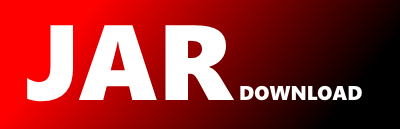
com.diozero.remote.message.protobuf.SerialServiceGrpc Maven / Gradle / Ivy
The newest version!
package com.diozero.remote.message.protobuf;
import static io.grpc.MethodDescriptor.generateFullMethodName;
/**
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.68.0)",
comments = "Source: diozero.proto")
@io.grpc.stub.annotations.GrpcGenerated
public final class SerialServiceGrpc {
private SerialServiceGrpc() {}
public static final java.lang.String SERVICE_NAME = "diozero.SerialService";
// Static method descriptors that strictly reflect the proto.
private static volatile io.grpc.MethodDescriptor getOpenMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Open",
requestType = com.diozero.remote.message.protobuf.Serial.Open.class,
responseType = com.diozero.remote.message.protobuf.Response.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getOpenMethod() {
io.grpc.MethodDescriptor getOpenMethod;
if ((getOpenMethod = SerialServiceGrpc.getOpenMethod) == null) {
synchronized (SerialServiceGrpc.class) {
if ((getOpenMethod = SerialServiceGrpc.getOpenMethod) == null) {
SerialServiceGrpc.getOpenMethod = getOpenMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "Open"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Serial.Open.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Response.getDefaultInstance()))
.setSchemaDescriptor(new SerialServiceMethodDescriptorSupplier("Open"))
.build();
}
}
}
return getOpenMethod;
}
private static volatile io.grpc.MethodDescriptor getReadMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Read",
requestType = com.diozero.remote.message.protobuf.Serial.Identifier.class,
responseType = com.diozero.remote.message.protobuf.IntegerResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getReadMethod() {
io.grpc.MethodDescriptor getReadMethod;
if ((getReadMethod = SerialServiceGrpc.getReadMethod) == null) {
synchronized (SerialServiceGrpc.class) {
if ((getReadMethod = SerialServiceGrpc.getReadMethod) == null) {
SerialServiceGrpc.getReadMethod = getReadMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "Read"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Serial.Identifier.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.IntegerResponse.getDefaultInstance()))
.setSchemaDescriptor(new SerialServiceMethodDescriptorSupplier("Read"))
.build();
}
}
}
return getReadMethod;
}
private static volatile io.grpc.MethodDescriptor getReadByteMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ReadByte",
requestType = com.diozero.remote.message.protobuf.Serial.Identifier.class,
responseType = com.diozero.remote.message.protobuf.ByteResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getReadByteMethod() {
io.grpc.MethodDescriptor getReadByteMethod;
if ((getReadByteMethod = SerialServiceGrpc.getReadByteMethod) == null) {
synchronized (SerialServiceGrpc.class) {
if ((getReadByteMethod = SerialServiceGrpc.getReadByteMethod) == null) {
SerialServiceGrpc.getReadByteMethod = getReadByteMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ReadByte"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Serial.Identifier.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.ByteResponse.getDefaultInstance()))
.setSchemaDescriptor(new SerialServiceMethodDescriptorSupplier("ReadByte"))
.build();
}
}
}
return getReadByteMethod;
}
private static volatile io.grpc.MethodDescriptor getWriteByteMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "WriteByte",
requestType = com.diozero.remote.message.protobuf.Serial.ByteMessage.class,
responseType = com.diozero.remote.message.protobuf.Response.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getWriteByteMethod() {
io.grpc.MethodDescriptor getWriteByteMethod;
if ((getWriteByteMethod = SerialServiceGrpc.getWriteByteMethod) == null) {
synchronized (SerialServiceGrpc.class) {
if ((getWriteByteMethod = SerialServiceGrpc.getWriteByteMethod) == null) {
SerialServiceGrpc.getWriteByteMethod = getWriteByteMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "WriteByte"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Serial.ByteMessage.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Response.getDefaultInstance()))
.setSchemaDescriptor(new SerialServiceMethodDescriptorSupplier("WriteByte"))
.build();
}
}
}
return getWriteByteMethod;
}
private static volatile io.grpc.MethodDescriptor getReadBytesMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ReadBytes",
requestType = com.diozero.remote.message.protobuf.Serial.NumBytes.class,
responseType = com.diozero.remote.message.protobuf.BytesResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getReadBytesMethod() {
io.grpc.MethodDescriptor getReadBytesMethod;
if ((getReadBytesMethod = SerialServiceGrpc.getReadBytesMethod) == null) {
synchronized (SerialServiceGrpc.class) {
if ((getReadBytesMethod = SerialServiceGrpc.getReadBytesMethod) == null) {
SerialServiceGrpc.getReadBytesMethod = getReadBytesMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ReadBytes"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Serial.NumBytes.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.BytesResponse.getDefaultInstance()))
.setSchemaDescriptor(new SerialServiceMethodDescriptorSupplier("ReadBytes"))
.build();
}
}
}
return getReadBytesMethod;
}
private static volatile io.grpc.MethodDescriptor getWriteBytesMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "WriteBytes",
requestType = com.diozero.remote.message.protobuf.Serial.ByteArray.class,
responseType = com.diozero.remote.message.protobuf.Response.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getWriteBytesMethod() {
io.grpc.MethodDescriptor getWriteBytesMethod;
if ((getWriteBytesMethod = SerialServiceGrpc.getWriteBytesMethod) == null) {
synchronized (SerialServiceGrpc.class) {
if ((getWriteBytesMethod = SerialServiceGrpc.getWriteBytesMethod) == null) {
SerialServiceGrpc.getWriteBytesMethod = getWriteBytesMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "WriteBytes"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Serial.ByteArray.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Response.getDefaultInstance()))
.setSchemaDescriptor(new SerialServiceMethodDescriptorSupplier("WriteBytes"))
.build();
}
}
}
return getWriteBytesMethod;
}
private static volatile io.grpc.MethodDescriptor getBytesAvailableMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "BytesAvailable",
requestType = com.diozero.remote.message.protobuf.Serial.Identifier.class,
responseType = com.diozero.remote.message.protobuf.IntegerResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getBytesAvailableMethod() {
io.grpc.MethodDescriptor getBytesAvailableMethod;
if ((getBytesAvailableMethod = SerialServiceGrpc.getBytesAvailableMethod) == null) {
synchronized (SerialServiceGrpc.class) {
if ((getBytesAvailableMethod = SerialServiceGrpc.getBytesAvailableMethod) == null) {
SerialServiceGrpc.getBytesAvailableMethod = getBytesAvailableMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "BytesAvailable"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Serial.Identifier.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.IntegerResponse.getDefaultInstance()))
.setSchemaDescriptor(new SerialServiceMethodDescriptorSupplier("BytesAvailable"))
.build();
}
}
}
return getBytesAvailableMethod;
}
private static volatile io.grpc.MethodDescriptor getCloseMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Close",
requestType = com.diozero.remote.message.protobuf.Serial.Identifier.class,
responseType = com.diozero.remote.message.protobuf.Response.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getCloseMethod() {
io.grpc.MethodDescriptor getCloseMethod;
if ((getCloseMethod = SerialServiceGrpc.getCloseMethod) == null) {
synchronized (SerialServiceGrpc.class) {
if ((getCloseMethod = SerialServiceGrpc.getCloseMethod) == null) {
SerialServiceGrpc.getCloseMethod = getCloseMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "Close"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Serial.Identifier.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.diozero.remote.message.protobuf.Response.getDefaultInstance()))
.setSchemaDescriptor(new SerialServiceMethodDescriptorSupplier("Close"))
.build();
}
}
}
return getCloseMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static SerialServiceStub newStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public SerialServiceStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new SerialServiceStub(channel, callOptions);
}
};
return SerialServiceStub.newStub(factory, channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static SerialServiceBlockingStub newBlockingStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public SerialServiceBlockingStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new SerialServiceBlockingStub(channel, callOptions);
}
};
return SerialServiceBlockingStub.newStub(factory, channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static SerialServiceFutureStub newFutureStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public SerialServiceFutureStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new SerialServiceFutureStub(channel, callOptions);
}
};
return SerialServiceFutureStub.newStub(factory, channel);
}
/**
*/
public interface AsyncService {
/**
*/
default void open(com.diozero.remote.message.protobuf.Serial.Open request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getOpenMethod(), responseObserver);
}
/**
*/
default void read(com.diozero.remote.message.protobuf.Serial.Identifier request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getReadMethod(), responseObserver);
}
/**
*/
default void readByte(com.diozero.remote.message.protobuf.Serial.Identifier request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getReadByteMethod(), responseObserver);
}
/**
*/
default void writeByte(com.diozero.remote.message.protobuf.Serial.ByteMessage request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getWriteByteMethod(), responseObserver);
}
/**
*/
default void readBytes(com.diozero.remote.message.protobuf.Serial.NumBytes request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getReadBytesMethod(), responseObserver);
}
/**
*/
default void writeBytes(com.diozero.remote.message.protobuf.Serial.ByteArray request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getWriteBytesMethod(), responseObserver);
}
/**
*/
default void bytesAvailable(com.diozero.remote.message.protobuf.Serial.Identifier request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getBytesAvailableMethod(), responseObserver);
}
/**
*/
default void close(com.diozero.remote.message.protobuf.Serial.Identifier request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getCloseMethod(), responseObserver);
}
}
/**
* Base class for the server implementation of the service SerialService.
*/
public static abstract class SerialServiceImplBase
implements io.grpc.BindableService, AsyncService {
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return SerialServiceGrpc.bindService(this);
}
}
/**
* A stub to allow clients to do asynchronous rpc calls to service SerialService.
*/
public static final class SerialServiceStub
extends io.grpc.stub.AbstractAsyncStub {
private SerialServiceStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected SerialServiceStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new SerialServiceStub(channel, callOptions);
}
/**
*/
public void open(com.diozero.remote.message.protobuf.Serial.Open request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getOpenMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void read(com.diozero.remote.message.protobuf.Serial.Identifier request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getReadMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void readByte(com.diozero.remote.message.protobuf.Serial.Identifier request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getReadByteMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void writeByte(com.diozero.remote.message.protobuf.Serial.ByteMessage request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getWriteByteMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void readBytes(com.diozero.remote.message.protobuf.Serial.NumBytes request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getReadBytesMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void writeBytes(com.diozero.remote.message.protobuf.Serial.ByteArray request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getWriteBytesMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void bytesAvailable(com.diozero.remote.message.protobuf.Serial.Identifier request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getBytesAvailableMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void close(com.diozero.remote.message.protobuf.Serial.Identifier request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getCloseMethod(), getCallOptions()), request, responseObserver);
}
}
/**
* A stub to allow clients to do synchronous rpc calls to service SerialService.
*/
public static final class SerialServiceBlockingStub
extends io.grpc.stub.AbstractBlockingStub {
private SerialServiceBlockingStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected SerialServiceBlockingStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new SerialServiceBlockingStub(channel, callOptions);
}
/**
*/
public com.diozero.remote.message.protobuf.Response open(com.diozero.remote.message.protobuf.Serial.Open request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getOpenMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.IntegerResponse read(com.diozero.remote.message.protobuf.Serial.Identifier request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getReadMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.ByteResponse readByte(com.diozero.remote.message.protobuf.Serial.Identifier request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getReadByteMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.Response writeByte(com.diozero.remote.message.protobuf.Serial.ByteMessage request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getWriteByteMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.BytesResponse readBytes(com.diozero.remote.message.protobuf.Serial.NumBytes request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getReadBytesMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.Response writeBytes(com.diozero.remote.message.protobuf.Serial.ByteArray request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getWriteBytesMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.IntegerResponse bytesAvailable(com.diozero.remote.message.protobuf.Serial.Identifier request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getBytesAvailableMethod(), getCallOptions(), request);
}
/**
*/
public com.diozero.remote.message.protobuf.Response close(com.diozero.remote.message.protobuf.Serial.Identifier request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getCloseMethod(), getCallOptions(), request);
}
}
/**
* A stub to allow clients to do ListenableFuture-style rpc calls to service SerialService.
*/
public static final class SerialServiceFutureStub
extends io.grpc.stub.AbstractFutureStub {
private SerialServiceFutureStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected SerialServiceFutureStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new SerialServiceFutureStub(channel, callOptions);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture open(
com.diozero.remote.message.protobuf.Serial.Open request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getOpenMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture read(
com.diozero.remote.message.protobuf.Serial.Identifier request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getReadMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture readByte(
com.diozero.remote.message.protobuf.Serial.Identifier request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getReadByteMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture writeByte(
com.diozero.remote.message.protobuf.Serial.ByteMessage request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getWriteByteMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture readBytes(
com.diozero.remote.message.protobuf.Serial.NumBytes request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getReadBytesMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture writeBytes(
com.diozero.remote.message.protobuf.Serial.ByteArray request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getWriteBytesMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture bytesAvailable(
com.diozero.remote.message.protobuf.Serial.Identifier request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getBytesAvailableMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture close(
com.diozero.remote.message.protobuf.Serial.Identifier request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getCloseMethod(), getCallOptions()), request);
}
}
private static final int METHODID_OPEN = 0;
private static final int METHODID_READ = 1;
private static final int METHODID_READ_BYTE = 2;
private static final int METHODID_WRITE_BYTE = 3;
private static final int METHODID_READ_BYTES = 4;
private static final int METHODID_WRITE_BYTES = 5;
private static final int METHODID_BYTES_AVAILABLE = 6;
private static final int METHODID_CLOSE = 7;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final AsyncService serviceImpl;
private final int methodId;
MethodHandlers(AsyncService serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_OPEN:
serviceImpl.open((com.diozero.remote.message.protobuf.Serial.Open) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_READ:
serviceImpl.read((com.diozero.remote.message.protobuf.Serial.Identifier) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_READ_BYTE:
serviceImpl.readByte((com.diozero.remote.message.protobuf.Serial.Identifier) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_WRITE_BYTE:
serviceImpl.writeByte((com.diozero.remote.message.protobuf.Serial.ByteMessage) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_READ_BYTES:
serviceImpl.readBytes((com.diozero.remote.message.protobuf.Serial.NumBytes) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_WRITE_BYTES:
serviceImpl.writeBytes((com.diozero.remote.message.protobuf.Serial.ByteArray) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_BYTES_AVAILABLE:
serviceImpl.bytesAvailable((com.diozero.remote.message.protobuf.Serial.Identifier) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_CLOSE:
serviceImpl.close((com.diozero.remote.message.protobuf.Serial.Identifier) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new AssertionError();
}
}
}
public static final io.grpc.ServerServiceDefinition bindService(AsyncService service) {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
getOpenMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.Serial.Open,
com.diozero.remote.message.protobuf.Response>(
service, METHODID_OPEN)))
.addMethod(
getReadMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.Serial.Identifier,
com.diozero.remote.message.protobuf.IntegerResponse>(
service, METHODID_READ)))
.addMethod(
getReadByteMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.Serial.Identifier,
com.diozero.remote.message.protobuf.ByteResponse>(
service, METHODID_READ_BYTE)))
.addMethod(
getWriteByteMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.Serial.ByteMessage,
com.diozero.remote.message.protobuf.Response>(
service, METHODID_WRITE_BYTE)))
.addMethod(
getReadBytesMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.Serial.NumBytes,
com.diozero.remote.message.protobuf.BytesResponse>(
service, METHODID_READ_BYTES)))
.addMethod(
getWriteBytesMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.Serial.ByteArray,
com.diozero.remote.message.protobuf.Response>(
service, METHODID_WRITE_BYTES)))
.addMethod(
getBytesAvailableMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.Serial.Identifier,
com.diozero.remote.message.protobuf.IntegerResponse>(
service, METHODID_BYTES_AVAILABLE)))
.addMethod(
getCloseMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.diozero.remote.message.protobuf.Serial.Identifier,
com.diozero.remote.message.protobuf.Response>(
service, METHODID_CLOSE)))
.build();
}
private static abstract class SerialServiceBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoFileDescriptorSupplier, io.grpc.protobuf.ProtoServiceDescriptorSupplier {
SerialServiceBaseDescriptorSupplier() {}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return com.diozero.remote.message.protobuf.Diozero.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("SerialService");
}
}
private static final class SerialServiceFileDescriptorSupplier
extends SerialServiceBaseDescriptorSupplier {
SerialServiceFileDescriptorSupplier() {}
}
private static final class SerialServiceMethodDescriptorSupplier
extends SerialServiceBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final java.lang.String methodName;
SerialServiceMethodDescriptorSupplier(java.lang.String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (SerialServiceGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new SerialServiceFileDescriptorSupplier())
.addMethod(getOpenMethod())
.addMethod(getReadMethod())
.addMethod(getReadByteMethod())
.addMethod(getWriteByteMethod())
.addMethod(getReadBytesMethod())
.addMethod(getWriteBytesMethod())
.addMethod(getBytesAvailableMethod())
.addMethod(getCloseMethod())
.build();
}
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy