ = 0;
address_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_Identifier_descriptor;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.Identifier getDefaultInstanceForType() {
return com.diozero.remote.message.protobuf.I2C.Identifier.getDefaultInstance();
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.Identifier build() {
com.diozero.remote.message.protobuf.I2C.Identifier result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.Identifier buildPartial() {
com.diozero.remote.message.protobuf.I2C.Identifier result = new com.diozero.remote.message.protobuf.I2C.Identifier(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.diozero.remote.message.protobuf.I2C.Identifier result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.controller_ = controller_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.address_ = address_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.diozero.remote.message.protobuf.I2C.Identifier) {
return mergeFrom((com.diozero.remote.message.protobuf.I2C.Identifier)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.diozero.remote.message.protobuf.I2C.Identifier other) {
if (other == com.diozero.remote.message.protobuf.I2C.Identifier.getDefaultInstance()) return this;
if (other.getController() != 0) {
setController(other.getController());
}
if (other.getAddress() != 0) {
setAddress(other.getAddress());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
controller_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
address_ = input.readInt32();
bitField0_ |= 0x00000002;
break;
} // case 16
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int controller_ ;
/**
* int32 controller = 1;
* @return The controller.
*/
@java.lang.Override
public int getController() {
return controller_;
}
/**
* int32 controller = 1;
* @param value The controller to set.
* @return This builder for chaining.
*/
public Builder setController(int value) {
controller_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* int32 controller = 1;
* @return This builder for chaining.
*/
public Builder clearController() {
bitField0_ = (bitField0_ & ~0x00000001);
controller_ = 0;
onChanged();
return this;
}
private int address_ ;
/**
* int32 address = 2;
* @return The address.
*/
@java.lang.Override
public int getAddress() {
return address_;
}
/**
* int32 address = 2;
* @param value The address to set.
* @return This builder for chaining.
*/
public Builder setAddress(int value) {
address_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* int32 address = 2;
* @return This builder for chaining.
*/
public Builder clearAddress() {
bitField0_ = (bitField0_ & ~0x00000002);
address_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:diozero.I2C.Identifier)
}
// @@protoc_insertion_point(class_scope:diozero.I2C.Identifier)
private static final com.diozero.remote.message.protobuf.I2C.Identifier DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.diozero.remote.message.protobuf.I2C.Identifier();
}
public static com.diozero.remote.message.protobuf.I2C.Identifier getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Identifier parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.Identifier getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface OpenOrBuilder extends
// @@protoc_insertion_point(interface_extends:diozero.I2C.Open)
com.google.protobuf.MessageOrBuilder {
/**
* int32 controller = 1;
* @return The controller.
*/
int getController();
/**
* int32 address = 2;
* @return The address.
*/
int getAddress();
/**
* int32 addressSize = 3;
* @return The addressSize.
*/
int getAddressSize();
}
/**
* Protobuf type {@code diozero.I2C.Open}
*/
public static final class Open extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:diozero.I2C.Open)
OpenOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
Open.class.getName());
}
// Use Open.newBuilder() to construct.
private Open(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Open() {
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_Open_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_Open_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.I2C.Open.class, com.diozero.remote.message.protobuf.I2C.Open.Builder.class);
}
public static final int CONTROLLER_FIELD_NUMBER = 1;
private int controller_ = 0;
/**
* int32 controller = 1;
* @return The controller.
*/
@java.lang.Override
public int getController() {
return controller_;
}
public static final int ADDRESS_FIELD_NUMBER = 2;
private int address_ = 0;
/**
* int32 address = 2;
* @return The address.
*/
@java.lang.Override
public int getAddress() {
return address_;
}
public static final int ADDRESSSIZE_FIELD_NUMBER = 3;
private int addressSize_ = 0;
/**
* int32 addressSize = 3;
* @return The addressSize.
*/
@java.lang.Override
public int getAddressSize() {
return addressSize_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (controller_ != 0) {
output.writeInt32(1, controller_);
}
if (address_ != 0) {
output.writeInt32(2, address_);
}
if (addressSize_ != 0) {
output.writeInt32(3, addressSize_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (controller_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, controller_);
}
if (address_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, address_);
}
if (addressSize_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, addressSize_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.diozero.remote.message.protobuf.I2C.Open)) {
return super.equals(obj);
}
com.diozero.remote.message.protobuf.I2C.Open other = (com.diozero.remote.message.protobuf.I2C.Open) obj;
if (getController()
!= other.getController()) return false;
if (getAddress()
!= other.getAddress()) return false;
if (getAddressSize()
!= other.getAddressSize()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CONTROLLER_FIELD_NUMBER;
hash = (53 * hash) + getController();
hash = (37 * hash) + ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getAddress();
hash = (37 * hash) + ADDRESSSIZE_FIELD_NUMBER;
hash = (53 * hash) + getAddressSize();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.diozero.remote.message.protobuf.I2C.Open parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.Open parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.Open parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.Open parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.Open parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.Open parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.Open parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.Open parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.Open parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.Open parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.Open parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.Open parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.diozero.remote.message.protobuf.I2C.Open prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code diozero.I2C.Open}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:diozero.I2C.Open)
com.diozero.remote.message.protobuf.I2C.OpenOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_Open_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_Open_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.I2C.Open.class, com.diozero.remote.message.protobuf.I2C.Open.Builder.class);
}
// Construct using com.diozero.remote.message.protobuf.I2C.Open.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
controller_ = 0;
address_ = 0;
addressSize_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_Open_descriptor;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.Open getDefaultInstanceForType() {
return com.diozero.remote.message.protobuf.I2C.Open.getDefaultInstance();
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.Open build() {
com.diozero.remote.message.protobuf.I2C.Open result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.Open buildPartial() {
com.diozero.remote.message.protobuf.I2C.Open result = new com.diozero.remote.message.protobuf.I2C.Open(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.diozero.remote.message.protobuf.I2C.Open result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.controller_ = controller_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.address_ = address_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.addressSize_ = addressSize_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.diozero.remote.message.protobuf.I2C.Open) {
return mergeFrom((com.diozero.remote.message.protobuf.I2C.Open)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.diozero.remote.message.protobuf.I2C.Open other) {
if (other == com.diozero.remote.message.protobuf.I2C.Open.getDefaultInstance()) return this;
if (other.getController() != 0) {
setController(other.getController());
}
if (other.getAddress() != 0) {
setAddress(other.getAddress());
}
if (other.getAddressSize() != 0) {
setAddressSize(other.getAddressSize());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
controller_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
address_ = input.readInt32();
bitField0_ |= 0x00000002;
break;
} // case 16
case 24: {
addressSize_ = input.readInt32();
bitField0_ |= 0x00000004;
break;
} // case 24
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int controller_ ;
/**
* int32 controller = 1;
* @return The controller.
*/
@java.lang.Override
public int getController() {
return controller_;
}
/**
* int32 controller = 1;
* @param value The controller to set.
* @return This builder for chaining.
*/
public Builder setController(int value) {
controller_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* int32 controller = 1;
* @return This builder for chaining.
*/
public Builder clearController() {
bitField0_ = (bitField0_ & ~0x00000001);
controller_ = 0;
onChanged();
return this;
}
private int address_ ;
/**
* int32 address = 2;
* @return The address.
*/
@java.lang.Override
public int getAddress() {
return address_;
}
/**
* int32 address = 2;
* @param value The address to set.
* @return This builder for chaining.
*/
public Builder setAddress(int value) {
address_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* int32 address = 2;
* @return This builder for chaining.
*/
public Builder clearAddress() {
bitField0_ = (bitField0_ & ~0x00000002);
address_ = 0;
onChanged();
return this;
}
private int addressSize_ ;
/**
* int32 addressSize = 3;
* @return The addressSize.
*/
@java.lang.Override
public int getAddressSize() {
return addressSize_;
}
/**
* int32 addressSize = 3;
* @param value The addressSize to set.
* @return This builder for chaining.
*/
public Builder setAddressSize(int value) {
addressSize_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* int32 addressSize = 3;
* @return This builder for chaining.
*/
public Builder clearAddressSize() {
bitField0_ = (bitField0_ & ~0x00000004);
addressSize_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:diozero.I2C.Open)
}
// @@protoc_insertion_point(class_scope:diozero.I2C.Open)
private static final com.diozero.remote.message.protobuf.I2C.Open DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.diozero.remote.message.protobuf.I2C.Open();
}
public static com.diozero.remote.message.protobuf.I2C.Open getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Open parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.Open getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ProbeOrBuilder extends
// @@protoc_insertion_point(interface_extends:diozero.I2C.Probe)
com.google.protobuf.MessageOrBuilder {
/**
* int32 controller = 1;
* @return The controller.
*/
int getController();
/**
* int32 address = 2;
* @return The address.
*/
int getAddress();
/**
* .diozero.I2C.ProbeMode probeMode = 3;
* @return The enum numeric value on the wire for probeMode.
*/
int getProbeModeValue();
/**
* .diozero.I2C.ProbeMode probeMode = 3;
* @return The probeMode.
*/
com.diozero.remote.message.protobuf.I2C.ProbeMode getProbeMode();
}
/**
* Protobuf type {@code diozero.I2C.Probe}
*/
public static final class Probe extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:diozero.I2C.Probe)
ProbeOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
Probe.class.getName());
}
// Use Probe.newBuilder() to construct.
private Probe(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Probe() {
probeMode_ = 0;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_Probe_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_Probe_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.I2C.Probe.class, com.diozero.remote.message.protobuf.I2C.Probe.Builder.class);
}
public static final int CONTROLLER_FIELD_NUMBER = 1;
private int controller_ = 0;
/**
* int32 controller = 1;
* @return The controller.
*/
@java.lang.Override
public int getController() {
return controller_;
}
public static final int ADDRESS_FIELD_NUMBER = 2;
private int address_ = 0;
/**
* int32 address = 2;
* @return The address.
*/
@java.lang.Override
public int getAddress() {
return address_;
}
public static final int PROBEMODE_FIELD_NUMBER = 3;
private int probeMode_ = 0;
/**
* .diozero.I2C.ProbeMode probeMode = 3;
* @return The enum numeric value on the wire for probeMode.
*/
@java.lang.Override public int getProbeModeValue() {
return probeMode_;
}
/**
* .diozero.I2C.ProbeMode probeMode = 3;
* @return The probeMode.
*/
@java.lang.Override public com.diozero.remote.message.protobuf.I2C.ProbeMode getProbeMode() {
com.diozero.remote.message.protobuf.I2C.ProbeMode result = com.diozero.remote.message.protobuf.I2C.ProbeMode.forNumber(probeMode_);
return result == null ? com.diozero.remote.message.protobuf.I2C.ProbeMode.UNRECOGNIZED : result;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (controller_ != 0) {
output.writeInt32(1, controller_);
}
if (address_ != 0) {
output.writeInt32(2, address_);
}
if (probeMode_ != com.diozero.remote.message.protobuf.I2C.ProbeMode.QUICK.getNumber()) {
output.writeEnum(3, probeMode_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (controller_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, controller_);
}
if (address_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, address_);
}
if (probeMode_ != com.diozero.remote.message.protobuf.I2C.ProbeMode.QUICK.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, probeMode_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.diozero.remote.message.protobuf.I2C.Probe)) {
return super.equals(obj);
}
com.diozero.remote.message.protobuf.I2C.Probe other = (com.diozero.remote.message.protobuf.I2C.Probe) obj;
if (getController()
!= other.getController()) return false;
if (getAddress()
!= other.getAddress()) return false;
if (probeMode_ != other.probeMode_) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CONTROLLER_FIELD_NUMBER;
hash = (53 * hash) + getController();
hash = (37 * hash) + ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getAddress();
hash = (37 * hash) + PROBEMODE_FIELD_NUMBER;
hash = (53 * hash) + probeMode_;
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.diozero.remote.message.protobuf.I2C.Probe parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.Probe parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.Probe parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.Probe parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.Probe parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.Probe parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.Probe parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.Probe parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.Probe parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.Probe parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.Probe parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.Probe parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.diozero.remote.message.protobuf.I2C.Probe prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code diozero.I2C.Probe}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:diozero.I2C.Probe)
com.diozero.remote.message.protobuf.I2C.ProbeOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_Probe_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_Probe_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.I2C.Probe.class, com.diozero.remote.message.protobuf.I2C.Probe.Builder.class);
}
// Construct using com.diozero.remote.message.protobuf.I2C.Probe.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
controller_ = 0;
address_ = 0;
probeMode_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_Probe_descriptor;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.Probe getDefaultInstanceForType() {
return com.diozero.remote.message.protobuf.I2C.Probe.getDefaultInstance();
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.Probe build() {
com.diozero.remote.message.protobuf.I2C.Probe result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.Probe buildPartial() {
com.diozero.remote.message.protobuf.I2C.Probe result = new com.diozero.remote.message.protobuf.I2C.Probe(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.diozero.remote.message.protobuf.I2C.Probe result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.controller_ = controller_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.address_ = address_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.probeMode_ = probeMode_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.diozero.remote.message.protobuf.I2C.Probe) {
return mergeFrom((com.diozero.remote.message.protobuf.I2C.Probe)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.diozero.remote.message.protobuf.I2C.Probe other) {
if (other == com.diozero.remote.message.protobuf.I2C.Probe.getDefaultInstance()) return this;
if (other.getController() != 0) {
setController(other.getController());
}
if (other.getAddress() != 0) {
setAddress(other.getAddress());
}
if (other.probeMode_ != 0) {
setProbeModeValue(other.getProbeModeValue());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
controller_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
address_ = input.readInt32();
bitField0_ |= 0x00000002;
break;
} // case 16
case 24: {
probeMode_ = input.readEnum();
bitField0_ |= 0x00000004;
break;
} // case 24
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int controller_ ;
/**
* int32 controller = 1;
* @return The controller.
*/
@java.lang.Override
public int getController() {
return controller_;
}
/**
* int32 controller = 1;
* @param value The controller to set.
* @return This builder for chaining.
*/
public Builder setController(int value) {
controller_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* int32 controller = 1;
* @return This builder for chaining.
*/
public Builder clearController() {
bitField0_ = (bitField0_ & ~0x00000001);
controller_ = 0;
onChanged();
return this;
}
private int address_ ;
/**
* int32 address = 2;
* @return The address.
*/
@java.lang.Override
public int getAddress() {
return address_;
}
/**
* int32 address = 2;
* @param value The address to set.
* @return This builder for chaining.
*/
public Builder setAddress(int value) {
address_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* int32 address = 2;
* @return This builder for chaining.
*/
public Builder clearAddress() {
bitField0_ = (bitField0_ & ~0x00000002);
address_ = 0;
onChanged();
return this;
}
private int probeMode_ = 0;
/**
* .diozero.I2C.ProbeMode probeMode = 3;
* @return The enum numeric value on the wire for probeMode.
*/
@java.lang.Override public int getProbeModeValue() {
return probeMode_;
}
/**
* .diozero.I2C.ProbeMode probeMode = 3;
* @param value The enum numeric value on the wire for probeMode to set.
* @return This builder for chaining.
*/
public Builder setProbeModeValue(int value) {
probeMode_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* .diozero.I2C.ProbeMode probeMode = 3;
* @return The probeMode.
*/
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.ProbeMode getProbeMode() {
com.diozero.remote.message.protobuf.I2C.ProbeMode result = com.diozero.remote.message.protobuf.I2C.ProbeMode.forNumber(probeMode_);
return result == null ? com.diozero.remote.message.protobuf.I2C.ProbeMode.UNRECOGNIZED : result;
}
/**
* .diozero.I2C.ProbeMode probeMode = 3;
* @param value The probeMode to set.
* @return This builder for chaining.
*/
public Builder setProbeMode(com.diozero.remote.message.protobuf.I2C.ProbeMode value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
probeMode_ = value.getNumber();
onChanged();
return this;
}
/**
* .diozero.I2C.ProbeMode probeMode = 3;
* @return This builder for chaining.
*/
public Builder clearProbeMode() {
bitField0_ = (bitField0_ & ~0x00000004);
probeMode_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:diozero.I2C.Probe)
}
// @@protoc_insertion_point(class_scope:diozero.I2C.Probe)
private static final com.diozero.remote.message.protobuf.I2C.Probe DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.diozero.remote.message.protobuf.I2C.Probe();
}
public static com.diozero.remote.message.protobuf.I2C.Probe getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Probe parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.Probe getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface BitOrBuilder extends
// @@protoc_insertion_point(interface_extends:diozero.I2C.Bit)
com.google.protobuf.MessageOrBuilder {
/**
* int32 controller = 1;
* @return The controller.
*/
int getController();
/**
* int32 address = 2;
* @return The address.
*/
int getAddress();
/**
* int32 bit = 3;
* @return The bit.
*/
int getBit();
}
/**
* Protobuf type {@code diozero.I2C.Bit}
*/
public static final class Bit extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:diozero.I2C.Bit)
BitOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
Bit.class.getName());
}
// Use Bit.newBuilder() to construct.
private Bit(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Bit() {
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_Bit_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_Bit_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.I2C.Bit.class, com.diozero.remote.message.protobuf.I2C.Bit.Builder.class);
}
public static final int CONTROLLER_FIELD_NUMBER = 1;
private int controller_ = 0;
/**
* int32 controller = 1;
* @return The controller.
*/
@java.lang.Override
public int getController() {
return controller_;
}
public static final int ADDRESS_FIELD_NUMBER = 2;
private int address_ = 0;
/**
* int32 address = 2;
* @return The address.
*/
@java.lang.Override
public int getAddress() {
return address_;
}
public static final int BIT_FIELD_NUMBER = 3;
private int bit_ = 0;
/**
* int32 bit = 3;
* @return The bit.
*/
@java.lang.Override
public int getBit() {
return bit_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (controller_ != 0) {
output.writeInt32(1, controller_);
}
if (address_ != 0) {
output.writeInt32(2, address_);
}
if (bit_ != 0) {
output.writeInt32(3, bit_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (controller_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, controller_);
}
if (address_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, address_);
}
if (bit_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, bit_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.diozero.remote.message.protobuf.I2C.Bit)) {
return super.equals(obj);
}
com.diozero.remote.message.protobuf.I2C.Bit other = (com.diozero.remote.message.protobuf.I2C.Bit) obj;
if (getController()
!= other.getController()) return false;
if (getAddress()
!= other.getAddress()) return false;
if (getBit()
!= other.getBit()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CONTROLLER_FIELD_NUMBER;
hash = (53 * hash) + getController();
hash = (37 * hash) + ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getAddress();
hash = (37 * hash) + BIT_FIELD_NUMBER;
hash = (53 * hash) + getBit();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.diozero.remote.message.protobuf.I2C.Bit parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.Bit parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.Bit parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.Bit parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.Bit parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.Bit parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.Bit parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.Bit parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.Bit parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.Bit parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.Bit parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.Bit parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.diozero.remote.message.protobuf.I2C.Bit prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code diozero.I2C.Bit}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:diozero.I2C.Bit)
com.diozero.remote.message.protobuf.I2C.BitOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_Bit_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_Bit_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.I2C.Bit.class, com.diozero.remote.message.protobuf.I2C.Bit.Builder.class);
}
// Construct using com.diozero.remote.message.protobuf.I2C.Bit.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
controller_ = 0;
address_ = 0;
bit_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_Bit_descriptor;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.Bit getDefaultInstanceForType() {
return com.diozero.remote.message.protobuf.I2C.Bit.getDefaultInstance();
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.Bit build() {
com.diozero.remote.message.protobuf.I2C.Bit result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.Bit buildPartial() {
com.diozero.remote.message.protobuf.I2C.Bit result = new com.diozero.remote.message.protobuf.I2C.Bit(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.diozero.remote.message.protobuf.I2C.Bit result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.controller_ = controller_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.address_ = address_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.bit_ = bit_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.diozero.remote.message.protobuf.I2C.Bit) {
return mergeFrom((com.diozero.remote.message.protobuf.I2C.Bit)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.diozero.remote.message.protobuf.I2C.Bit other) {
if (other == com.diozero.remote.message.protobuf.I2C.Bit.getDefaultInstance()) return this;
if (other.getController() != 0) {
setController(other.getController());
}
if (other.getAddress() != 0) {
setAddress(other.getAddress());
}
if (other.getBit() != 0) {
setBit(other.getBit());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
controller_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
address_ = input.readInt32();
bitField0_ |= 0x00000002;
break;
} // case 16
case 24: {
bit_ = input.readInt32();
bitField0_ |= 0x00000004;
break;
} // case 24
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int controller_ ;
/**
* int32 controller = 1;
* @return The controller.
*/
@java.lang.Override
public int getController() {
return controller_;
}
/**
* int32 controller = 1;
* @param value The controller to set.
* @return This builder for chaining.
*/
public Builder setController(int value) {
controller_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* int32 controller = 1;
* @return This builder for chaining.
*/
public Builder clearController() {
bitField0_ = (bitField0_ & ~0x00000001);
controller_ = 0;
onChanged();
return this;
}
private int address_ ;
/**
* int32 address = 2;
* @return The address.
*/
@java.lang.Override
public int getAddress() {
return address_;
}
/**
* int32 address = 2;
* @param value The address to set.
* @return This builder for chaining.
*/
public Builder setAddress(int value) {
address_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* int32 address = 2;
* @return This builder for chaining.
*/
public Builder clearAddress() {
bitField0_ = (bitField0_ & ~0x00000002);
address_ = 0;
onChanged();
return this;
}
private int bit_ ;
/**
* int32 bit = 3;
* @return The bit.
*/
@java.lang.Override
public int getBit() {
return bit_;
}
/**
* int32 bit = 3;
* @param value The bit to set.
* @return This builder for chaining.
*/
public Builder setBit(int value) {
bit_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* int32 bit = 3;
* @return This builder for chaining.
*/
public Builder clearBit() {
bitField0_ = (bitField0_ & ~0x00000004);
bit_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:diozero.I2C.Bit)
}
// @@protoc_insertion_point(class_scope:diozero.I2C.Bit)
private static final com.diozero.remote.message.protobuf.I2C.Bit DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.diozero.remote.message.protobuf.I2C.Bit();
}
public static com.diozero.remote.message.protobuf.I2C.Bit getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Bit parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.Bit getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ByteMessageOrBuilder extends
// @@protoc_insertion_point(interface_extends:diozero.I2C.ByteMessage)
com.google.protobuf.MessageOrBuilder {
/**
* int32 controller = 1;
* @return The controller.
*/
int getController();
/**
* int32 address = 2;
* @return The address.
*/
int getAddress();
/**
* int32 data = 3;
* @return The data.
*/
int getData();
}
/**
* Protobuf type {@code diozero.I2C.ByteMessage}
*/
public static final class ByteMessage extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:diozero.I2C.ByteMessage)
ByteMessageOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
ByteMessage.class.getName());
}
// Use ByteMessage.newBuilder() to construct.
private ByteMessage(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private ByteMessage() {
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_ByteMessage_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_ByteMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.I2C.ByteMessage.class, com.diozero.remote.message.protobuf.I2C.ByteMessage.Builder.class);
}
public static final int CONTROLLER_FIELD_NUMBER = 1;
private int controller_ = 0;
/**
* int32 controller = 1;
* @return The controller.
*/
@java.lang.Override
public int getController() {
return controller_;
}
public static final int ADDRESS_FIELD_NUMBER = 2;
private int address_ = 0;
/**
* int32 address = 2;
* @return The address.
*/
@java.lang.Override
public int getAddress() {
return address_;
}
public static final int DATA_FIELD_NUMBER = 3;
private int data_ = 0;
/**
* int32 data = 3;
* @return The data.
*/
@java.lang.Override
public int getData() {
return data_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (controller_ != 0) {
output.writeInt32(1, controller_);
}
if (address_ != 0) {
output.writeInt32(2, address_);
}
if (data_ != 0) {
output.writeInt32(3, data_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (controller_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, controller_);
}
if (address_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, address_);
}
if (data_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, data_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.diozero.remote.message.protobuf.I2C.ByteMessage)) {
return super.equals(obj);
}
com.diozero.remote.message.protobuf.I2C.ByteMessage other = (com.diozero.remote.message.protobuf.I2C.ByteMessage) obj;
if (getController()
!= other.getController()) return false;
if (getAddress()
!= other.getAddress()) return false;
if (getData()
!= other.getData()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CONTROLLER_FIELD_NUMBER;
hash = (53 * hash) + getController();
hash = (37 * hash) + ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getAddress();
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + getData();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.diozero.remote.message.protobuf.I2C.ByteMessage parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.ByteMessage parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.ByteMessage parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.ByteMessage parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.ByteMessage parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.ByteMessage parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.ByteMessage parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.ByteMessage parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.ByteMessage parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.ByteMessage parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.ByteMessage parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.ByteMessage parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.diozero.remote.message.protobuf.I2C.ByteMessage prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code diozero.I2C.ByteMessage}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:diozero.I2C.ByteMessage)
com.diozero.remote.message.protobuf.I2C.ByteMessageOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_ByteMessage_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_ByteMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.I2C.ByteMessage.class, com.diozero.remote.message.protobuf.I2C.ByteMessage.Builder.class);
}
// Construct using com.diozero.remote.message.protobuf.I2C.ByteMessage.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
controller_ = 0;
address_ = 0;
data_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_ByteMessage_descriptor;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.ByteMessage getDefaultInstanceForType() {
return com.diozero.remote.message.protobuf.I2C.ByteMessage.getDefaultInstance();
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.ByteMessage build() {
com.diozero.remote.message.protobuf.I2C.ByteMessage result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.ByteMessage buildPartial() {
com.diozero.remote.message.protobuf.I2C.ByteMessage result = new com.diozero.remote.message.protobuf.I2C.ByteMessage(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.diozero.remote.message.protobuf.I2C.ByteMessage result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.controller_ = controller_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.address_ = address_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.data_ = data_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.diozero.remote.message.protobuf.I2C.ByteMessage) {
return mergeFrom((com.diozero.remote.message.protobuf.I2C.ByteMessage)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.diozero.remote.message.protobuf.I2C.ByteMessage other) {
if (other == com.diozero.remote.message.protobuf.I2C.ByteMessage.getDefaultInstance()) return this;
if (other.getController() != 0) {
setController(other.getController());
}
if (other.getAddress() != 0) {
setAddress(other.getAddress());
}
if (other.getData() != 0) {
setData(other.getData());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
controller_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
address_ = input.readInt32();
bitField0_ |= 0x00000002;
break;
} // case 16
case 24: {
data_ = input.readInt32();
bitField0_ |= 0x00000004;
break;
} // case 24
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int controller_ ;
/**
* int32 controller = 1;
* @return The controller.
*/
@java.lang.Override
public int getController() {
return controller_;
}
/**
* int32 controller = 1;
* @param value The controller to set.
* @return This builder for chaining.
*/
public Builder setController(int value) {
controller_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* int32 controller = 1;
* @return This builder for chaining.
*/
public Builder clearController() {
bitField0_ = (bitField0_ & ~0x00000001);
controller_ = 0;
onChanged();
return this;
}
private int address_ ;
/**
* int32 address = 2;
* @return The address.
*/
@java.lang.Override
public int getAddress() {
return address_;
}
/**
* int32 address = 2;
* @param value The address to set.
* @return This builder for chaining.
*/
public Builder setAddress(int value) {
address_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* int32 address = 2;
* @return This builder for chaining.
*/
public Builder clearAddress() {
bitField0_ = (bitField0_ & ~0x00000002);
address_ = 0;
onChanged();
return this;
}
private int data_ ;
/**
* int32 data = 3;
* @return The data.
*/
@java.lang.Override
public int getData() {
return data_;
}
/**
* int32 data = 3;
* @param value The data to set.
* @return This builder for chaining.
*/
public Builder setData(int value) {
data_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* int32 data = 3;
* @return This builder for chaining.
*/
public Builder clearData() {
bitField0_ = (bitField0_ & ~0x00000004);
data_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:diozero.I2C.ByteMessage)
}
// @@protoc_insertion_point(class_scope:diozero.I2C.ByteMessage)
private static final com.diozero.remote.message.protobuf.I2C.ByteMessage DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.diozero.remote.message.protobuf.I2C.ByteMessage();
}
public static com.diozero.remote.message.protobuf.I2C.ByteMessage getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ByteMessage parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.ByteMessage getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RegisterOrBuilder extends
// @@protoc_insertion_point(interface_extends:diozero.I2C.Register)
com.google.protobuf.MessageOrBuilder {
/**
* int32 controller = 1;
* @return The controller.
*/
int getController();
/**
* int32 address = 2;
* @return The address.
*/
int getAddress();
/**
* int32 register = 3;
* @return The register.
*/
int getRegister();
}
/**
* Protobuf type {@code diozero.I2C.Register}
*/
public static final class Register extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:diozero.I2C.Register)
RegisterOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
Register.class.getName());
}
// Use Register.newBuilder() to construct.
private Register(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Register() {
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_Register_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_Register_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.I2C.Register.class, com.diozero.remote.message.protobuf.I2C.Register.Builder.class);
}
public static final int CONTROLLER_FIELD_NUMBER = 1;
private int controller_ = 0;
/**
* int32 controller = 1;
* @return The controller.
*/
@java.lang.Override
public int getController() {
return controller_;
}
public static final int ADDRESS_FIELD_NUMBER = 2;
private int address_ = 0;
/**
* int32 address = 2;
* @return The address.
*/
@java.lang.Override
public int getAddress() {
return address_;
}
public static final int REGISTER_FIELD_NUMBER = 3;
private int register_ = 0;
/**
* int32 register = 3;
* @return The register.
*/
@java.lang.Override
public int getRegister() {
return register_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (controller_ != 0) {
output.writeInt32(1, controller_);
}
if (address_ != 0) {
output.writeInt32(2, address_);
}
if (register_ != 0) {
output.writeInt32(3, register_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (controller_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, controller_);
}
if (address_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, address_);
}
if (register_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, register_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.diozero.remote.message.protobuf.I2C.Register)) {
return super.equals(obj);
}
com.diozero.remote.message.protobuf.I2C.Register other = (com.diozero.remote.message.protobuf.I2C.Register) obj;
if (getController()
!= other.getController()) return false;
if (getAddress()
!= other.getAddress()) return false;
if (getRegister()
!= other.getRegister()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CONTROLLER_FIELD_NUMBER;
hash = (53 * hash) + getController();
hash = (37 * hash) + ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getAddress();
hash = (37 * hash) + REGISTER_FIELD_NUMBER;
hash = (53 * hash) + getRegister();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.diozero.remote.message.protobuf.I2C.Register parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.Register parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.Register parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.Register parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.Register parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.Register parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.Register parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.Register parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.Register parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.Register parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.Register parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.Register parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.diozero.remote.message.protobuf.I2C.Register prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code diozero.I2C.Register}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:diozero.I2C.Register)
com.diozero.remote.message.protobuf.I2C.RegisterOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_Register_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_Register_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.I2C.Register.class, com.diozero.remote.message.protobuf.I2C.Register.Builder.class);
}
// Construct using com.diozero.remote.message.protobuf.I2C.Register.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
controller_ = 0;
address_ = 0;
register_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_Register_descriptor;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.Register getDefaultInstanceForType() {
return com.diozero.remote.message.protobuf.I2C.Register.getDefaultInstance();
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.Register build() {
com.diozero.remote.message.protobuf.I2C.Register result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.Register buildPartial() {
com.diozero.remote.message.protobuf.I2C.Register result = new com.diozero.remote.message.protobuf.I2C.Register(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.diozero.remote.message.protobuf.I2C.Register result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.controller_ = controller_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.address_ = address_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.register_ = register_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.diozero.remote.message.protobuf.I2C.Register) {
return mergeFrom((com.diozero.remote.message.protobuf.I2C.Register)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.diozero.remote.message.protobuf.I2C.Register other) {
if (other == com.diozero.remote.message.protobuf.I2C.Register.getDefaultInstance()) return this;
if (other.getController() != 0) {
setController(other.getController());
}
if (other.getAddress() != 0) {
setAddress(other.getAddress());
}
if (other.getRegister() != 0) {
setRegister(other.getRegister());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
controller_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
address_ = input.readInt32();
bitField0_ |= 0x00000002;
break;
} // case 16
case 24: {
register_ = input.readInt32();
bitField0_ |= 0x00000004;
break;
} // case 24
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int controller_ ;
/**
* int32 controller = 1;
* @return The controller.
*/
@java.lang.Override
public int getController() {
return controller_;
}
/**
* int32 controller = 1;
* @param value The controller to set.
* @return This builder for chaining.
*/
public Builder setController(int value) {
controller_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* int32 controller = 1;
* @return This builder for chaining.
*/
public Builder clearController() {
bitField0_ = (bitField0_ & ~0x00000001);
controller_ = 0;
onChanged();
return this;
}
private int address_ ;
/**
* int32 address = 2;
* @return The address.
*/
@java.lang.Override
public int getAddress() {
return address_;
}
/**
* int32 address = 2;
* @param value The address to set.
* @return This builder for chaining.
*/
public Builder setAddress(int value) {
address_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* int32 address = 2;
* @return This builder for chaining.
*/
public Builder clearAddress() {
bitField0_ = (bitField0_ & ~0x00000002);
address_ = 0;
onChanged();
return this;
}
private int register_ ;
/**
* int32 register = 3;
* @return The register.
*/
@java.lang.Override
public int getRegister() {
return register_;
}
/**
* int32 register = 3;
* @param value The register to set.
* @return This builder for chaining.
*/
public Builder setRegister(int value) {
register_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* int32 register = 3;
* @return This builder for chaining.
*/
public Builder clearRegister() {
bitField0_ = (bitField0_ & ~0x00000004);
register_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:diozero.I2C.Register)
}
// @@protoc_insertion_point(class_scope:diozero.I2C.Register)
private static final com.diozero.remote.message.protobuf.I2C.Register DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.diozero.remote.message.protobuf.I2C.Register();
}
public static com.diozero.remote.message.protobuf.I2C.Register getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Register parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.Register getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RegisterAndByteOrBuilder extends
// @@protoc_insertion_point(interface_extends:diozero.I2C.RegisterAndByte)
com.google.protobuf.MessageOrBuilder {
/**
* int32 controller = 1;
* @return The controller.
*/
int getController();
/**
* int32 address = 2;
* @return The address.
*/
int getAddress();
/**
* int32 register = 3;
* @return The register.
*/
int getRegister();
/**
* int32 data = 4;
* @return The data.
*/
int getData();
}
/**
* Protobuf type {@code diozero.I2C.RegisterAndByte}
*/
public static final class RegisterAndByte extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:diozero.I2C.RegisterAndByte)
RegisterAndByteOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
RegisterAndByte.class.getName());
}
// Use RegisterAndByte.newBuilder() to construct.
private RegisterAndByte(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private RegisterAndByte() {
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_RegisterAndByte_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_RegisterAndByte_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.I2C.RegisterAndByte.class, com.diozero.remote.message.protobuf.I2C.RegisterAndByte.Builder.class);
}
public static final int CONTROLLER_FIELD_NUMBER = 1;
private int controller_ = 0;
/**
* int32 controller = 1;
* @return The controller.
*/
@java.lang.Override
public int getController() {
return controller_;
}
public static final int ADDRESS_FIELD_NUMBER = 2;
private int address_ = 0;
/**
* int32 address = 2;
* @return The address.
*/
@java.lang.Override
public int getAddress() {
return address_;
}
public static final int REGISTER_FIELD_NUMBER = 3;
private int register_ = 0;
/**
* int32 register = 3;
* @return The register.
*/
@java.lang.Override
public int getRegister() {
return register_;
}
public static final int DATA_FIELD_NUMBER = 4;
private int data_ = 0;
/**
* int32 data = 4;
* @return The data.
*/
@java.lang.Override
public int getData() {
return data_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (controller_ != 0) {
output.writeInt32(1, controller_);
}
if (address_ != 0) {
output.writeInt32(2, address_);
}
if (register_ != 0) {
output.writeInt32(3, register_);
}
if (data_ != 0) {
output.writeInt32(4, data_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (controller_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, controller_);
}
if (address_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, address_);
}
if (register_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, register_);
}
if (data_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(4, data_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.diozero.remote.message.protobuf.I2C.RegisterAndByte)) {
return super.equals(obj);
}
com.diozero.remote.message.protobuf.I2C.RegisterAndByte other = (com.diozero.remote.message.protobuf.I2C.RegisterAndByte) obj;
if (getController()
!= other.getController()) return false;
if (getAddress()
!= other.getAddress()) return false;
if (getRegister()
!= other.getRegister()) return false;
if (getData()
!= other.getData()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CONTROLLER_FIELD_NUMBER;
hash = (53 * hash) + getController();
hash = (37 * hash) + ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getAddress();
hash = (37 * hash) + REGISTER_FIELD_NUMBER;
hash = (53 * hash) + getRegister();
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + getData();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndByte parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndByte parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndByte parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndByte parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndByte parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndByte parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndByte parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndByte parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndByte parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndByte parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndByte parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndByte parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.diozero.remote.message.protobuf.I2C.RegisterAndByte prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code diozero.I2C.RegisterAndByte}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:diozero.I2C.RegisterAndByte)
com.diozero.remote.message.protobuf.I2C.RegisterAndByteOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_RegisterAndByte_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_RegisterAndByte_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.I2C.RegisterAndByte.class, com.diozero.remote.message.protobuf.I2C.RegisterAndByte.Builder.class);
}
// Construct using com.diozero.remote.message.protobuf.I2C.RegisterAndByte.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
controller_ = 0;
address_ = 0;
register_ = 0;
data_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_RegisterAndByte_descriptor;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.RegisterAndByte getDefaultInstanceForType() {
return com.diozero.remote.message.protobuf.I2C.RegisterAndByte.getDefaultInstance();
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.RegisterAndByte build() {
com.diozero.remote.message.protobuf.I2C.RegisterAndByte result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.RegisterAndByte buildPartial() {
com.diozero.remote.message.protobuf.I2C.RegisterAndByte result = new com.diozero.remote.message.protobuf.I2C.RegisterAndByte(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.diozero.remote.message.protobuf.I2C.RegisterAndByte result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.controller_ = controller_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.address_ = address_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.register_ = register_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.data_ = data_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.diozero.remote.message.protobuf.I2C.RegisterAndByte) {
return mergeFrom((com.diozero.remote.message.protobuf.I2C.RegisterAndByte)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.diozero.remote.message.protobuf.I2C.RegisterAndByte other) {
if (other == com.diozero.remote.message.protobuf.I2C.RegisterAndByte.getDefaultInstance()) return this;
if (other.getController() != 0) {
setController(other.getController());
}
if (other.getAddress() != 0) {
setAddress(other.getAddress());
}
if (other.getRegister() != 0) {
setRegister(other.getRegister());
}
if (other.getData() != 0) {
setData(other.getData());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
controller_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
address_ = input.readInt32();
bitField0_ |= 0x00000002;
break;
} // case 16
case 24: {
register_ = input.readInt32();
bitField0_ |= 0x00000004;
break;
} // case 24
case 32: {
data_ = input.readInt32();
bitField0_ |= 0x00000008;
break;
} // case 32
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int controller_ ;
/**
* int32 controller = 1;
* @return The controller.
*/
@java.lang.Override
public int getController() {
return controller_;
}
/**
* int32 controller = 1;
* @param value The controller to set.
* @return This builder for chaining.
*/
public Builder setController(int value) {
controller_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* int32 controller = 1;
* @return This builder for chaining.
*/
public Builder clearController() {
bitField0_ = (bitField0_ & ~0x00000001);
controller_ = 0;
onChanged();
return this;
}
private int address_ ;
/**
* int32 address = 2;
* @return The address.
*/
@java.lang.Override
public int getAddress() {
return address_;
}
/**
* int32 address = 2;
* @param value The address to set.
* @return This builder for chaining.
*/
public Builder setAddress(int value) {
address_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* int32 address = 2;
* @return This builder for chaining.
*/
public Builder clearAddress() {
bitField0_ = (bitField0_ & ~0x00000002);
address_ = 0;
onChanged();
return this;
}
private int register_ ;
/**
* int32 register = 3;
* @return The register.
*/
@java.lang.Override
public int getRegister() {
return register_;
}
/**
* int32 register = 3;
* @param value The register to set.
* @return This builder for chaining.
*/
public Builder setRegister(int value) {
register_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* int32 register = 3;
* @return This builder for chaining.
*/
public Builder clearRegister() {
bitField0_ = (bitField0_ & ~0x00000004);
register_ = 0;
onChanged();
return this;
}
private int data_ ;
/**
* int32 data = 4;
* @return The data.
*/
@java.lang.Override
public int getData() {
return data_;
}
/**
* int32 data = 4;
* @param value The data to set.
* @return This builder for chaining.
*/
public Builder setData(int value) {
data_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* int32 data = 4;
* @return This builder for chaining.
*/
public Builder clearData() {
bitField0_ = (bitField0_ & ~0x00000008);
data_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:diozero.I2C.RegisterAndByte)
}
// @@protoc_insertion_point(class_scope:diozero.I2C.RegisterAndByte)
private static final com.diozero.remote.message.protobuf.I2C.RegisterAndByte DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.diozero.remote.message.protobuf.I2C.RegisterAndByte();
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndByte getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RegisterAndByte parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.RegisterAndByte getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RegisterAndWordDataOrBuilder extends
// @@protoc_insertion_point(interface_extends:diozero.I2C.RegisterAndWordData)
com.google.protobuf.MessageOrBuilder {
/**
* int32 controller = 1;
* @return The controller.
*/
int getController();
/**
* int32 address = 2;
* @return The address.
*/
int getAddress();
/**
* int32 register = 3;
* @return The register.
*/
int getRegister();
/**
* int32 data = 4;
* @return The data.
*/
int getData();
}
/**
* Protobuf type {@code diozero.I2C.RegisterAndWordData}
*/
public static final class RegisterAndWordData extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:diozero.I2C.RegisterAndWordData)
RegisterAndWordDataOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
RegisterAndWordData.class.getName());
}
// Use RegisterAndWordData.newBuilder() to construct.
private RegisterAndWordData(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private RegisterAndWordData() {
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_RegisterAndWordData_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_RegisterAndWordData_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.I2C.RegisterAndWordData.class, com.diozero.remote.message.protobuf.I2C.RegisterAndWordData.Builder.class);
}
public static final int CONTROLLER_FIELD_NUMBER = 1;
private int controller_ = 0;
/**
* int32 controller = 1;
* @return The controller.
*/
@java.lang.Override
public int getController() {
return controller_;
}
public static final int ADDRESS_FIELD_NUMBER = 2;
private int address_ = 0;
/**
* int32 address = 2;
* @return The address.
*/
@java.lang.Override
public int getAddress() {
return address_;
}
public static final int REGISTER_FIELD_NUMBER = 3;
private int register_ = 0;
/**
* int32 register = 3;
* @return The register.
*/
@java.lang.Override
public int getRegister() {
return register_;
}
public static final int DATA_FIELD_NUMBER = 4;
private int data_ = 0;
/**
* int32 data = 4;
* @return The data.
*/
@java.lang.Override
public int getData() {
return data_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (controller_ != 0) {
output.writeInt32(1, controller_);
}
if (address_ != 0) {
output.writeInt32(2, address_);
}
if (register_ != 0) {
output.writeInt32(3, register_);
}
if (data_ != 0) {
output.writeInt32(4, data_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (controller_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, controller_);
}
if (address_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, address_);
}
if (register_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, register_);
}
if (data_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(4, data_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.diozero.remote.message.protobuf.I2C.RegisterAndWordData)) {
return super.equals(obj);
}
com.diozero.remote.message.protobuf.I2C.RegisterAndWordData other = (com.diozero.remote.message.protobuf.I2C.RegisterAndWordData) obj;
if (getController()
!= other.getController()) return false;
if (getAddress()
!= other.getAddress()) return false;
if (getRegister()
!= other.getRegister()) return false;
if (getData()
!= other.getData()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CONTROLLER_FIELD_NUMBER;
hash = (53 * hash) + getController();
hash = (37 * hash) + ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getAddress();
hash = (37 * hash) + REGISTER_FIELD_NUMBER;
hash = (53 * hash) + getRegister();
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + getData();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndWordData parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndWordData parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndWordData parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndWordData parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndWordData parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndWordData parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndWordData parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndWordData parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndWordData parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndWordData parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndWordData parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndWordData parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.diozero.remote.message.protobuf.I2C.RegisterAndWordData prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code diozero.I2C.RegisterAndWordData}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:diozero.I2C.RegisterAndWordData)
com.diozero.remote.message.protobuf.I2C.RegisterAndWordDataOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_RegisterAndWordData_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_RegisterAndWordData_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.I2C.RegisterAndWordData.class, com.diozero.remote.message.protobuf.I2C.RegisterAndWordData.Builder.class);
}
// Construct using com.diozero.remote.message.protobuf.I2C.RegisterAndWordData.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
controller_ = 0;
address_ = 0;
register_ = 0;
data_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_RegisterAndWordData_descriptor;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.RegisterAndWordData getDefaultInstanceForType() {
return com.diozero.remote.message.protobuf.I2C.RegisterAndWordData.getDefaultInstance();
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.RegisterAndWordData build() {
com.diozero.remote.message.protobuf.I2C.RegisterAndWordData result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.RegisterAndWordData buildPartial() {
com.diozero.remote.message.protobuf.I2C.RegisterAndWordData result = new com.diozero.remote.message.protobuf.I2C.RegisterAndWordData(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.diozero.remote.message.protobuf.I2C.RegisterAndWordData result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.controller_ = controller_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.address_ = address_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.register_ = register_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.data_ = data_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.diozero.remote.message.protobuf.I2C.RegisterAndWordData) {
return mergeFrom((com.diozero.remote.message.protobuf.I2C.RegisterAndWordData)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.diozero.remote.message.protobuf.I2C.RegisterAndWordData other) {
if (other == com.diozero.remote.message.protobuf.I2C.RegisterAndWordData.getDefaultInstance()) return this;
if (other.getController() != 0) {
setController(other.getController());
}
if (other.getAddress() != 0) {
setAddress(other.getAddress());
}
if (other.getRegister() != 0) {
setRegister(other.getRegister());
}
if (other.getData() != 0) {
setData(other.getData());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
controller_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
address_ = input.readInt32();
bitField0_ |= 0x00000002;
break;
} // case 16
case 24: {
register_ = input.readInt32();
bitField0_ |= 0x00000004;
break;
} // case 24
case 32: {
data_ = input.readInt32();
bitField0_ |= 0x00000008;
break;
} // case 32
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int controller_ ;
/**
* int32 controller = 1;
* @return The controller.
*/
@java.lang.Override
public int getController() {
return controller_;
}
/**
* int32 controller = 1;
* @param value The controller to set.
* @return This builder for chaining.
*/
public Builder setController(int value) {
controller_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* int32 controller = 1;
* @return This builder for chaining.
*/
public Builder clearController() {
bitField0_ = (bitField0_ & ~0x00000001);
controller_ = 0;
onChanged();
return this;
}
private int address_ ;
/**
* int32 address = 2;
* @return The address.
*/
@java.lang.Override
public int getAddress() {
return address_;
}
/**
* int32 address = 2;
* @param value The address to set.
* @return This builder for chaining.
*/
public Builder setAddress(int value) {
address_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* int32 address = 2;
* @return This builder for chaining.
*/
public Builder clearAddress() {
bitField0_ = (bitField0_ & ~0x00000002);
address_ = 0;
onChanged();
return this;
}
private int register_ ;
/**
* int32 register = 3;
* @return The register.
*/
@java.lang.Override
public int getRegister() {
return register_;
}
/**
* int32 register = 3;
* @param value The register to set.
* @return This builder for chaining.
*/
public Builder setRegister(int value) {
register_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* int32 register = 3;
* @return This builder for chaining.
*/
public Builder clearRegister() {
bitField0_ = (bitField0_ & ~0x00000004);
register_ = 0;
onChanged();
return this;
}
private int data_ ;
/**
* int32 data = 4;
* @return The data.
*/
@java.lang.Override
public int getData() {
return data_;
}
/**
* int32 data = 4;
* @param value The data to set.
* @return This builder for chaining.
*/
public Builder setData(int value) {
data_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* int32 data = 4;
* @return This builder for chaining.
*/
public Builder clearData() {
bitField0_ = (bitField0_ & ~0x00000008);
data_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:diozero.I2C.RegisterAndWordData)
}
// @@protoc_insertion_point(class_scope:diozero.I2C.RegisterAndWordData)
private static final com.diozero.remote.message.protobuf.I2C.RegisterAndWordData DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.diozero.remote.message.protobuf.I2C.RegisterAndWordData();
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndWordData getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RegisterAndWordData parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.RegisterAndWordData getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RegisterAndByteArrayOrBuilder extends
// @@protoc_insertion_point(interface_extends:diozero.I2C.RegisterAndByteArray)
com.google.protobuf.MessageOrBuilder {
/**
* int32 controller = 1;
* @return The controller.
*/
int getController();
/**
* int32 address = 2;
* @return The address.
*/
int getAddress();
/**
* int32 register = 3;
* @return The register.
*/
int getRegister();
/**
* bytes data = 4;
* @return The data.
*/
com.google.protobuf.ByteString getData();
}
/**
* Protobuf type {@code diozero.I2C.RegisterAndByteArray}
*/
public static final class RegisterAndByteArray extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:diozero.I2C.RegisterAndByteArray)
RegisterAndByteArrayOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
RegisterAndByteArray.class.getName());
}
// Use RegisterAndByteArray.newBuilder() to construct.
private RegisterAndByteArray(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private RegisterAndByteArray() {
data_ = com.google.protobuf.ByteString.EMPTY;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_RegisterAndByteArray_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_RegisterAndByteArray_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray.class, com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray.Builder.class);
}
public static final int CONTROLLER_FIELD_NUMBER = 1;
private int controller_ = 0;
/**
* int32 controller = 1;
* @return The controller.
*/
@java.lang.Override
public int getController() {
return controller_;
}
public static final int ADDRESS_FIELD_NUMBER = 2;
private int address_ = 0;
/**
* int32 address = 2;
* @return The address.
*/
@java.lang.Override
public int getAddress() {
return address_;
}
public static final int REGISTER_FIELD_NUMBER = 3;
private int register_ = 0;
/**
* int32 register = 3;
* @return The register.
*/
@java.lang.Override
public int getRegister() {
return register_;
}
public static final int DATA_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString data_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes data = 4;
* @return The data.
*/
@java.lang.Override
public com.google.protobuf.ByteString getData() {
return data_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (controller_ != 0) {
output.writeInt32(1, controller_);
}
if (address_ != 0) {
output.writeInt32(2, address_);
}
if (register_ != 0) {
output.writeInt32(3, register_);
}
if (!data_.isEmpty()) {
output.writeBytes(4, data_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (controller_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, controller_);
}
if (address_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, address_);
}
if (register_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, register_);
}
if (!data_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, data_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray)) {
return super.equals(obj);
}
com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray other = (com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray) obj;
if (getController()
!= other.getController()) return false;
if (getAddress()
!= other.getAddress()) return false;
if (getRegister()
!= other.getRegister()) return false;
if (!getData()
.equals(other.getData())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CONTROLLER_FIELD_NUMBER;
hash = (53 * hash) + getController();
hash = (37 * hash) + ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getAddress();
hash = (37 * hash) + REGISTER_FIELD_NUMBER;
hash = (53 * hash) + getRegister();
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + getData().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code diozero.I2C.RegisterAndByteArray}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:diozero.I2C.RegisterAndByteArray)
com.diozero.remote.message.protobuf.I2C.RegisterAndByteArrayOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_RegisterAndByteArray_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_RegisterAndByteArray_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray.class, com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray.Builder.class);
}
// Construct using com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
controller_ = 0;
address_ = 0;
register_ = 0;
data_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_RegisterAndByteArray_descriptor;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray getDefaultInstanceForType() {
return com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray.getDefaultInstance();
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray build() {
com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray buildPartial() {
com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray result = new com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.controller_ = controller_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.address_ = address_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.register_ = register_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.data_ = data_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray) {
return mergeFrom((com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray other) {
if (other == com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray.getDefaultInstance()) return this;
if (other.getController() != 0) {
setController(other.getController());
}
if (other.getAddress() != 0) {
setAddress(other.getAddress());
}
if (other.getRegister() != 0) {
setRegister(other.getRegister());
}
if (other.getData() != com.google.protobuf.ByteString.EMPTY) {
setData(other.getData());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
controller_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
address_ = input.readInt32();
bitField0_ |= 0x00000002;
break;
} // case 16
case 24: {
register_ = input.readInt32();
bitField0_ |= 0x00000004;
break;
} // case 24
case 34: {
data_ = input.readBytes();
bitField0_ |= 0x00000008;
break;
} // case 34
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int controller_ ;
/**
* int32 controller = 1;
* @return The controller.
*/
@java.lang.Override
public int getController() {
return controller_;
}
/**
* int32 controller = 1;
* @param value The controller to set.
* @return This builder for chaining.
*/
public Builder setController(int value) {
controller_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* int32 controller = 1;
* @return This builder for chaining.
*/
public Builder clearController() {
bitField0_ = (bitField0_ & ~0x00000001);
controller_ = 0;
onChanged();
return this;
}
private int address_ ;
/**
* int32 address = 2;
* @return The address.
*/
@java.lang.Override
public int getAddress() {
return address_;
}
/**
* int32 address = 2;
* @param value The address to set.
* @return This builder for chaining.
*/
public Builder setAddress(int value) {
address_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* int32 address = 2;
* @return This builder for chaining.
*/
public Builder clearAddress() {
bitField0_ = (bitField0_ & ~0x00000002);
address_ = 0;
onChanged();
return this;
}
private int register_ ;
/**
* int32 register = 3;
* @return The register.
*/
@java.lang.Override
public int getRegister() {
return register_;
}
/**
* int32 register = 3;
* @param value The register to set.
* @return This builder for chaining.
*/
public Builder setRegister(int value) {
register_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* int32 register = 3;
* @return This builder for chaining.
*/
public Builder clearRegister() {
bitField0_ = (bitField0_ & ~0x00000004);
register_ = 0;
onChanged();
return this;
}
private com.google.protobuf.ByteString data_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes data = 4;
* @return The data.
*/
@java.lang.Override
public com.google.protobuf.ByteString getData() {
return data_;
}
/**
* bytes data = 4;
* @param value The data to set.
* @return This builder for chaining.
*/
public Builder setData(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
data_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* bytes data = 4;
* @return This builder for chaining.
*/
public Builder clearData() {
bitField0_ = (bitField0_ & ~0x00000008);
data_ = getDefaultInstance().getData();
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:diozero.I2C.RegisterAndByteArray)
}
// @@protoc_insertion_point(class_scope:diozero.I2C.RegisterAndByteArray)
private static final com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray();
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RegisterAndByteArray parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RegisterAndNumBytesOrBuilder extends
// @@protoc_insertion_point(interface_extends:diozero.I2C.RegisterAndNumBytes)
com.google.protobuf.MessageOrBuilder {
/**
* int32 controller = 1;
* @return The controller.
*/
int getController();
/**
* int32 address = 2;
* @return The address.
*/
int getAddress();
/**
* int32 register = 3;
* @return The register.
*/
int getRegister();
/**
* int32 length = 4;
* @return The length.
*/
int getLength();
}
/**
* Protobuf type {@code diozero.I2C.RegisterAndNumBytes}
*/
public static final class RegisterAndNumBytes extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:diozero.I2C.RegisterAndNumBytes)
RegisterAndNumBytesOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
RegisterAndNumBytes.class.getName());
}
// Use RegisterAndNumBytes.newBuilder() to construct.
private RegisterAndNumBytes(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private RegisterAndNumBytes() {
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_RegisterAndNumBytes_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_RegisterAndNumBytes_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes.class, com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes.Builder.class);
}
public static final int CONTROLLER_FIELD_NUMBER = 1;
private int controller_ = 0;
/**
* int32 controller = 1;
* @return The controller.
*/
@java.lang.Override
public int getController() {
return controller_;
}
public static final int ADDRESS_FIELD_NUMBER = 2;
private int address_ = 0;
/**
* int32 address = 2;
* @return The address.
*/
@java.lang.Override
public int getAddress() {
return address_;
}
public static final int REGISTER_FIELD_NUMBER = 3;
private int register_ = 0;
/**
* int32 register = 3;
* @return The register.
*/
@java.lang.Override
public int getRegister() {
return register_;
}
public static final int LENGTH_FIELD_NUMBER = 4;
private int length_ = 0;
/**
* int32 length = 4;
* @return The length.
*/
@java.lang.Override
public int getLength() {
return length_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (controller_ != 0) {
output.writeInt32(1, controller_);
}
if (address_ != 0) {
output.writeInt32(2, address_);
}
if (register_ != 0) {
output.writeInt32(3, register_);
}
if (length_ != 0) {
output.writeInt32(4, length_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (controller_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, controller_);
}
if (address_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, address_);
}
if (register_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, register_);
}
if (length_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(4, length_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes)) {
return super.equals(obj);
}
com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes other = (com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes) obj;
if (getController()
!= other.getController()) return false;
if (getAddress()
!= other.getAddress()) return false;
if (getRegister()
!= other.getRegister()) return false;
if (getLength()
!= other.getLength()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CONTROLLER_FIELD_NUMBER;
hash = (53 * hash) + getController();
hash = (37 * hash) + ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getAddress();
hash = (37 * hash) + REGISTER_FIELD_NUMBER;
hash = (53 * hash) + getRegister();
hash = (37 * hash) + LENGTH_FIELD_NUMBER;
hash = (53 * hash) + getLength();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code diozero.I2C.RegisterAndNumBytes}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:diozero.I2C.RegisterAndNumBytes)
com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytesOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_RegisterAndNumBytes_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_RegisterAndNumBytes_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes.class, com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes.Builder.class);
}
// Construct using com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
controller_ = 0;
address_ = 0;
register_ = 0;
length_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_RegisterAndNumBytes_descriptor;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes getDefaultInstanceForType() {
return com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes.getDefaultInstance();
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes build() {
com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes buildPartial() {
com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes result = new com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.controller_ = controller_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.address_ = address_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.register_ = register_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.length_ = length_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes) {
return mergeFrom((com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes other) {
if (other == com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes.getDefaultInstance()) return this;
if (other.getController() != 0) {
setController(other.getController());
}
if (other.getAddress() != 0) {
setAddress(other.getAddress());
}
if (other.getRegister() != 0) {
setRegister(other.getRegister());
}
if (other.getLength() != 0) {
setLength(other.getLength());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
controller_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
address_ = input.readInt32();
bitField0_ |= 0x00000002;
break;
} // case 16
case 24: {
register_ = input.readInt32();
bitField0_ |= 0x00000004;
break;
} // case 24
case 32: {
length_ = input.readInt32();
bitField0_ |= 0x00000008;
break;
} // case 32
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int controller_ ;
/**
* int32 controller = 1;
* @return The controller.
*/
@java.lang.Override
public int getController() {
return controller_;
}
/**
* int32 controller = 1;
* @param value The controller to set.
* @return This builder for chaining.
*/
public Builder setController(int value) {
controller_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* int32 controller = 1;
* @return This builder for chaining.
*/
public Builder clearController() {
bitField0_ = (bitField0_ & ~0x00000001);
controller_ = 0;
onChanged();
return this;
}
private int address_ ;
/**
* int32 address = 2;
* @return The address.
*/
@java.lang.Override
public int getAddress() {
return address_;
}
/**
* int32 address = 2;
* @param value The address to set.
* @return This builder for chaining.
*/
public Builder setAddress(int value) {
address_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* int32 address = 2;
* @return This builder for chaining.
*/
public Builder clearAddress() {
bitField0_ = (bitField0_ & ~0x00000002);
address_ = 0;
onChanged();
return this;
}
private int register_ ;
/**
* int32 register = 3;
* @return The register.
*/
@java.lang.Override
public int getRegister() {
return register_;
}
/**
* int32 register = 3;
* @param value The register to set.
* @return This builder for chaining.
*/
public Builder setRegister(int value) {
register_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* int32 register = 3;
* @return This builder for chaining.
*/
public Builder clearRegister() {
bitField0_ = (bitField0_ & ~0x00000004);
register_ = 0;
onChanged();
return this;
}
private int length_ ;
/**
* int32 length = 4;
* @return The length.
*/
@java.lang.Override
public int getLength() {
return length_;
}
/**
* int32 length = 4;
* @param value The length to set.
* @return This builder for chaining.
*/
public Builder setLength(int value) {
length_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* int32 length = 4;
* @return This builder for chaining.
*/
public Builder clearLength() {
bitField0_ = (bitField0_ & ~0x00000008);
length_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:diozero.I2C.RegisterAndNumBytes)
}
// @@protoc_insertion_point(class_scope:diozero.I2C.RegisterAndNumBytes)
private static final com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes();
}
public static com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RegisterAndNumBytes parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface NumBytesOrBuilder extends
// @@protoc_insertion_point(interface_extends:diozero.I2C.NumBytes)
com.google.protobuf.MessageOrBuilder {
/**
* int32 controller = 1;
* @return The controller.
*/
int getController();
/**
* int32 address = 2;
* @return The address.
*/
int getAddress();
/**
* int32 length = 3;
* @return The length.
*/
int getLength();
}
/**
* Protobuf type {@code diozero.I2C.NumBytes}
*/
public static final class NumBytes extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:diozero.I2C.NumBytes)
NumBytesOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
NumBytes.class.getName());
}
// Use NumBytes.newBuilder() to construct.
private NumBytes(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private NumBytes() {
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_NumBytes_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_NumBytes_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.I2C.NumBytes.class, com.diozero.remote.message.protobuf.I2C.NumBytes.Builder.class);
}
public static final int CONTROLLER_FIELD_NUMBER = 1;
private int controller_ = 0;
/**
* int32 controller = 1;
* @return The controller.
*/
@java.lang.Override
public int getController() {
return controller_;
}
public static final int ADDRESS_FIELD_NUMBER = 2;
private int address_ = 0;
/**
* int32 address = 2;
* @return The address.
*/
@java.lang.Override
public int getAddress() {
return address_;
}
public static final int LENGTH_FIELD_NUMBER = 3;
private int length_ = 0;
/**
* int32 length = 3;
* @return The length.
*/
@java.lang.Override
public int getLength() {
return length_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (controller_ != 0) {
output.writeInt32(1, controller_);
}
if (address_ != 0) {
output.writeInt32(2, address_);
}
if (length_ != 0) {
output.writeInt32(3, length_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (controller_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, controller_);
}
if (address_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, address_);
}
if (length_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, length_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.diozero.remote.message.protobuf.I2C.NumBytes)) {
return super.equals(obj);
}
com.diozero.remote.message.protobuf.I2C.NumBytes other = (com.diozero.remote.message.protobuf.I2C.NumBytes) obj;
if (getController()
!= other.getController()) return false;
if (getAddress()
!= other.getAddress()) return false;
if (getLength()
!= other.getLength()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CONTROLLER_FIELD_NUMBER;
hash = (53 * hash) + getController();
hash = (37 * hash) + ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getAddress();
hash = (37 * hash) + LENGTH_FIELD_NUMBER;
hash = (53 * hash) + getLength();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.diozero.remote.message.protobuf.I2C.NumBytes parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.NumBytes parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.NumBytes parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.NumBytes parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.NumBytes parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.NumBytes parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.NumBytes parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.NumBytes parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.NumBytes parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.NumBytes parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.NumBytes parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.NumBytes parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.diozero.remote.message.protobuf.I2C.NumBytes prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code diozero.I2C.NumBytes}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:diozero.I2C.NumBytes)
com.diozero.remote.message.protobuf.I2C.NumBytesOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_NumBytes_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_NumBytes_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.I2C.NumBytes.class, com.diozero.remote.message.protobuf.I2C.NumBytes.Builder.class);
}
// Construct using com.diozero.remote.message.protobuf.I2C.NumBytes.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
controller_ = 0;
address_ = 0;
length_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_NumBytes_descriptor;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.NumBytes getDefaultInstanceForType() {
return com.diozero.remote.message.protobuf.I2C.NumBytes.getDefaultInstance();
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.NumBytes build() {
com.diozero.remote.message.protobuf.I2C.NumBytes result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.NumBytes buildPartial() {
com.diozero.remote.message.protobuf.I2C.NumBytes result = new com.diozero.remote.message.protobuf.I2C.NumBytes(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.diozero.remote.message.protobuf.I2C.NumBytes result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.controller_ = controller_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.address_ = address_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.length_ = length_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.diozero.remote.message.protobuf.I2C.NumBytes) {
return mergeFrom((com.diozero.remote.message.protobuf.I2C.NumBytes)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.diozero.remote.message.protobuf.I2C.NumBytes other) {
if (other == com.diozero.remote.message.protobuf.I2C.NumBytes.getDefaultInstance()) return this;
if (other.getController() != 0) {
setController(other.getController());
}
if (other.getAddress() != 0) {
setAddress(other.getAddress());
}
if (other.getLength() != 0) {
setLength(other.getLength());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
controller_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
address_ = input.readInt32();
bitField0_ |= 0x00000002;
break;
} // case 16
case 24: {
length_ = input.readInt32();
bitField0_ |= 0x00000004;
break;
} // case 24
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int controller_ ;
/**
* int32 controller = 1;
* @return The controller.
*/
@java.lang.Override
public int getController() {
return controller_;
}
/**
* int32 controller = 1;
* @param value The controller to set.
* @return This builder for chaining.
*/
public Builder setController(int value) {
controller_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* int32 controller = 1;
* @return This builder for chaining.
*/
public Builder clearController() {
bitField0_ = (bitField0_ & ~0x00000001);
controller_ = 0;
onChanged();
return this;
}
private int address_ ;
/**
* int32 address = 2;
* @return The address.
*/
@java.lang.Override
public int getAddress() {
return address_;
}
/**
* int32 address = 2;
* @param value The address to set.
* @return This builder for chaining.
*/
public Builder setAddress(int value) {
address_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* int32 address = 2;
* @return This builder for chaining.
*/
public Builder clearAddress() {
bitField0_ = (bitField0_ & ~0x00000002);
address_ = 0;
onChanged();
return this;
}
private int length_ ;
/**
* int32 length = 3;
* @return The length.
*/
@java.lang.Override
public int getLength() {
return length_;
}
/**
* int32 length = 3;
* @param value The length to set.
* @return This builder for chaining.
*/
public Builder setLength(int value) {
length_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* int32 length = 3;
* @return This builder for chaining.
*/
public Builder clearLength() {
bitField0_ = (bitField0_ & ~0x00000004);
length_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:diozero.I2C.NumBytes)
}
// @@protoc_insertion_point(class_scope:diozero.I2C.NumBytes)
private static final com.diozero.remote.message.protobuf.I2C.NumBytes DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.diozero.remote.message.protobuf.I2C.NumBytes();
}
public static com.diozero.remote.message.protobuf.I2C.NumBytes getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public NumBytes parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.NumBytes getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ByteArrayOrBuilder extends
// @@protoc_insertion_point(interface_extends:diozero.I2C.ByteArray)
com.google.protobuf.MessageOrBuilder {
/**
* int32 controller = 1;
* @return The controller.
*/
int getController();
/**
* int32 address = 2;
* @return The address.
*/
int getAddress();
/**
* bytes data = 3;
* @return The data.
*/
com.google.protobuf.ByteString getData();
}
/**
* Protobuf type {@code diozero.I2C.ByteArray}
*/
public static final class ByteArray extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:diozero.I2C.ByteArray)
ByteArrayOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
ByteArray.class.getName());
}
// Use ByteArray.newBuilder() to construct.
private ByteArray(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private ByteArray() {
data_ = com.google.protobuf.ByteString.EMPTY;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_ByteArray_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_ByteArray_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.I2C.ByteArray.class, com.diozero.remote.message.protobuf.I2C.ByteArray.Builder.class);
}
public static final int CONTROLLER_FIELD_NUMBER = 1;
private int controller_ = 0;
/**
* int32 controller = 1;
* @return The controller.
*/
@java.lang.Override
public int getController() {
return controller_;
}
public static final int ADDRESS_FIELD_NUMBER = 2;
private int address_ = 0;
/**
* int32 address = 2;
* @return The address.
*/
@java.lang.Override
public int getAddress() {
return address_;
}
public static final int DATA_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString data_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes data = 3;
* @return The data.
*/
@java.lang.Override
public com.google.protobuf.ByteString getData() {
return data_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (controller_ != 0) {
output.writeInt32(1, controller_);
}
if (address_ != 0) {
output.writeInt32(2, address_);
}
if (!data_.isEmpty()) {
output.writeBytes(3, data_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (controller_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, controller_);
}
if (address_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, address_);
}
if (!data_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, data_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.diozero.remote.message.protobuf.I2C.ByteArray)) {
return super.equals(obj);
}
com.diozero.remote.message.protobuf.I2C.ByteArray other = (com.diozero.remote.message.protobuf.I2C.ByteArray) obj;
if (getController()
!= other.getController()) return false;
if (getAddress()
!= other.getAddress()) return false;
if (!getData()
.equals(other.getData())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CONTROLLER_FIELD_NUMBER;
hash = (53 * hash) + getController();
hash = (37 * hash) + ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getAddress();
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + getData().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.diozero.remote.message.protobuf.I2C.ByteArray parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.ByteArray parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.ByteArray parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.ByteArray parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.ByteArray parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.ByteArray parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.ByteArray parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.ByteArray parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.ByteArray parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.ByteArray parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.ByteArray parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.ByteArray parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.diozero.remote.message.protobuf.I2C.ByteArray prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code diozero.I2C.ByteArray}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:diozero.I2C.ByteArray)
com.diozero.remote.message.protobuf.I2C.ByteArrayOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_ByteArray_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_ByteArray_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.I2C.ByteArray.class, com.diozero.remote.message.protobuf.I2C.ByteArray.Builder.class);
}
// Construct using com.diozero.remote.message.protobuf.I2C.ByteArray.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
controller_ = 0;
address_ = 0;
data_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_ByteArray_descriptor;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.ByteArray getDefaultInstanceForType() {
return com.diozero.remote.message.protobuf.I2C.ByteArray.getDefaultInstance();
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.ByteArray build() {
com.diozero.remote.message.protobuf.I2C.ByteArray result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.ByteArray buildPartial() {
com.diozero.remote.message.protobuf.I2C.ByteArray result = new com.diozero.remote.message.protobuf.I2C.ByteArray(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.diozero.remote.message.protobuf.I2C.ByteArray result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.controller_ = controller_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.address_ = address_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.data_ = data_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.diozero.remote.message.protobuf.I2C.ByteArray) {
return mergeFrom((com.diozero.remote.message.protobuf.I2C.ByteArray)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.diozero.remote.message.protobuf.I2C.ByteArray other) {
if (other == com.diozero.remote.message.protobuf.I2C.ByteArray.getDefaultInstance()) return this;
if (other.getController() != 0) {
setController(other.getController());
}
if (other.getAddress() != 0) {
setAddress(other.getAddress());
}
if (other.getData() != com.google.protobuf.ByteString.EMPTY) {
setData(other.getData());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
controller_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
address_ = input.readInt32();
bitField0_ |= 0x00000002;
break;
} // case 16
case 26: {
data_ = input.readBytes();
bitField0_ |= 0x00000004;
break;
} // case 26
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int controller_ ;
/**
* int32 controller = 1;
* @return The controller.
*/
@java.lang.Override
public int getController() {
return controller_;
}
/**
* int32 controller = 1;
* @param value The controller to set.
* @return This builder for chaining.
*/
public Builder setController(int value) {
controller_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* int32 controller = 1;
* @return This builder for chaining.
*/
public Builder clearController() {
bitField0_ = (bitField0_ & ~0x00000001);
controller_ = 0;
onChanged();
return this;
}
private int address_ ;
/**
* int32 address = 2;
* @return The address.
*/
@java.lang.Override
public int getAddress() {
return address_;
}
/**
* int32 address = 2;
* @param value The address to set.
* @return This builder for chaining.
*/
public Builder setAddress(int value) {
address_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* int32 address = 2;
* @return This builder for chaining.
*/
public Builder clearAddress() {
bitField0_ = (bitField0_ & ~0x00000002);
address_ = 0;
onChanged();
return this;
}
private com.google.protobuf.ByteString data_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes data = 3;
* @return The data.
*/
@java.lang.Override
public com.google.protobuf.ByteString getData() {
return data_;
}
/**
* bytes data = 3;
* @param value The data to set.
* @return This builder for chaining.
*/
public Builder setData(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
data_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* bytes data = 3;
* @return This builder for chaining.
*/
public Builder clearData() {
bitField0_ = (bitField0_ & ~0x00000004);
data_ = getDefaultInstance().getData();
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:diozero.I2C.ByteArray)
}
// @@protoc_insertion_point(class_scope:diozero.I2C.ByteArray)
private static final com.diozero.remote.message.protobuf.I2C.ByteArray DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.diozero.remote.message.protobuf.I2C.ByteArray();
}
public static com.diozero.remote.message.protobuf.I2C.ByteArray getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ByteArray parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.ByteArray getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface I2CMessageOrBuilder extends
// @@protoc_insertion_point(interface_extends:diozero.I2C.I2CMessage)
com.google.protobuf.MessageOrBuilder {
/**
* int32 flags = 1;
* @return The flags.
*/
int getFlags();
/**
* int32 len = 2;
* @return The len.
*/
int getLen();
}
/**
* Protobuf type {@code diozero.I2C.I2CMessage}
*/
public static final class I2CMessage extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:diozero.I2C.I2CMessage)
I2CMessageOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
I2CMessage.class.getName());
}
// Use I2CMessage.newBuilder() to construct.
private I2CMessage(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private I2CMessage() {
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_I2CMessage_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_I2CMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.I2C.I2CMessage.class, com.diozero.remote.message.protobuf.I2C.I2CMessage.Builder.class);
}
public static final int FLAGS_FIELD_NUMBER = 1;
private int flags_ = 0;
/**
* int32 flags = 1;
* @return The flags.
*/
@java.lang.Override
public int getFlags() {
return flags_;
}
public static final int LEN_FIELD_NUMBER = 2;
private int len_ = 0;
/**
* int32 len = 2;
* @return The len.
*/
@java.lang.Override
public int getLen() {
return len_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (flags_ != 0) {
output.writeInt32(1, flags_);
}
if (len_ != 0) {
output.writeInt32(2, len_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (flags_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, flags_);
}
if (len_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, len_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.diozero.remote.message.protobuf.I2C.I2CMessage)) {
return super.equals(obj);
}
com.diozero.remote.message.protobuf.I2C.I2CMessage other = (com.diozero.remote.message.protobuf.I2C.I2CMessage) obj;
if (getFlags()
!= other.getFlags()) return false;
if (getLen()
!= other.getLen()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + FLAGS_FIELD_NUMBER;
hash = (53 * hash) + getFlags();
hash = (37 * hash) + LEN_FIELD_NUMBER;
hash = (53 * hash) + getLen();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.diozero.remote.message.protobuf.I2C.I2CMessage parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.I2CMessage parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.I2CMessage parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.I2CMessage parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.I2CMessage parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.I2CMessage parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.I2CMessage parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.I2CMessage parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.I2CMessage parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.I2CMessage parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.I2CMessage parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.I2CMessage parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.diozero.remote.message.protobuf.I2C.I2CMessage prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code diozero.I2C.I2CMessage}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:diozero.I2C.I2CMessage)
com.diozero.remote.message.protobuf.I2C.I2CMessageOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_I2CMessage_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_I2CMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.I2C.I2CMessage.class, com.diozero.remote.message.protobuf.I2C.I2CMessage.Builder.class);
}
// Construct using com.diozero.remote.message.protobuf.I2C.I2CMessage.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
flags_ = 0;
len_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_I2CMessage_descriptor;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.I2CMessage getDefaultInstanceForType() {
return com.diozero.remote.message.protobuf.I2C.I2CMessage.getDefaultInstance();
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.I2CMessage build() {
com.diozero.remote.message.protobuf.I2C.I2CMessage result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.I2CMessage buildPartial() {
com.diozero.remote.message.protobuf.I2C.I2CMessage result = new com.diozero.remote.message.protobuf.I2C.I2CMessage(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.diozero.remote.message.protobuf.I2C.I2CMessage result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.flags_ = flags_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.len_ = len_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.diozero.remote.message.protobuf.I2C.I2CMessage) {
return mergeFrom((com.diozero.remote.message.protobuf.I2C.I2CMessage)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.diozero.remote.message.protobuf.I2C.I2CMessage other) {
if (other == com.diozero.remote.message.protobuf.I2C.I2CMessage.getDefaultInstance()) return this;
if (other.getFlags() != 0) {
setFlags(other.getFlags());
}
if (other.getLen() != 0) {
setLen(other.getLen());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
flags_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
len_ = input.readInt32();
bitField0_ |= 0x00000002;
break;
} // case 16
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int flags_ ;
/**
* int32 flags = 1;
* @return The flags.
*/
@java.lang.Override
public int getFlags() {
return flags_;
}
/**
* int32 flags = 1;
* @param value The flags to set.
* @return This builder for chaining.
*/
public Builder setFlags(int value) {
flags_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* int32 flags = 1;
* @return This builder for chaining.
*/
public Builder clearFlags() {
bitField0_ = (bitField0_ & ~0x00000001);
flags_ = 0;
onChanged();
return this;
}
private int len_ ;
/**
* int32 len = 2;
* @return The len.
*/
@java.lang.Override
public int getLen() {
return len_;
}
/**
* int32 len = 2;
* @param value The len to set.
* @return This builder for chaining.
*/
public Builder setLen(int value) {
len_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* int32 len = 2;
* @return This builder for chaining.
*/
public Builder clearLen() {
bitField0_ = (bitField0_ & ~0x00000002);
len_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:diozero.I2C.I2CMessage)
}
// @@protoc_insertion_point(class_scope:diozero.I2C.I2CMessage)
private static final com.diozero.remote.message.protobuf.I2C.I2CMessage DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.diozero.remote.message.protobuf.I2C.I2CMessage();
}
public static com.diozero.remote.message.protobuf.I2C.I2CMessage getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public I2CMessage parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.I2CMessage getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ReadWriteOrBuilder extends
// @@protoc_insertion_point(interface_extends:diozero.I2C.ReadWrite)
com.google.protobuf.MessageOrBuilder {
/**
* int32 controller = 1;
* @return The controller.
*/
int getController();
/**
* int32 address = 2;
* @return The address.
*/
int getAddress();
/**
* repeated .diozero.I2C.I2CMessage message = 3;
*/
java.util.List
getMessageList();
/**
* repeated .diozero.I2C.I2CMessage message = 3;
*/
com.diozero.remote.message.protobuf.I2C.I2CMessage getMessage(int index);
/**
* repeated .diozero.I2C.I2CMessage message = 3;
*/
int getMessageCount();
/**
* repeated .diozero.I2C.I2CMessage message = 3;
*/
java.util.List extends com.diozero.remote.message.protobuf.I2C.I2CMessageOrBuilder>
getMessageOrBuilderList();
/**
* repeated .diozero.I2C.I2CMessage message = 3;
*/
com.diozero.remote.message.protobuf.I2C.I2CMessageOrBuilder getMessageOrBuilder(
int index);
/**
* bytes data = 4;
* @return The data.
*/
com.google.protobuf.ByteString getData();
}
/**
* Protobuf type {@code diozero.I2C.ReadWrite}
*/
public static final class ReadWrite extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:diozero.I2C.ReadWrite)
ReadWriteOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
ReadWrite.class.getName());
}
// Use ReadWrite.newBuilder() to construct.
private ReadWrite(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private ReadWrite() {
message_ = java.util.Collections.emptyList();
data_ = com.google.protobuf.ByteString.EMPTY;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_ReadWrite_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_ReadWrite_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.I2C.ReadWrite.class, com.diozero.remote.message.protobuf.I2C.ReadWrite.Builder.class);
}
public static final int CONTROLLER_FIELD_NUMBER = 1;
private int controller_ = 0;
/**
* int32 controller = 1;
* @return The controller.
*/
@java.lang.Override
public int getController() {
return controller_;
}
public static final int ADDRESS_FIELD_NUMBER = 2;
private int address_ = 0;
/**
* int32 address = 2;
* @return The address.
*/
@java.lang.Override
public int getAddress() {
return address_;
}
public static final int MESSAGE_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private java.util.List message_;
/**
* repeated .diozero.I2C.I2CMessage message = 3;
*/
@java.lang.Override
public java.util.List getMessageList() {
return message_;
}
/**
* repeated .diozero.I2C.I2CMessage message = 3;
*/
@java.lang.Override
public java.util.List extends com.diozero.remote.message.protobuf.I2C.I2CMessageOrBuilder>
getMessageOrBuilderList() {
return message_;
}
/**
* repeated .diozero.I2C.I2CMessage message = 3;
*/
@java.lang.Override
public int getMessageCount() {
return message_.size();
}
/**
* repeated .diozero.I2C.I2CMessage message = 3;
*/
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.I2CMessage getMessage(int index) {
return message_.get(index);
}
/**
* repeated .diozero.I2C.I2CMessage message = 3;
*/
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.I2CMessageOrBuilder getMessageOrBuilder(
int index) {
return message_.get(index);
}
public static final int DATA_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString data_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes data = 4;
* @return The data.
*/
@java.lang.Override
public com.google.protobuf.ByteString getData() {
return data_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (controller_ != 0) {
output.writeInt32(1, controller_);
}
if (address_ != 0) {
output.writeInt32(2, address_);
}
for (int i = 0; i < message_.size(); i++) {
output.writeMessage(3, message_.get(i));
}
if (!data_.isEmpty()) {
output.writeBytes(4, data_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (controller_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, controller_);
}
if (address_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, address_);
}
for (int i = 0; i < message_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, message_.get(i));
}
if (!data_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, data_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.diozero.remote.message.protobuf.I2C.ReadWrite)) {
return super.equals(obj);
}
com.diozero.remote.message.protobuf.I2C.ReadWrite other = (com.diozero.remote.message.protobuf.I2C.ReadWrite) obj;
if (getController()
!= other.getController()) return false;
if (getAddress()
!= other.getAddress()) return false;
if (!getMessageList()
.equals(other.getMessageList())) return false;
if (!getData()
.equals(other.getData())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CONTROLLER_FIELD_NUMBER;
hash = (53 * hash) + getController();
hash = (37 * hash) + ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getAddress();
if (getMessageCount() > 0) {
hash = (37 * hash) + MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getMessageList().hashCode();
}
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + getData().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.diozero.remote.message.protobuf.I2C.ReadWrite parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.ReadWrite parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.ReadWrite parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.ReadWrite parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.ReadWrite parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.ReadWrite parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.ReadWrite parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.ReadWrite parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.ReadWrite parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.ReadWrite parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.ReadWrite parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.ReadWrite parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.diozero.remote.message.protobuf.I2C.ReadWrite prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code diozero.I2C.ReadWrite}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:diozero.I2C.ReadWrite)
com.diozero.remote.message.protobuf.I2C.ReadWriteOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_ReadWrite_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_ReadWrite_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.I2C.ReadWrite.class, com.diozero.remote.message.protobuf.I2C.ReadWrite.Builder.class);
}
// Construct using com.diozero.remote.message.protobuf.I2C.ReadWrite.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
controller_ = 0;
address_ = 0;
if (messageBuilder_ == null) {
message_ = java.util.Collections.emptyList();
} else {
message_ = null;
messageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
data_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_ReadWrite_descriptor;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.ReadWrite getDefaultInstanceForType() {
return com.diozero.remote.message.protobuf.I2C.ReadWrite.getDefaultInstance();
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.ReadWrite build() {
com.diozero.remote.message.protobuf.I2C.ReadWrite result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.ReadWrite buildPartial() {
com.diozero.remote.message.protobuf.I2C.ReadWrite result = new com.diozero.remote.message.protobuf.I2C.ReadWrite(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.diozero.remote.message.protobuf.I2C.ReadWrite result) {
if (messageBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)) {
message_ = java.util.Collections.unmodifiableList(message_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.message_ = message_;
} else {
result.message_ = messageBuilder_.build();
}
}
private void buildPartial0(com.diozero.remote.message.protobuf.I2C.ReadWrite result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.controller_ = controller_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.address_ = address_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.data_ = data_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.diozero.remote.message.protobuf.I2C.ReadWrite) {
return mergeFrom((com.diozero.remote.message.protobuf.I2C.ReadWrite)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.diozero.remote.message.protobuf.I2C.ReadWrite other) {
if (other == com.diozero.remote.message.protobuf.I2C.ReadWrite.getDefaultInstance()) return this;
if (other.getController() != 0) {
setController(other.getController());
}
if (other.getAddress() != 0) {
setAddress(other.getAddress());
}
if (messageBuilder_ == null) {
if (!other.message_.isEmpty()) {
if (message_.isEmpty()) {
message_ = other.message_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureMessageIsMutable();
message_.addAll(other.message_);
}
onChanged();
}
} else {
if (!other.message_.isEmpty()) {
if (messageBuilder_.isEmpty()) {
messageBuilder_.dispose();
messageBuilder_ = null;
message_ = other.message_;
bitField0_ = (bitField0_ & ~0x00000004);
messageBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getMessageFieldBuilder() : null;
} else {
messageBuilder_.addAllMessages(other.message_);
}
}
}
if (other.getData() != com.google.protobuf.ByteString.EMPTY) {
setData(other.getData());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
controller_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
address_ = input.readInt32();
bitField0_ |= 0x00000002;
break;
} // case 16
case 26: {
com.diozero.remote.message.protobuf.I2C.I2CMessage m =
input.readMessage(
com.diozero.remote.message.protobuf.I2C.I2CMessage.parser(),
extensionRegistry);
if (messageBuilder_ == null) {
ensureMessageIsMutable();
message_.add(m);
} else {
messageBuilder_.addMessage(m);
}
break;
} // case 26
case 34: {
data_ = input.readBytes();
bitField0_ |= 0x00000008;
break;
} // case 34
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int controller_ ;
/**
* int32 controller = 1;
* @return The controller.
*/
@java.lang.Override
public int getController() {
return controller_;
}
/**
* int32 controller = 1;
* @param value The controller to set.
* @return This builder for chaining.
*/
public Builder setController(int value) {
controller_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* int32 controller = 1;
* @return This builder for chaining.
*/
public Builder clearController() {
bitField0_ = (bitField0_ & ~0x00000001);
controller_ = 0;
onChanged();
return this;
}
private int address_ ;
/**
* int32 address = 2;
* @return The address.
*/
@java.lang.Override
public int getAddress() {
return address_;
}
/**
* int32 address = 2;
* @param value The address to set.
* @return This builder for chaining.
*/
public Builder setAddress(int value) {
address_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* int32 address = 2;
* @return This builder for chaining.
*/
public Builder clearAddress() {
bitField0_ = (bitField0_ & ~0x00000002);
address_ = 0;
onChanged();
return this;
}
private java.util.List message_ =
java.util.Collections.emptyList();
private void ensureMessageIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
message_ = new java.util.ArrayList(message_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.diozero.remote.message.protobuf.I2C.I2CMessage, com.diozero.remote.message.protobuf.I2C.I2CMessage.Builder, com.diozero.remote.message.protobuf.I2C.I2CMessageOrBuilder> messageBuilder_;
/**
* repeated .diozero.I2C.I2CMessage message = 3;
*/
public java.util.List getMessageList() {
if (messageBuilder_ == null) {
return java.util.Collections.unmodifiableList(message_);
} else {
return messageBuilder_.getMessageList();
}
}
/**
* repeated .diozero.I2C.I2CMessage message = 3;
*/
public int getMessageCount() {
if (messageBuilder_ == null) {
return message_.size();
} else {
return messageBuilder_.getCount();
}
}
/**
* repeated .diozero.I2C.I2CMessage message = 3;
*/
public com.diozero.remote.message.protobuf.I2C.I2CMessage getMessage(int index) {
if (messageBuilder_ == null) {
return message_.get(index);
} else {
return messageBuilder_.getMessage(index);
}
}
/**
* repeated .diozero.I2C.I2CMessage message = 3;
*/
public Builder setMessage(
int index, com.diozero.remote.message.protobuf.I2C.I2CMessage value) {
if (messageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMessageIsMutable();
message_.set(index, value);
onChanged();
} else {
messageBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .diozero.I2C.I2CMessage message = 3;
*/
public Builder setMessage(
int index, com.diozero.remote.message.protobuf.I2C.I2CMessage.Builder builderForValue) {
if (messageBuilder_ == null) {
ensureMessageIsMutable();
message_.set(index, builderForValue.build());
onChanged();
} else {
messageBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .diozero.I2C.I2CMessage message = 3;
*/
public Builder addMessage(com.diozero.remote.message.protobuf.I2C.I2CMessage value) {
if (messageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMessageIsMutable();
message_.add(value);
onChanged();
} else {
messageBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .diozero.I2C.I2CMessage message = 3;
*/
public Builder addMessage(
int index, com.diozero.remote.message.protobuf.I2C.I2CMessage value) {
if (messageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMessageIsMutable();
message_.add(index, value);
onChanged();
} else {
messageBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .diozero.I2C.I2CMessage message = 3;
*/
public Builder addMessage(
com.diozero.remote.message.protobuf.I2C.I2CMessage.Builder builderForValue) {
if (messageBuilder_ == null) {
ensureMessageIsMutable();
message_.add(builderForValue.build());
onChanged();
} else {
messageBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .diozero.I2C.I2CMessage message = 3;
*/
public Builder addMessage(
int index, com.diozero.remote.message.protobuf.I2C.I2CMessage.Builder builderForValue) {
if (messageBuilder_ == null) {
ensureMessageIsMutable();
message_.add(index, builderForValue.build());
onChanged();
} else {
messageBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .diozero.I2C.I2CMessage message = 3;
*/
public Builder addAllMessage(
java.lang.Iterable extends com.diozero.remote.message.protobuf.I2C.I2CMessage> values) {
if (messageBuilder_ == null) {
ensureMessageIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, message_);
onChanged();
} else {
messageBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .diozero.I2C.I2CMessage message = 3;
*/
public Builder clearMessage() {
if (messageBuilder_ == null) {
message_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
messageBuilder_.clear();
}
return this;
}
/**
* repeated .diozero.I2C.I2CMessage message = 3;
*/
public Builder removeMessage(int index) {
if (messageBuilder_ == null) {
ensureMessageIsMutable();
message_.remove(index);
onChanged();
} else {
messageBuilder_.remove(index);
}
return this;
}
/**
* repeated .diozero.I2C.I2CMessage message = 3;
*/
public com.diozero.remote.message.protobuf.I2C.I2CMessage.Builder getMessageBuilder(
int index) {
return getMessageFieldBuilder().getBuilder(index);
}
/**
* repeated .diozero.I2C.I2CMessage message = 3;
*/
public com.diozero.remote.message.protobuf.I2C.I2CMessageOrBuilder getMessageOrBuilder(
int index) {
if (messageBuilder_ == null) {
return message_.get(index); } else {
return messageBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .diozero.I2C.I2CMessage message = 3;
*/
public java.util.List extends com.diozero.remote.message.protobuf.I2C.I2CMessageOrBuilder>
getMessageOrBuilderList() {
if (messageBuilder_ != null) {
return messageBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(message_);
}
}
/**
* repeated .diozero.I2C.I2CMessage message = 3;
*/
public com.diozero.remote.message.protobuf.I2C.I2CMessage.Builder addMessageBuilder() {
return getMessageFieldBuilder().addBuilder(
com.diozero.remote.message.protobuf.I2C.I2CMessage.getDefaultInstance());
}
/**
* repeated .diozero.I2C.I2CMessage message = 3;
*/
public com.diozero.remote.message.protobuf.I2C.I2CMessage.Builder addMessageBuilder(
int index) {
return getMessageFieldBuilder().addBuilder(
index, com.diozero.remote.message.protobuf.I2C.I2CMessage.getDefaultInstance());
}
/**
* repeated .diozero.I2C.I2CMessage message = 3;
*/
public java.util.List
getMessageBuilderList() {
return getMessageFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.diozero.remote.message.protobuf.I2C.I2CMessage, com.diozero.remote.message.protobuf.I2C.I2CMessage.Builder, com.diozero.remote.message.protobuf.I2C.I2CMessageOrBuilder>
getMessageFieldBuilder() {
if (messageBuilder_ == null) {
messageBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.diozero.remote.message.protobuf.I2C.I2CMessage, com.diozero.remote.message.protobuf.I2C.I2CMessage.Builder, com.diozero.remote.message.protobuf.I2C.I2CMessageOrBuilder>(
message_,
((bitField0_ & 0x00000004) != 0),
getParentForChildren(),
isClean());
message_ = null;
}
return messageBuilder_;
}
private com.google.protobuf.ByteString data_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes data = 4;
* @return The data.
*/
@java.lang.Override
public com.google.protobuf.ByteString getData() {
return data_;
}
/**
* bytes data = 4;
* @param value The data to set.
* @return This builder for chaining.
*/
public Builder setData(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
data_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* bytes data = 4;
* @return This builder for chaining.
*/
public Builder clearData() {
bitField0_ = (bitField0_ & ~0x00000008);
data_ = getDefaultInstance().getData();
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:diozero.I2C.ReadWrite)
}
// @@protoc_insertion_point(class_scope:diozero.I2C.ReadWrite)
private static final com.diozero.remote.message.protobuf.I2C.ReadWrite DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.diozero.remote.message.protobuf.I2C.ReadWrite();
}
public static com.diozero.remote.message.protobuf.I2C.ReadWrite getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ReadWrite parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.ReadWrite getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ByteArrayWithLengthResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:diozero.I2C.ByteArrayWithLengthResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .diozero.Status status = 1;
* @return The enum numeric value on the wire for status.
*/
int getStatusValue();
/**
* .diozero.Status status = 1;
* @return The status.
*/
com.diozero.remote.message.protobuf.Status getStatus();
/**
* string detail = 2;
* @return The detail.
*/
java.lang.String getDetail();
/**
* string detail = 2;
* @return The bytes for detail.
*/
com.google.protobuf.ByteString
getDetailBytes();
/**
* int32 bytesRead = 3;
* @return The bytesRead.
*/
int getBytesRead();
/**
* bytes data = 4;
* @return The data.
*/
com.google.protobuf.ByteString getData();
}
/**
* Protobuf type {@code diozero.I2C.ByteArrayWithLengthResponse}
*/
public static final class ByteArrayWithLengthResponse extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:diozero.I2C.ByteArrayWithLengthResponse)
ByteArrayWithLengthResponseOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
ByteArrayWithLengthResponse.class.getName());
}
// Use ByteArrayWithLengthResponse.newBuilder() to construct.
private ByteArrayWithLengthResponse(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private ByteArrayWithLengthResponse() {
status_ = 0;
detail_ = "";
data_ = com.google.protobuf.ByteString.EMPTY;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_ByteArrayWithLengthResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_ByteArrayWithLengthResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse.class, com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse.Builder.class);
}
public static final int STATUS_FIELD_NUMBER = 1;
private int status_ = 0;
/**
* .diozero.Status status = 1;
* @return The enum numeric value on the wire for status.
*/
@java.lang.Override public int getStatusValue() {
return status_;
}
/**
* .diozero.Status status = 1;
* @return The status.
*/
@java.lang.Override public com.diozero.remote.message.protobuf.Status getStatus() {
com.diozero.remote.message.protobuf.Status result = com.diozero.remote.message.protobuf.Status.forNumber(status_);
return result == null ? com.diozero.remote.message.protobuf.Status.UNRECOGNIZED : result;
}
public static final int DETAIL_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object detail_ = "";
/**
* string detail = 2;
* @return The detail.
*/
@java.lang.Override
public java.lang.String getDetail() {
java.lang.Object ref = detail_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
detail_ = s;
return s;
}
}
/**
* string detail = 2;
* @return The bytes for detail.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDetailBytes() {
java.lang.Object ref = detail_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
detail_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int BYTESREAD_FIELD_NUMBER = 3;
private int bytesRead_ = 0;
/**
* int32 bytesRead = 3;
* @return The bytesRead.
*/
@java.lang.Override
public int getBytesRead() {
return bytesRead_;
}
public static final int DATA_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString data_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes data = 4;
* @return The data.
*/
@java.lang.Override
public com.google.protobuf.ByteString getData() {
return data_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (status_ != com.diozero.remote.message.protobuf.Status.OK.getNumber()) {
output.writeEnum(1, status_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(detail_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 2, detail_);
}
if (bytesRead_ != 0) {
output.writeInt32(3, bytesRead_);
}
if (!data_.isEmpty()) {
output.writeBytes(4, data_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (status_ != com.diozero.remote.message.protobuf.Status.OK.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, status_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(detail_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(2, detail_);
}
if (bytesRead_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, bytesRead_);
}
if (!data_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, data_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse)) {
return super.equals(obj);
}
com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse other = (com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse) obj;
if (status_ != other.status_) return false;
if (!getDetail()
.equals(other.getDetail())) return false;
if (getBytesRead()
!= other.getBytesRead()) return false;
if (!getData()
.equals(other.getData())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + status_;
hash = (37 * hash) + DETAIL_FIELD_NUMBER;
hash = (53 * hash) + getDetail().hashCode();
hash = (37 * hash) + BYTESREAD_FIELD_NUMBER;
hash = (53 * hash) + getBytesRead();
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + getData().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code diozero.I2C.ByteArrayWithLengthResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:diozero.I2C.ByteArrayWithLengthResponse)
com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_ByteArrayWithLengthResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_ByteArrayWithLengthResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse.class, com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse.Builder.class);
}
// Construct using com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
status_ = 0;
detail_ = "";
bytesRead_ = 0;
data_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_ByteArrayWithLengthResponse_descriptor;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse getDefaultInstanceForType() {
return com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse.getDefaultInstance();
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse build() {
com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse buildPartial() {
com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse result = new com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.status_ = status_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.detail_ = detail_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.bytesRead_ = bytesRead_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.data_ = data_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse) {
return mergeFrom((com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse other) {
if (other == com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse.getDefaultInstance()) return this;
if (other.status_ != 0) {
setStatusValue(other.getStatusValue());
}
if (!other.getDetail().isEmpty()) {
detail_ = other.detail_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.getBytesRead() != 0) {
setBytesRead(other.getBytesRead());
}
if (other.getData() != com.google.protobuf.ByteString.EMPTY) {
setData(other.getData());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
status_ = input.readEnum();
bitField0_ |= 0x00000001;
break;
} // case 8
case 18: {
detail_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 18
case 24: {
bytesRead_ = input.readInt32();
bitField0_ |= 0x00000004;
break;
} // case 24
case 34: {
data_ = input.readBytes();
bitField0_ |= 0x00000008;
break;
} // case 34
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int status_ = 0;
/**
* .diozero.Status status = 1;
* @return The enum numeric value on the wire for status.
*/
@java.lang.Override public int getStatusValue() {
return status_;
}
/**
* .diozero.Status status = 1;
* @param value The enum numeric value on the wire for status to set.
* @return This builder for chaining.
*/
public Builder setStatusValue(int value) {
status_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .diozero.Status status = 1;
* @return The status.
*/
@java.lang.Override
public com.diozero.remote.message.protobuf.Status getStatus() {
com.diozero.remote.message.protobuf.Status result = com.diozero.remote.message.protobuf.Status.forNumber(status_);
return result == null ? com.diozero.remote.message.protobuf.Status.UNRECOGNIZED : result;
}
/**
* .diozero.Status status = 1;
* @param value The status to set.
* @return This builder for chaining.
*/
public Builder setStatus(com.diozero.remote.message.protobuf.Status value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
status_ = value.getNumber();
onChanged();
return this;
}
/**
* .diozero.Status status = 1;
* @return This builder for chaining.
*/
public Builder clearStatus() {
bitField0_ = (bitField0_ & ~0x00000001);
status_ = 0;
onChanged();
return this;
}
private java.lang.Object detail_ = "";
/**
* string detail = 2;
* @return The detail.
*/
public java.lang.String getDetail() {
java.lang.Object ref = detail_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
detail_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string detail = 2;
* @return The bytes for detail.
*/
public com.google.protobuf.ByteString
getDetailBytes() {
java.lang.Object ref = detail_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
detail_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string detail = 2;
* @param value The detail to set.
* @return This builder for chaining.
*/
public Builder setDetail(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
detail_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* string detail = 2;
* @return This builder for chaining.
*/
public Builder clearDetail() {
detail_ = getDefaultInstance().getDetail();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* string detail = 2;
* @param value The bytes for detail to set.
* @return This builder for chaining.
*/
public Builder setDetailBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
detail_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private int bytesRead_ ;
/**
* int32 bytesRead = 3;
* @return The bytesRead.
*/
@java.lang.Override
public int getBytesRead() {
return bytesRead_;
}
/**
* int32 bytesRead = 3;
* @param value The bytesRead to set.
* @return This builder for chaining.
*/
public Builder setBytesRead(int value) {
bytesRead_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* int32 bytesRead = 3;
* @return This builder for chaining.
*/
public Builder clearBytesRead() {
bitField0_ = (bitField0_ & ~0x00000004);
bytesRead_ = 0;
onChanged();
return this;
}
private com.google.protobuf.ByteString data_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes data = 4;
* @return The data.
*/
@java.lang.Override
public com.google.protobuf.ByteString getData() {
return data_;
}
/**
* bytes data = 4;
* @param value The data to set.
* @return This builder for chaining.
*/
public Builder setData(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
data_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* bytes data = 4;
* @return This builder for chaining.
*/
public Builder clearData() {
bitField0_ = (bitField0_ & ~0x00000008);
data_ = getDefaultInstance().getData();
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:diozero.I2C.ByteArrayWithLengthResponse)
}
// @@protoc_insertion_point(class_scope:diozero.I2C.ByteArrayWithLengthResponse)
private static final com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse();
}
public static com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ByteArrayWithLengthResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.diozero.remote.message.protobuf.I2C)) {
return super.equals(obj);
}
com.diozero.remote.message.protobuf.I2C other = (com.diozero.remote.message.protobuf.I2C) obj;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.diozero.remote.message.protobuf.I2C parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.diozero.remote.message.protobuf.I2C parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.diozero.remote.message.protobuf.I2C parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.diozero.remote.message.protobuf.I2C parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.diozero.remote.message.protobuf.I2C prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code diozero.I2C}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:diozero.I2C)
com.diozero.remote.message.protobuf.I2COrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.diozero.remote.message.protobuf.I2C.class, com.diozero.remote.message.protobuf.I2C.Builder.class);
}
// Construct using com.diozero.remote.message.protobuf.I2C.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.diozero.remote.message.protobuf.Diozero.internal_static_diozero_I2C_descriptor;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C getDefaultInstanceForType() {
return com.diozero.remote.message.protobuf.I2C.getDefaultInstance();
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C build() {
com.diozero.remote.message.protobuf.I2C result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C buildPartial() {
com.diozero.remote.message.protobuf.I2C result = new com.diozero.remote.message.protobuf.I2C(this);
onBuilt();
return result;
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.diozero.remote.message.protobuf.I2C) {
return mergeFrom((com.diozero.remote.message.protobuf.I2C)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.diozero.remote.message.protobuf.I2C other) {
if (other == com.diozero.remote.message.protobuf.I2C.getDefaultInstance()) return this;
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
// @@protoc_insertion_point(builder_scope:diozero.I2C)
}
// @@protoc_insertion_point(class_scope:diozero.I2C)
private static final com.diozero.remote.message.protobuf.I2C DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.diozero.remote.message.protobuf.I2C();
}
public static com.diozero.remote.message.protobuf.I2C getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public I2C parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.diozero.remote.message.protobuf.I2C getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy