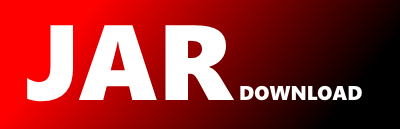
com.youtube.vitess.proto.grpc.VitessGrpc Maven / Gradle / Ivy
The newest version!
package com.youtube.vitess.proto.grpc;
import static io.grpc.stub.ClientCalls.asyncUnaryCall;
import static io.grpc.stub.ClientCalls.asyncServerStreamingCall;
import static io.grpc.stub.ClientCalls.asyncClientStreamingCall;
import static io.grpc.stub.ClientCalls.asyncBidiStreamingCall;
import static io.grpc.stub.ClientCalls.blockingUnaryCall;
import static io.grpc.stub.ClientCalls.blockingServerStreamingCall;
import static io.grpc.stub.ClientCalls.futureUnaryCall;
import static io.grpc.MethodDescriptor.generateFullMethodName;
import static io.grpc.stub.ServerCalls.asyncUnaryCall;
import static io.grpc.stub.ServerCalls.asyncServerStreamingCall;
import static io.grpc.stub.ServerCalls.asyncClientStreamingCall;
import static io.grpc.stub.ServerCalls.asyncBidiStreamingCall;
import static io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall;
import static io.grpc.stub.ServerCalls.asyncUnimplementedStreamingCall;
/**
*
* Vitess is the main service to access a Vitess cluster. It is the API that vtgate
* exposes to serve all queries.
*
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.1.2)",
comments = "Source: vtgateservice.proto")
public class VitessGrpc {
private VitessGrpc() {}
public static final String SERVICE_NAME = "vtgateservice.Vitess";
// Static method descriptors that strictly reflect the proto.
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_EXECUTE =
io.grpc.MethodDescriptor.create(
io.grpc.MethodDescriptor.MethodType.UNARY,
generateFullMethodName(
"vtgateservice.Vitess", "Execute"),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.ExecuteRequest.getDefaultInstance()),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.ExecuteResponse.getDefaultInstance()));
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_EXECUTE_SHARDS =
io.grpc.MethodDescriptor.create(
io.grpc.MethodDescriptor.MethodType.UNARY,
generateFullMethodName(
"vtgateservice.Vitess", "ExecuteShards"),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.ExecuteShardsRequest.getDefaultInstance()),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.ExecuteShardsResponse.getDefaultInstance()));
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_EXECUTE_KEYSPACE_IDS =
io.grpc.MethodDescriptor.create(
io.grpc.MethodDescriptor.MethodType.UNARY,
generateFullMethodName(
"vtgateservice.Vitess", "ExecuteKeyspaceIds"),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.ExecuteKeyspaceIdsRequest.getDefaultInstance()),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.ExecuteKeyspaceIdsResponse.getDefaultInstance()));
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_EXECUTE_KEY_RANGES =
io.grpc.MethodDescriptor.create(
io.grpc.MethodDescriptor.MethodType.UNARY,
generateFullMethodName(
"vtgateservice.Vitess", "ExecuteKeyRanges"),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.ExecuteKeyRangesRequest.getDefaultInstance()),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.ExecuteKeyRangesResponse.getDefaultInstance()));
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_EXECUTE_ENTITY_IDS =
io.grpc.MethodDescriptor.create(
io.grpc.MethodDescriptor.MethodType.UNARY,
generateFullMethodName(
"vtgateservice.Vitess", "ExecuteEntityIds"),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.ExecuteEntityIdsRequest.getDefaultInstance()),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.ExecuteEntityIdsResponse.getDefaultInstance()));
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_EXECUTE_BATCH =
io.grpc.MethodDescriptor.create(
io.grpc.MethodDescriptor.MethodType.UNARY,
generateFullMethodName(
"vtgateservice.Vitess", "ExecuteBatch"),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.ExecuteBatchRequest.getDefaultInstance()),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.ExecuteBatchResponse.getDefaultInstance()));
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_EXECUTE_BATCH_SHARDS =
io.grpc.MethodDescriptor.create(
io.grpc.MethodDescriptor.MethodType.UNARY,
generateFullMethodName(
"vtgateservice.Vitess", "ExecuteBatchShards"),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.ExecuteBatchShardsRequest.getDefaultInstance()),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.ExecuteBatchShardsResponse.getDefaultInstance()));
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_EXECUTE_BATCH_KEYSPACE_IDS =
io.grpc.MethodDescriptor.create(
io.grpc.MethodDescriptor.MethodType.UNARY,
generateFullMethodName(
"vtgateservice.Vitess", "ExecuteBatchKeyspaceIds"),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.ExecuteBatchKeyspaceIdsRequest.getDefaultInstance()),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.ExecuteBatchKeyspaceIdsResponse.getDefaultInstance()));
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_STREAM_EXECUTE =
io.grpc.MethodDescriptor.create(
io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING,
generateFullMethodName(
"vtgateservice.Vitess", "StreamExecute"),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.StreamExecuteRequest.getDefaultInstance()),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.StreamExecuteResponse.getDefaultInstance()));
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_STREAM_EXECUTE_SHARDS =
io.grpc.MethodDescriptor.create(
io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING,
generateFullMethodName(
"vtgateservice.Vitess", "StreamExecuteShards"),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.StreamExecuteShardsRequest.getDefaultInstance()),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.StreamExecuteShardsResponse.getDefaultInstance()));
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_STREAM_EXECUTE_KEYSPACE_IDS =
io.grpc.MethodDescriptor.create(
io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING,
generateFullMethodName(
"vtgateservice.Vitess", "StreamExecuteKeyspaceIds"),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.StreamExecuteKeyspaceIdsRequest.getDefaultInstance()),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.StreamExecuteKeyspaceIdsResponse.getDefaultInstance()));
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_STREAM_EXECUTE_KEY_RANGES =
io.grpc.MethodDescriptor.create(
io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING,
generateFullMethodName(
"vtgateservice.Vitess", "StreamExecuteKeyRanges"),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.StreamExecuteKeyRangesRequest.getDefaultInstance()),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.StreamExecuteKeyRangesResponse.getDefaultInstance()));
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_BEGIN =
io.grpc.MethodDescriptor.create(
io.grpc.MethodDescriptor.MethodType.UNARY,
generateFullMethodName(
"vtgateservice.Vitess", "Begin"),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.BeginRequest.getDefaultInstance()),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.BeginResponse.getDefaultInstance()));
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_COMMIT =
io.grpc.MethodDescriptor.create(
io.grpc.MethodDescriptor.MethodType.UNARY,
generateFullMethodName(
"vtgateservice.Vitess", "Commit"),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.CommitRequest.getDefaultInstance()),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.CommitResponse.getDefaultInstance()));
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_ROLLBACK =
io.grpc.MethodDescriptor.create(
io.grpc.MethodDescriptor.MethodType.UNARY,
generateFullMethodName(
"vtgateservice.Vitess", "Rollback"),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.RollbackRequest.getDefaultInstance()),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.RollbackResponse.getDefaultInstance()));
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_RESOLVE_TRANSACTION =
io.grpc.MethodDescriptor.create(
io.grpc.MethodDescriptor.MethodType.UNARY,
generateFullMethodName(
"vtgateservice.Vitess", "ResolveTransaction"),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.ResolveTransactionRequest.getDefaultInstance()),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.ResolveTransactionResponse.getDefaultInstance()));
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_MESSAGE_STREAM =
io.grpc.MethodDescriptor.create(
io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING,
generateFullMethodName(
"vtgateservice.Vitess", "MessageStream"),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.MessageStreamRequest.getDefaultInstance()),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Query.MessageStreamResponse.getDefaultInstance()));
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_MESSAGE_ACK =
io.grpc.MethodDescriptor.create(
io.grpc.MethodDescriptor.MethodType.UNARY,
generateFullMethodName(
"vtgateservice.Vitess", "MessageAck"),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.MessageAckRequest.getDefaultInstance()),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Query.MessageAckResponse.getDefaultInstance()));
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_SPLIT_QUERY =
io.grpc.MethodDescriptor.create(
io.grpc.MethodDescriptor.MethodType.UNARY,
generateFullMethodName(
"vtgateservice.Vitess", "SplitQuery"),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.SplitQueryRequest.getDefaultInstance()),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.SplitQueryResponse.getDefaultInstance()));
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_GET_SRV_KEYSPACE =
io.grpc.MethodDescriptor.create(
io.grpc.MethodDescriptor.MethodType.UNARY,
generateFullMethodName(
"vtgateservice.Vitess", "GetSrvKeyspace"),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.GetSrvKeyspaceRequest.getDefaultInstance()),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.GetSrvKeyspaceResponse.getDefaultInstance()));
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static final io.grpc.MethodDescriptor METHOD_UPDATE_STREAM =
io.grpc.MethodDescriptor.create(
io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING,
generateFullMethodName(
"vtgateservice.Vitess", "UpdateStream"),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.UpdateStreamRequest.getDefaultInstance()),
io.grpc.protobuf.ProtoUtils.marshaller(com.youtube.vitess.proto.Vtgate.UpdateStreamResponse.getDefaultInstance()));
/**
* Creates a new async stub that supports all call types for the service
*/
public static VitessStub newStub(io.grpc.Channel channel) {
return new VitessStub(channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static VitessBlockingStub newBlockingStub(
io.grpc.Channel channel) {
return new VitessBlockingStub(channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary and streaming output calls on the service
*/
public static VitessFutureStub newFutureStub(
io.grpc.Channel channel) {
return new VitessFutureStub(channel);
}
/**
*
* Vitess is the main service to access a Vitess cluster. It is the API that vtgate
* exposes to serve all queries.
*
*/
public static abstract class VitessImplBase implements io.grpc.BindableService {
/**
*
* Execute tries to route the query to the right shard.
* It depends on the query and bind variables to provide enough
* information in conjonction with the vindexes to route the query.
* API group: v3 API (alpha)
*
*/
public void execute(com.youtube.vitess.proto.Vtgate.ExecuteRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_EXECUTE, responseObserver);
}
/**
*
* ExecuteShards executes the query on the specified shards.
* API group: Custom Sharding
*
*/
public void executeShards(com.youtube.vitess.proto.Vtgate.ExecuteShardsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_EXECUTE_SHARDS, responseObserver);
}
/**
*
* ExecuteKeyspaceIds executes the query based on the specified keyspace ids.
* API group: Range-based Sharding
*
*/
public void executeKeyspaceIds(com.youtube.vitess.proto.Vtgate.ExecuteKeyspaceIdsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_EXECUTE_KEYSPACE_IDS, responseObserver);
}
/**
*
* ExecuteKeyRanges executes the query based on the specified key ranges.
* API group: Range-based Sharding
*
*/
public void executeKeyRanges(com.youtube.vitess.proto.Vtgate.ExecuteKeyRangesRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_EXECUTE_KEY_RANGES, responseObserver);
}
/**
*
* ExecuteEntityIds executes the query based on the specified external id to keyspace id map.
* API group: Range-based Sharding
*
*/
public void executeEntityIds(com.youtube.vitess.proto.Vtgate.ExecuteEntityIdsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_EXECUTE_ENTITY_IDS, responseObserver);
}
/**
*
* ExecuteBatch tries to route the list of queries on the right shards.
* It depends on the query and bind variables to provide enough
* information in conjonction with the vindexes to route the query.
* API group: v3 API
*
*/
public void executeBatch(com.youtube.vitess.proto.Vtgate.ExecuteBatchRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_EXECUTE_BATCH, responseObserver);
}
/**
*
* ExecuteBatchShards executes the list of queries on the specified shards.
* API group: Custom Sharding
*
*/
public void executeBatchShards(com.youtube.vitess.proto.Vtgate.ExecuteBatchShardsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_EXECUTE_BATCH_SHARDS, responseObserver);
}
/**
*
* ExecuteBatchKeyspaceIds executes the list of queries based on the specified keyspace ids.
* API group: Range-based Sharding
*
*/
public void executeBatchKeyspaceIds(com.youtube.vitess.proto.Vtgate.ExecuteBatchKeyspaceIdsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_EXECUTE_BATCH_KEYSPACE_IDS, responseObserver);
}
/**
*
* StreamExecute executes a streaming query based on shards.
* It depends on the query and bind variables to provide enough
* information in conjonction with the vindexes to route the query.
* Use this method if the query returns a large number of rows.
* API group: v3 API (alpha)
*
*/
public void streamExecute(com.youtube.vitess.proto.Vtgate.StreamExecuteRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_STREAM_EXECUTE, responseObserver);
}
/**
*
* StreamExecuteShards executes a streaming query based on shards.
* Use this method if the query returns a large number of rows.
* API group: Custom Sharding
*
*/
public void streamExecuteShards(com.youtube.vitess.proto.Vtgate.StreamExecuteShardsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_STREAM_EXECUTE_SHARDS, responseObserver);
}
/**
*
* StreamExecuteKeyspaceIds executes a streaming query based on keyspace ids.
* Use this method if the query returns a large number of rows.
* API group: Range-based Sharding
*
*/
public void streamExecuteKeyspaceIds(com.youtube.vitess.proto.Vtgate.StreamExecuteKeyspaceIdsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_STREAM_EXECUTE_KEYSPACE_IDS, responseObserver);
}
/**
*
* StreamExecuteKeyRanges executes a streaming query based on key ranges.
* Use this method if the query returns a large number of rows.
* API group: Range-based Sharding
*
*/
public void streamExecuteKeyRanges(com.youtube.vitess.proto.Vtgate.StreamExecuteKeyRangesRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_STREAM_EXECUTE_KEY_RANGES, responseObserver);
}
/**
*
* Begin a transaction.
* API group: Transactions
*
*/
public void begin(com.youtube.vitess.proto.Vtgate.BeginRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_BEGIN, responseObserver);
}
/**
*
* Commit a transaction.
* API group: Transactions
*
*/
public void commit(com.youtube.vitess.proto.Vtgate.CommitRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_COMMIT, responseObserver);
}
/**
*
* Rollback a transaction.
* API group: Transactions
*
*/
public void rollback(com.youtube.vitess.proto.Vtgate.RollbackRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_ROLLBACK, responseObserver);
}
/**
*
* ResolveTransaction resolves a transaction.
* API group: Transactions
*
*/
public void resolveTransaction(com.youtube.vitess.proto.Vtgate.ResolveTransactionRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_RESOLVE_TRANSACTION, responseObserver);
}
/**
*
* MessageStream streams messages from a message table.
*
*/
public void messageStream(com.youtube.vitess.proto.Vtgate.MessageStreamRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_MESSAGE_STREAM, responseObserver);
}
/**
*
* MessageAck acks messages for a table.
*
*/
public void messageAck(com.youtube.vitess.proto.Vtgate.MessageAckRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_MESSAGE_ACK, responseObserver);
}
/**
*
* Split a query into non-overlapping sub queries
* API group: Map Reduce
*
*/
public void splitQuery(com.youtube.vitess.proto.Vtgate.SplitQueryRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_SPLIT_QUERY, responseObserver);
}
/**
*
* GetSrvKeyspace returns a SrvKeyspace object (as seen by this vtgate).
* This method is provided as a convenient way for clients to take a
* look at the sharding configuration for a Keyspace. Looking at the
* sharding information should not be used for routing queries (as the
* information may change, use the Execute calls for that).
* It is convenient for monitoring applications for instance, or if
* using custom sharding.
* API group: Topology
*
*/
public void getSrvKeyspace(com.youtube.vitess.proto.Vtgate.GetSrvKeyspaceRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_GET_SRV_KEYSPACE, responseObserver);
}
/**
*
* UpdateStream asks the server for a stream of StreamEvent objects.
* API group: Update Stream
*
*/
public void updateStream(com.youtube.vitess.proto.Vtgate.UpdateStreamRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(METHOD_UPDATE_STREAM, responseObserver);
}
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
METHOD_EXECUTE,
asyncUnaryCall(
new MethodHandlers<
com.youtube.vitess.proto.Vtgate.ExecuteRequest,
com.youtube.vitess.proto.Vtgate.ExecuteResponse>(
this, METHODID_EXECUTE)))
.addMethod(
METHOD_EXECUTE_SHARDS,
asyncUnaryCall(
new MethodHandlers<
com.youtube.vitess.proto.Vtgate.ExecuteShardsRequest,
com.youtube.vitess.proto.Vtgate.ExecuteShardsResponse>(
this, METHODID_EXECUTE_SHARDS)))
.addMethod(
METHOD_EXECUTE_KEYSPACE_IDS,
asyncUnaryCall(
new MethodHandlers<
com.youtube.vitess.proto.Vtgate.ExecuteKeyspaceIdsRequest,
com.youtube.vitess.proto.Vtgate.ExecuteKeyspaceIdsResponse>(
this, METHODID_EXECUTE_KEYSPACE_IDS)))
.addMethod(
METHOD_EXECUTE_KEY_RANGES,
asyncUnaryCall(
new MethodHandlers<
com.youtube.vitess.proto.Vtgate.ExecuteKeyRangesRequest,
com.youtube.vitess.proto.Vtgate.ExecuteKeyRangesResponse>(
this, METHODID_EXECUTE_KEY_RANGES)))
.addMethod(
METHOD_EXECUTE_ENTITY_IDS,
asyncUnaryCall(
new MethodHandlers<
com.youtube.vitess.proto.Vtgate.ExecuteEntityIdsRequest,
com.youtube.vitess.proto.Vtgate.ExecuteEntityIdsResponse>(
this, METHODID_EXECUTE_ENTITY_IDS)))
.addMethod(
METHOD_EXECUTE_BATCH,
asyncUnaryCall(
new MethodHandlers<
com.youtube.vitess.proto.Vtgate.ExecuteBatchRequest,
com.youtube.vitess.proto.Vtgate.ExecuteBatchResponse>(
this, METHODID_EXECUTE_BATCH)))
.addMethod(
METHOD_EXECUTE_BATCH_SHARDS,
asyncUnaryCall(
new MethodHandlers<
com.youtube.vitess.proto.Vtgate.ExecuteBatchShardsRequest,
com.youtube.vitess.proto.Vtgate.ExecuteBatchShardsResponse>(
this, METHODID_EXECUTE_BATCH_SHARDS)))
.addMethod(
METHOD_EXECUTE_BATCH_KEYSPACE_IDS,
asyncUnaryCall(
new MethodHandlers<
com.youtube.vitess.proto.Vtgate.ExecuteBatchKeyspaceIdsRequest,
com.youtube.vitess.proto.Vtgate.ExecuteBatchKeyspaceIdsResponse>(
this, METHODID_EXECUTE_BATCH_KEYSPACE_IDS)))
.addMethod(
METHOD_STREAM_EXECUTE,
asyncServerStreamingCall(
new MethodHandlers<
com.youtube.vitess.proto.Vtgate.StreamExecuteRequest,
com.youtube.vitess.proto.Vtgate.StreamExecuteResponse>(
this, METHODID_STREAM_EXECUTE)))
.addMethod(
METHOD_STREAM_EXECUTE_SHARDS,
asyncServerStreamingCall(
new MethodHandlers<
com.youtube.vitess.proto.Vtgate.StreamExecuteShardsRequest,
com.youtube.vitess.proto.Vtgate.StreamExecuteShardsResponse>(
this, METHODID_STREAM_EXECUTE_SHARDS)))
.addMethod(
METHOD_STREAM_EXECUTE_KEYSPACE_IDS,
asyncServerStreamingCall(
new MethodHandlers<
com.youtube.vitess.proto.Vtgate.StreamExecuteKeyspaceIdsRequest,
com.youtube.vitess.proto.Vtgate.StreamExecuteKeyspaceIdsResponse>(
this, METHODID_STREAM_EXECUTE_KEYSPACE_IDS)))
.addMethod(
METHOD_STREAM_EXECUTE_KEY_RANGES,
asyncServerStreamingCall(
new MethodHandlers<
com.youtube.vitess.proto.Vtgate.StreamExecuteKeyRangesRequest,
com.youtube.vitess.proto.Vtgate.StreamExecuteKeyRangesResponse>(
this, METHODID_STREAM_EXECUTE_KEY_RANGES)))
.addMethod(
METHOD_BEGIN,
asyncUnaryCall(
new MethodHandlers<
com.youtube.vitess.proto.Vtgate.BeginRequest,
com.youtube.vitess.proto.Vtgate.BeginResponse>(
this, METHODID_BEGIN)))
.addMethod(
METHOD_COMMIT,
asyncUnaryCall(
new MethodHandlers<
com.youtube.vitess.proto.Vtgate.CommitRequest,
com.youtube.vitess.proto.Vtgate.CommitResponse>(
this, METHODID_COMMIT)))
.addMethod(
METHOD_ROLLBACK,
asyncUnaryCall(
new MethodHandlers<
com.youtube.vitess.proto.Vtgate.RollbackRequest,
com.youtube.vitess.proto.Vtgate.RollbackResponse>(
this, METHODID_ROLLBACK)))
.addMethod(
METHOD_RESOLVE_TRANSACTION,
asyncUnaryCall(
new MethodHandlers<
com.youtube.vitess.proto.Vtgate.ResolveTransactionRequest,
com.youtube.vitess.proto.Vtgate.ResolveTransactionResponse>(
this, METHODID_RESOLVE_TRANSACTION)))
.addMethod(
METHOD_MESSAGE_STREAM,
asyncServerStreamingCall(
new MethodHandlers<
com.youtube.vitess.proto.Vtgate.MessageStreamRequest,
com.youtube.vitess.proto.Query.MessageStreamResponse>(
this, METHODID_MESSAGE_STREAM)))
.addMethod(
METHOD_MESSAGE_ACK,
asyncUnaryCall(
new MethodHandlers<
com.youtube.vitess.proto.Vtgate.MessageAckRequest,
com.youtube.vitess.proto.Query.MessageAckResponse>(
this, METHODID_MESSAGE_ACK)))
.addMethod(
METHOD_SPLIT_QUERY,
asyncUnaryCall(
new MethodHandlers<
com.youtube.vitess.proto.Vtgate.SplitQueryRequest,
com.youtube.vitess.proto.Vtgate.SplitQueryResponse>(
this, METHODID_SPLIT_QUERY)))
.addMethod(
METHOD_GET_SRV_KEYSPACE,
asyncUnaryCall(
new MethodHandlers<
com.youtube.vitess.proto.Vtgate.GetSrvKeyspaceRequest,
com.youtube.vitess.proto.Vtgate.GetSrvKeyspaceResponse>(
this, METHODID_GET_SRV_KEYSPACE)))
.addMethod(
METHOD_UPDATE_STREAM,
asyncServerStreamingCall(
new MethodHandlers<
com.youtube.vitess.proto.Vtgate.UpdateStreamRequest,
com.youtube.vitess.proto.Vtgate.UpdateStreamResponse>(
this, METHODID_UPDATE_STREAM)))
.build();
}
}
/**
*
* Vitess is the main service to access a Vitess cluster. It is the API that vtgate
* exposes to serve all queries.
*
*/
public static final class VitessStub extends io.grpc.stub.AbstractStub {
private VitessStub(io.grpc.Channel channel) {
super(channel);
}
private VitessStub(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected VitessStub build(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
return new VitessStub(channel, callOptions);
}
/**
*
* Execute tries to route the query to the right shard.
* It depends on the query and bind variables to provide enough
* information in conjonction with the vindexes to route the query.
* API group: v3 API (alpha)
*
*/
public void execute(com.youtube.vitess.proto.Vtgate.ExecuteRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(METHOD_EXECUTE, getCallOptions()), request, responseObserver);
}
/**
*
* ExecuteShards executes the query on the specified shards.
* API group: Custom Sharding
*
*/
public void executeShards(com.youtube.vitess.proto.Vtgate.ExecuteShardsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(METHOD_EXECUTE_SHARDS, getCallOptions()), request, responseObserver);
}
/**
*
* ExecuteKeyspaceIds executes the query based on the specified keyspace ids.
* API group: Range-based Sharding
*
*/
public void executeKeyspaceIds(com.youtube.vitess.proto.Vtgate.ExecuteKeyspaceIdsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(METHOD_EXECUTE_KEYSPACE_IDS, getCallOptions()), request, responseObserver);
}
/**
*
* ExecuteKeyRanges executes the query based on the specified key ranges.
* API group: Range-based Sharding
*
*/
public void executeKeyRanges(com.youtube.vitess.proto.Vtgate.ExecuteKeyRangesRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(METHOD_EXECUTE_KEY_RANGES, getCallOptions()), request, responseObserver);
}
/**
*
* ExecuteEntityIds executes the query based on the specified external id to keyspace id map.
* API group: Range-based Sharding
*
*/
public void executeEntityIds(com.youtube.vitess.proto.Vtgate.ExecuteEntityIdsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(METHOD_EXECUTE_ENTITY_IDS, getCallOptions()), request, responseObserver);
}
/**
*
* ExecuteBatch tries to route the list of queries on the right shards.
* It depends on the query and bind variables to provide enough
* information in conjonction with the vindexes to route the query.
* API group: v3 API
*
*/
public void executeBatch(com.youtube.vitess.proto.Vtgate.ExecuteBatchRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(METHOD_EXECUTE_BATCH, getCallOptions()), request, responseObserver);
}
/**
*
* ExecuteBatchShards executes the list of queries on the specified shards.
* API group: Custom Sharding
*
*/
public void executeBatchShards(com.youtube.vitess.proto.Vtgate.ExecuteBatchShardsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(METHOD_EXECUTE_BATCH_SHARDS, getCallOptions()), request, responseObserver);
}
/**
*
* ExecuteBatchKeyspaceIds executes the list of queries based on the specified keyspace ids.
* API group: Range-based Sharding
*
*/
public void executeBatchKeyspaceIds(com.youtube.vitess.proto.Vtgate.ExecuteBatchKeyspaceIdsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(METHOD_EXECUTE_BATCH_KEYSPACE_IDS, getCallOptions()), request, responseObserver);
}
/**
*
* StreamExecute executes a streaming query based on shards.
* It depends on the query and bind variables to provide enough
* information in conjonction with the vindexes to route the query.
* Use this method if the query returns a large number of rows.
* API group: v3 API (alpha)
*
*/
public void streamExecute(com.youtube.vitess.proto.Vtgate.StreamExecuteRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncServerStreamingCall(
getChannel().newCall(METHOD_STREAM_EXECUTE, getCallOptions()), request, responseObserver);
}
/**
*
* StreamExecuteShards executes a streaming query based on shards.
* Use this method if the query returns a large number of rows.
* API group: Custom Sharding
*
*/
public void streamExecuteShards(com.youtube.vitess.proto.Vtgate.StreamExecuteShardsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncServerStreamingCall(
getChannel().newCall(METHOD_STREAM_EXECUTE_SHARDS, getCallOptions()), request, responseObserver);
}
/**
*
* StreamExecuteKeyspaceIds executes a streaming query based on keyspace ids.
* Use this method if the query returns a large number of rows.
* API group: Range-based Sharding
*
*/
public void streamExecuteKeyspaceIds(com.youtube.vitess.proto.Vtgate.StreamExecuteKeyspaceIdsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncServerStreamingCall(
getChannel().newCall(METHOD_STREAM_EXECUTE_KEYSPACE_IDS, getCallOptions()), request, responseObserver);
}
/**
*
* StreamExecuteKeyRanges executes a streaming query based on key ranges.
* Use this method if the query returns a large number of rows.
* API group: Range-based Sharding
*
*/
public void streamExecuteKeyRanges(com.youtube.vitess.proto.Vtgate.StreamExecuteKeyRangesRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncServerStreamingCall(
getChannel().newCall(METHOD_STREAM_EXECUTE_KEY_RANGES, getCallOptions()), request, responseObserver);
}
/**
*
* Begin a transaction.
* API group: Transactions
*
*/
public void begin(com.youtube.vitess.proto.Vtgate.BeginRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(METHOD_BEGIN, getCallOptions()), request, responseObserver);
}
/**
*
* Commit a transaction.
* API group: Transactions
*
*/
public void commit(com.youtube.vitess.proto.Vtgate.CommitRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(METHOD_COMMIT, getCallOptions()), request, responseObserver);
}
/**
*
* Rollback a transaction.
* API group: Transactions
*
*/
public void rollback(com.youtube.vitess.proto.Vtgate.RollbackRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(METHOD_ROLLBACK, getCallOptions()), request, responseObserver);
}
/**
*
* ResolveTransaction resolves a transaction.
* API group: Transactions
*
*/
public void resolveTransaction(com.youtube.vitess.proto.Vtgate.ResolveTransactionRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(METHOD_RESOLVE_TRANSACTION, getCallOptions()), request, responseObserver);
}
/**
*
* MessageStream streams messages from a message table.
*
*/
public void messageStream(com.youtube.vitess.proto.Vtgate.MessageStreamRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncServerStreamingCall(
getChannel().newCall(METHOD_MESSAGE_STREAM, getCallOptions()), request, responseObserver);
}
/**
*
* MessageAck acks messages for a table.
*
*/
public void messageAck(com.youtube.vitess.proto.Vtgate.MessageAckRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(METHOD_MESSAGE_ACK, getCallOptions()), request, responseObserver);
}
/**
*
* Split a query into non-overlapping sub queries
* API group: Map Reduce
*
*/
public void splitQuery(com.youtube.vitess.proto.Vtgate.SplitQueryRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(METHOD_SPLIT_QUERY, getCallOptions()), request, responseObserver);
}
/**
*
* GetSrvKeyspace returns a SrvKeyspace object (as seen by this vtgate).
* This method is provided as a convenient way for clients to take a
* look at the sharding configuration for a Keyspace. Looking at the
* sharding information should not be used for routing queries (as the
* information may change, use the Execute calls for that).
* It is convenient for monitoring applications for instance, or if
* using custom sharding.
* API group: Topology
*
*/
public void getSrvKeyspace(com.youtube.vitess.proto.Vtgate.GetSrvKeyspaceRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(METHOD_GET_SRV_KEYSPACE, getCallOptions()), request, responseObserver);
}
/**
*
* UpdateStream asks the server for a stream of StreamEvent objects.
* API group: Update Stream
*
*/
public void updateStream(com.youtube.vitess.proto.Vtgate.UpdateStreamRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncServerStreamingCall(
getChannel().newCall(METHOD_UPDATE_STREAM, getCallOptions()), request, responseObserver);
}
}
/**
*
* Vitess is the main service to access a Vitess cluster. It is the API that vtgate
* exposes to serve all queries.
*
*/
public static final class VitessBlockingStub extends io.grpc.stub.AbstractStub {
private VitessBlockingStub(io.grpc.Channel channel) {
super(channel);
}
private VitessBlockingStub(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected VitessBlockingStub build(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
return new VitessBlockingStub(channel, callOptions);
}
/**
*
* Execute tries to route the query to the right shard.
* It depends on the query and bind variables to provide enough
* information in conjonction with the vindexes to route the query.
* API group: v3 API (alpha)
*
*/
public com.youtube.vitess.proto.Vtgate.ExecuteResponse execute(com.youtube.vitess.proto.Vtgate.ExecuteRequest request) {
return blockingUnaryCall(
getChannel(), METHOD_EXECUTE, getCallOptions(), request);
}
/**
*
* ExecuteShards executes the query on the specified shards.
* API group: Custom Sharding
*
*/
public com.youtube.vitess.proto.Vtgate.ExecuteShardsResponse executeShards(com.youtube.vitess.proto.Vtgate.ExecuteShardsRequest request) {
return blockingUnaryCall(
getChannel(), METHOD_EXECUTE_SHARDS, getCallOptions(), request);
}
/**
*
* ExecuteKeyspaceIds executes the query based on the specified keyspace ids.
* API group: Range-based Sharding
*
*/
public com.youtube.vitess.proto.Vtgate.ExecuteKeyspaceIdsResponse executeKeyspaceIds(com.youtube.vitess.proto.Vtgate.ExecuteKeyspaceIdsRequest request) {
return blockingUnaryCall(
getChannel(), METHOD_EXECUTE_KEYSPACE_IDS, getCallOptions(), request);
}
/**
*
* ExecuteKeyRanges executes the query based on the specified key ranges.
* API group: Range-based Sharding
*
*/
public com.youtube.vitess.proto.Vtgate.ExecuteKeyRangesResponse executeKeyRanges(com.youtube.vitess.proto.Vtgate.ExecuteKeyRangesRequest request) {
return blockingUnaryCall(
getChannel(), METHOD_EXECUTE_KEY_RANGES, getCallOptions(), request);
}
/**
*
* ExecuteEntityIds executes the query based on the specified external id to keyspace id map.
* API group: Range-based Sharding
*
*/
public com.youtube.vitess.proto.Vtgate.ExecuteEntityIdsResponse executeEntityIds(com.youtube.vitess.proto.Vtgate.ExecuteEntityIdsRequest request) {
return blockingUnaryCall(
getChannel(), METHOD_EXECUTE_ENTITY_IDS, getCallOptions(), request);
}
/**
*
* ExecuteBatch tries to route the list of queries on the right shards.
* It depends on the query and bind variables to provide enough
* information in conjonction with the vindexes to route the query.
* API group: v3 API
*
*/
public com.youtube.vitess.proto.Vtgate.ExecuteBatchResponse executeBatch(com.youtube.vitess.proto.Vtgate.ExecuteBatchRequest request) {
return blockingUnaryCall(
getChannel(), METHOD_EXECUTE_BATCH, getCallOptions(), request);
}
/**
*
* ExecuteBatchShards executes the list of queries on the specified shards.
* API group: Custom Sharding
*
*/
public com.youtube.vitess.proto.Vtgate.ExecuteBatchShardsResponse executeBatchShards(com.youtube.vitess.proto.Vtgate.ExecuteBatchShardsRequest request) {
return blockingUnaryCall(
getChannel(), METHOD_EXECUTE_BATCH_SHARDS, getCallOptions(), request);
}
/**
*
* ExecuteBatchKeyspaceIds executes the list of queries based on the specified keyspace ids.
* API group: Range-based Sharding
*
*/
public com.youtube.vitess.proto.Vtgate.ExecuteBatchKeyspaceIdsResponse executeBatchKeyspaceIds(com.youtube.vitess.proto.Vtgate.ExecuteBatchKeyspaceIdsRequest request) {
return blockingUnaryCall(
getChannel(), METHOD_EXECUTE_BATCH_KEYSPACE_IDS, getCallOptions(), request);
}
/**
*
* StreamExecute executes a streaming query based on shards.
* It depends on the query and bind variables to provide enough
* information in conjonction with the vindexes to route the query.
* Use this method if the query returns a large number of rows.
* API group: v3 API (alpha)
*
*/
public java.util.Iterator streamExecute(
com.youtube.vitess.proto.Vtgate.StreamExecuteRequest request) {
return blockingServerStreamingCall(
getChannel(), METHOD_STREAM_EXECUTE, getCallOptions(), request);
}
/**
*
* StreamExecuteShards executes a streaming query based on shards.
* Use this method if the query returns a large number of rows.
* API group: Custom Sharding
*
*/
public java.util.Iterator streamExecuteShards(
com.youtube.vitess.proto.Vtgate.StreamExecuteShardsRequest request) {
return blockingServerStreamingCall(
getChannel(), METHOD_STREAM_EXECUTE_SHARDS, getCallOptions(), request);
}
/**
*
* StreamExecuteKeyspaceIds executes a streaming query based on keyspace ids.
* Use this method if the query returns a large number of rows.
* API group: Range-based Sharding
*
*/
public java.util.Iterator streamExecuteKeyspaceIds(
com.youtube.vitess.proto.Vtgate.StreamExecuteKeyspaceIdsRequest request) {
return blockingServerStreamingCall(
getChannel(), METHOD_STREAM_EXECUTE_KEYSPACE_IDS, getCallOptions(), request);
}
/**
*
* StreamExecuteKeyRanges executes a streaming query based on key ranges.
* Use this method if the query returns a large number of rows.
* API group: Range-based Sharding
*
*/
public java.util.Iterator streamExecuteKeyRanges(
com.youtube.vitess.proto.Vtgate.StreamExecuteKeyRangesRequest request) {
return blockingServerStreamingCall(
getChannel(), METHOD_STREAM_EXECUTE_KEY_RANGES, getCallOptions(), request);
}
/**
*
* Begin a transaction.
* API group: Transactions
*
*/
public com.youtube.vitess.proto.Vtgate.BeginResponse begin(com.youtube.vitess.proto.Vtgate.BeginRequest request) {
return blockingUnaryCall(
getChannel(), METHOD_BEGIN, getCallOptions(), request);
}
/**
*
* Commit a transaction.
* API group: Transactions
*
*/
public com.youtube.vitess.proto.Vtgate.CommitResponse commit(com.youtube.vitess.proto.Vtgate.CommitRequest request) {
return blockingUnaryCall(
getChannel(), METHOD_COMMIT, getCallOptions(), request);
}
/**
*
* Rollback a transaction.
* API group: Transactions
*
*/
public com.youtube.vitess.proto.Vtgate.RollbackResponse rollback(com.youtube.vitess.proto.Vtgate.RollbackRequest request) {
return blockingUnaryCall(
getChannel(), METHOD_ROLLBACK, getCallOptions(), request);
}
/**
*
* ResolveTransaction resolves a transaction.
* API group: Transactions
*
*/
public com.youtube.vitess.proto.Vtgate.ResolveTransactionResponse resolveTransaction(com.youtube.vitess.proto.Vtgate.ResolveTransactionRequest request) {
return blockingUnaryCall(
getChannel(), METHOD_RESOLVE_TRANSACTION, getCallOptions(), request);
}
/**
*
* MessageStream streams messages from a message table.
*
*/
public java.util.Iterator messageStream(
com.youtube.vitess.proto.Vtgate.MessageStreamRequest request) {
return blockingServerStreamingCall(
getChannel(), METHOD_MESSAGE_STREAM, getCallOptions(), request);
}
/**
*
* MessageAck acks messages for a table.
*
*/
public com.youtube.vitess.proto.Query.MessageAckResponse messageAck(com.youtube.vitess.proto.Vtgate.MessageAckRequest request) {
return blockingUnaryCall(
getChannel(), METHOD_MESSAGE_ACK, getCallOptions(), request);
}
/**
*
* Split a query into non-overlapping sub queries
* API group: Map Reduce
*
*/
public com.youtube.vitess.proto.Vtgate.SplitQueryResponse splitQuery(com.youtube.vitess.proto.Vtgate.SplitQueryRequest request) {
return blockingUnaryCall(
getChannel(), METHOD_SPLIT_QUERY, getCallOptions(), request);
}
/**
*
* GetSrvKeyspace returns a SrvKeyspace object (as seen by this vtgate).
* This method is provided as a convenient way for clients to take a
* look at the sharding configuration for a Keyspace. Looking at the
* sharding information should not be used for routing queries (as the
* information may change, use the Execute calls for that).
* It is convenient for monitoring applications for instance, or if
* using custom sharding.
* API group: Topology
*
*/
public com.youtube.vitess.proto.Vtgate.GetSrvKeyspaceResponse getSrvKeyspace(com.youtube.vitess.proto.Vtgate.GetSrvKeyspaceRequest request) {
return blockingUnaryCall(
getChannel(), METHOD_GET_SRV_KEYSPACE, getCallOptions(), request);
}
/**
*
* UpdateStream asks the server for a stream of StreamEvent objects.
* API group: Update Stream
*
*/
public java.util.Iterator updateStream(
com.youtube.vitess.proto.Vtgate.UpdateStreamRequest request) {
return blockingServerStreamingCall(
getChannel(), METHOD_UPDATE_STREAM, getCallOptions(), request);
}
}
/**
*
* Vitess is the main service to access a Vitess cluster. It is the API that vtgate
* exposes to serve all queries.
*
*/
public static final class VitessFutureStub extends io.grpc.stub.AbstractStub {
private VitessFutureStub(io.grpc.Channel channel) {
super(channel);
}
private VitessFutureStub(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected VitessFutureStub build(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
return new VitessFutureStub(channel, callOptions);
}
/**
*
* Execute tries to route the query to the right shard.
* It depends on the query and bind variables to provide enough
* information in conjonction with the vindexes to route the query.
* API group: v3 API (alpha)
*
*/
public com.google.common.util.concurrent.ListenableFuture execute(
com.youtube.vitess.proto.Vtgate.ExecuteRequest request) {
return futureUnaryCall(
getChannel().newCall(METHOD_EXECUTE, getCallOptions()), request);
}
/**
*
* ExecuteShards executes the query on the specified shards.
* API group: Custom Sharding
*
*/
public com.google.common.util.concurrent.ListenableFuture executeShards(
com.youtube.vitess.proto.Vtgate.ExecuteShardsRequest request) {
return futureUnaryCall(
getChannel().newCall(METHOD_EXECUTE_SHARDS, getCallOptions()), request);
}
/**
*
* ExecuteKeyspaceIds executes the query based on the specified keyspace ids.
* API group: Range-based Sharding
*
*/
public com.google.common.util.concurrent.ListenableFuture executeKeyspaceIds(
com.youtube.vitess.proto.Vtgate.ExecuteKeyspaceIdsRequest request) {
return futureUnaryCall(
getChannel().newCall(METHOD_EXECUTE_KEYSPACE_IDS, getCallOptions()), request);
}
/**
*
* ExecuteKeyRanges executes the query based on the specified key ranges.
* API group: Range-based Sharding
*
*/
public com.google.common.util.concurrent.ListenableFuture executeKeyRanges(
com.youtube.vitess.proto.Vtgate.ExecuteKeyRangesRequest request) {
return futureUnaryCall(
getChannel().newCall(METHOD_EXECUTE_KEY_RANGES, getCallOptions()), request);
}
/**
*
* ExecuteEntityIds executes the query based on the specified external id to keyspace id map.
* API group: Range-based Sharding
*
*/
public com.google.common.util.concurrent.ListenableFuture executeEntityIds(
com.youtube.vitess.proto.Vtgate.ExecuteEntityIdsRequest request) {
return futureUnaryCall(
getChannel().newCall(METHOD_EXECUTE_ENTITY_IDS, getCallOptions()), request);
}
/**
*
* ExecuteBatch tries to route the list of queries on the right shards.
* It depends on the query and bind variables to provide enough
* information in conjonction with the vindexes to route the query.
* API group: v3 API
*
*/
public com.google.common.util.concurrent.ListenableFuture executeBatch(
com.youtube.vitess.proto.Vtgate.ExecuteBatchRequest request) {
return futureUnaryCall(
getChannel().newCall(METHOD_EXECUTE_BATCH, getCallOptions()), request);
}
/**
*
* ExecuteBatchShards executes the list of queries on the specified shards.
* API group: Custom Sharding
*
*/
public com.google.common.util.concurrent.ListenableFuture executeBatchShards(
com.youtube.vitess.proto.Vtgate.ExecuteBatchShardsRequest request) {
return futureUnaryCall(
getChannel().newCall(METHOD_EXECUTE_BATCH_SHARDS, getCallOptions()), request);
}
/**
*
* ExecuteBatchKeyspaceIds executes the list of queries based on the specified keyspace ids.
* API group: Range-based Sharding
*
*/
public com.google.common.util.concurrent.ListenableFuture executeBatchKeyspaceIds(
com.youtube.vitess.proto.Vtgate.ExecuteBatchKeyspaceIdsRequest request) {
return futureUnaryCall(
getChannel().newCall(METHOD_EXECUTE_BATCH_KEYSPACE_IDS, getCallOptions()), request);
}
/**
*
* Begin a transaction.
* API group: Transactions
*
*/
public com.google.common.util.concurrent.ListenableFuture begin(
com.youtube.vitess.proto.Vtgate.BeginRequest request) {
return futureUnaryCall(
getChannel().newCall(METHOD_BEGIN, getCallOptions()), request);
}
/**
*
* Commit a transaction.
* API group: Transactions
*
*/
public com.google.common.util.concurrent.ListenableFuture commit(
com.youtube.vitess.proto.Vtgate.CommitRequest request) {
return futureUnaryCall(
getChannel().newCall(METHOD_COMMIT, getCallOptions()), request);
}
/**
*
* Rollback a transaction.
* API group: Transactions
*
*/
public com.google.common.util.concurrent.ListenableFuture rollback(
com.youtube.vitess.proto.Vtgate.RollbackRequest request) {
return futureUnaryCall(
getChannel().newCall(METHOD_ROLLBACK, getCallOptions()), request);
}
/**
*
* ResolveTransaction resolves a transaction.
* API group: Transactions
*
*/
public com.google.common.util.concurrent.ListenableFuture resolveTransaction(
com.youtube.vitess.proto.Vtgate.ResolveTransactionRequest request) {
return futureUnaryCall(
getChannel().newCall(METHOD_RESOLVE_TRANSACTION, getCallOptions()), request);
}
/**
*
* MessageAck acks messages for a table.
*
*/
public com.google.common.util.concurrent.ListenableFuture messageAck(
com.youtube.vitess.proto.Vtgate.MessageAckRequest request) {
return futureUnaryCall(
getChannel().newCall(METHOD_MESSAGE_ACK, getCallOptions()), request);
}
/**
*
* Split a query into non-overlapping sub queries
* API group: Map Reduce
*
*/
public com.google.common.util.concurrent.ListenableFuture splitQuery(
com.youtube.vitess.proto.Vtgate.SplitQueryRequest request) {
return futureUnaryCall(
getChannel().newCall(METHOD_SPLIT_QUERY, getCallOptions()), request);
}
/**
*
* GetSrvKeyspace returns a SrvKeyspace object (as seen by this vtgate).
* This method is provided as a convenient way for clients to take a
* look at the sharding configuration for a Keyspace. Looking at the
* sharding information should not be used for routing queries (as the
* information may change, use the Execute calls for that).
* It is convenient for monitoring applications for instance, or if
* using custom sharding.
* API group: Topology
*
*/
public com.google.common.util.concurrent.ListenableFuture getSrvKeyspace(
com.youtube.vitess.proto.Vtgate.GetSrvKeyspaceRequest request) {
return futureUnaryCall(
getChannel().newCall(METHOD_GET_SRV_KEYSPACE, getCallOptions()), request);
}
}
private static final int METHODID_EXECUTE = 0;
private static final int METHODID_EXECUTE_SHARDS = 1;
private static final int METHODID_EXECUTE_KEYSPACE_IDS = 2;
private static final int METHODID_EXECUTE_KEY_RANGES = 3;
private static final int METHODID_EXECUTE_ENTITY_IDS = 4;
private static final int METHODID_EXECUTE_BATCH = 5;
private static final int METHODID_EXECUTE_BATCH_SHARDS = 6;
private static final int METHODID_EXECUTE_BATCH_KEYSPACE_IDS = 7;
private static final int METHODID_STREAM_EXECUTE = 8;
private static final int METHODID_STREAM_EXECUTE_SHARDS = 9;
private static final int METHODID_STREAM_EXECUTE_KEYSPACE_IDS = 10;
private static final int METHODID_STREAM_EXECUTE_KEY_RANGES = 11;
private static final int METHODID_BEGIN = 12;
private static final int METHODID_COMMIT = 13;
private static final int METHODID_ROLLBACK = 14;
private static final int METHODID_RESOLVE_TRANSACTION = 15;
private static final int METHODID_MESSAGE_STREAM = 16;
private static final int METHODID_MESSAGE_ACK = 17;
private static final int METHODID_SPLIT_QUERY = 18;
private static final int METHODID_GET_SRV_KEYSPACE = 19;
private static final int METHODID_UPDATE_STREAM = 20;
private static class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final VitessImplBase serviceImpl;
private final int methodId;
public MethodHandlers(VitessImplBase serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_EXECUTE:
serviceImpl.execute((com.youtube.vitess.proto.Vtgate.ExecuteRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_EXECUTE_SHARDS:
serviceImpl.executeShards((com.youtube.vitess.proto.Vtgate.ExecuteShardsRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_EXECUTE_KEYSPACE_IDS:
serviceImpl.executeKeyspaceIds((com.youtube.vitess.proto.Vtgate.ExecuteKeyspaceIdsRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_EXECUTE_KEY_RANGES:
serviceImpl.executeKeyRanges((com.youtube.vitess.proto.Vtgate.ExecuteKeyRangesRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_EXECUTE_ENTITY_IDS:
serviceImpl.executeEntityIds((com.youtube.vitess.proto.Vtgate.ExecuteEntityIdsRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_EXECUTE_BATCH:
serviceImpl.executeBatch((com.youtube.vitess.proto.Vtgate.ExecuteBatchRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_EXECUTE_BATCH_SHARDS:
serviceImpl.executeBatchShards((com.youtube.vitess.proto.Vtgate.ExecuteBatchShardsRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_EXECUTE_BATCH_KEYSPACE_IDS:
serviceImpl.executeBatchKeyspaceIds((com.youtube.vitess.proto.Vtgate.ExecuteBatchKeyspaceIdsRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_STREAM_EXECUTE:
serviceImpl.streamExecute((com.youtube.vitess.proto.Vtgate.StreamExecuteRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_STREAM_EXECUTE_SHARDS:
serviceImpl.streamExecuteShards((com.youtube.vitess.proto.Vtgate.StreamExecuteShardsRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_STREAM_EXECUTE_KEYSPACE_IDS:
serviceImpl.streamExecuteKeyspaceIds((com.youtube.vitess.proto.Vtgate.StreamExecuteKeyspaceIdsRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_STREAM_EXECUTE_KEY_RANGES:
serviceImpl.streamExecuteKeyRanges((com.youtube.vitess.proto.Vtgate.StreamExecuteKeyRangesRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_BEGIN:
serviceImpl.begin((com.youtube.vitess.proto.Vtgate.BeginRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_COMMIT:
serviceImpl.commit((com.youtube.vitess.proto.Vtgate.CommitRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_ROLLBACK:
serviceImpl.rollback((com.youtube.vitess.proto.Vtgate.RollbackRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_RESOLVE_TRANSACTION:
serviceImpl.resolveTransaction((com.youtube.vitess.proto.Vtgate.ResolveTransactionRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_MESSAGE_STREAM:
serviceImpl.messageStream((com.youtube.vitess.proto.Vtgate.MessageStreamRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_MESSAGE_ACK:
serviceImpl.messageAck((com.youtube.vitess.proto.Vtgate.MessageAckRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_SPLIT_QUERY:
serviceImpl.splitQuery((com.youtube.vitess.proto.Vtgate.SplitQueryRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_SRV_KEYSPACE:
serviceImpl.getSrvKeyspace((com.youtube.vitess.proto.Vtgate.GetSrvKeyspaceRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_UPDATE_STREAM:
serviceImpl.updateStream((com.youtube.vitess.proto.Vtgate.UpdateStreamRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new AssertionError();
}
}
}
private static final class VitessDescriptorSupplier implements io.grpc.protobuf.ProtoFileDescriptorSupplier {
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return com.youtube.vitess.proto.grpc.Vtgateservice.getDescriptor();
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (VitessGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new VitessDescriptorSupplier())
.addMethod(METHOD_EXECUTE)
.addMethod(METHOD_EXECUTE_SHARDS)
.addMethod(METHOD_EXECUTE_KEYSPACE_IDS)
.addMethod(METHOD_EXECUTE_KEY_RANGES)
.addMethod(METHOD_EXECUTE_ENTITY_IDS)
.addMethod(METHOD_EXECUTE_BATCH)
.addMethod(METHOD_EXECUTE_BATCH_SHARDS)
.addMethod(METHOD_EXECUTE_BATCH_KEYSPACE_IDS)
.addMethod(METHOD_STREAM_EXECUTE)
.addMethod(METHOD_STREAM_EXECUTE_SHARDS)
.addMethod(METHOD_STREAM_EXECUTE_KEYSPACE_IDS)
.addMethod(METHOD_STREAM_EXECUTE_KEY_RANGES)
.addMethod(METHOD_BEGIN)
.addMethod(METHOD_COMMIT)
.addMethod(METHOD_ROLLBACK)
.addMethod(METHOD_RESOLVE_TRANSACTION)
.addMethod(METHOD_MESSAGE_STREAM)
.addMethod(METHOD_MESSAGE_ACK)
.addMethod(METHOD_SPLIT_QUERY)
.addMethod(METHOD_GET_SRV_KEYSPACE)
.addMethod(METHOD_UPDATE_STREAM)
.build();
}
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy