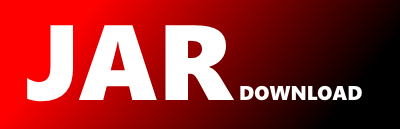
com.distelli.jackson.transform.WrappedObjectCodec Maven / Gradle / Ivy
package com.distelli.jackson.transform;
import com.fasterxml.jackson.core.ObjectCodec;
// Fix ObjectCodec.treeToValue() so a null TreeNode isn't a problem.
class WrappedObjectCodec extends ObjectCodec {
private ObjectCodec _codec;
public WrappedObjectCodec(ObjectCodec codec) {
_codec = codec;
}
// javap -cp ~/.m2/repository/com/fasterxml/jackson/core/jackson-core/2.7.5/jackson-core-2.7.5.jar com.fasterxml.jackson.core.ObjectCodec
@Override
public T readTree(com.fasterxml.jackson.core.JsonParser a) throws java.io.IOException, com.fasterxml.jackson.core.JsonProcessingException {
return _codec.readTree(a);
}
@Override
public void writeTree(com.fasterxml.jackson.core.JsonGenerator a, com.fasterxml.jackson.core.TreeNode b) throws java.io.IOException, com.fasterxml.jackson.core.JsonProcessingException {
_codec.writeTree(a, b);
}
@Override
public com.fasterxml.jackson.core.TreeNode createArrayNode() {
return _codec.createArrayNode();
}
@Override
public com.fasterxml.jackson.core.TreeNode createObjectNode() {
return _codec.createObjectNode();
}
@Override
public com.fasterxml.jackson.core.JsonParser treeAsTokens(com.fasterxml.jackson.core.TreeNode a) {
return _codec.treeAsTokens(a);
}
@Override
public com.fasterxml.jackson.core.Version version() {
return _codec.version();
}
@Override
public T readValue(com.fasterxml.jackson.core.JsonParser a, java.lang.Class b) throws java.io.IOException, com.fasterxml.jackson.core.JsonProcessingException {
return _codec.readValue(a, b);
}
@Override
public T readValue(com.fasterxml.jackson.core.JsonParser a, com.fasterxml.jackson.core.type.TypeReference> b) throws java.io.IOException, com.fasterxml.jackson.core.JsonProcessingException {
return _codec.readValue(a, b);
}
@Override
public T readValue(com.fasterxml.jackson.core.JsonParser a, com.fasterxml.jackson.core.type.ResolvedType b) throws java.io.IOException, com.fasterxml.jackson.core.JsonProcessingException {
return _codec.readValue(a, b);
}
@Override
public java.util.Iterator readValues(com.fasterxml.jackson.core.JsonParser a, java.lang.Class b) throws java.io.IOException, com.fasterxml.jackson.core.JsonProcessingException {
return _codec.readValues(a, b);
}
@Override
public java.util.Iterator readValues(com.fasterxml.jackson.core.JsonParser a, com.fasterxml.jackson.core.type.TypeReference> b) throws java.io.IOException, com.fasterxml.jackson.core.JsonProcessingException {
return _codec.readValues(a, b);
}
@Override
public java.util.Iterator readValues(com.fasterxml.jackson.core.JsonParser a, com.fasterxml.jackson.core.type.ResolvedType b) throws java.io.IOException, com.fasterxml.jackson.core.JsonProcessingException {
return _codec.readValues(a, b);
}
@Override
public void writeValue(com.fasterxml.jackson.core.JsonGenerator a, java.lang.Object b) throws java.io.IOException, com.fasterxml.jackson.core.JsonProcessingException {
_codec.writeValue(a, b);
}
@Override
public T treeToValue(com.fasterxml.jackson.core.TreeNode a, java.lang.Class b) throws com.fasterxml.jackson.core.JsonProcessingException {
if ( null == a || a.isMissingNode() ) return null;
return _codec.treeToValue(a, b);
}
@Override
public com.fasterxml.jackson.core.JsonFactory getJsonFactory() {
return _codec.getJsonFactory();
}
@Override
public com.fasterxml.jackson.core.JsonFactory getFactory() {
return _codec.getFactory();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy