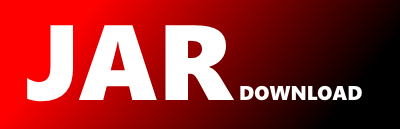
smithy.waiters.Matcher.scala Maven / Gradle / Ivy
package smithy.waiters
import smithy4s.Hints
import smithy4s.Schema
import smithy4s.ShapeId
import smithy4s.ShapeTag
import smithy4s.schema.Schema.bijection
import smithy4s.schema.Schema.boolean
import smithy4s.schema.Schema.string
import smithy4s.schema.Schema.union
/** Defines how an acceptor determines if it matches the current state of
* a resource.
*/
sealed trait Matcher extends scala.Product with scala.Serializable { self =>
@inline final def widen: Matcher = this
def $ordinal: Int
object project {
def output: Option[PathMatcher] = Matcher.OutputCase.alt.project.lift(self).map(_.output)
def inputOutput: Option[PathMatcher] = Matcher.InputOutputCase.alt.project.lift(self).map(_.inputOutput)
def errorType: Option[String] = Matcher.ErrorTypeCase.alt.project.lift(self).map(_.errorType)
def success: Option[Boolean] = Matcher.SuccessCase.alt.project.lift(self).map(_.success)
}
def accept[A](visitor: Matcher.Visitor[A]): A = this match {
case value: Matcher.OutputCase => visitor.output(value.output)
case value: Matcher.InputOutputCase => visitor.inputOutput(value.inputOutput)
case value: Matcher.ErrorTypeCase => visitor.errorType(value.errorType)
case value: Matcher.SuccessCase => visitor.success(value.success)
}
}
object Matcher extends ShapeTag.Companion[Matcher] {
/** Matches on the successful output of an operation using a
* JMESPath expression.
*/
def output(output: PathMatcher): Matcher = OutputCase(output)
/** Matches on both the input and output of an operation using a JMESPath
* expression. Input parameters are available through the top-level
* `input` field, and output data is available through the top-level
* `output` field. This matcher can only be used on operations that
* define both input and output. This matcher is checked only if an
* operation completes successfully.
*/
def inputOutput(inputOutput: PathMatcher): Matcher = InputOutputCase(inputOutput)
/** Matches if an operation returns an error and the error matches
* the expected error type. If an absolute shape ID is provided, the
* error is matched exactly on the shape ID. A shape name can be
* provided to match an error in any namespace with the given name.
*/
def errorType(errorType: String): Matcher = ErrorTypeCase(errorType)
/** When set to `true`, matches when an operation returns a successful
* response. When set to `false`, matches when an operation fails with
* any error.
*/
def success(success: Boolean): Matcher = SuccessCase(success)
val id: ShapeId = ShapeId("smithy.waiters", "Matcher")
val hints: Hints = Hints(
smithy.api.Documentation("Defines how an acceptor determines if it matches the current state of\na resource."),
smithy.api.Private(),
).lazily
/** Matches on the successful output of an operation using a
* JMESPath expression.
*/
final case class OutputCase(output: PathMatcher) extends Matcher { final def $ordinal: Int = 0 }
/** Matches on both the input and output of an operation using a JMESPath
* expression. Input parameters are available through the top-level
* `input` field, and output data is available through the top-level
* `output` field. This matcher can only be used on operations that
* define both input and output. This matcher is checked only if an
* operation completes successfully.
*/
final case class InputOutputCase(inputOutput: PathMatcher) extends Matcher { final def $ordinal: Int = 1 }
/** Matches if an operation returns an error and the error matches
* the expected error type. If an absolute shape ID is provided, the
* error is matched exactly on the shape ID. A shape name can be
* provided to match an error in any namespace with the given name.
*/
final case class ErrorTypeCase(errorType: String) extends Matcher { final def $ordinal: Int = 2 }
/** When set to `true`, matches when an operation returns a successful
* response. When set to `false`, matches when an operation fails with
* any error.
*/
final case class SuccessCase(success: Boolean) extends Matcher { final def $ordinal: Int = 3 }
object OutputCase {
val hints: Hints = Hints(
smithy.api.Documentation("Matches on the successful output of an operation using a\nJMESPath expression."),
).lazily
val schema: Schema[Matcher.OutputCase] = bijection(PathMatcher.schema.addHints(hints), Matcher.OutputCase(_), _.output)
val alt = schema.oneOf[Matcher]("output")
}
object InputOutputCase {
val hints: Hints = Hints(
smithy.api.Documentation("Matches on both the input and output of an operation using a JMESPath\nexpression. Input parameters are available through the top-level\n`input` field, and output data is available through the top-level\n`output` field. This matcher can only be used on operations that\ndefine both input and output. This matcher is checked only if an\noperation completes successfully."),
).lazily
val schema: Schema[Matcher.InputOutputCase] = bijection(PathMatcher.schema.addHints(hints), Matcher.InputOutputCase(_), _.inputOutput)
val alt = schema.oneOf[Matcher]("inputOutput")
}
object ErrorTypeCase {
val hints: Hints = Hints(
smithy.api.Documentation("Matches if an operation returns an error and the error matches\nthe expected error type. If an absolute shape ID is provided, the\nerror is matched exactly on the shape ID. A shape name can be\nprovided to match an error in any namespace with the given name."),
).lazily
val schema: Schema[Matcher.ErrorTypeCase] = bijection(string.addHints(hints), Matcher.ErrorTypeCase(_), _.errorType)
val alt = schema.oneOf[Matcher]("errorType")
}
object SuccessCase {
val hints: Hints = Hints(
smithy.api.Documentation("When set to `true`, matches when an operation returns a successful\nresponse. When set to `false`, matches when an operation fails with\nany error."),
).lazily
val schema: Schema[Matcher.SuccessCase] = bijection(boolean.addHints(hints), Matcher.SuccessCase(_), _.success)
val alt = schema.oneOf[Matcher]("success")
}
trait Visitor[A] {
def output(value: PathMatcher): A
def inputOutput(value: PathMatcher): A
def errorType(value: String): A
def success(value: Boolean): A
}
object Visitor {
trait Default[A] extends Visitor[A] {
def default: A
def output(value: PathMatcher): A = default
def inputOutput(value: PathMatcher): A = default
def errorType(value: String): A = default
def success(value: Boolean): A = default
}
}
implicit val schema: Schema[Matcher] = union(
Matcher.OutputCase.alt,
Matcher.InputOutputCase.alt,
Matcher.ErrorTypeCase.alt,
Matcher.SuccessCase.alt,
){
_.$ordinal
}.withId(id).addHints(hints)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy