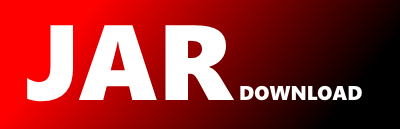
META-INF.smithy.snowdevicemanagement.smithy Maven / Gradle / Ivy
Show all versions of aws-snow-device-management-spec Show documentation
$version: "2.0"
namespace com.amazonaws.snowdevicemanagement
use aws.api#arn
use aws.api#service
use aws.auth#sigv4
use aws.protocols#restJson1
/// Amazon Web Services Snow Device Management documentation.
@service(
sdkId: "Snow Device Management"
arnNamespace: "snow-device-management"
cloudFormationName: "SnowDeviceManagement"
cloudTrailEventSource: "snow-device-management.amazonaws.com"
endpointPrefix: "snow-device-management"
)
@sigv4(
name: "snow-device-management"
)
@restJson1
@cors
@title("AWS Snow Device Management")
service SnowDeviceManagement {
version: "2021-08-04"
operations: [
ListTagsForResource
TagResource
UntagResource
]
resources: [
ManagedDevice
Task
]
}
resource Execution {
identifiers: {
managedDeviceId: ManagedDeviceId
taskId: TaskId
}
read: DescribeExecution
list: ListExecutions
}
@arn(
template: "managed-device/{managedDeviceId}"
absolute: false
noAccount: false
noRegion: false
)
resource ManagedDevice {
identifiers: {
managedDeviceId: ManagedDeviceId
}
read: DescribeDevice
list: ListDevices
operations: [
DescribeDeviceEc2Instances
ListDeviceResources
]
}
@arn(
template: "task/{taskId}"
absolute: false
noAccount: false
noRegion: false
)
resource Task {
identifiers: {
taskId: TaskId
}
create: CreateTask
read: DescribeTask
list: ListTasks
operations: [
CancelTask
]
resources: [
Execution
]
}
/// Sends a cancel request for a specified task. You can cancel a task only if it's still in a
/// QUEUED
state. Tasks that are already running can't be cancelled.
///
/// A task might still run if it's processed from the queue before the
/// CancelTask
operation changes the task's state.
///
@http(
method: "POST"
uri: "/task/{taskId}/cancel"
code: 200
)
operation CancelTask {
input: CancelTaskInput
output: CancelTaskOutput
errors: [
AccessDeniedException
InternalServerException
ResourceNotFoundException
ThrottlingException
ValidationException
]
}
/// Instructs one or more devices to start a task, such as unlocking or rebooting.
@http(
method: "POST"
uri: "/task"
code: 200
)
operation CreateTask {
input: CreateTaskInput
output: CreateTaskOutput
errors: [
AccessDeniedException
InternalServerException
ResourceNotFoundException
ServiceQuotaExceededException
ThrottlingException
ValidationException
]
}
/// Checks device-specific information, such as the device type, software version, IP
/// addresses, and lock status.
@http(
method: "POST"
uri: "/managed-device/{managedDeviceId}/describe"
code: 200
)
@readonly
operation DescribeDevice {
input: DescribeDeviceInput
output: DescribeDeviceOutput
errors: [
AccessDeniedException
InternalServerException
ResourceNotFoundException
ThrottlingException
ValidationException
]
}
/// Checks the current state of the Amazon EC2 instances. The output is similar to
/// describeDevice
, but the results are sourced from the device cache in the
/// Amazon Web Services Cloud and include a subset of the available fields.
@http(
method: "POST"
uri: "/managed-device/{managedDeviceId}/resources/ec2/describe"
code: 200
)
@readonly
operation DescribeDeviceEc2Instances {
input: DescribeDeviceEc2Input
output: DescribeDeviceEc2Output
errors: [
AccessDeniedException
InternalServerException
ResourceNotFoundException
ThrottlingException
ValidationException
]
}
/// Checks the status of a remote task running on one or more target devices.
@http(
method: "POST"
uri: "/task/{taskId}/execution/{managedDeviceId}"
code: 200
)
@readonly
operation DescribeExecution {
input: DescribeExecutionInput
output: DescribeExecutionOutput
errors: [
AccessDeniedException
InternalServerException
ResourceNotFoundException
ThrottlingException
ValidationException
]
}
/// Checks the metadata for a given task on a device.
@http(
method: "POST"
uri: "/task/{taskId}"
code: 200
)
@readonly
operation DescribeTask {
input: DescribeTaskInput
output: DescribeTaskOutput
errors: [
AccessDeniedException
InternalServerException
ResourceNotFoundException
ThrottlingException
ValidationException
]
}
/// Returns a list of the Amazon Web Services resources available for a device. Currently, Amazon EC2 instances are the only supported resource type.
@http(
method: "GET"
uri: "/managed-device/{managedDeviceId}/resources"
code: 200
)
@paginated(
inputToken: "nextToken"
outputToken: "nextToken"
items: "resources"
pageSize: "maxResults"
)
@readonly
operation ListDeviceResources {
input: ListDeviceResourcesInput
output: ListDeviceResourcesOutput
errors: [
AccessDeniedException
InternalServerException
ResourceNotFoundException
ThrottlingException
ValidationException
]
}
/// Returns a list of all devices on your Amazon Web Services account that have Amazon Web Services Snow Device Management
/// enabled in the Amazon Web Services Region where the command is run.
@http(
method: "GET"
uri: "/managed-devices"
code: 200
)
@paginated(
inputToken: "nextToken"
outputToken: "nextToken"
items: "devices"
pageSize: "maxResults"
)
@readonly
operation ListDevices {
input: ListDevicesInput
output: ListDevicesOutput
errors: [
AccessDeniedException
InternalServerException
ThrottlingException
ValidationException
]
}
/// Returns the status of tasks for one or more target devices.
@http(
method: "GET"
uri: "/executions"
code: 200
)
@paginated(
inputToken: "nextToken"
outputToken: "nextToken"
items: "executions"
pageSize: "maxResults"
)
@readonly
operation ListExecutions {
input: ListExecutionsInput
output: ListExecutionsOutput
errors: [
AccessDeniedException
InternalServerException
ResourceNotFoundException
ThrottlingException
ValidationException
]
}
/// Returns a list of tags for a managed device or task.
@http(
method: "GET"
uri: "/tags/{resourceArn}"
code: 200
)
@readonly
operation ListTagsForResource {
input: ListTagsForResourceInput
output: ListTagsForResourceOutput
errors: [
InternalServerException
ResourceNotFoundException
ValidationException
]
}
/// Returns a list of tasks that can be filtered by state.
@http(
method: "GET"
uri: "/tasks"
code: 200
)
@paginated(
inputToken: "nextToken"
outputToken: "nextToken"
items: "tasks"
pageSize: "maxResults"
)
@readonly
operation ListTasks {
input: ListTasksInput
output: ListTasksOutput
errors: [
AccessDeniedException
InternalServerException
ThrottlingException
ValidationException
]
}
/// Adds or replaces tags on a device or task.
@http(
method: "POST"
uri: "/tags/{resourceArn}"
code: 200
)
operation TagResource {
input: TagResourceInput
output: Unit
errors: [
InternalServerException
ResourceNotFoundException
ValidationException
]
}
/// Removes a tag from a device or task.
@http(
method: "DELETE"
uri: "/tags/{resourceArn}"
code: 200
)
@idempotent
operation UntagResource {
input: UntagResourceInput
output: Unit
errors: [
InternalServerException
ResourceNotFoundException
ValidationException
]
}
/// You don't have sufficient access to perform this action.
@error("client")
@httpError(403)
structure AccessDeniedException {
@required
message: String
}
structure CancelTaskInput {
/// The ID of the task that you are attempting to cancel. You can retrieve a task ID by using
/// the ListTasks
operation.
@httpLabel
@required
taskId: TaskId
}
structure CancelTaskOutput {
/// The ID of the task that you are attempting to cancel.
taskId: String
}
/// The physical capacity of the Amazon Web Services Snow Family device.
structure Capacity {
/// The name of the type of capacity, such as memory.
@length(
min: 0
max: 100
)
name: String
/// The unit of measure for the type of capacity.
@length(
min: 0
max: 20
)
unit: String
/// The total capacity on the device.
total: Long
/// The amount of capacity used on the device.
used: Long
/// The amount of capacity available for use on the device.
available: Long
}
/// The options for how a device's CPU is configured.
structure CpuOptions {
/// The number of cores that the CPU can use.
coreCount: Integer
/// The number of threads per core in the CPU.
threadsPerCore: Integer
}
structure CreateTaskInput {
/// A list of managed device IDs.
@required
targets: TargetList
/// The task to be performed. Only one task is executed on a device at a time.
@required
command: Command
/// A description of the task and its targets.
description: TaskDescriptionString
/// Optional metadata that you assign to a resource. You can use tags to categorize a resource
/// in different ways, such as by purpose, owner, or environment.
tags: TagMap
/// A token ensuring that the action is called only once with the specified details.
@idempotencyToken
clientToken: IdempotencyToken
}
structure CreateTaskOutput {
/// The ID of the task that you created.
taskId: String
/// The Amazon Resource Name (ARN) of the task that you created.
taskArn: String
}
structure DescribeDeviceEc2Input {
/// The ID of the managed device.
@httpLabel
@required
managedDeviceId: ManagedDeviceId
/// A list of instance IDs associated with the managed device.
@required
instanceIds: InstanceIdsList
}
structure DescribeDeviceEc2Output {
/// A list of structures containing information about each instance.
instances: InstanceSummaryList
}
structure DescribeDeviceInput {
/// The ID of the device that you are checking the information of.
@httpLabel
@required
managedDeviceId: ManagedDeviceId
}
structure DescribeDeviceOutput {
/// When the device last contacted the Amazon Web Services Cloud. Indicates that the device is
/// online.
lastReachedOutAt: Timestamp
/// When the device last pushed an update to the Amazon Web Services Cloud. Indicates when the device cache
/// was refreshed.
lastUpdatedAt: Timestamp
/// Optional metadata that you assign to a resource. You can use tags to categorize a resource
/// in different ways, such as by purpose, owner, or environment.
tags: TagMap
/// The ID of the device that you checked the information for.
managedDeviceId: ManagedDeviceId
/// The Amazon Resource Name (ARN) of the device.
managedDeviceArn: String
/// The type of Amazon Web Services Snow Family device.
deviceType: String
/// The ID of the job used when ordering the device.
associatedWithJob: String
/// The current state of the device.
deviceState: UnlockState
/// The network interfaces available on the device.
physicalNetworkInterfaces: PhysicalNetworkInterfaceList
/// The hardware specifications of the device.
deviceCapacities: CapacityList
/// The software installed on the device.
software: SoftwareInformation
}
structure DescribeExecutionInput {
/// The ID of the task that the action is describing.
@httpLabel
@required
taskId: TaskId
/// The ID of the managed device.
@httpLabel
@required
managedDeviceId: ManagedDeviceId
}
structure DescribeExecutionOutput {
/// The ID of the task being executed on the device.
taskId: TaskId
/// The ID of the execution.
executionId: ExecutionId
/// The ID of the managed device that the task is being executed on.
managedDeviceId: ManagedDeviceId
/// The current state of the execution.
state: ExecutionState
/// When the execution began.
startedAt: Timestamp
/// When the status of the execution was last updated.
lastUpdatedAt: Timestamp
}
structure DescribeTaskInput {
/// The ID of the task to be described.
@httpLabel
@required
taskId: TaskId
}
structure DescribeTaskOutput {
/// The ID of the task.
taskId: String
/// The Amazon Resource Name (ARN) of the task.
taskArn: String
/// The managed devices that the task was sent to.
targets: TargetList
/// The current state of the task.
state: TaskState
/// When the CreateTask
operation was called.
createdAt: Timestamp
/// When the state of the task was last updated.
lastUpdatedAt: Timestamp
/// When the task was completed.
completedAt: Timestamp
/// The description provided of the task and managed devices.
description: TaskDescriptionString
/// Optional metadata that you assign to a resource. You can use tags to categorize a resource
/// in different ways, such as by purpose, owner, or environment.
tags: TagMap
}
/// Identifying information about the device.
structure DeviceSummary {
/// The ID of the device.
managedDeviceId: ManagedDeviceId
/// The Amazon Resource Name (ARN) of the device.
managedDeviceArn: String
/// The ID of the job used to order the device.
associatedWithJob: String
/// Optional metadata that you assign to a resource. You can use tags to categorize a resource
/// in different ways, such as by purpose, owner, or environment.
tags: TagMap
}
/// Describes a parameter used to set up an Amazon Elastic Block Store (Amazon EBS) volume
/// in a block device mapping.
structure EbsInstanceBlockDevice {
/// When the attachment was initiated.
attachTime: Timestamp
/// A value that indicates whether the volume is deleted on instance termination.
deleteOnTermination: Boolean
/// The attachment state.
status: AttachmentStatus
/// The ID of the Amazon EBS volume.
volumeId: String
}
/// The summary of a task execution on a specified device.
structure ExecutionSummary {
/// The ID of the task.
taskId: TaskId
/// The ID of the execution.
executionId: ExecutionId
/// The ID of the managed device that the task is being executed on.
managedDeviceId: ManagedDeviceId
/// The state of the execution.
state: ExecutionState
}
/// The description of an
/// instance.
/// Currently, Amazon EC2 instances are the only supported instance type.
structure Instance {
/// The ID of the AMI used to launch the instance.
imageId: String
/// The Amazon Machine Image (AMI) launch index, which you can use to find this instance in
/// the launch group.
amiLaunchIndex: Integer
/// The ID of the instance.
instanceId: String
state: InstanceState
/// The instance type.
instanceType: String
/// The private IPv4 address assigned to the instance.
privateIpAddress: String
/// The public IPv4 address assigned to the instance.
publicIpAddress: String
/// When the instance was created.
createdAt: Timestamp
/// When the instance was last updated.
updatedAt: Timestamp
/// Any block device mapping entries for the instance.
blockDeviceMappings: InstanceBlockDeviceMappingList
/// The security groups for the instance.
securityGroups: SecurityGroupIdentifierList
/// The CPU options for the instance.
cpuOptions: CpuOptions
/// The device name of the root device volume (for example, /dev/sda1
).
rootDeviceName: String
}
/// The description of a block device mapping.
structure InstanceBlockDeviceMapping {
/// The block device name.
deviceName: String
/// The parameters used to automatically set up Amazon Elastic Block Store (Amazon EBS)
/// volumes when the instance is launched.
ebs: EbsInstanceBlockDevice
}
/// The description of the current state of an instance.
structure InstanceState {
/// The state of the instance as a 16-bit unsigned integer.
/// The high byte is all of the bits between 2^8 and (2^16)-1, which equals decimal values
/// between 256 and 65,535. These numerical values are used for internal purposes and should be
/// ignored.
/// The low byte is all of the bits between 2^0 and (2^8)-1, which equals decimal values
/// between 0 and 255.
/// The valid values for the instance state code are all in the range of the low byte. These
/// values are:
///
/// -
///
/// 0
: pending
///
///
/// -
///
/// 16
: running
///
///
/// -
///
/// 32
: shutting-down
///
///
/// -
///
/// 48
: terminated
///
///
/// -
///
/// 64
: stopping
///
///
/// -
///
/// 80
: stopped
///
///
///
/// You can ignore the high byte value by zeroing out all of the bits above 2^8 or 256 in
/// decimal.
code: Integer
/// The current
/// state
/// of the instance.
name: InstanceStateName
}
/// The details about the instance.
structure InstanceSummary {
/// A structure containing details about the instance.
instance: Instance
/// When the instance summary was last updated.
lastUpdatedAt: Timestamp
}
/// An unexpected error occurred while processing the request.
@error("server")
@httpError(500)
@retryable
structure InternalServerException {
@required
message: String
}
structure ListDeviceResourcesInput {
/// The ID of the managed device that you are listing the resources of.
@httpLabel
@required
managedDeviceId: ManagedDeviceId
/// A structure used to filter the results by type of resource.
@httpQuery("type")
@length(
min: 1
max: 50
)
type: String
/// The maximum number of resources per page.
@httpQuery("maxResults")
maxResults: MaxResults
/// A pagination token to continue to the next page of results.
@httpQuery("nextToken")
nextToken: NextToken
}
structure ListDeviceResourcesOutput {
/// A structure defining the resource's type, Amazon Resource Name (ARN), and ID.
resources: ResourceSummaryList
/// A pagination token to continue to the next page of results.
nextToken: NextToken
}
structure ListDevicesInput {
/// The ID of the job used to order the device.
@httpQuery("jobId")
jobId: JobId
/// The maximum number of devices to list per page.
@httpQuery("maxResults")
maxResults: MaxResults
/// A pagination token to continue to the next page of results.
@httpQuery("nextToken")
nextToken: NextToken
}
structure ListDevicesOutput {
/// A list of device structures that contain information about the device.
devices: DeviceSummaryList
/// A pagination token to continue to the next page of devices.
nextToken: NextToken
}
structure ListExecutionsInput {
/// The ID of the task.
@httpQuery("taskId")
@required
taskId: TaskId
/// A structure used to filter the tasks by their current state.
@httpQuery("state")
state: ExecutionState
/// The maximum number of tasks to list per page.
@httpQuery("maxResults")
maxResults: MaxResults
/// A pagination token to continue to the next page of tasks.
@httpQuery("nextToken")
nextToken: NextToken
}
structure ListExecutionsOutput {
/// A list of executions. Each execution contains the task ID, the device that the task is
/// executing on, the execution ID, and the status of the execution.
executions: ExecutionSummaryList
/// A pagination token to continue to the next page of executions.
nextToken: NextToken
}
structure ListTagsForResourceInput {
/// The Amazon Resource Name (ARN) of the device or task.
@httpLabel
@required
resourceArn: String
}
structure ListTagsForResourceOutput {
/// The list of tags for the device or task.
tags: TagMap
}
structure ListTasksInput {
/// A structure used to filter the list of tasks.
@httpQuery("state")
state: TaskState
/// The maximum number of tasks per page.
@httpQuery("maxResults")
maxResults: MaxResults
/// A pagination token to continue to the next page of tasks.
@httpQuery("nextToken")
nextToken: NextToken
}
structure ListTasksOutput {
/// A list of task structures containing details about each task.
tasks: TaskSummaryList
/// A pagination token to continue to the next page of tasks.
nextToken: NextToken
}
/// The details about the physical network interface for the device.
structure PhysicalNetworkInterface {
/// The physical network interface ID.
physicalNetworkInterfaceId: String
/// The
/// physical
/// connector type.
physicalConnectorType: PhysicalConnectorType
/// A value that describes whether the IP address is dynamic or persistent.
ipAddressAssignment: IpAddressAssignment
/// The IP address of the device.
ipAddress: String
/// The netmask used to divide the IP address into subnets.
netmask: String
/// The default gateway of the device.
defaultGateway: String
/// The MAC address of the device.
macAddress: String
}
/// A structure used to reboot the device.
structure Reboot {}
/// The request references a resource that doesn't exist.
@error("client")
@httpError(404)
structure ResourceNotFoundException {
@required
message: String
}
/// A summary of a resource available on the device.
structure ResourceSummary {
/// The resource type.
@required
resourceType: String
/// The Amazon Resource Name (ARN) of the resource.
arn: String
/// The ID of the resource.
id: String
}
/// Information about the device's security group.
structure SecurityGroupIdentifier {
/// The security group ID.
groupId: String
/// The security group name.
groupName: String
}
/// The request would cause a service quota to be exceeded.
@error("client")
@httpError(402)
structure ServiceQuotaExceededException {
@required
message: String
}
/// Information about the software on the device.
structure SoftwareInformation {
/// The version of the software currently installed on the device.
installedVersion: String
/// The version of the software being installed on the device.
installingVersion: String
/// The state of the software that is installed or that is being installed on the
/// device.
installState: String
}
structure TagResourceInput {
/// The Amazon Resource Name (ARN) of the device or task.
@httpLabel
@required
resourceArn: String
/// Optional metadata that you assign to a resource. You can use tags to categorize a resource
/// in different ways, such as by purpose, owner, or environment.
@required
tags: TagMap
}
/// Information about the task assigned to one or many devices.
structure TaskSummary {
/// The task ID.
@required
taskId: TaskId
/// The Amazon Resource Name (ARN) of the task.
taskArn: String
/// The state of the task assigned to one or many devices.
state: TaskState
/// Optional metadata that you assign to a resource. You can use tags to categorize a resource
/// in different ways, such as by purpose, owner, or environment.
tags: TagMap
}
/// The request was denied due to request throttling.
@error("client")
@httpError(429)
@retryable(
throttling: true
)
structure ThrottlingException {
@required
message: String
}
/// A structure used to unlock a device.
structure Unlock {}
structure UntagResourceInput {
/// The Amazon Resource Name (ARN) of the device or task.
@httpLabel
@required
resourceArn: String
/// Optional metadata that you assign to a resource. You can use tags to categorize a resource
/// in different ways, such as by purpose, owner, or environment.
@httpQuery("tagKeys")
@required
tagKeys: TagKeys
}
/// The input fails to satisfy the constraints specified by an Amazon Web Services service.
@error("client")
@httpError(400)
structure ValidationException {
@required
message: String
}
/// The command given to the device to execute.
union Command {
/// Unlocks the device.
unlock: Unlock
/// Reboots the device.
reboot: Reboot
}
@length(
min: 0
max: 100
)
list CapacityList {
member: Capacity
}
list DeviceSummaryList {
member: DeviceSummary
}
list ExecutionSummaryList {
member: ExecutionSummary
}
list InstanceBlockDeviceMappingList {
member: InstanceBlockDeviceMapping
}
list InstanceIdsList {
member: String
}
list InstanceSummaryList {
member: InstanceSummary
}
list PhysicalNetworkInterfaceList {
member: PhysicalNetworkInterface
}
list ResourceSummaryList {
member: ResourceSummary
}
list SecurityGroupIdentifierList {
member: SecurityGroupIdentifier
}
list TagKeys {
member: String
}
@length(
min: 1
max: 10
)
list TargetList {
member: String
}
list TaskSummaryList {
member: TaskSummary
}
map TagMap {
key: String
value: String
}
@enum([
{
value: "ATTACHING"
name: "ATTACHING"
}
{
value: "ATTACHED"
name: "ATTACHED"
}
{
value: "DETACHING"
name: "DETACHING"
}
{
value: "DETACHED"
name: "DETACHED"
}
])
string AttachmentStatus
@length(
min: 1
max: 64
)
string ExecutionId
@enum([
{
value: "QUEUED"
name: "QUEUED"
}
{
value: "IN_PROGRESS"
name: "IN_PROGRESS"
}
{
value: "CANCELED"
name: "CANCELED"
}
{
value: "FAILED"
name: "FAILED"
}
{
value: "SUCCEEDED"
name: "SUCCEEDED"
}
{
value: "REJECTED"
name: "REJECTED"
}
{
value: "TIMED_OUT"
name: "TIMED_OUT"
}
])
string ExecutionState
@length(
min: 1
max: 64
)
@pattern("[!-~]+")
string IdempotencyToken
@enum([
{
value: "PENDING"
name: "PENDING"
}
{
value: "RUNNING"
name: "RUNNING"
}
{
value: "SHUTTING_DOWN"
name: "SHUTTING_DOWN"
}
{
value: "TERMINATED"
name: "TERMINATED"
}
{
value: "STOPPING"
name: "STOPPING"
}
{
value: "STOPPED"
name: "STOPPED"
}
])
string InstanceStateName
@enum([
{
value: "DHCP"
name: "DHCP"
}
{
value: "STATIC"
name: "STATIC"
}
])
string IpAddressAssignment
@length(
min: 1
max: 64
)
string JobId
@length(
min: 1
max: 64
)
string ManagedDeviceId
@range(
min: 1
max: 100
)
integer MaxResults
@length(
min: 1
max: 1024
)
@pattern("[a-zA-Z0-9+/=]*")
string NextToken
@enum([
{
value: "RJ45"
name: "RJ45"
}
{
value: "SFP_PLUS"
name: "SFP_PLUS"
}
{
value: "QSFP"
name: "QSFP"
}
{
value: "RJ45_2"
name: "RJ45_2"
}
{
value: "WIFI"
name: "WIFI"
}
])
string PhysicalConnectorType
@length(
min: 1
max: 128
)
@pattern("[A-Za-z0-9 _.,!#]*")
string TaskDescriptionString
@length(
min: 1
max: 64
)
string TaskId
@enum([
{
value: "IN_PROGRESS"
name: "IN_PROGRESS"
}
{
value: "CANCELED"
name: "CANCELED"
}
{
value: "COMPLETED"
name: "COMPLETED"
}
])
string TaskState
@enum([
{
value: "UNLOCKED"
name: "UNLOCKED"
}
{
value: "LOCKED"
name: "LOCKED"
}
{
value: "UNLOCKING"
name: "UNLOCKING"
}
])
string UnlockState