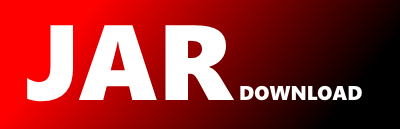
commonMain.com.ditchoom.mqtt3.persistence.ConnectionRequestQueries.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mqtt-4-models Show documentation
Show all versions of mqtt-4-models Show documentation
Defines the MQTT 3 and 4 control packets
package com.ditchoom.mqtt3.persistence
import app.cash.sqldelight.Query
import app.cash.sqldelight.TransacterImpl
import app.cash.sqldelight.db.QueryResult
import app.cash.sqldelight.db.SqlCursor
import app.cash.sqldelight.db.SqlDriver
import kotlin.Any
import kotlin.ByteArray
import kotlin.Long
import kotlin.String
import kotlin.Unit
public class ConnectionRequestQueries(
driver: SqlDriver,
) : TransacterImpl(driver) {
public fun connectionRequestByBrokerId(brokerId: Long, mapper: (
broker_id: Long,
protocol_name: String,
protocol_level: Long,
will_retain: Long,
will_qos: Long,
will_flag: Long,
clean_session: Long,
keep_alive_seconds: Long,
client_id: String,
will_topic: String?,
will_payload: ByteArray?,
username: String?,
password: String?,
) -> T): Query = ConnectionRequestByBrokerIdQuery(brokerId) { cursor ->
mapper(
cursor.getLong(0)!!,
cursor.getString(1)!!,
cursor.getLong(2)!!,
cursor.getLong(3)!!,
cursor.getLong(4)!!,
cursor.getLong(5)!!,
cursor.getLong(6)!!,
cursor.getLong(7)!!,
cursor.getString(8)!!,
cursor.getString(9),
cursor.getBytes(10),
cursor.getString(11),
cursor.getString(12)
)
}
public fun connectionRequestByBrokerId(brokerId: Long): Query =
connectionRequestByBrokerId(brokerId) { broker_id, protocol_name, protocol_level, will_retain,
will_qos, will_flag, clean_session, keep_alive_seconds, client_id, will_topic, will_payload,
username, password ->
ConnectionRequest(
broker_id,
protocol_name,
protocol_level,
will_retain,
will_qos,
will_flag,
clean_session,
keep_alive_seconds,
client_id,
will_topic,
will_payload,
username,
password
)
}
public fun insertConnectionRequest(
broker_id: Long,
protocol_name: String,
protocol_level: Long,
will_retain: Long,
will_qos: Long,
will_flag: Long,
clean_session: Long,
keep_alive_seconds: Long,
client_id: String,
will_topic: String?,
will_payload: ByteArray?,
username: String?,
password: String?,
): Unit {
driver.execute(-1592553957, """
|INSERT INTO ConnectionRequest
|(broker_id, protocol_name, protocol_level, will_retain, will_qos, will_flag, clean_session, keep_alive_seconds, client_id, will_topic, will_payload, username, password)
|VALUES
|(?, ?, ?, ?, ?, ?, ?, ?, ?, ?, ?, ?, ?)
""".trimMargin(), 13) {
bindLong(0, broker_id)
bindString(1, protocol_name)
bindLong(2, protocol_level)
bindLong(3, will_retain)
bindLong(4, will_qos)
bindLong(5, will_flag)
bindLong(6, clean_session)
bindLong(7, keep_alive_seconds)
bindString(8, client_id)
bindString(9, will_topic)
bindBytes(10, will_payload)
bindString(11, username)
bindString(12, password)
}
notifyQueries(-1592553957) { emit ->
emit("ConnectionRequest")
}
}
private inner class ConnectionRequestByBrokerIdQuery(
public val brokerId: Long,
mapper: (SqlCursor) -> T,
) : Query(mapper) {
public override fun addListener(listener: Query.Listener): Unit {
driver.addListener(listener, arrayOf("ConnectionRequest"))
}
public override fun removeListener(listener: Query.Listener): Unit {
driver.removeListener(listener, arrayOf("ConnectionRequest"))
}
public override fun execute(mapper: (SqlCursor) -> R): QueryResult =
driver.executeQuery(-691449473,
"""SELECT * FROM ConnectionRequest WHERE broker_id = ? LIMIT 1""", mapper, 1) {
bindLong(0, brokerId)
}
public override fun toString(): String = "ConnectionRequest.sq:connectionRequestByBrokerId"
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy