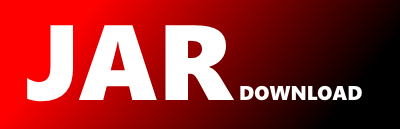
com.disdar.api.model.DocumentAnalysisResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of disdar-api-client-java Show documentation
Show all versions of disdar-api-client-java Show documentation
A simple Java client for the DISDAR extraction service
package com.disdar.api.model;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.google.common.base.Optional;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
/**
* A class storing the results of the extraction process.
*/
@JsonIgnoreProperties(ignoreUnknown = true)
public class DocumentAnalysisResult {
private String requestId;
private Optional errorCode;
private Optional errorMessage;
private Optional ocrResult;
private Optional extractionResult;
@JsonCreator
public DocumentAnalysisResult(@JsonProperty("requestId") String requestId,
@JsonProperty("errorCode") Optional errorCode,
@JsonProperty("errorMessage") Optional errorMessage,
@JsonProperty("ocrResult") Optional ocrResult,
@JsonProperty("extractionResult") Optional extractionResult) {
this.requestId = requestId;
this.errorCode = errorCode;
this.errorMessage = errorMessage;
this.ocrResult = ocrResult;
this.extractionResult = extractionResult;
}
public String getRequestId() {
return requestId;
}
public Optional getErrorCode() {
return errorCode;
}
public Optional getErrorMessage() {
return errorMessage;
}
public Optional getOcrResult() {
return ocrResult;
}
public Optional getExtractionResult() {
return extractionResult;
}
@Override
public boolean equals(Object obj) {
return EqualsBuilder.reflectionEquals(this, obj);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
@Override
public String toString() {
String success = (errorCode.isPresent()) ? errorCode.get().toString() : "success";
return String.format("DocumentAnalysisResult: %s\t%s", requestId, success);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy