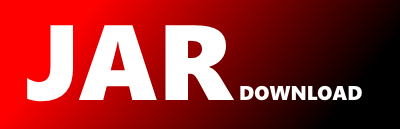
com.directmediatips.google.sheets.twitter.TwitterRichData Maven / Gradle / Ivy
package com.directmediatips.google.sheets.twitter;
/*
* Copyright 2017, Bruno Lowagie, Wil-Low BVBA
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may
* not use this file except in compliance with the License. You may obtain
* a copy of the License at http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the * specific language governing permissions and
* limitations under the License.
*/
import java.io.FileInputStream;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import java.util.TreeMap;
import com.directmediatips.google.sheets.SheetService;
import com.google.api.services.sheets.v4.Sheets;
import com.google.api.services.sheets.v4.model.ValueRange;
/**
* Uses a Google spreadsheet to list who is following us based on search criteria.
*/
public class TwitterRichData {
/**
* Inner class to store account info.
*/
public class Account {
/** The screen name. */
public String screenname;
/** The accounts of interest. */
public List accounts = new ArrayList();
}
/** An ordered map with account that follow at least one of our accounts. */
protected Map accounts = new TreeMap();
/** The range where we can find the WHERE clause in the Google spreadsheet. */
public static final String RANGE1 = "criteria!A1";
/** The range where we can find our twitter accounts in the Google spreadsheet. */
public static final String RANGE2 = "results!C1:Z";
/** The range where to put the results in the Google spreadsheet. */
public static final String RANGE3 = "results!A2:Z";
/** The range where we can find the direct message in the Google spreadsheet. */
public static final String RANGE4 = "mail!A1";
/** The Google Sheets service. */
protected Sheets service;
/** The ID of the spreadsheet with the Twitter information. */
protected String spreadsheetId;
/**
* Creates a TwitterData instance.
*
* @throws IOException Signals that an I/O exception has occurred.
*/
public TwitterRichData() throws IOException {
this.service = SheetService.getSheets();
Properties props = new Properties();
props.load(new FileInputStream("google/sheet.properties"));
this.spreadsheetId = props.getProperty("twitterRichData");
}
/**
* Gets the part of an SQL statement that defines the criteria.
*
* @return the part of the query that comes after WHERE
* @throws IOException Signals that an I/O exception has occurred.
*/
public String getWhereClause() throws IOException {
ValueRange response = service
.spreadsheets()
.values()
.get(spreadsheetId, RANGE1)
.execute();
List> values = response.getValues();
return values.get(0).get(0).toString();
}
/**
* Gets a list of Twitter accounts from the spreadsheet.
*
* @return a List of Twitter accounts
* @throws IOException Signals that an I/O exception has occurred.
*/
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy