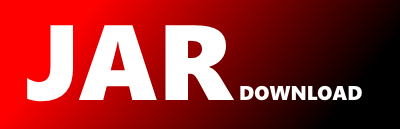
com.diozero.remote.message.protobuf.I2CServiceGrpc Maven / Gradle / Ivy
The newest version!
package com.diozero.remote.message.protobuf;
import static io.grpc.MethodDescriptor.generateFullMethodName;
/**
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.68.0)",
comments = "Source: diozero.proto")
@io.grpc.stub.annotations.GrpcGenerated
public final class I2CServiceGrpc {
private I2CServiceGrpc() {}
public static final java.lang.String SERVICE_NAME = "diozero.I2CService";
// Static method descriptors that strictly reflect the proto.
private static volatile io.grpc.MethodDescriptor getOpenMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Open",
requestType = com.diozero.remote.message.protobuf.I2C.Open.class,
responseType = com.diozero.remote.message.protobuf.Response.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getOpenMethod() {
io.grpc.MethodDescriptor getOpenMethod;
if ((getOpenMethod = I2CServiceGrpc.getOpenMethod) == null) {
synchronized (I2CServiceGrpc.class) {
if ((getOpenMethod = I2CServiceGrpc.getOpenMethod) == null) {
I2CServiceGrpc.getOpenMethod = getOpenMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "Open"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.I2C.Open.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Response.getDefaultInstance()))
.setSchemaDescriptor(new I2CServiceMethodDescriptorSupplier("Open"))
.build();
}
}
}
return getOpenMethod;
}
private static volatile io.grpc.MethodDescriptor getProbeMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Probe",
requestType = com.diozero.remote.message.protobuf.I2C.Probe.class,
responseType = com.diozero.remote.message.protobuf.BooleanResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getProbeMethod() {
io.grpc.MethodDescriptor getProbeMethod;
if ((getProbeMethod = I2CServiceGrpc.getProbeMethod) == null) {
synchronized (I2CServiceGrpc.class) {
if ((getProbeMethod = I2CServiceGrpc.getProbeMethod) == null) {
I2CServiceGrpc.getProbeMethod = getProbeMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "Probe"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.I2C.Probe.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.BooleanResponse.getDefaultInstance()))
.setSchemaDescriptor(new I2CServiceMethodDescriptorSupplier("Probe"))
.build();
}
}
}
return getProbeMethod;
}
private static volatile io.grpc.MethodDescriptor getWriteQuickMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "WriteQuick",
requestType = com.diozero.remote.message.protobuf.I2C.Bit.class,
responseType = com.diozero.remote.message.protobuf.Response.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getWriteQuickMethod() {
io.grpc.MethodDescriptor getWriteQuickMethod;
if ((getWriteQuickMethod = I2CServiceGrpc.getWriteQuickMethod) == null) {
synchronized (I2CServiceGrpc.class) {
if ((getWriteQuickMethod = I2CServiceGrpc.getWriteQuickMethod) == null) {
I2CServiceGrpc.getWriteQuickMethod = getWriteQuickMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "WriteQuick"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.I2C.Bit.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Response.getDefaultInstance()))
.setSchemaDescriptor(new I2CServiceMethodDescriptorSupplier("WriteQuick"))
.build();
}
}
}
return getWriteQuickMethod;
}
private static volatile io.grpc.MethodDescriptor getReadByteMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ReadByte",
requestType = com.diozero.remote.message.protobuf.I2C.Identifier.class,
responseType = com.diozero.remote.message.protobuf.ByteResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getReadByteMethod() {
io.grpc.MethodDescriptor getReadByteMethod;
if ((getReadByteMethod = I2CServiceGrpc.getReadByteMethod) == null) {
synchronized (I2CServiceGrpc.class) {
if ((getReadByteMethod = I2CServiceGrpc.getReadByteMethod) == null) {
I2CServiceGrpc.getReadByteMethod = getReadByteMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ReadByte"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.I2C.Identifier.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.ByteResponse.getDefaultInstance()))
.setSchemaDescriptor(new I2CServiceMethodDescriptorSupplier("ReadByte"))
.build();
}
}
}
return getReadByteMethod;
}
private static volatile io.grpc.MethodDescriptor getWriteByteMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "WriteByte",
requestType = com.diozero.remote.message.protobuf.I2C.ByteMessage.class,
responseType = com.diozero.remote.message.protobuf.Response.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getWriteByteMethod() {
io.grpc.MethodDescriptor getWriteByteMethod;
if ((getWriteByteMethod = I2CServiceGrpc.getWriteByteMethod) == null) {
synchronized (I2CServiceGrpc.class) {
if ((getWriteByteMethod = I2CServiceGrpc.getWriteByteMethod) == null) {
I2CServiceGrpc.getWriteByteMethod = getWriteByteMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "WriteByte"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.I2C.ByteMessage.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Response.getDefaultInstance()))
.setSchemaDescriptor(new I2CServiceMethodDescriptorSupplier("WriteByte"))
.build();
}
}
}
return getWriteByteMethod;
}
private static volatile io.grpc.MethodDescriptor getReadByteDataMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ReadByteData",
requestType = com.diozero.remote.message.protobuf.I2C.Register.class,
responseType = com.diozero.remote.message.protobuf.ByteResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getReadByteDataMethod() {
io.grpc.MethodDescriptor getReadByteDataMethod;
if ((getReadByteDataMethod = I2CServiceGrpc.getReadByteDataMethod) == null) {
synchronized (I2CServiceGrpc.class) {
if ((getReadByteDataMethod = I2CServiceGrpc.getReadByteDataMethod) == null) {
I2CServiceGrpc.getReadByteDataMethod = getReadByteDataMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ReadByteData"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.I2C.Register.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.ByteResponse.getDefaultInstance()))
.setSchemaDescriptor(new I2CServiceMethodDescriptorSupplier("ReadByteData"))
.build();
}
}
}
return getReadByteDataMethod;
}
private static volatile io.grpc.MethodDescriptor getWriteByteDataMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "WriteByteData",
requestType = com.diozero.remote.message.protobuf.I2C.RegisterAndByte.class,
responseType = com.diozero.remote.message.protobuf.Response.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getWriteByteDataMethod() {
io.grpc.MethodDescriptor getWriteByteDataMethod;
if ((getWriteByteDataMethod = I2CServiceGrpc.getWriteByteDataMethod) == null) {
synchronized (I2CServiceGrpc.class) {
if ((getWriteByteDataMethod = I2CServiceGrpc.getWriteByteDataMethod) == null) {
I2CServiceGrpc.getWriteByteDataMethod = getWriteByteDataMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "WriteByteData"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.I2C.RegisterAndByte.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Response.getDefaultInstance()))
.setSchemaDescriptor(new I2CServiceMethodDescriptorSupplier("WriteByteData"))
.build();
}
}
}
return getWriteByteDataMethod;
}
private static volatile io.grpc.MethodDescriptor getReadWordDataMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ReadWordData",
requestType = com.diozero.remote.message.protobuf.I2C.Register.class,
responseType = com.diozero.remote.message.protobuf.WordResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getReadWordDataMethod() {
io.grpc.MethodDescriptor getReadWordDataMethod;
if ((getReadWordDataMethod = I2CServiceGrpc.getReadWordDataMethod) == null) {
synchronized (I2CServiceGrpc.class) {
if ((getReadWordDataMethod = I2CServiceGrpc.getReadWordDataMethod) == null) {
I2CServiceGrpc.getReadWordDataMethod = getReadWordDataMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ReadWordData"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.I2C.Register.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.WordResponse.getDefaultInstance()))
.setSchemaDescriptor(new I2CServiceMethodDescriptorSupplier("ReadWordData"))
.build();
}
}
}
return getReadWordDataMethod;
}
private static volatile io.grpc.MethodDescriptor getWriteWordDataMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "WriteWordData",
requestType = com.diozero.remote.message.protobuf.I2C.RegisterAndWordData.class,
responseType = com.diozero.remote.message.protobuf.Response.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getWriteWordDataMethod() {
io.grpc.MethodDescriptor getWriteWordDataMethod;
if ((getWriteWordDataMethod = I2CServiceGrpc.getWriteWordDataMethod) == null) {
synchronized (I2CServiceGrpc.class) {
if ((getWriteWordDataMethod = I2CServiceGrpc.getWriteWordDataMethod) == null) {
I2CServiceGrpc.getWriteWordDataMethod = getWriteWordDataMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "WriteWordData"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.I2C.RegisterAndWordData.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Response.getDefaultInstance()))
.setSchemaDescriptor(new I2CServiceMethodDescriptorSupplier("WriteWordData"))
.build();
}
}
}
return getWriteWordDataMethod;
}
private static volatile io.grpc.MethodDescriptor getProcessCallMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ProcessCall",
requestType = com.diozero.remote.message.protobuf.I2C.RegisterAndWordData.class,
responseType = com.diozero.remote.message.protobuf.WordResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getProcessCallMethod() {
io.grpc.MethodDescriptor getProcessCallMethod;
if ((getProcessCallMethod = I2CServiceGrpc.getProcessCallMethod) == null) {
synchronized (I2CServiceGrpc.class) {
if ((getProcessCallMethod = I2CServiceGrpc.getProcessCallMethod) == null) {
I2CServiceGrpc.getProcessCallMethod = getProcessCallMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ProcessCall"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.I2C.RegisterAndWordData.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.WordResponse.getDefaultInstance()))
.setSchemaDescriptor(new I2CServiceMethodDescriptorSupplier("ProcessCall"))
.build();
}
}
}
return getProcessCallMethod;
}
private static volatile io.grpc.MethodDescriptor getReadBlockDataMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ReadBlockData",
requestType = com.diozero.remote.message.protobuf.I2C.Register.class,
responseType = com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getReadBlockDataMethod() {
io.grpc.MethodDescriptor getReadBlockDataMethod;
if ((getReadBlockDataMethod = I2CServiceGrpc.getReadBlockDataMethod) == null) {
synchronized (I2CServiceGrpc.class) {
if ((getReadBlockDataMethod = I2CServiceGrpc.getReadBlockDataMethod) == null) {
I2CServiceGrpc.getReadBlockDataMethod = getReadBlockDataMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ReadBlockData"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.I2C.Register.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse.getDefaultInstance()))
.setSchemaDescriptor(new I2CServiceMethodDescriptorSupplier("ReadBlockData"))
.build();
}
}
}
return getReadBlockDataMethod;
}
private static volatile io.grpc.MethodDescriptor getWriteBlockDataMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "WriteBlockData",
requestType = com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray.class,
responseType = com.diozero.remote.message.protobuf.Response.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getWriteBlockDataMethod() {
io.grpc.MethodDescriptor getWriteBlockDataMethod;
if ((getWriteBlockDataMethod = I2CServiceGrpc.getWriteBlockDataMethod) == null) {
synchronized (I2CServiceGrpc.class) {
if ((getWriteBlockDataMethod = I2CServiceGrpc.getWriteBlockDataMethod) == null) {
I2CServiceGrpc.getWriteBlockDataMethod = getWriteBlockDataMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "WriteBlockData"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Response.getDefaultInstance()))
.setSchemaDescriptor(new I2CServiceMethodDescriptorSupplier("WriteBlockData"))
.build();
}
}
}
return getWriteBlockDataMethod;
}
private static volatile io.grpc.MethodDescriptor getBlockProcessCallMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "BlockProcessCall",
requestType = com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray.class,
responseType = com.diozero.remote.message.protobuf.BytesResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getBlockProcessCallMethod() {
io.grpc.MethodDescriptor getBlockProcessCallMethod;
if ((getBlockProcessCallMethod = I2CServiceGrpc.getBlockProcessCallMethod) == null) {
synchronized (I2CServiceGrpc.class) {
if ((getBlockProcessCallMethod = I2CServiceGrpc.getBlockProcessCallMethod) == null) {
I2CServiceGrpc.getBlockProcessCallMethod = getBlockProcessCallMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "BlockProcessCall"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.BytesResponse.getDefaultInstance()))
.setSchemaDescriptor(new I2CServiceMethodDescriptorSupplier("BlockProcessCall"))
.build();
}
}
}
return getBlockProcessCallMethod;
}
private static volatile io.grpc.MethodDescriptor getReadI2CBlockDataMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ReadI2CBlockData",
requestType = com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes.class,
responseType = com.diozero.remote.message.protobuf.BytesResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getReadI2CBlockDataMethod() {
io.grpc.MethodDescriptor getReadI2CBlockDataMethod;
if ((getReadI2CBlockDataMethod = I2CServiceGrpc.getReadI2CBlockDataMethod) == null) {
synchronized (I2CServiceGrpc.class) {
if ((getReadI2CBlockDataMethod = I2CServiceGrpc.getReadI2CBlockDataMethod) == null) {
I2CServiceGrpc.getReadI2CBlockDataMethod = getReadI2CBlockDataMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ReadI2CBlockData"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.BytesResponse.getDefaultInstance()))
.setSchemaDescriptor(new I2CServiceMethodDescriptorSupplier("ReadI2CBlockData"))
.build();
}
}
}
return getReadI2CBlockDataMethod;
}
private static volatile io.grpc.MethodDescriptor getWriteI2CBlockDataMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "WriteI2CBlockData",
requestType = com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray.class,
responseType = com.diozero.remote.message.protobuf.Response.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getWriteI2CBlockDataMethod() {
io.grpc.MethodDescriptor getWriteI2CBlockDataMethod;
if ((getWriteI2CBlockDataMethod = I2CServiceGrpc.getWriteI2CBlockDataMethod) == null) {
synchronized (I2CServiceGrpc.class) {
if ((getWriteI2CBlockDataMethod = I2CServiceGrpc.getWriteI2CBlockDataMethod) == null) {
I2CServiceGrpc.getWriteI2CBlockDataMethod = getWriteI2CBlockDataMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "WriteI2CBlockData"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Response.getDefaultInstance()))
.setSchemaDescriptor(new I2CServiceMethodDescriptorSupplier("WriteI2CBlockData"))
.build();
}
}
}
return getWriteI2CBlockDataMethod;
}
private static volatile io.grpc.MethodDescriptor getReadBytesMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ReadBytes",
requestType = com.diozero.remote.message.protobuf.I2C.NumBytes.class,
responseType = com.diozero.remote.message.protobuf.BytesResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getReadBytesMethod() {
io.grpc.MethodDescriptor getReadBytesMethod;
if ((getReadBytesMethod = I2CServiceGrpc.getReadBytesMethod) == null) {
synchronized (I2CServiceGrpc.class) {
if ((getReadBytesMethod = I2CServiceGrpc.getReadBytesMethod) == null) {
I2CServiceGrpc.getReadBytesMethod = getReadBytesMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ReadBytes"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.I2C.NumBytes.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.BytesResponse.getDefaultInstance()))
.setSchemaDescriptor(new I2CServiceMethodDescriptorSupplier("ReadBytes"))
.build();
}
}
}
return getReadBytesMethod;
}
private static volatile io.grpc.MethodDescriptor getWriteBytesMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "WriteBytes",
requestType = com.diozero.remote.message.protobuf.I2C.ByteArray.class,
responseType = com.diozero.remote.message.protobuf.Response.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getWriteBytesMethod() {
io.grpc.MethodDescriptor getWriteBytesMethod;
if ((getWriteBytesMethod = I2CServiceGrpc.getWriteBytesMethod) == null) {
synchronized (I2CServiceGrpc.class) {
if ((getWriteBytesMethod = I2CServiceGrpc.getWriteBytesMethod) == null) {
I2CServiceGrpc.getWriteBytesMethod = getWriteBytesMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "WriteBytes"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.I2C.ByteArray.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Response.getDefaultInstance()))
.setSchemaDescriptor(new I2CServiceMethodDescriptorSupplier("WriteBytes"))
.build();
}
}
}
return getWriteBytesMethod;
}
private static volatile io.grpc.MethodDescriptor getReadWriteMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ReadWrite",
requestType = com.diozero.remote.message.protobuf.I2C.ReadWrite.class,
responseType = com.diozero.remote.message.protobuf.BytesResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getReadWriteMethod() {
io.grpc.MethodDescriptor getReadWriteMethod;
if ((getReadWriteMethod = I2CServiceGrpc.getReadWriteMethod) == null) {
synchronized (I2CServiceGrpc.class) {
if ((getReadWriteMethod = I2CServiceGrpc.getReadWriteMethod) == null) {
I2CServiceGrpc.getReadWriteMethod = getReadWriteMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ReadWrite"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.I2C.ReadWrite.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.BytesResponse.getDefaultInstance()))
.setSchemaDescriptor(new I2CServiceMethodDescriptorSupplier("ReadWrite"))
.build();
}
}
}
return getReadWriteMethod;
}
private static volatile io.grpc.MethodDescriptor getCloseMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Close",
requestType = com.diozero.remote.message.protobuf.I2C.Identifier.class,
responseType = com.diozero.remote.message.protobuf.Response.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getCloseMethod() {
io.grpc.MethodDescriptor getCloseMethod;
if ((getCloseMethod = I2CServiceGrpc.getCloseMethod) == null) {
synchronized (I2CServiceGrpc.class) {
if ((getCloseMethod = I2CServiceGrpc.getCloseMethod) == null) {
I2CServiceGrpc.getCloseMethod = getCloseMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "Close"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.I2C.Identifier.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Response.getDefaultInstance()))
.setSchemaDescriptor(new I2CServiceMethodDescriptorSupplier("Close"))
.build();
}
}
}
return getCloseMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static I2CServiceStub newStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public I2CServiceStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new I2CServiceStub(channel, callOptions);
}
};
return I2CServiceStub.newStub(factory, channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static I2CServiceBlockingStub newBlockingStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public I2CServiceBlockingStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new I2CServiceBlockingStub(channel, callOptions);
}
};
return I2CServiceBlockingStub.newStub(factory, channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static I2CServiceFutureStub newFutureStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public I2CServiceFutureStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new I2CServiceFutureStub(channel, callOptions);
}
};
return I2CServiceFutureStub.newStub(factory, channel);
}
/**
*/
public interface AsyncService {
/**
*/
default void open(com.diozero.remote.message.protobuf.I2C.Open request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getOpenMethod(), responseObserver);
}
/**
*/
default void probe(com.diozero.remote.message.protobuf.I2C.Probe request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getProbeMethod(), responseObserver);
}
/**
*/
default void writeQuick(com.diozero.remote.message.protobuf.I2C.Bit request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getWriteQuickMethod(), responseObserver);
}
/**
*/
default void readByte(com.diozero.remote.message.protobuf.I2C.Identifier request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getReadByteMethod(), responseObserver);
}
/**
*/
default void writeByte(com.diozero.remote.message.protobuf.I2C.ByteMessage request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getWriteByteMethod(), responseObserver);
}
/**
*/
default void readByteData(com.diozero.remote.message.protobuf.I2C.Register request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getReadByteDataMethod(), responseObserver);
}
/**
*/
default void writeByteData(com.diozero.remote.message.protobuf.I2C.RegisterAndByte request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getWriteByteDataMethod(), responseObserver);
}
/**
*/
default void readWordData(com.diozero.remote.message.protobuf.I2C.Register request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getReadWordDataMethod(), responseObserver);
}
/**
*/
default void writeWordData(com.diozero.remote.message.protobuf.I2C.RegisterAndWordData request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getWriteWordDataMethod(), responseObserver);
}
/**
*/
default void processCall(com.diozero.remote.message.protobuf.I2C.RegisterAndWordData request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getProcessCallMethod(), responseObserver);
}
/**
*/
default void readBlockData(com.diozero.remote.message.protobuf.I2C.Register request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getReadBlockDataMethod(), responseObserver);
}
/**
*/
default void writeBlockData(com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getWriteBlockDataMethod(), responseObserver);
}
/**
*/
default void blockProcessCall(com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getBlockProcessCallMethod(), responseObserver);
}
/**
*/
default void readI2CBlockData(com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getReadI2CBlockDataMethod(), responseObserver);
}
/**
*/
default void writeI2CBlockData(com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getWriteI2CBlockDataMethod(), responseObserver);
}
/**
*/
default void readBytes(com.diozero.remote.message.protobuf.I2C.NumBytes request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getReadBytesMethod(), responseObserver);
}
/**
*/
default void writeBytes(com.diozero.remote.message.protobuf.I2C.ByteArray request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getWriteBytesMethod(), responseObserver);
}
/**
*/
default void readWrite(com.diozero.remote.message.protobuf.I2C.ReadWrite request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getReadWriteMethod(), responseObserver);
}
/**
*/
default void close(com.diozero.remote.message.protobuf.I2C.Identifier request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getCloseMethod(), responseObserver);
}
}
/**
* Base class for the server implementation of the service I2CService.
*/
public static abstract class I2CServiceImplBase
implements io.grpc.BindableService, AsyncService {
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return I2CServiceGrpc.bindService(this);
}
}
/**
* A stub to allow clients to do asynchronous rpc calls to service I2CService.
*/
public static final class I2CServiceStub
extends io.grpc.stub.AbstractAsyncStub {
private I2CServiceStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected I2CServiceStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new I2CServiceStub(channel, callOptions);
}
/**
*/
public void open(com.diozero.remote.message.protobuf.I2C.Open request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getOpenMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void probe(com.diozero.remote.message.protobuf.I2C.Probe request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getProbeMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void writeQuick(com.diozero.remote.message.protobuf.I2C.Bit request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getWriteQuickMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void readByte(com.diozero.remote.message.protobuf.I2C.Identifier request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getReadByteMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void writeByte(com.diozero.remote.message.protobuf.I2C.ByteMessage request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getWriteByteMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void readByteData(com.diozero.remote.message.protobuf.I2C.Register request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getReadByteDataMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void writeByteData(com.diozero.remote.message.protobuf.I2C.RegisterAndByte request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getWriteByteDataMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void readWordData(com.diozero.remote.message.protobuf.I2C.Register request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getReadWordDataMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void writeWordData(com.diozero.remote.message.protobuf.I2C.RegisterAndWordData request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getWriteWordDataMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void processCall(com.diozero.remote.message.protobuf.I2C.RegisterAndWordData request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getProcessCallMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void readBlockData(com.diozero.remote.message.protobuf.I2C.Register request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getReadBlockDataMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void writeBlockData(com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getWriteBlockDataMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void blockProcessCall(com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getBlockProcessCallMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void readI2CBlockData(com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getReadI2CBlockDataMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void writeI2CBlockData(com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getWriteI2CBlockDataMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void readBytes(com.diozero.remote.message.protobuf.I2C.NumBytes request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getReadBytesMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void writeBytes(com.diozero.remote.message.protobuf.I2C.ByteArray request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getWriteBytesMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void readWrite(com.diozero.remote.message.protobuf.I2C.ReadWrite request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getReadWriteMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void close(com.diozero.remote.message.protobuf.I2C.Identifier request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getCloseMethod(), getCallOptions()), request, responseObserver);
}
}
/**
* A stub to allow clients to do synchronous rpc calls to service I2CService.
*/
public static final class I2CServiceBlockingStub
extends io.grpc.stub.AbstractBlockingStub {
private I2CServiceBlockingStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected I2CServiceBlockingStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new I2CServiceBlockingStub(channel, callOptions);
}
/**
*/
public com.diozero.remote.message.protobuf.Response open(com.diozero.remote.message.protobuf.I2C.Open request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getOpenMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.BooleanResponse probe(com.diozero.remote.message.protobuf.I2C.Probe request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getProbeMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.Response writeQuick(com.diozero.remote.message.protobuf.I2C.Bit request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getWriteQuickMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.ByteResponse readByte(com.diozero.remote.message.protobuf.I2C.Identifier request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getReadByteMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.Response writeByte(com.diozero.remote.message.protobuf.I2C.ByteMessage request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getWriteByteMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.ByteResponse readByteData(com.diozero.remote.message.protobuf.I2C.Register request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getReadByteDataMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.Response writeByteData(com.diozero.remote.message.protobuf.I2C.RegisterAndByte request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getWriteByteDataMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.WordResponse readWordData(com.diozero.remote.message.protobuf.I2C.Register request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getReadWordDataMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.Response writeWordData(com.diozero.remote.message.protobuf.I2C.RegisterAndWordData request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getWriteWordDataMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.WordResponse processCall(com.diozero.remote.message.protobuf.I2C.RegisterAndWordData request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getProcessCallMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse readBlockData(com.diozero.remote.message.protobuf.I2C.Register request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getReadBlockDataMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.Response writeBlockData(com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getWriteBlockDataMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.BytesResponse blockProcessCall(com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getBlockProcessCallMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.BytesResponse readI2CBlockData(com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getReadI2CBlockDataMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.Response writeI2CBlockData(com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getWriteI2CBlockDataMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.BytesResponse readBytes(com.diozero.remote.message.protobuf.I2C.NumBytes request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getReadBytesMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.Response writeBytes(com.diozero.remote.message.protobuf.I2C.ByteArray request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getWriteBytesMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.BytesResponse readWrite(com.diozero.remote.message.protobuf.I2C.ReadWrite request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getReadWriteMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.Response close(com.diozero.remote.message.protobuf.I2C.Identifier request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getCloseMethod(), getCallOptions(), request);
}
}
/**
* A stub to allow clients to do ListenableFuture-style rpc calls to service I2CService.
*/
public static final class I2CServiceFutureStub
extends io.grpc.stub.AbstractFutureStub {
private I2CServiceFutureStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected I2CServiceFutureStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new I2CServiceFutureStub(channel, callOptions);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture open(
com.diozero.remote.message.protobuf.I2C.Open request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getOpenMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture probe(
com.diozero.remote.message.protobuf.I2C.Probe request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getProbeMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture writeQuick(
com.diozero.remote.message.protobuf.I2C.Bit request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getWriteQuickMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture readByte(
com.diozero.remote.message.protobuf.I2C.Identifier request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getReadByteMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture writeByte(
com.diozero.remote.message.protobuf.I2C.ByteMessage request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getWriteByteMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture readByteData(
com.diozero.remote.message.protobuf.I2C.Register request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getReadByteDataMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture writeByteData(
com.diozero.remote.message.protobuf.I2C.RegisterAndByte request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getWriteByteDataMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture readWordData(
com.diozero.remote.message.protobuf.I2C.Register request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getReadWordDataMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture writeWordData(
com.diozero.remote.message.protobuf.I2C.RegisterAndWordData request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getWriteWordDataMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture processCall(
com.diozero.remote.message.protobuf.I2C.RegisterAndWordData request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getProcessCallMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture readBlockData(
com.diozero.remote.message.protobuf.I2C.Register request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getReadBlockDataMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture writeBlockData(
com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getWriteBlockDataMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture blockProcessCall(
com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getBlockProcessCallMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture readI2CBlockData(
com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getReadI2CBlockDataMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture writeI2CBlockData(
com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getWriteI2CBlockDataMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture readBytes(
com.diozero.remote.message.protobuf.I2C.NumBytes request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getReadBytesMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture writeBytes(
com.diozero.remote.message.protobuf.I2C.ByteArray request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getWriteBytesMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture readWrite(
com.diozero.remote.message.protobuf.I2C.ReadWrite request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getReadWriteMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture close(
com.diozero.remote.message.protobuf.I2C.Identifier request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getCloseMethod(), getCallOptions()), request);
}
}
private static final int METHODID_OPEN = 0;
private static final int METHODID_PROBE = 1;
private static final int METHODID_WRITE_QUICK = 2;
private static final int METHODID_READ_BYTE = 3;
private static final int METHODID_WRITE_BYTE = 4;
private static final int METHODID_READ_BYTE_DATA = 5;
private static final int METHODID_WRITE_BYTE_DATA = 6;
private static final int METHODID_READ_WORD_DATA = 7;
private static final int METHODID_WRITE_WORD_DATA = 8;
private static final int METHODID_PROCESS_CALL = 9;
private static final int METHODID_READ_BLOCK_DATA = 10;
private static final int METHODID_WRITE_BLOCK_DATA = 11;
private static final int METHODID_BLOCK_PROCESS_CALL = 12;
private static final int METHODID_READ_I2CBLOCK_DATA = 13;
private static final int METHODID_WRITE_I2CBLOCK_DATA = 14;
private static final int METHODID_READ_BYTES = 15;
private static final int METHODID_WRITE_BYTES = 16;
private static final int METHODID_READ_WRITE = 17;
private static final int METHODID_CLOSE = 18;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final AsyncService serviceImpl;
private final int methodId;
MethodHandlers(AsyncService serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_OPEN:
serviceImpl.open((com.diozero.remote.message.protobuf.I2C.Open) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_PROBE:
serviceImpl.probe((com.diozero.remote.message.protobuf.I2C.Probe) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_WRITE_QUICK:
serviceImpl.writeQuick((com.diozero.remote.message.protobuf.I2C.Bit) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_READ_BYTE:
serviceImpl.readByte((com.diozero.remote.message.protobuf.I2C.Identifier) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_WRITE_BYTE:
serviceImpl.writeByte((com.diozero.remote.message.protobuf.I2C.ByteMessage) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_READ_BYTE_DATA:
serviceImpl.readByteData((com.diozero.remote.message.protobuf.I2C.Register) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_WRITE_BYTE_DATA:
serviceImpl.writeByteData((com.diozero.remote.message.protobuf.I2C.RegisterAndByte) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_READ_WORD_DATA:
serviceImpl.readWordData((com.diozero.remote.message.protobuf.I2C.Register) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_WRITE_WORD_DATA:
serviceImpl.writeWordData((com.diozero.remote.message.protobuf.I2C.RegisterAndWordData) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_PROCESS_CALL:
serviceImpl.processCall((com.diozero.remote.message.protobuf.I2C.RegisterAndWordData) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_READ_BLOCK_DATA:
serviceImpl.readBlockData((com.diozero.remote.message.protobuf.I2C.Register) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_WRITE_BLOCK_DATA:
serviceImpl.writeBlockData((com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_BLOCK_PROCESS_CALL:
serviceImpl.blockProcessCall((com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_READ_I2CBLOCK_DATA:
serviceImpl.readI2CBlockData((com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_WRITE_I2CBLOCK_DATA:
serviceImpl.writeI2CBlockData((com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_READ_BYTES:
serviceImpl.readBytes((com.diozero.remote.message.protobuf.I2C.NumBytes) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_WRITE_BYTES:
serviceImpl.writeBytes((com.diozero.remote.message.protobuf.I2C.ByteArray) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_READ_WRITE:
serviceImpl.readWrite((com.diozero.remote.message.protobuf.I2C.ReadWrite) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_CLOSE:
serviceImpl.close((com.diozero.remote.message.protobuf.I2C.Identifier) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new AssertionError();
}
}
}
public static final io.grpc.ServerServiceDefinition bindService(AsyncService service) {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
getOpenMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.I2C.Open,
com.diozero.remote.message.protobuf.Response>(
service, METHODID_OPEN)))
.addMethod(
getProbeMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.I2C.Probe,
com.diozero.remote.message.protobuf.BooleanResponse>(
service, METHODID_PROBE)))
.addMethod(
getWriteQuickMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.I2C.Bit,
com.diozero.remote.message.protobuf.Response>(
service, METHODID_WRITE_QUICK)))
.addMethod(
getReadByteMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.I2C.Identifier,
com.diozero.remote.message.protobuf.ByteResponse>(
service, METHODID_READ_BYTE)))
.addMethod(
getWriteByteMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.I2C.ByteMessage,
com.diozero.remote.message.protobuf.Response>(
service, METHODID_WRITE_BYTE)))
.addMethod(
getReadByteDataMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.I2C.Register,
com.diozero.remote.message.protobuf.ByteResponse>(
service, METHODID_READ_BYTE_DATA)))
.addMethod(
getWriteByteDataMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.I2C.RegisterAndByte,
com.diozero.remote.message.protobuf.Response>(
service, METHODID_WRITE_BYTE_DATA)))
.addMethod(
getReadWordDataMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.I2C.Register,
com.diozero.remote.message.protobuf.WordResponse>(
service, METHODID_READ_WORD_DATA)))
.addMethod(
getWriteWordDataMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.I2C.RegisterAndWordData,
com.diozero.remote.message.protobuf.Response>(
service, METHODID_WRITE_WORD_DATA)))
.addMethod(
getProcessCallMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.I2C.RegisterAndWordData,
com.diozero.remote.message.protobuf.WordResponse>(
service, METHODID_PROCESS_CALL)))
.addMethod(
getReadBlockDataMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.I2C.Register,
com.diozero.remote.message.protobuf.I2C.ByteArrayWithLengthResponse>(
service, METHODID_READ_BLOCK_DATA)))
.addMethod(
getWriteBlockDataMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray,
com.diozero.remote.message.protobuf.Response>(
service, METHODID_WRITE_BLOCK_DATA)))
.addMethod(
getBlockProcessCallMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray,
com.diozero.remote.message.protobuf.BytesResponse>(
service, METHODID_BLOCK_PROCESS_CALL)))
.addMethod(
getReadI2CBlockDataMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.I2C.RegisterAndNumBytes,
com.diozero.remote.message.protobuf.BytesResponse>(
service, METHODID_READ_I2CBLOCK_DATA)))
.addMethod(
getWriteI2CBlockDataMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.I2C.RegisterAndByteArray,
com.diozero.remote.message.protobuf.Response>(
service, METHODID_WRITE_I2CBLOCK_DATA)))
.addMethod(
getReadBytesMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.I2C.NumBytes,
com.diozero.remote.message.protobuf.BytesResponse>(
service, METHODID_READ_BYTES)))
.addMethod(
getWriteBytesMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.I2C.ByteArray,
com.diozero.remote.message.protobuf.Response>(
service, METHODID_WRITE_BYTES)))
.addMethod(
getReadWriteMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.I2C.ReadWrite,
com.diozero.remote.message.protobuf.BytesResponse>(
service, METHODID_READ_WRITE)))
.addMethod(
getCloseMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.I2C.Identifier,
com.diozero.remote.message.protobuf.Response>(
service, METHODID_CLOSE)))
.build();
}
private static abstract class I2CServiceBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoFileDescriptorSupplier, io.grpc.protobuf.ProtoServiceDescriptorSupplier {
I2CServiceBaseDescriptorSupplier() {}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("I2CService");
}
}
private static final class I2CServiceFileDescriptorSupplier
extends I2CServiceBaseDescriptorSupplier {
I2CServiceFileDescriptorSupplier() {}
}
private static final class I2CServiceMethodDescriptorSupplier
extends I2CServiceBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final java.lang.String methodName;
I2CServiceMethodDescriptorSupplier(java.lang.String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (I2CServiceGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new I2CServiceFileDescriptorSupplier())
.addMethod(getOpenMethod())
.addMethod(getProbeMethod())
.addMethod(getWriteQuickMethod())
.addMethod(getReadByteMethod())
.addMethod(getWriteByteMethod())
.addMethod(getReadByteDataMethod())
.addMethod(getWriteByteDataMethod())
.addMethod(getReadWordDataMethod())
.addMethod(getWriteWordDataMethod())
.addMethod(getProcessCallMethod())
.addMethod(getReadBlockDataMethod())
.addMethod(getWriteBlockDataMethod())
.addMethod(getBlockProcessCallMethod())
.addMethod(getReadI2CBlockDataMethod())
.addMethod(getWriteI2CBlockDataMethod())
.addMethod(getReadBytesMethod())
.addMethod(getWriteBytesMethod())
.addMethod(getReadWriteMethod())
.addMethod(getCloseMethod())
.build();
}
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy