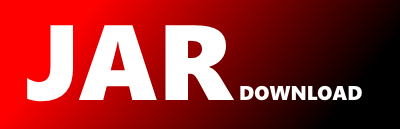
commonMain.com.ditchoom.mqtt3.persistence.QoS2MessagesQueries.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mqtt-4-models Show documentation
Show all versions of mqtt-4-models Show documentation
Defines the MQTT 3 and 4 control packets
package com.ditchoom.mqtt3.persistence
import app.cash.sqldelight.Query
import app.cash.sqldelight.TransacterImpl
import app.cash.sqldelight.db.QueryResult
import app.cash.sqldelight.db.SqlCursor
import app.cash.sqldelight.db.SqlDriver
import kotlin.Any
import kotlin.Long
import kotlin.String
import kotlin.Unit
public class QoS2MessagesQueries(
driver: SqlDriver,
) : TransacterImpl(driver) {
public fun queuedQos2Messages(brokerId: Long, mapper: (
broker_id: Long,
incoming: Long,
packet_id: Long,
type: Long,
) -> T): Query = QueuedQos2MessagesQuery(brokerId) { cursor ->
mapper(
cursor.getLong(0)!!,
cursor.getLong(1)!!,
cursor.getLong(2)!!,
cursor.getLong(3)!!
)
}
public fun queuedQos2Messages(brokerId: Long): Query =
queuedQos2Messages(brokerId) { broker_id, incoming, packet_id, type ->
Qos2Messages(
broker_id,
incoming,
packet_id,
type
)
}
public fun queuedMessageCount(brokerId: Long): Query = QueuedMessageCountQuery(brokerId) {
cursor ->
cursor.getLong(0)!!
}
public fun allMessages(brokerId: Long, mapper: (
broker_id: Long,
incoming: Long,
packet_id: Long,
type: Long,
) -> T): Query = AllMessagesQuery(brokerId) { cursor ->
mapper(
cursor.getLong(0)!!,
cursor.getLong(1)!!,
cursor.getLong(2)!!,
cursor.getLong(3)!!
)
}
public fun allMessages(brokerId: Long): Query = allMessages(brokerId) { broker_id,
incoming, packet_id, type ->
Qos2Messages(
broker_id,
incoming,
packet_id,
type
)
}
public fun insertQos2Message(
broker_id: Long,
incoming: Long,
packet_id: Long,
type: Long,
): Unit {
driver.execute(1487741400, """
|INSERT INTO Qos2Messages (broker_id, incoming, packet_id, type)
|VALUES (?, ?, ?, ?)
""".trimMargin(), 4) {
bindLong(0, broker_id)
bindLong(1, incoming)
bindLong(2, packet_id)
bindLong(3, type)
}
notifyQueries(1487741400) { emit ->
emit("Qos2Messages")
}
}
public fun updateQos2Message(
type: Long,
brokerId: Long,
incoming: Long,
packetId: Long,
): Unit {
driver.execute(-2112732728, """
|UPDATE Qos2Messages
|SET type = ?
|WHERE broker_id = ? AND incoming = ? AND packet_id = ?
""".trimMargin(), 4) {
bindLong(0, type)
bindLong(1, brokerId)
bindLong(2, incoming)
bindLong(3, packetId)
}
notifyQueries(-2112732728) { emit ->
emit("Qos2Messages")
}
}
public fun deleteQos2Message(
brokerId: Long,
incoming: Long,
packetId: Long,
): Unit {
driver.execute(-875007066,
"""DELETE FROM Qos2Messages WHERE broker_id = ? AND incoming = ? AND packet_id = ?""", 3) {
bindLong(0, brokerId)
bindLong(1, incoming)
bindLong(2, packetId)
}
notifyQueries(-875007066) { emit ->
emit("Qos2Messages")
}
}
public fun deleteAll(brokerId: Long): Unit {
driver.execute(462003389, """DELETE FROM Qos2Messages WHERE broker_id = ?""", 1) {
bindLong(0, brokerId)
}
notifyQueries(462003389) { emit ->
emit("Qos2Messages")
}
}
private inner class QueuedQos2MessagesQuery(
public val brokerId: Long,
mapper: (SqlCursor) -> T,
) : Query(mapper) {
public override fun addListener(listener: Query.Listener): Unit {
driver.addListener(listener, arrayOf("Qos2Messages"))
}
public override fun removeListener(listener: Query.Listener): Unit {
driver.removeListener(listener, arrayOf("Qos2Messages"))
}
public override fun execute(mapper: (SqlCursor) -> R): QueryResult =
driver.executeQuery(-42722987,
"""SELECT * FROM Qos2Messages WHERE broker_id = ? AND incoming = 0""", mapper, 1) {
bindLong(0, brokerId)
}
public override fun toString(): String = "QoS2Messages.sq:queuedQos2Messages"
}
private inner class QueuedMessageCountQuery(
public val brokerId: Long,
mapper: (SqlCursor) -> T,
) : Query(mapper) {
public override fun addListener(listener: Query.Listener): Unit {
driver.addListener(listener, arrayOf("Qos2Messages"))
}
public override fun removeListener(listener: Query.Listener): Unit {
driver.removeListener(listener, arrayOf("Qos2Messages"))
}
public override fun execute(mapper: (SqlCursor) -> R): QueryResult =
driver.executeQuery(536936820,
"""SELECT COUNT(broker_id) FROM Qos2Messages WHERE broker_id = ?""", mapper, 1) {
bindLong(0, brokerId)
}
public override fun toString(): String = "QoS2Messages.sq:queuedMessageCount"
}
private inner class AllMessagesQuery(
public val brokerId: Long,
mapper: (SqlCursor) -> T,
) : Query(mapper) {
public override fun addListener(listener: Query.Listener): Unit {
driver.addListener(listener, arrayOf("Qos2Messages"))
}
public override fun removeListener(listener: Query.Listener): Unit {
driver.removeListener(listener, arrayOf("Qos2Messages"))
}
public override fun execute(mapper: (SqlCursor) -> R): QueryResult =
driver.executeQuery(-1829823212, """SELECT * FROM Qos2Messages WHERE broker_id = ?""",
mapper, 1) {
bindLong(0, brokerId)
}
public override fun toString(): String = "QoS2Messages.sq:allMessages"
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy